Posted 27 April 2012 - 02:32 AM
This is my first program I will be putting on the website.
Using BuildCraft, this program is able to play music discs from a chest, and can automatically switch to a different random disc at any time.
You can manually tell the program to play/stop/switch discs, or you can use an AutoPlay feature that will automatically switch to a new disc every so often. This is nice if you want various songs playing in the background automatically.
Screenshots:
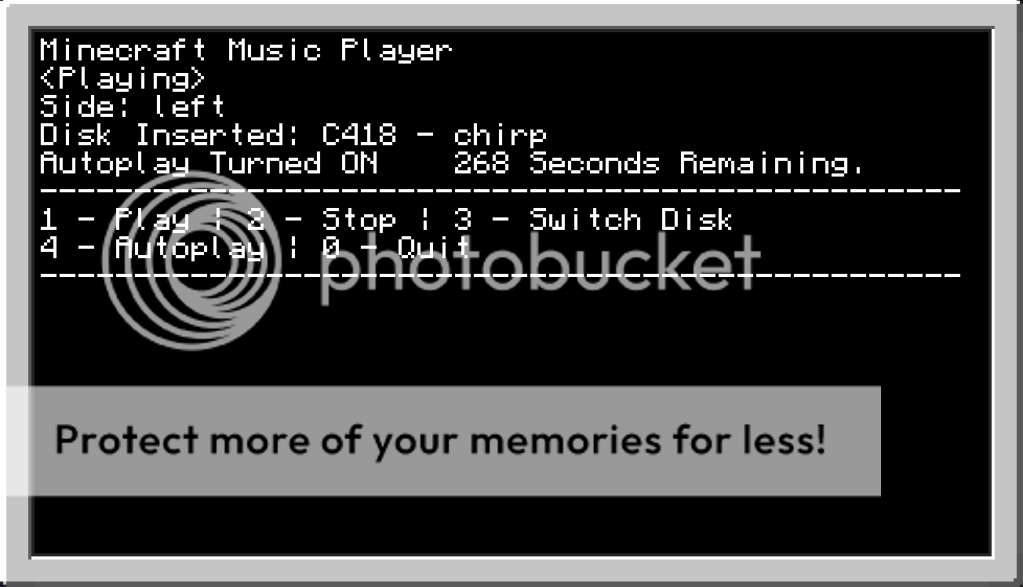
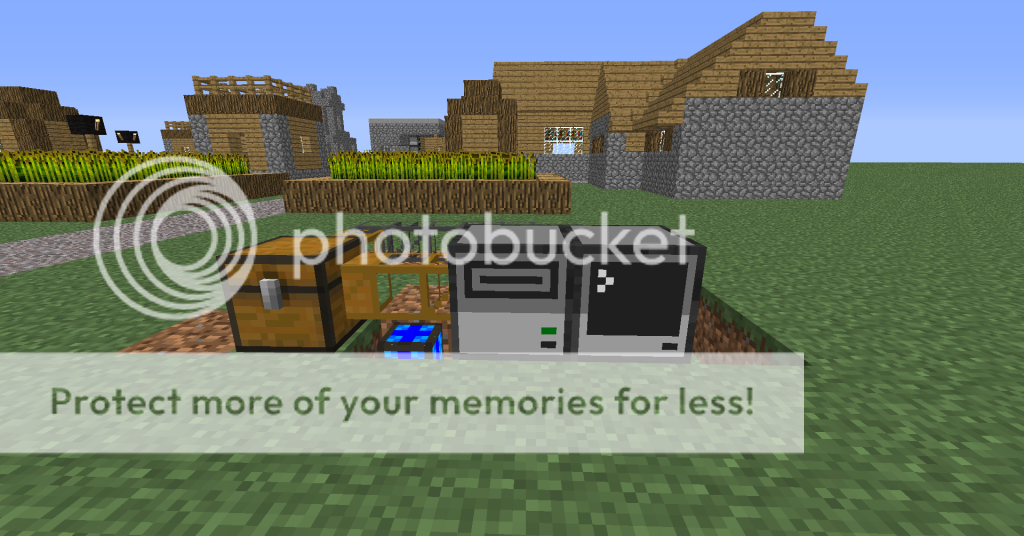
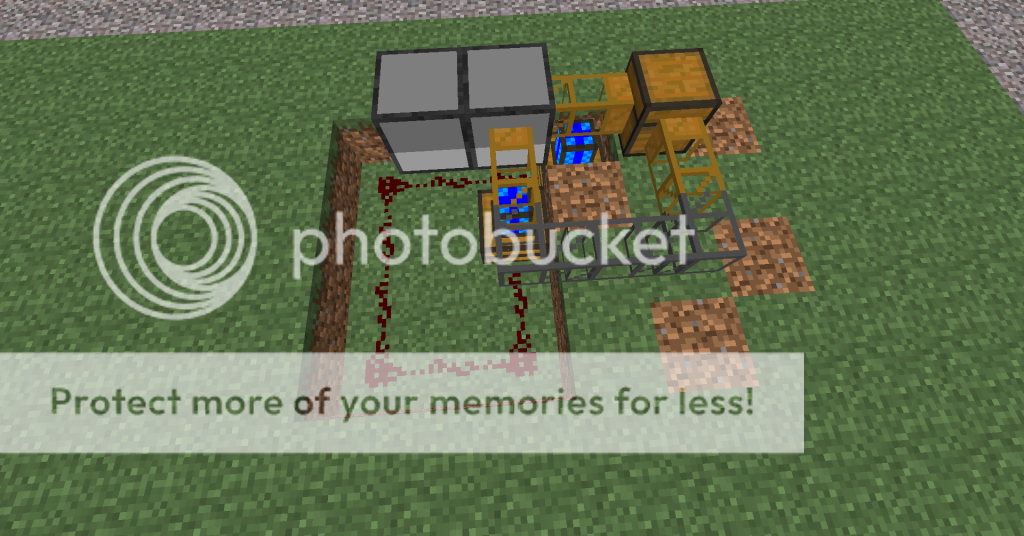
Code:
How to Set Up:
(See Pictures)
Place your computer and a disk drive next to it. Then place a chest next to the disk drive with a wooden pipe between them. Place a wooden pipe behind the chest and behind the disk drive, and then connect