Spoiler
What is it?
cPrint is an API which was originally created as past of uGUI for my OS, but it is powerful enough to be an standalone tool.
It allows to change text and background colors inside of a string - like Minecraft's color codes!
How to use it?
Color coding:
To set text color: "&<color-code>"
To set background color: "$<color-code>"
To print "$" sign: Normal string: "\\$", Square bracket string: [[\$]]
To print "&" sign: Normal string: "\\&", Square bracket string: [[\&]]
Functions:
cprint.cprint(string)
Prints color parsed string.
Returns: nothing
cprint.cwrite(string)
Prints color parsed string.
Returns: nothing
cprint.update()
Updates cPrint and palette
Returns: true on update, false on no update.
cprint.toCode(int)
Changes int to it's string-hexadecimal form.
Returns: string
cprint.cCode(string)
Turns color code to color
Returns: int
cprint.clear([bool])
Clears terminal and if bool is not equal to false (or unset) sets cursor pos to 1, 1
cprint.sb(int)
Wrapper for term.setBackgroundColor()
cprint.st(int)
Wrapper for term.setTextColor()
cprint.sc(int, int)
Wrapper for term.setCursorPos()
Colors:
if code == "0" then return colors.white end
if code == "1" then return colors.orange end
if code == "2" then return colors.magenta end
if code == "3" then return colors.lightBlue end
if code == "4" then return colors.yellow end
if code == "5" then return colors.lime end
if code == "6" then return colors.pink end
if code == "7" then return colors.gray end
if code == "8" then return colors.lightGray end
if code == "9" then return colors.cyan end
if code == "a" then return colors.purple end
if code == "b" then return colors.blue end
if code == "c" then return colors.brown end
if code == "d" then return colors.green end
if code == "e" then return colors.red end
if code == "f" then return colors.black end
@up If you can't read it, it means you probably should look at the screenshot below :P/>
Versions:
Spoiler
cPrint:
--[[
Version log:
1.0:
* Initial release
1.1:
* Added cwrite
* Added update
* cprint now adds "\n" to the end of the line
* Improved cCode
* Improved toCode
* Auto updater
1.2:
* Added non-advanced computer support
* Bugfixes
1.3:
* Even better non-advanced computer support
]]--
Palette:
--[[
Version log:
1.0:
* Initial release
1.1:
* Ported to cPrint 1.1
]]--
Screenshot (animated, woo!):
From demo app (palette):
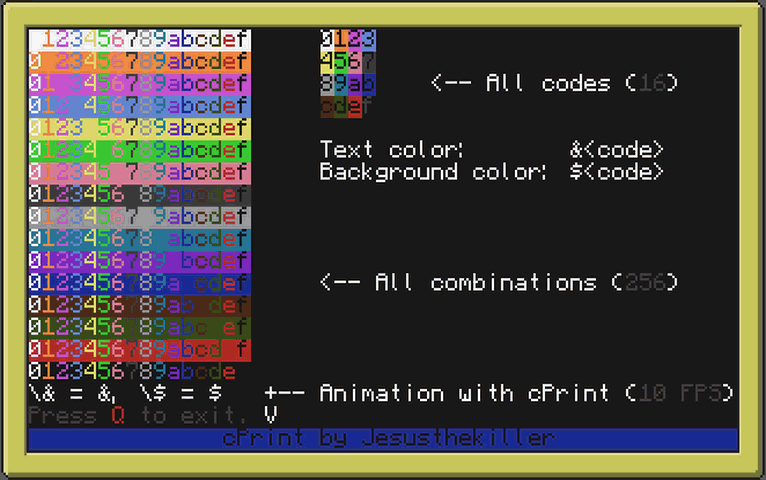
Download:
Pastebin:
- cPrint: pastebin get 2sxYu2Mq cprint
- Palette (demo app): pastebin get Q6vtbpf6 palette
- cPrint:
Spoiler
-- Do auto update?
local auto = true
--[[
###INFO###
* Language
Lua (ComputerCraft)
* Version
1.3
* Status
Working, not closed.
]]--
--[[
cPrint API by Jesusthekiller
This work is licensed under the Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License.
More info: http://creativecommons.org/licenses/by-nc-sa/3.0/deed.en_US
]]--
--[[
Big thanks to theorginalbit for help with cCode and toCode!
