Posted 15 November 2013 - 10:17 PM
This program isn't setup yet so that you can simply start it up and detect any monitors, tanks and power storage devices attached to it, because I haven't yet reached the Lua experience to modify it to do so. Though, I know a lot of people want to know how to do some of the things that I am doing in this program.
I had a really hard time getting the information that I needed through Google searches, IRC and unfortunately even searching topics on this forum (doesn't seem be a big wealth of information on some of these topics like iron tanks). Probably because the documentation isn't really there and what is, isn't understandable for most who don't already have a great understanding of Lua already; like myself. With all of the work and research that I had to do to work this out, I felt like I needed to share it so that people could benefit from it. The code snippets below work for me, unlike pretty much most that I could find on posts for things like Iron Tanks. So I provided them and this post so this information can be found in one post.
Download or view the full program here: Pastebin.
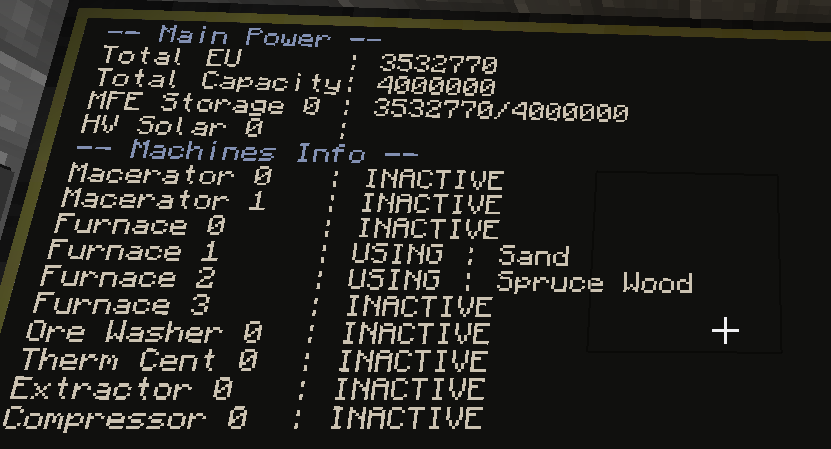
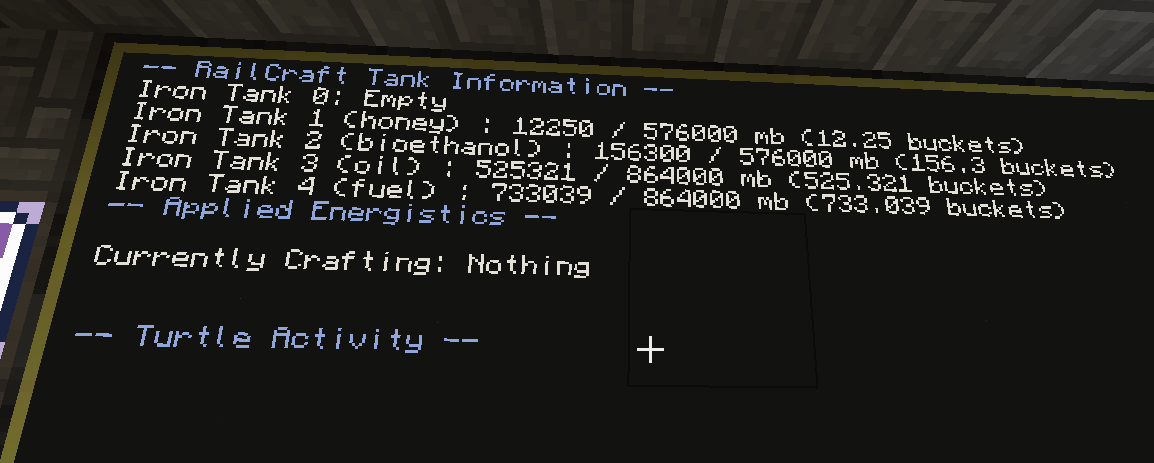
If I explain any of this incorrectly, or any of this code isn't "perfect" then I apologize. I am working on less than one week of Lua experience.