the wooden pipes with cobblestone pipes. You then need to place a redstone engine beneath both wooden pipes connected to the disk drive (one powers a disc in, the other powers a disc out). Place redstone directly underneath the computer (the program outputs redstone through the bottom), and connect the redstone to both engines. If you did this corectly, turning the redstone on should power both the the redstone engines, which pipes things in/out of the disk drive. Then just place your music discs in the chest.
Known Issues:
Unfortunately BuildCraft pipes take the item in the very first slot out of the chest, meaning things are not taken out entirely "randomly." This will lead to only a few discs ending up being cycled through. To solve this you may be able to set up a more sophisticated machine to allow more variety.
If you find any problems or have any suggestions feel free to say them here.
Using BuildCraft, this program is able to play music discs from a chest, and can automatically switch to a different random disc at any time.
You can manually tell the program to play/stop/switch discs, or you can use an AutoPlay feature that will automatically switch to a new disc every so often. This is nice if you want various songs playing in the background automatically.
Screenshots:
Spoiler
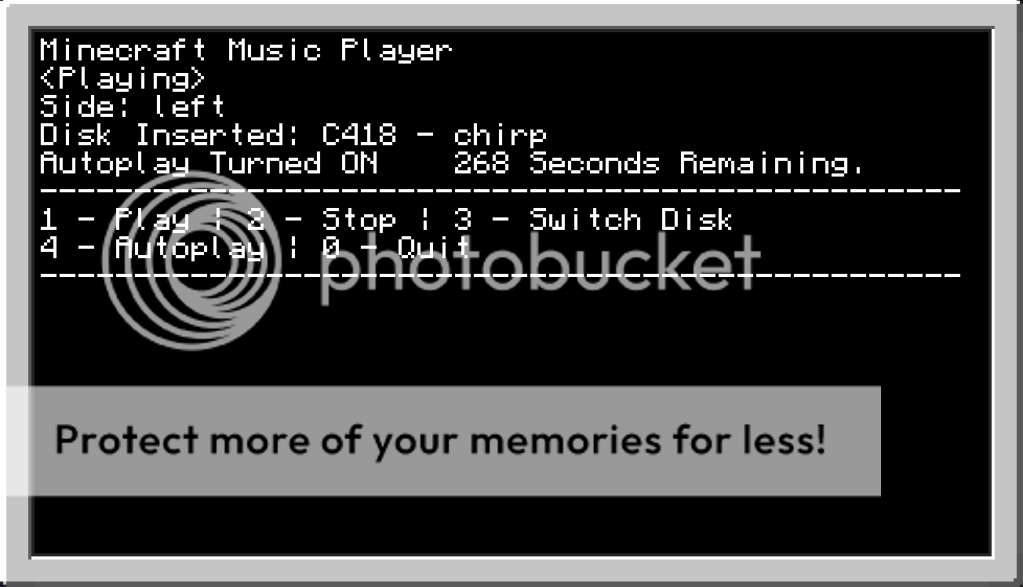
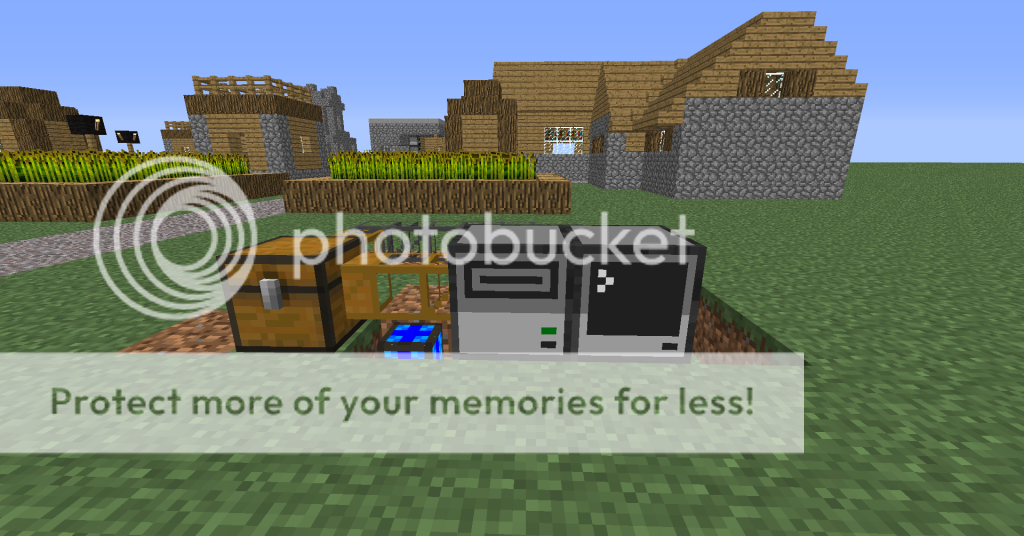
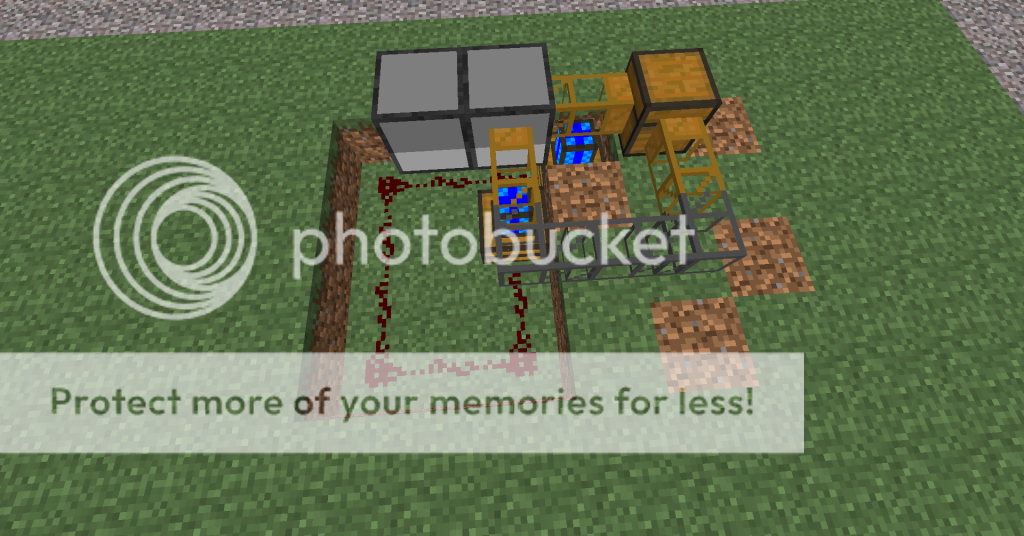
Code:
Spoiler
--------------------------
-- Minecraft Music Player with BuildCraft
-- By: Negeator, 2012
--------------------------
-- When set up correcty, this program can automatically play and switch music discs without the player touching the disk drive.
