Posted 20 November 2013 - 05:19 PM
Executive Summary
Install: rom/programs/http/pastebin get agGfpAbX geode
What: A program to harvest a 1,830 abyssal stone geode from Railcraft
Where: Ocean biomes below 40 or so
Requirements: A mining turtle preferably chunk loading and an ender chest unloaded back at your base
Fuel: 875 fuel; I use lava buckets
Time: 22 minutes give or take a few seconds based on what is mined out
Resume from crash: Not currently; sorry
Game Version: I play FTB Ultimate on MC 1.5.2 (any pack with Railcraft 7.1.0.0 or later will work though)
I really love building my home from abyssal stone from Railcraft but harvesting it can be very tedious. Previously I would make an ocean biome world with Mystcraft and chuck an eight position frame mining well machine in it (RedPower2) and never worry about my abyssal stone supply (or many other resources for that matter). Sadly in a post-RedPower2 world that isn't possible anymore and I've had to resort to more hands on means.
My current 4-chunk base built from abyssal block and vibrant quartz
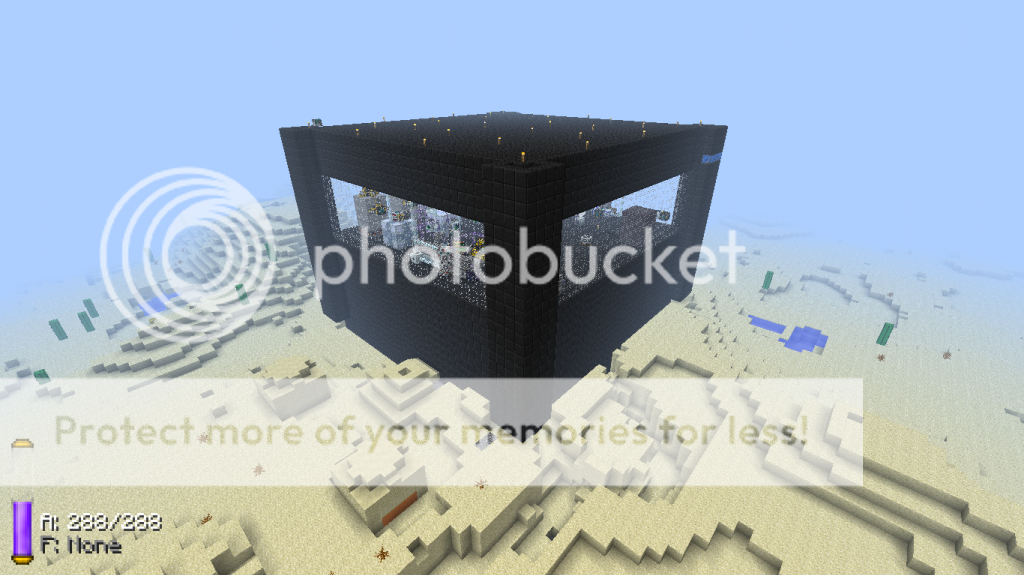
One of the few semi automatic methods still open to me is ComputerCraft and thus this post. I realize this is a pretty narrow task to undertake and don't expect flocks of people to use this but still I searched the interwebs and found zilch which did surprise me a little.
This program expects the turtle to begin in the lowest spot of the interior of the geode and facing in the negative z direction. The direction is not very important, it will only make a difference of two abyssal stone blocks because the geodes have an extra block in the negative x , y and z directions. It also expects an ender chest in slot 16 which is automatically unloaded back at base (pretty standard). It will prompt you if this is missing and wait until something is placed in slot 16. Finally, it takes 875 fuel to carry out this program and again the program will pause and refuel from slot 1 until the fuel level is greater than 875. This minFuel local variable can be easily changed in code if you play in a world without turtle fuel. I use a chunk loading mining turtle since it takes 22 minutes to mine out the geode which yields 1,830 abyssal stone blocks. I obviously don't stay and watch thus the chunk loading turtle.
