Posted 31 May 2013 - 04:19 PM
Button API:
Features:
- Color changes on clicks
- Clicks handling
- Single use buttons
- Synchronous callback
- Asynchronous callback
Usage:
makeButton(localtionArray, buttonTypeArray, callback, [callback_pressed])
locationArray:
Array, has to contain those keys:
* xstart
* ystart
* xstop
* ystop
buttonTypeArray:
Array, has to contain those keys:
* color_bkg
* click_color_bkg
* color_text
* click_color_text
* text
* textStartPosX
* textStartPosY
* event_value
callback:
Function with code to be run in parallel with makeButton
Button will terminate if this code ends.
callback_pressed:
Optional, function to be run when button is pressed.
Do not make it infinite (it will freeze button pressed and unclickable until finished)
makeButton will create "button" event with event_value as 1st value when button will be pressed.
makeSingleUseButton(localtionArray, buttonTypeArray, animationTime, callback, [callback_pressed])
localtionArray, buttonTypeArray, callback, callback_pressed same as in makeButton
animatonTime:
Controls for how long button has to be pressed after clicked. If set to >= 0 then animation will be disabled.
callback_pressed is executed after animation, not in parallel with it.
removeButton(event_value)
Removes button
!!By default this API will print to Terminal, if you want monitor output, do term.redirect()!!
Demo code:
makeButton:
os.unloadAPI("button")
os.loadAPI("button")
button.makeButton({["startx"] = 2, ["starty"] = 2, ["stopx"] = 4, ["stopy"] = 5},
{["color_bkg"] = colors.lime, ["color_text"] = colors.white, ["text"] = "lol", ["textStartPosX"] = 2, ["textStartPosY"] = 3, ["click_color_text"] = colors.black, ["click_color_bkg"] = colors.green, ["event_value"] = "lol"},
function() while true do local e, k = os.pullEvent("key"); if k == keys.q then button.removeButton("lol"); return end end end,
function() term.setTextColor(colors.white); term.setBackgroundColor(colors.black); print("Click!") end)
Pastebin: 55UqSQxL
Note: Press Q to exit program.
makeSingleUseButton:
os.unloadAPI("button")
os.loadAPI("button")
button.makeSingleUseButton({["startx"] = 2, ["starty"] = 2, ["stopx"] = 4, ["stopy"] = 5},
{["color_bkg"] = colors.lime, ["color_text"] = colors.white, ["text"] = "lol", ["textStartPosX"] = 2, ["textStartPosY"] = 3, ["click_color_text"] = colors.black, ["click_color_bkg"] = colors.green, ["event_value"] = "lol"},
1,
function() while true do local e, k = os.pullEvent("key"); if k == keys.q then button.removeButton("lol"); return end end end,
function() term.setTextColor(colors.white); term.setBackgroundColor(colors.black); print("Click!"); end)
Pastebin: Q23HJ70u
Note: Press Q to exit program.
Download:
pastebin get fHA8k25J button
pastebin get 0r9k5qUT license
Screenshot (Demo app):
Spoiler
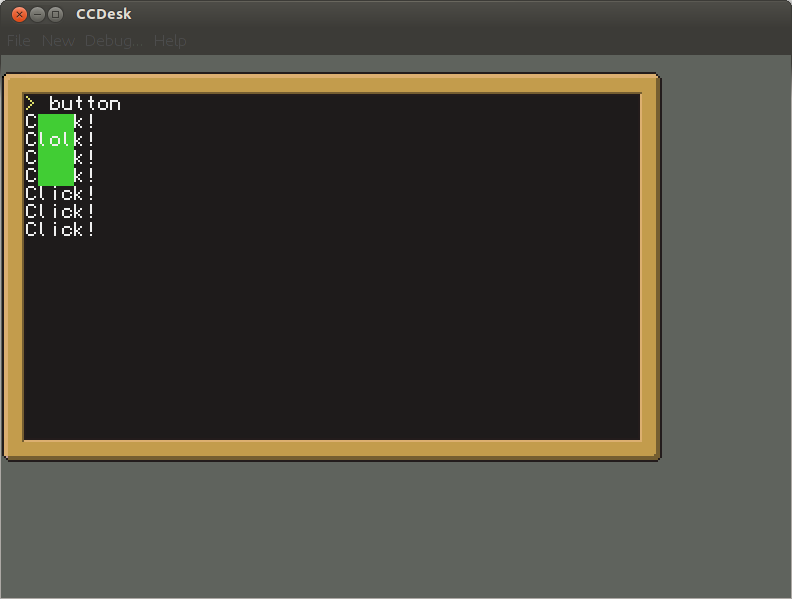
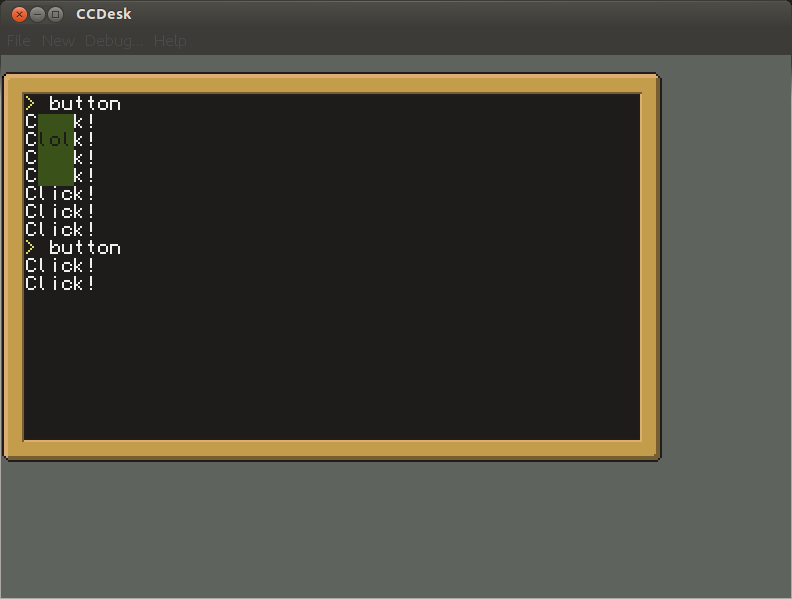
License (GNU GPL):
http://www.jesusthek...icense-gpl.html
Spoiler
Button API
Copyright © 2013 Jesusthekiller
This program is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with this program. If not, see <http://www.gnu.org/licenses/>.