]]--
--[[
Version log:
1.0:
* Initial release
1.1:
* Added cwrite
* Added update
* cprint now adds "\n" to the end of the line
* Improved cCode
* Improved toCode
* Auto updater
1.2:
* Added non-advanced computer support
* Bugfixes
1.3:
* Even better non-advanced computer support
]]--
local DO_NOT_TOUCH = 5
-- Updater func:
function update()
if not http then
error("HTTP API required!")
end
cprint("&5Checking for &4cprint &5update...")
local v = http.get("http://mindblow.no-ip.org/code/cprint/ver")
if v == nil then
error("nil response from update server! Check your internet connection!")
end
if tonumber(v.readLine()) <= DO_NOT_TOUCH then
cprint("&5No new updates!")
return false
end
-- Install cPrint
cprint("&5Downloading &4cPrint&5...")
local f = http.get(v.readLine())
cprint("&5Installing &4cPrint&5 as &4cprint &5in main directory...")
local e = fs.open("cprint", "w") -- API Folder
e.write(f.readAll())
e.close()
f.close()
-- Install palette
cprint("&5Downloading &4Palette&5...")
local f = http.get(v.readLine())
v.close()
cprint("&5Installing &4Palette&5 as &4palette &5in main directory...")
local e = fs.open("palette", "w") -- API Folder
e.write(f.readAll())
e.close()
f.close()
v.close()
print("Done!")
return true
end
function sc(x, y)
term.setCursorPos(x, y)
end
function clear(move)
sb(colors.black)
term.clear()
if move ~= false then sc(1,1) end
end
function sb(color)
term.setBackgroundColor(color)
end
function st(color)
term.setTextColor(color)
end
function cCode(h)
if term.isColor() and term.isColor then
return 2 ^ (tonumber(h, 16) or 0)
else
if h == "f" then
return colors.black
else
return colors.white
end
end
end
function toCode(n)
return string.format('%x', n)
end
function cwrite(text)
text = tostring(text)
local i = 0
while true do
i = i + 1
if i > #text then break end
local c = text:sub(i, i)
if c == "\\" then
if text:sub(i+1, i+1) == "&" then
write("&")
i = i + 1
elseif text:sub(i+1, i+1) == "$" then
write("$")
i = i + 1
else
write(c)
end
elseif c == "&" then
st(cCode(text:sub(i+1, i+1)))
i = i + 1
elseif c == "$" then
sb(cCode(text:sub(i+1, i+1)))
i = i + 1
else
write(c)
end
end
return
end
function cprint(text)
return cwrite(tostring(text).."\n")
end
if auto then update() end
- Palette (demo app)
Spoiler
--[[
###INFO###
* Language
Lua (ComputerCraft)
* Version
1.1
* Status
Working, not closed.
]]--
--[[
Palette program by Jesusthekiller
This work is licensed under the Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License.
More info: http://creativecommons.org/licenses/by-nc-sa/3.0/deed.en_US
]]--
--[[
Version log:
1.0:
* Initial release
1.1:
* Ported to cPrint 1.1
]]--
if not fs.exists('cprint') then
error('No cprint API in main directory')
end
os.unloadAPI('cprint')
os.loadAPI('cprint')
cprint.clear()
cprint.cwrite([[
$0&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&f $00$11$22$33
$1&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&f $44$55$66$77
$2&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&f $88$99$aa$bb$f &0 <-- All codes (&716&0)
$3&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&f $cc$dd$ee$f&7f
$4&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$5&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&0 Text color: \&<code>
$6&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&0 Background color: \$<code>
$7&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$8&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$9&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$a&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$b&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff$f&0 <-- All combinations (&7256&0)
$c&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$d&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$e&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$f&00&11&22&33&44&55&66&77&88&99&aa&bb&cc&dd&ee&ff
$f&0\\& = \&, \\$ = \$ $f&0+-- Animation with cPrint (&710 FPS&0)
&7Press &eQ &7to exit. $f&0V]])
local function wait()
local e, k = nil
while k ~= "q" do
e, k = os.pullEvent("char")
end
end
local function cols()
local x, y = term.getSize()
while true do
for i = 0, 14 do
cprint.sc(1, y)
cprint.cwrite("$"..cprint.toCode(i).."&f cPrint by Jesusthekiller ")
sleep(0.1)
end
end
end
parallel.waitForAny(wait, cols)
License:
Spoiler
THIS SOFTWARE IS PROVIDED "AS IS" AND ANY EXPRESSED OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.This work is licensed under the Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License. Copy of this license.