Turtle placed in the geode's interior dimple facing in the -z direction
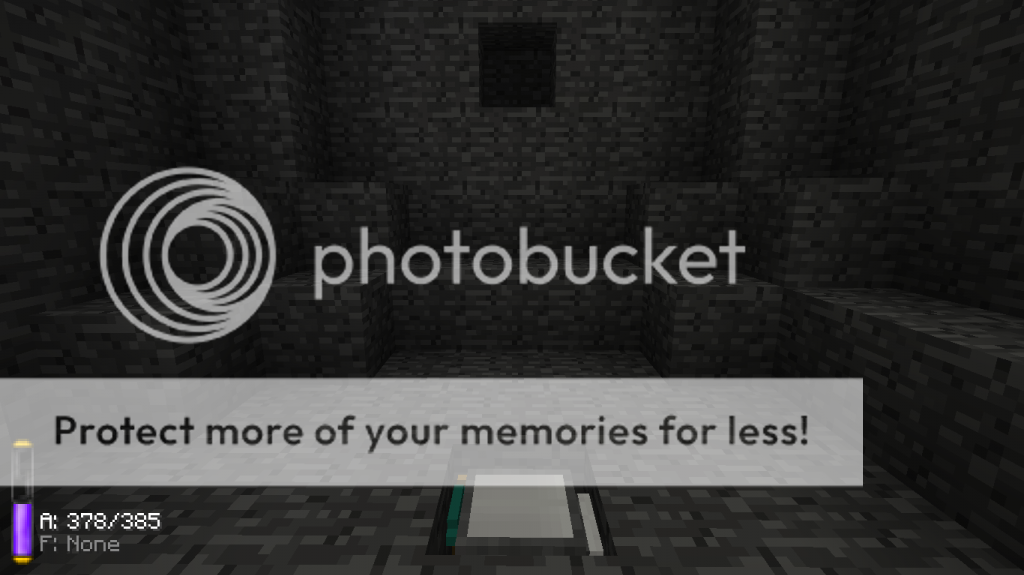
Inventory being emptied into an ender chest
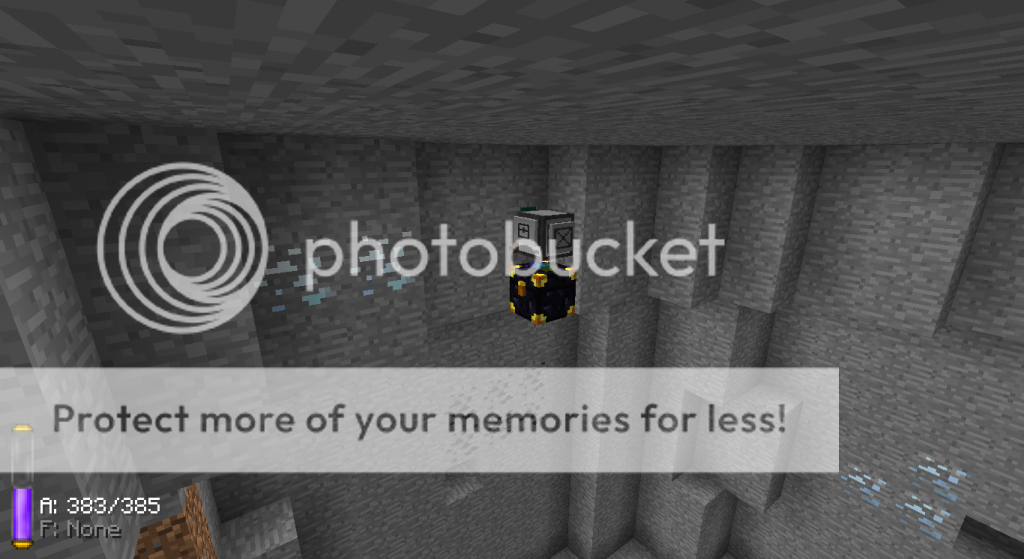
Mining complete
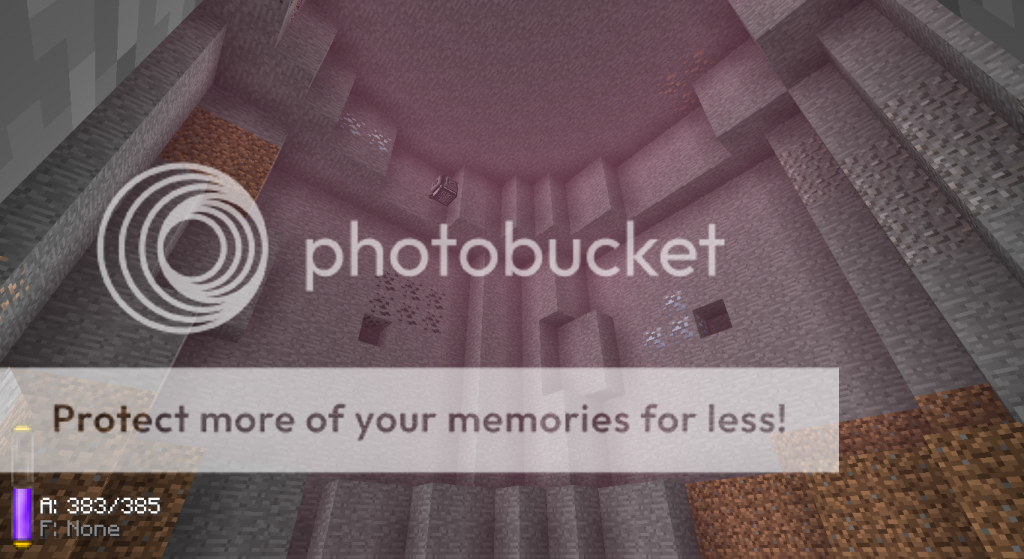
The code could certainly use more comments and lua is not my primary programming language so there are probably more succinct ways of doing what I'm doing. If anyone has any lua advice, I am always happy to learn.
Finally the pastebin ID is agGfpAbX so the command line would be: rom/programs/http/pastebin get agGfpAbX geode
I hope others find this as useful as I have!
Install: rom/programs/http/pastebin get agGfpAbX geode
What: A program to harvest a 1,830 abyssal stone geode from Railcraft
Where: Ocean biomes below 40 or so
Requirements: A mining turtle preferably chunk loading and an ender chest unloaded back at your base
Fuel: 875 fuel; I use lava buckets
Time: 22 minutes give or take a few seconds based on what is mined out
Resume from crash: Not currently; sorry
Game Version: I play FTB Ultimate on MC 1.5.2 (any pack with Railcraft 7.1.0.0 or later will work though)
I really love building my home from abyssal stone from Railcraft but harvesting it can be very tedious. Previously I would make an ocean biome world with Mystcraft and chuck an eight position frame mining well machine in it (RedPower2) and never worry about my abyssal stone supply (or many other resources for that matter). Sadly in a post-RedPower2 world that isn't possible anymore and I've had to resort to more hands on means.
