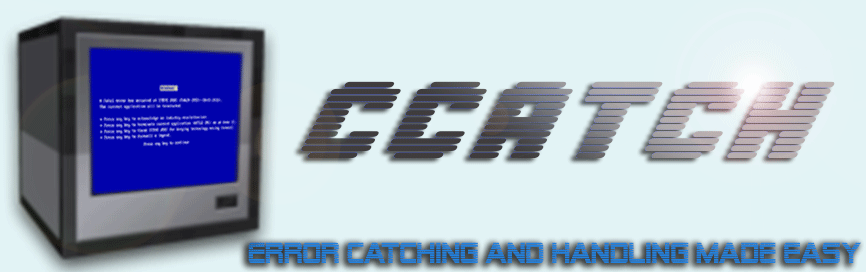
–Note! This API was inspired by [member='TheOriginalBIT'] 's Handling and BSoD tutorial found here
Features:
- Backwards compatible CC1.3+
- Functions for both Advanced Computers and Standard Computers
- Pre-designed error screens
- Custom error screens
- Custom crash handle functions
- Colour and non colour support
- Save error log to custom directory function
- C# style error catching function for C# style main functions
Spoiler
The Functions:Usage and Examples:
For this I will use the following code as an example program, the uses will be direct to this code as if I was calling the function in this program
os.loadAPI("CCrash")
local tArgs = {...}
term.clear()
local w,h = term.getSize()
local col = "a"
local main = function()
term.setBackgroundColor(col)
end
local tmpErr = {
"Custom Crash";
" ";
"Error Code";
":error: ";
" ";
"Please report this code to the dev if this is";
"no fault of your own";
" ";
" ";
"Please do refrain from modifying the base code!"
}
res = function()
col = 1
main()
end
main()
Now looking at that code you can see that it will error on line 8 with the error: 8 : Expected number, this is fine since I want it to error
You can also see that the main code is wrapped in a function titled "main", this is needed since the API can only check functions for errors, this is due to the pcall function that is used so just wrap you main code in a function, the function can be titled anything you want, just remember it.
Next you will see a table called tmpErr, this will be used in the Custom crash screens and is shown in here for example and demonstration purposes.
You will also see ":error:" in the table, this gets replaced with the error message and is needed to print the error message
Next is the function titled "res" this is a crash resolve function, this will be used to try recover an error by using a different function or different information.
Now that thats out of the way lets explain the usage of the functions, firstly lets explain the args:
func = The function you want to catch error in, your main function/main code wrapped in a function
resolvefunc = The function used to resolve an error without showing an error
align = text alignment on the crash screen - options("left","center")
bcol = Background colour in binary bits example - (white = 1, gray = 128, lightblue = 8)
tcol = Text colour in binary bits
t = Table to use for custom crash screens (contains custom text as shown above in tmpErr)
x = xPos to print "error log saved to" text on left align
y = yPos to print "error log saved to" text on left and center align
sDir = Directory to save the error log to
sPath = The name of the error log
argc = C# style arg count for main function
argv = C# stye args for main function
"(error)" = Used in custom tables to tell the API where to print the error message
Right now on to how to use it:First you need to wrap your main code/loop in a function as shown above then add one of the CCrash functions to the end of the code.
Advanced Computers(CC1.4+)
Spoiler
Quick pre-made crash screen
CCatch.A_crash(func,align,bcol,tcol)
Custom crash screen
CCatch.A_custCrash(func,align,bcol,tcol,t)
Custom crash resolve
CCatch.A_resolveCrash(func,resolvefunc)
Custom save error log
CCatch.A_saveCrash(func,align,bcol,tcol,t,x,y,sDir,sPath)
Custom crash for C# style functions
CCatch.A_c_Crash(func,#{...},{...},align,bcol,tcol,t)
Custom save error log + crash screen for C# style functions
CCatch.A_save_c_Crash(func,align,bcol,tcol,t,x,y,sDir,sPath,#{...},{...})
Normal Computers(CC1.3+)
Spoiler
Quick pre-made crash screen
CCatch.B_crash(func,align)
Custom crash screen
CCatch.B_custCrash(func,align,t)
Custom crash resolve
CCatch.B_resolveCrash(func,resolvefunc)
Custom save error log
CCatch.B_saveCrash(func,align,t,x,y,sDir,sPath)
Custom crash for C# style functions
CCatch.B_c_Crash(func,#{...},{...},align,t)
Custom save error log + crash screen for C# style functions
CCatch.A_save_c_Crash(func,align,t,x,y,sDir,sPath,#{...},{...})
Coming Soon:
- Custom logo printing and positioning
- Email error report
- From pastebin - http://pastebin.com/Q07qDtje
- In game using pastebin - pastebin get Q07qDtje CCrash