Spoiler
What is it?:
WCON is an API (but not only) which allows you to download and upload data to WCON server.
You have 6 boolean registers (for redstone states) and 5 string registers (up to 5119 characters).
You can change all registers from in-game computer or from online control panel.
Note: HTTP API required.
How to get started?:
Get your software on your computer:
- Run "pastebin get jQLjRUnt install"
- Run "install"
- Hook up some redstone or lamps
- Run "launch" in main directory.
- Login into control panel.
- Change redstone states and see what happens!
Spoiler
Version 2:- Added auto updater
- Changed how stuff is located
- Added getVersion() function
- BETTER INSTALLER! YAAAY
- Better password hashing on server side.
- Initial release
API reference:
Spoiler
sendData(string state, int registry):Sets given string registry to state.
Returns false at wrong registry, nil at communication error, true at success.
sendRsData(bool state, string side):
Sets given redstone registry to state.
Returns false at wrong side string, nil at communication error, true at success.
getData():
Gets string registers table.
Returns:
nil at server communication error,
"fatal: [ERROR CODE]" at WCON_FATAL_ERROR,
table (5 element, 1 to 5) at success.
getRsData():
Gets redstone registers table.
Returns:
nil at server communication error,
"fatal: [ERROR CODE]" at WCON_FATAL_ERROR,
table (6 element, 1 to 6) at success.
Each element in table corresponds to one side:
1 = top
2 = bottom
3 = right
4 = left
5 = back
6 = front
getSide(int side):
Converts side in int into side in string.
getServer():
Returns server address.
getId():
Returns ID.
getPassword():
Returns password.
getVersion():
Returns Version.
About security:
Spoiler
Passwords are encrypted with SHA-256, than encrypted with SHA-256 again. It means it's basically impossible to crack password. It also means that no one can see passwords, even me.They are stored in MySQL Database (with randomly generated password), which allows only localhost connections, not in a plain text file.