My current 4-chunk base built from abyssal block and vibrant quartz
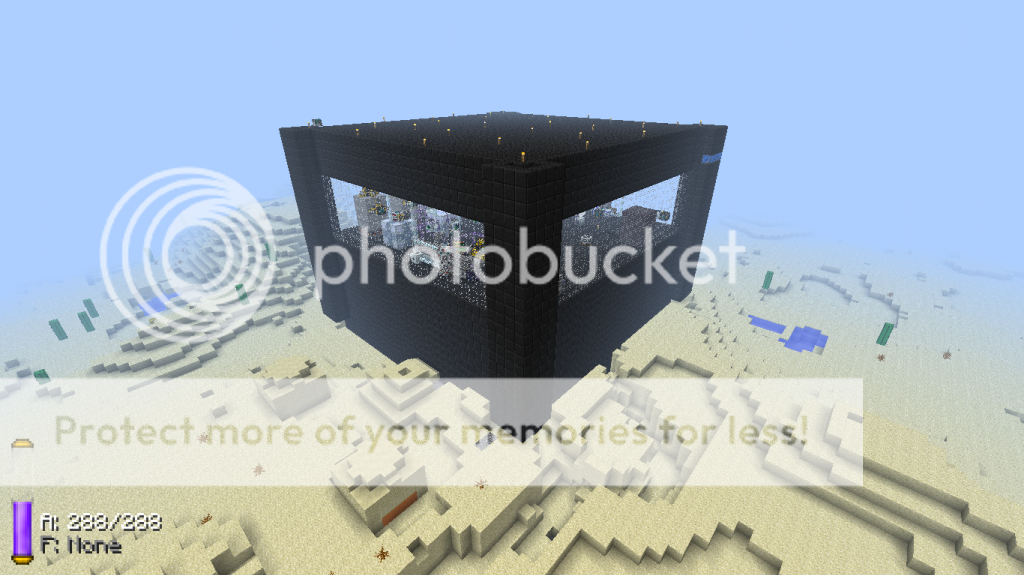
One of the few semi automatic methods still open to me is ComputerCraft and thus this post. I realize this is a pretty narrow task to undertake and don't expect flocks of people to use this but still I searched the interwebs and found zilch which did surprise me a little.
This program expects the turtle to begin in the lowest spot of the interior of the geode and facing in the negative z direction. The direction is not very important, it will only make a difference of two abyssal stone blocks because the geodes have an extra block in the negative x , y and z directions. It also expects an ender chest in slot 16 which is automatically unloaded back at base (pretty standard). It will prompt you if this is missing and wait until something is placed in slot 16. Finally, it takes 