IC2 Machine Slot Numbers / Peripheral IDs
I don't have them all, but this may help as a reference for a few. I was told that there is a large list somewhere, but I was unable to find it. If you know where it is, please let me know.
Most IC2 machines: Slot 7 (input) / Slot 2 (output)
Ore Washer / Thermal Centrifuge: Slot 9 (input)
Note: Peripheral IDs (wired modem) are first come first serve in MP. If macerator_0 has been taken by another player your macerator may be assigned macerator_1.
Method List
The OpenPeripherals documentation often lists only a few methods and some blocks actually have over 20 methods (for example: getTankInfo() which gives you the fluid name and amount from a RailCraft iron tank). To get the list pull up a computer, type "lua" at the prompt and use this (replace rcirontankvalve_0) with whatever the peripheral name is. Peripheral.call just seems to be a shorter way to go about it.
Tables - Finding the information that you are after
Note: This is not how you assign the information in the table to variables that your program can access. For that: look under the Iron Tanks section. However, it is how you see the table and the keys and values inside of it, which you will need to do so (such as "capacity" and "576000"). When I was attempting to figure out this mess there was a lot of confusion about this and from what I've seen on the forum, many others have been confused by being told to do this, but not how to use the information gained from it.
The result hopefully is similar to this:
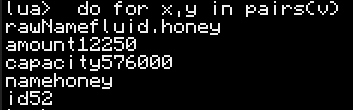
Iron Tanks
First up: wrap the peripheral. Attach a wired modem to the iron tank valve by network cable to a computer. Right click the modem to get the peripheral's ID (starts at rcirontankvalvetile_0)
Second: a function to pull the information from it (fluid amount, name, capacity, id, etc). The method getTankInfo(side) does this. I'm told that you are supposed to provide a side, but unknown should work if you do not know.
Next: display the information. The code is the same for every one except for getTank(irontank0) which you will change to irontank0, irontank1, etc (or whatever you decided to wrap the peripheral as). There is more to my code for each tank, but again, I'm keeping it simple. See the Pastebin for the full version.
IC2 Machines
This one was a lot easier to figure out. Example of wrapping the peripheral:
Now to access the information from an IC2 machine. The first thing that I had to figure out is the slot number for each machine (the item being used and the item being created). Generally it seems that
slot 7 is where the item goes, such as sand in a furnace when creating glass. The ore washer and thermal centrifuge use slot 9. If I remember correctly, sometimes slot 2 is the item being created.
Simplified version:
IC2 Machine Slot Timing Issue
I had to place a timer in the IC2 machine code to wait for a while and hold the information on the screen. Otherwise it would go away as soon as it appeared, because the program would for whatever reason only receive the information that an item was in the slot when an item was being pushed out into the output slot (sand->glass). While it was working on the item it was not actively sending the information to the program. So in every loop of the program, it would clear the message. Look at the pastebin to see the it and search for "timer". Just be aware that this is a problem and you might get frustrated if you don't use something similar, because you'll think the program doesn't work when the message only appears on screen for a split second and you don't see it.
Links / Helpful Sites
Direwolf ComputerCraft Tutorial Series - very basic tutorials but explained very well as always
Edoreld OpenPeripheral Mod Spotlight
OpenPeripheral Documentation - It is not complete, but it will give you an idea of what mods are supported.
Lua.Org - a bit difficult to understand sometimes for new Lua scripters
Stack Exchange
I had a really hard time getting the information that I needed through Google searches, IRC and unfortunately even searching topics on this forum (doesn't seem be a big wealth of information on some of these topics like iron tanks). Probably because the documentation isn't really there and what is, isn't understandable for most who don't already have a great understanding of Lua already; like myself. With all of the work and research that I had to do to work this out, I felt like I needed to share it so that people could benefit from it. The code snippets below work for me, unlike pretty much most that I could find on posts for things like Iron Tanks. So I provided them and this post so this information can be found in one post.
Download or view the full program here: Pastebin.