--------------------------
--Variables--
--DO NOT MODIFY THESE--
local side, title, time
local state = "none" --What the media player is currently doing
local stateView = "" --What to display
local quit = false --Whether to exit the program or not
local timing = false --Whether the program is timing (Autoplay0
local currTime = 0 --Current Time
local visual = 0 --Visualization state variable
--MODIFY THESE IF YOU WANT--
local timeAmount = 300 --How long (in seconds) until we switch disks for Autoplay
--Check for a disk in
function checkSide()
if disk.isPresent("top") == true and disk.hasAudio("top") == true then
side = "top"
elseif disk.isPresent("bottom") == true and disk.hasAudio("bottom") == true then
side = "bottom"
elseif disk.isPresent("left") == true and disk.hasAudio("left") == true then
side = "left"
elseif disk.isPresent("right") == true and disk.hasAudio("right") == true then
side = "right"
elseif disk.isPresent("front") == true and disk.hasAudio("front") == true then
side = "front"
elseif disk.isPresent("back") == true and disk.hasAudio("back") == true then
side = "back"
else
side = "No Disc Found"
end
end
--Clears the Screen
function clear()
term.clear()
term.setCursorPos(1,1)
end
--Display
function printInfo()
term.write("Minecraft Music Player")
term.setCursorPos(1,2)
term.write(stateView)
term.setCursorPos(1,3)
term.write("Side: " .. side)
if side ~= "No Disc Found" and state ~= "play" then
if disk.hasAudio(side) then
term.setCursorPos(1,4)
term.write("Disk Inserted: "..disk.getAudioTitle(side))
end
end
term.setCursorPos(1,5)
if timing == true then
term.write("Autoplay Turned ON "..timeAmount - currTime.." Seconds Remaining.")
else
term.write("Autoplay Turned OFF")
end
term.setCursorPos(1,6)
term.write("-------------------------------------------------")
term.setCursorPos(1,7)
term.write("1 - Play | 2 - Stop | 3 - Switch Disk")
term.setCursorPos(1,8)
term.write("4 - Autoplay | 0 - Quit ")
term.setCursorPos(1,9)
term.write("-------------------------------------------------")
term.setCursorPos(1,10)
end
--Does stuff based on the state variable
function musicControl()
--Play the disk automatically if in Autoplay mode
if timing == true and state ~= "stop" then
if state ~= "play" then
state = "play"
end
end
if state == "play" then
if side ~= "No Disc Found" then
if stateView ~= "<Playing>" then
disk.playAudio(side)
stateView = "<Playing>"
end
end
elseif state == "stop" then
if side ~= "No Disc Found" then
timing = false
disk.stopAudio(side)
stateView = "<Stopped>"
end
end
--If no disc is present, change the display
if side == "No Disc Found" then
stateView = "<INSERT DISC>"
end
--Make sure it displays as stopped when a new disc is just inserted
if stateView == "<INSERT DISC" and side ~= "No Disc Found" then
stateView = "<Stopped>"
end
state = ""
end
--Switch Disks
function cycleDisk()
clear()
term.write("Switching Disk")
rs.setOutput("bottom",true)
sleep(1)
rs.setOutput("bottom",false)
if timing == true then
os.startTimer(9) --Wait for new Disc
currTime = 0
end
end
--Updates the timer
function updateTimer()
if timing == true then
currTime = currTime + 1
if currTime >= timeAmount then
currTime = 0
cycleDisk()
os.startTimer(9) --Wait for new Disc
else
os.startTimer(1)
end
end
end
--Main Function
function main()
while quit == false do
clear()
checkSide()
musicControl()
printInfo()
event, p1, p2 = os.pullEvent()
if event == "key" then
if p1 == 2 then
state = "play"
elseif p1 == 3 then
state = "stop"
elseif p1 == 4 then
cycleDisk()
elseif p1 == 5 then
if timing == true then
timing = false
else
timing = true
currTime = 0
os.startTimer(1)
end
sleep(.25)
elseif p1 == 11 then
clear()
quit = true
end
elseif event == "timer" then
updateTimer()
end
end
end
--START PROGRAM--
main()
--END PROGRAM--
How to Set Up:
(See Pictures)
Place your computer and a disk drive next to it. Then place a chest next to the disk drive with a wooden pipe between them. Place a wooden pipe behind the chest and behind the disk drive, and then connect the wooden pipes with cobblestone pipes. You then need to place a redstone engine beneath both wooden pipes connected to the disk drive (one powers a disc in, the other powers a disc out). Place redstone directly underneath the computer (the program outputs redstone through the bottom), and connect the redstone to both engines. If you did this corectly, turning the redstone on should power both the the redstone engines, which pipes things in/out of the disk drive. Then just place your music discs in the chest.
Known Issues:
Unfortunately BuildCraft pipes take the item in the very first slot out of the chest, meaning things are not taken out entirely "randomly." This will lead to only a few discs ending up being cycled through. To solve this you may be able to set up a more sophisticated machine to allow more variety.
If you find any problems or have any suggestions feel free to say them here.