875 fuel to carry out this program and again the program will pause and refuel from slot 1 until the fuel level is greater than 875. This minFuel local variable can be easily changed in code if you play in a world without turtle fuel. I use a chunk loading mining turtle since it takes 22 minutes to mine out the geode which yields 1,830 abyssal stone blocks. I obviously don't stay and watch thus the chunk loading turtle.
Turtle placed in the geode's interior dimple facing in the -z direction
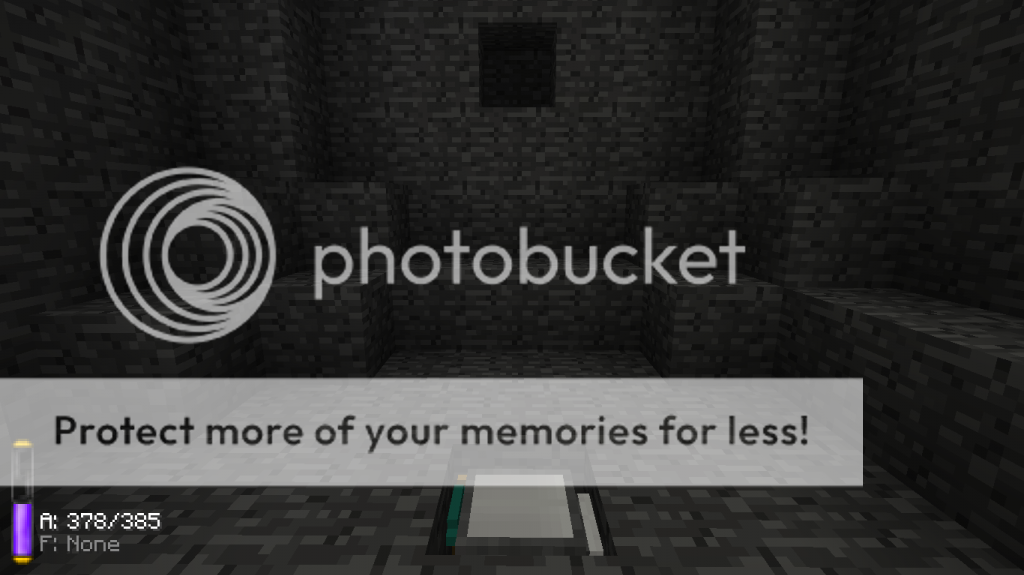
Inventory being emptied into an ender chest
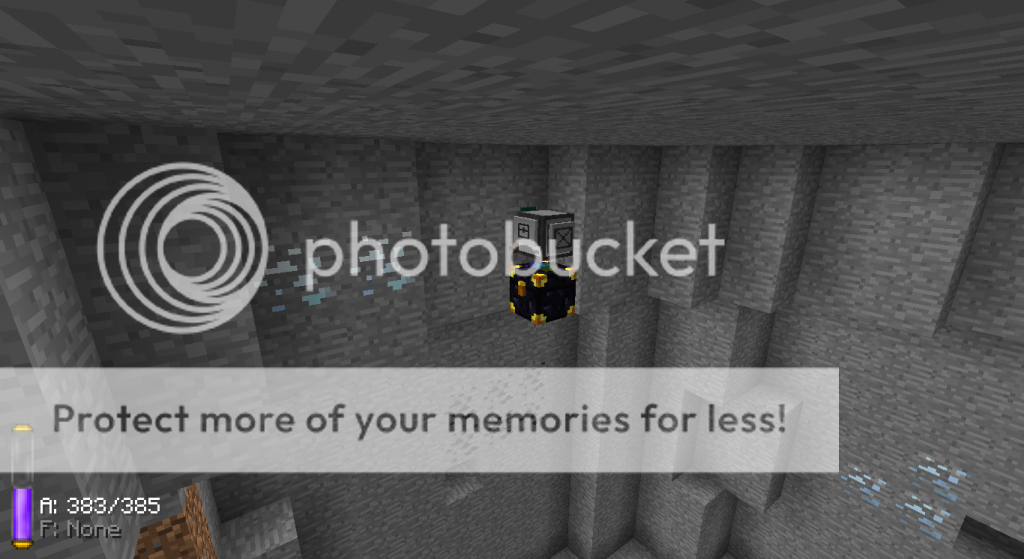
Mining complete
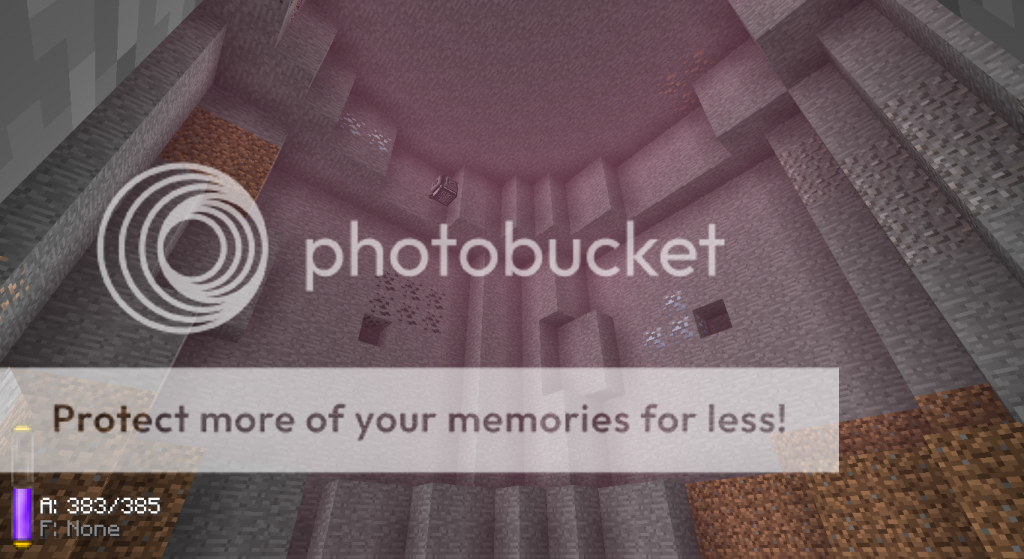
The code could certainly use more comments and lua is not my primary programming language so there are probably more succinct ways of doing what I'm doing. If anyone has any lua advice, I am always happy to learn.