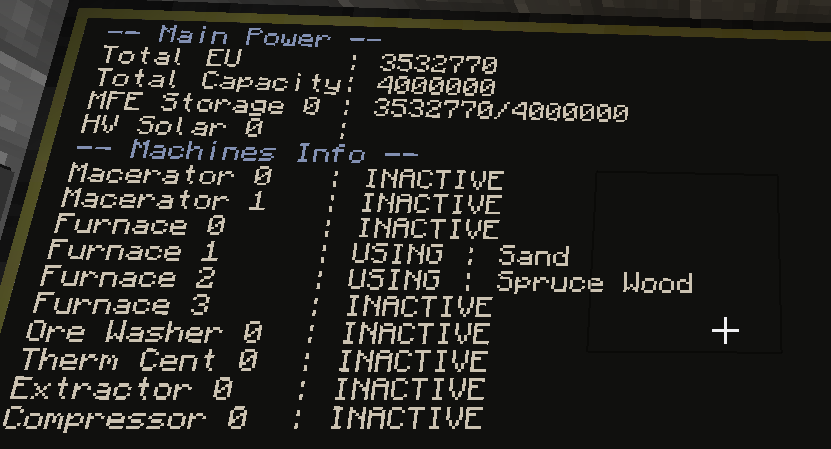
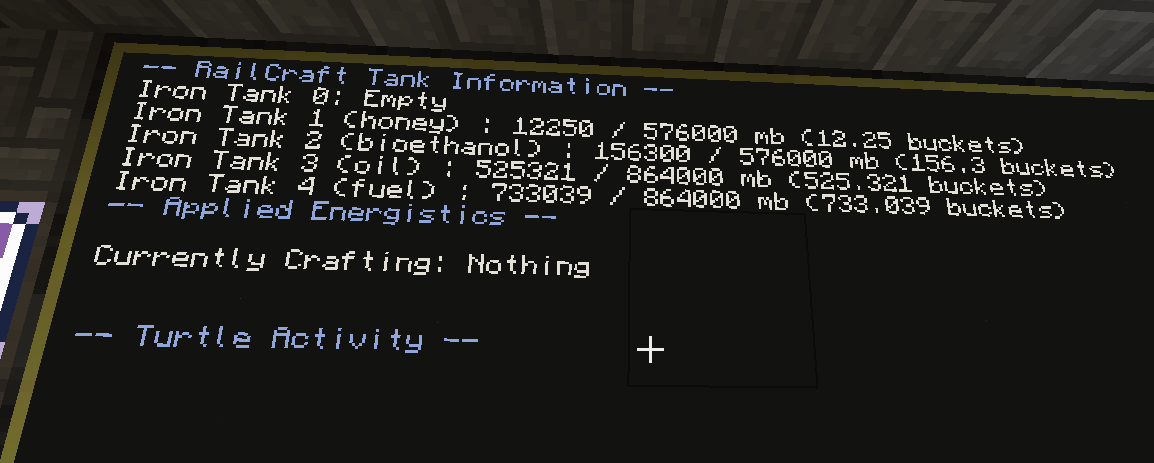
If I explain any of this incorrectly, or any of this code isn't "perfect" then I apologize. I am working on less than one week of Lua experience.
IC2 Machine Slot Numbers / Peripheral IDs
I don't have them all, but this may help as a reference for a few. I was told that there is a large list somewhere, but I was unable to find it. If you know where it is, please let me know.
Most IC2 machines: Slot 7 (input) / Slot 2 (output)
Ore Washer / Thermal Centrifuge: Slot 9 (input)
Note: Peripheral IDs (wired modem) are first come first serve in MP. If macerator_0 has been taken by another player your macerator may be assigned macerator_1.
