In this tutorial, you will learn why APIs are used, how to use them, and how to create your own API. It is helpful to first read Lqyd's series on Computer Basics (start here) before reading this tutorial. You should also have at least basic knowledge of Lua. For the Programming in Lua manual, go here.
The API (Application Programming Interface) system in ComputerCraft provides a standard way to load libraries from separate files. Without the API system, you would find yourself making programs which contain the same code to do common things. For example, suppose you write a custom text editor, and you write many GUI functions for it inside the program. Later, you might want to do something else with these GUI functions, like create a file explorer. The problem is, you would have to copy and paste all of those functions from your old program into the new one. You'll end up spending more time and writing more code than you bargained for! A common solution is to separate your common functions into an API. This way, any program you make which needs to use those GUI functions can just call them from the API, and you can make improvements to your API in one file, instead of having to make changes across multiple files.
Let's first have a look at the help message for the apis command. Enter help apis into the shell prompt.

To restate what the picture says, the apis command lists all of the currently loaded APIs. We're also told that we can run help <api> (e.g. help os) to get help for a specific API, and the message tells us how we can load an API using os.loadAPI. Let's first try running apis and see what the console spits at us.
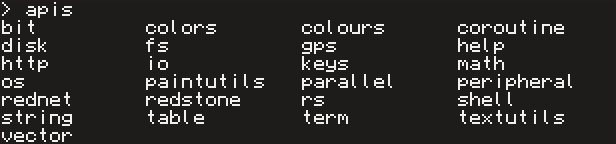
What we have here is a complete list of all the APIs that are automatically loaded from the /rom/apis/ folder when CraftOS starts. You don't have to load these yourself, since they're automatically made available. Let's try using some of these APIs in the Lua prompt. Open the Lua prompt by entering lua into the shell prompt, and then enter these lines into the Lua prompt.
math.floor(22.2)
string.sub("Hello", 2, 4)
textutils.serialize({a=2, b={"b"}, c="c"})
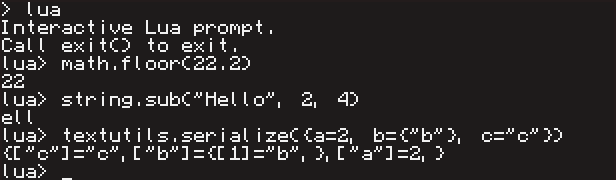
Exit the Lua prompt by entering exit() into the Lua prompt. By example, you can see that you can call an API function using the form someAPI.someFunction(…). Now let's look at how we can create and use our own API. Start off by creating the file that will hold the API functions. Enter edit testAPI into the shell prompt. Then, enter the code into the editor as shown below.
function sayHello()
print("Hello from testAPI!")
end
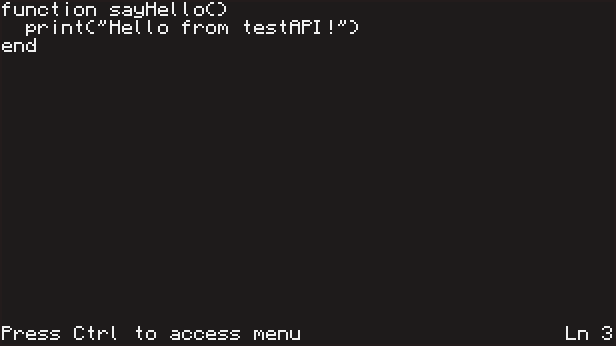
Press Ctrl and select exit to leave the editor. The sayHello function will now be available to any program that loads this API. Let's see that in action. Go back to the Lua prompt and enter os.loadAPI("testAPI"). This will load our API so that any program can use it. Note that the argument given to os.loadAPI must be the precise path to the API file. The name of the file determines the name of the API in code. To make sure it's loaded, run the apis command again.
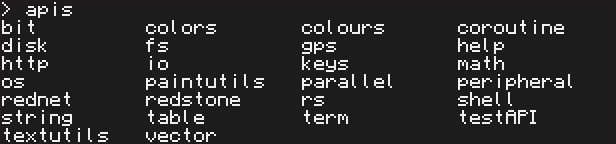
If you look closely, you can see that our API is now listed! Since it's loaded, let's try calling that function we defined inside of it. Go back to the Lua prompt and enter testAPI.sayHello().

Now that we're done with it, let's unload the API. Enter os.unloadAPI("testAPI") into the Lua prompt. Just like os.loadAPI, the argument must be the precise path to the API you want to unload. Exit the Lua prompt and run the apis command again so we can verify the API has been unloaded.
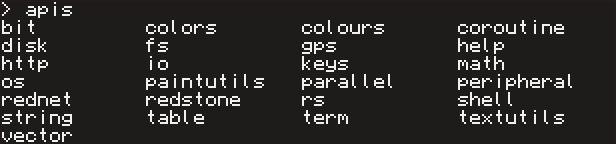
Our API is no longer listed, since it has been unloaded.
Let's backtrack. In this tutorial you learned:
- Why APIs are used.
- How to call API functions.
- How to create, load, and unload your own simple API.