Screenshots (control pannel):
Spoiler
Homepage: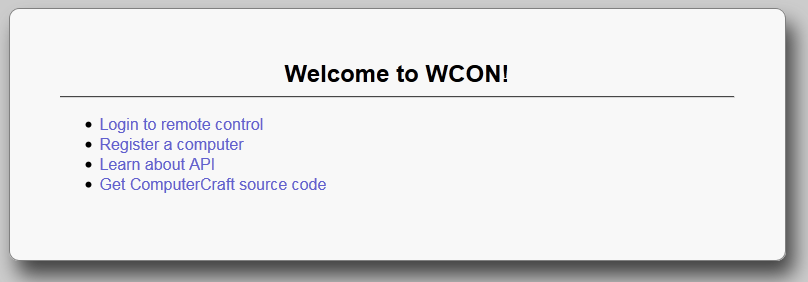
Login:
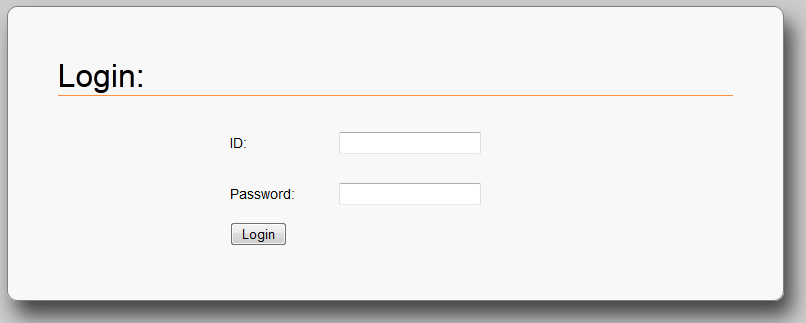
Control panel:
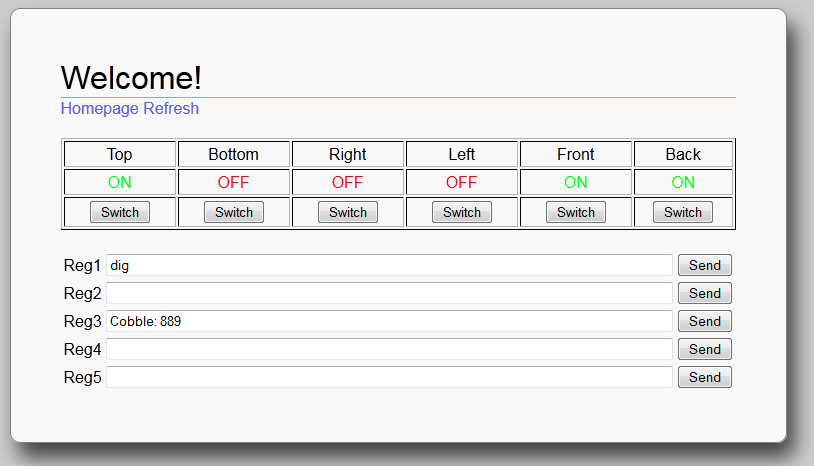
Register:
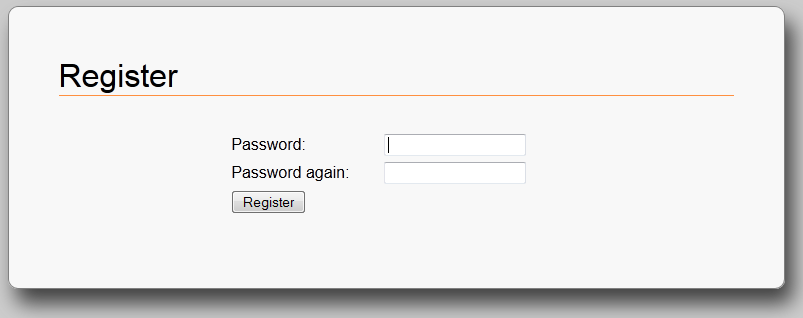
Registration complete:
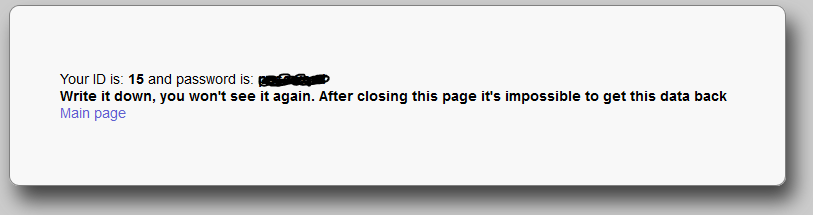
Legal notice:
Spoiler
THIS SOFTWARE IS PROVIDED "AS IS" AND ANY EXPRESSED OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.This work is licensed under the Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License. Copy of this license.
Spoiler
I've quickly wrote this app after seeing this thread - I've thought this idea can be developed a bit more :)/>
What is does:
It basically logs apps and saves it to "debug_files/<app name>.log".
How to use:
Usage:
- debug run <program full path> [args]
Logs are saves in debug_files/<program name>.log
- debug log <program name>
Prints a log
- debug restore
Use if program was terminated or to manually restore write & print functions
Where to get it:
- pastebin get 9YSN2Mht debug
- Code:
Spoiler
local args = {...}
--[[
Backup for old functions
]]--
local fOld = {
term = {
write = term.write,
clear = term.clear,
setCursorPos = term.setCursorPos,
clearLine = term.clearLine,
},
print = print,
write = write,
read = read,
}
local function addlog(t, i)
if i == false then fLog.write(t)
else fLog.write(t.."\n") end
fLog.flush()
end
local function openlog()
fLog = fs.open(debug_path, "w") -- Global...
end
local function closelog()
fLog.close()
fLog = nil
end
local function restore()
term.write = fOld.term.write
term.clear = fOld.term.clear
term.setCursorPos = fOld.term.setCursorPos
term.clearLine = fOld.term.clearLine
print = fOld.print
write = fOld.write
closelog()
debug_path = nil
fs.delete("debug_files/.launch")
print("\n\n\nDebbuging finished!")
end
local function mkfunc()
-- Open log
openlog()
--[[
All global override functions go here.