Method List
The OpenPeripherals documentation often lists only a few methods and some blocks actually have over 20 methods (for example: getTankInfo() which gives you the fluid name and amount from a RailCraft iron tank). To get the list pull up a computer, type "lua" at the prompt and use this (replace rcirontankvalve_0) with whatever the peripheral name is. Peripheral.call just seems to be a shorter way to go about it.
peripheral.call("rcirontankvalvetile_0", "listMethods")
Tables - Finding the information that you are after
Note: This is not how you assign the information in the table to variables that your program can access. For that: look under the Iron Tanks section. However, it is how you see the table and the keys and values inside of it, which you will need to do so (such as "capacity" and "576000"). When I was attempting to figure out this mess there was a lot of confusion about this and from what I've seen on the forum, many others have been confused by being told to do this, but not how to use the information gained from it.
local tank = peripheral.wrap("rcirontankvalvetile_1")
local tableInfo = tank.getTanks("unknown")
for k, v in pairs(tableInfo) do
for x, y in pairs(v) do
print(x .. ": " .. y)
end
end
The result hopefully is similar to this:
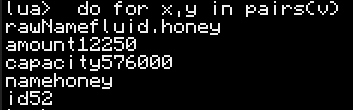
Iron Tanks
First up: wrap the peripheral. Attach a wired modem to the iron tank valve by network cable to a computer. Right click the modem to get the peripheral's ID (starts at rcirontankvalvetile_0)
local irontank0 = peripheral.wrap("rcirontankvalvetile_0")
Second: a function to pull the information from it (fluid amount, name, capacity, id, etc). The method getTankInfo(side) does this. I'm told that you are supposed to provide a side, but unknown should work if you do not know.
function getTank(tankPeriph)
local tableInfo = tankPeriph.getTankInfo("unknown")[1]
local fluidRaw, fluidName, fluidAmount, fluidCapacity, fluidID
fluidRaw = tableInfo.rawName
fluidName = tableInfo.name
fluidAmount = tableInfo.amount
fluidCapacity = tableInfo.capacity
fluidID = tableInfo.id
return fluidRaw, fluidName, fluidAmount, fluidCapacity, fluidID
end
Next: display the information. The code is the same for every one except for getTank(irontank0) which you will change to irontank0, irontank1, etc (or whatever you decided to wrap the peripheral as). There is more to my code for each tank, but again, I'm keeping it simple. See the Pastebin for the full version.
function dispTanks()
-- TANK 0
local fluidName, fluidAmount, fluidCapacity, fluidID = getTank(irontank0)
mon2.write("Iron Tank 0 (" .. fluidName .. ") : " .. fluidAmount .. " / " .. fluidCapacity)
end
end
IC2 Machines
This one was a lot easier to figure out. Example of wrapping the peripheral:
local furnace = peripheral.wrap("electric_furnace_0")
Now to access the information from an IC2 machine. The first thing that I had to figure out is the slot number for each machine (the item being used and the item being created). Generally it seems that
slot 7 is where the item goes, such as sand in a furnace when creating glass. The ore washer and thermal centrifuge use slot 9. If I remember correctly, sometimes slot 2 is the item being created.
Simplified version:
furnaceStack = furnace.getStackInSlot(7)
if furnaceStack ~= nil then -- if something is in the slot
itemName = furnaceStack.name
mon.write("Furnace 0 : USING " : " .. itemName)
elseif furnacestack == nil then -- if nothing is in the slot
mon.write("Furnace 0 : INACTIVE")
end
end
IC2 Machine Slot Timing Issue
I had to place a timer in the IC2 machine code to wait for a while and hold the information on the screen. Otherwise it would go away as soon as it appeared, because the program would for whatever reason only receive the information that an item was in the slot when an item was being pushed out into the output slot (sand->glass). While it was working on the item it was not actively sending the information to the program. So in every loop of the program, it would clear the message. Look at the pastebin to see the it and search for "timer". Just be aware that this is a problem and you might get frustrated if you don't use something similar, because you'll think the program doesn't work when the message only appears on screen for a split second and you don't see it.
Links / Helpful Sites
Direwolf ComputerCraft Tutorial Series - very basic tutorials but explained very well as always
Edoreld OpenPeripheral Mod Spotlight
OpenPeripheral Documentation - It is not complete, but it will give you an idea of what mods are supported.
Lua.Org - a bit difficult to understand sometimes for new Lua scripters
Stack Exchange
Edited on 17 November 2013 - 02:31 AM