]]--
function term.writeNew(t)
addlog(t)
return fOld.term.write(t)
end
term.write = term.writeNew
function read(t)
term.write = fOld.term.write
local r
if t == nil then
r = fOld.read()
else
r = fOld.read(t)
end
term.write = term.writeNew
addlog("[read("..tostring(t)..") = "..tostring(r).."]")
return r
end
function print(t)
local r = fOld.print(t)
addlog("[print("..tostring(t)..") = "..tostring(r).."]")
return r
end
function write(t)
local r = fOld.write(t)
addlog("[write("..tostring(t)..") = "..tostring(r).."]")
return r
end
function term.clear()
local r = fOld.term.clear()
addlog("[term.clear() = "..tostring(r).."]")
return r
end
function term.setCursorPos(a, B)/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>
local r = fOld.term.setCursorPos(a, B)/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>
addlog("[term.setCursorPos("..tostring(a)..", "..tostring(B)/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>/>..") = "..tostring(r).."]")
return r
end
function term.clearLine()
local r = fOld.term.clearLine()
addlog("[term.clearLine() = "..tostring(r).."]")
return r
end
end
if args[1] == "run" then
debug_path = "debug_files/"..args[2]..".log" --Globalize args[2]
term.clear()
term.setCursorPos(1, 1)
print("Debugging "..args[2]..".\n\nDo not terminate program, if you do, run \"debug restore\" to restore functions.\n\nPress any key to continue")
local e = nil
while(e ~= "char") do e = os.pullEvent("char") end --Wait for key
term.clear()
term.setCursorPos(1, 1)
if(not fs.exists("debug_files")) then fs.makeDir("debug_files") end -- Check & make "debug" dir
mkfunc()
local l = fs.open("debug_files/.launch", "w") -- Open temp launch file
local s = "shell.run(\""..args[2].."\""-- Temp string
for i = 3, #args do
s = s..", \""..args[i].."\""
end
s = s..")"
l.write(s)
l.close()
shell.run("debug_files/.launch") --Run app
restore() -- Restore old functions
return
end
if args[1] == "log" then
if(not fs.exists("debug_files/"..args[2]..".log")) then print("No such file!") return end
local f = fs.open("debug_files/"..args[2]..".log", "r") -- Open and print
print(f.readAll())
f.close()
return
end
if args[1] == "restore" then
restore() -- Restore
return
end
print([[
Usage:
- debug run <program full path> [args]
Logs are saves in debug_files/<program name>.log
- debug log <program name>
Prints a log
- debug restore
Use if program was terminated or to manually restore write & print functions
Debug by jesysthekiller, idea from urielsalis
Version 1.1
]])
Spoiler
- 1.0: Initial release
- 1.1: Logging part of term.*, read, write, print
License:
Spoiler
THIS SOFTWARE IS PROVIDED "AS IS" AND ANY EXPRESSED OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.This work is licensed under the Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License. Copy of this license.
Spoiler
function makeString(l)
if l < 1 then return nil end -- Check for l < 1
local s = "" -- Start string
for i = 1, l do
s = s .. string.char(math.random(32, 126)) -- Generate random number from 32 to 126, turn it into character and add to string
end
return s -- Return string
end
print("Guess the number! (1 - 100)")
local num = math.random(1, 100), g
while true do
local g = tonumber(read())
if type(g) == "number" then
if g > num then print("Incorrect! Number is smaller") elseif g < num then print("Incorrect! Number is bigger") elseif g == num then break end
else
print("This is not a number!")
end
end
print("Congratulations!")
local f = fs.open("o", "w")
local function q(t, a) – Make list of table t with prefix a in file
for k, v in pairs(t) do
f.writeLine(a.."."..k..": "..type(v)) – Write to file
f.flush() – Flush it
if type(v) == "number" then
f.writeLine(" "..v) – Write number's value to file
elseif type(v) == "table" and k ~= "_G" then
q(v, a.."."..k) – Call itself if it's table and it's not _G
end
end
end
q(_G, "_G")
f.close()
print("Saved to file 'o'")
- Pacman - Package manager for Computer Craft. Website
- linuxOS - Goal: Bring linux experience to CC!