Spoiler
local minFuel = 875
local i=0
local function ClearTerminal()
term.clear()
term.setCursorPos(1,1)
end
local function AssureInv()
if turtle.getItemCount(15)>0 then
turtle.digDown()
turtle.select(16)
turtle.placeDown()
for i=1,15,1 do
turtle.select(i)
turtle.dropDown()
end
turtle.select(16)
turtle.digDown()
--turtle.select(1)
end
end
local function DigForward(count)
for i=1,count,1 do
AssureInv()
turtle.dig()
while turtle.forward()==false do
turtle.dig()
turtle.attack()
sleep(0.05)
end
turtle.digUp()
turtle.digDown()
end
end
local function RightFace()
turtle.turnRight()
DigForward(1)
turtle.turnRight()
end
local function LeftFace()
turtle.turnLeft()
DigForward(1)
turtle.turnLeft()
end
local function GoUp()
for i=1,3,1 do
while turtle.up()==false do
turtle.attackUp()
turtle.digUp()
end
end
turtle.digUp()
end
local function DigTierOne()
--Dig down to starting position
turtle.digDown()
turtle.down()
turtle.digDown()
turtle.down()
turtle.digDown()
turtle.down()
turtle.digDown()
turtle.up()
DigForward(6)
RightFace()
DigForward(12)
turtle.back()
LeftFace()
DigForward(10)
RightFace()
DigForward(10)
turtle.back()
LeftFace()
DigForward(8)
turtle.back()
RightFace()
DigForward(6)
turtle.back()
turtle.back()
LeftFace()
DigForward(2)
turtle.back()
turtle.turnLeft()
DigForward(6)
turtle.turnLeft()
DigForward(6)
RightFace()
DigForward(12)
turtle.back()
LeftFace()
DigForward(10)
RightFace()
DigForward(10)
turtle.back()
LeftFace()
DigForward(8)
turtle.back()
RightFace()
DigForward(6)
turtle.back()
turtle.back()
LeftFace()
DigForward(2)
RightFace()
GoUp()
end
local function DigTierTwo()
--Row 1
DigForward(4)
turtle.turnRight()
turtle.turnRight()
DigForward(7)
--Row 2
LeftFace()
DigForward(9)
--Row 3
RightFace()
DigForward(11)
--Row 4
LeftFace()
DigForward(13)
--Row 5
RightFace()
DigForward(14)
--Row 6
LeftFace()
DigForward(14)
--Row 7
RightFace()
DigForward(14)
--Row 8
LeftFace()
DigForward(14)
--Row 9
RightFace()
DigForward(14)
--Row 10
LeftFace()
DigForward(14)
--Row 11
RightFace()
DigForward(14)
turtle.back()
--Row 12
LeftFace()
DigForward(12)
turtle.back()
--Row 13
RightFace()
DigForward(10)
turtle.back()
--Row 14
LeftFace()
DigForward(8)
turtle.back()
--Row 15
RightFace()
DigForward(6)
end
local function DigTierThree()
turtle.turnLeft()
turtle.turnLeft()
DigForward(8)
LeftFace()
DigForward(11)
RightFace()
DigForward(12)
LeftFace()
DigForward(13)
RightFace()
DigForward(14)
LeftFace()
DigForward(14)
RightFace()
DigForward(14)
--Get the sole abyssal block projecting in the -x direction
turtle.turnLeft()
DigForward(1)
turtle.turnRight()
turtle.dig()
turtle.turnLeft()
turtle.turnLeft()
DigForward(14)
RightFace()
DigForward(14)
LeftFace()
DigForward(14)
RightFace()
DigForward(14)
turtle.back()
LeftFace()
DigForward(12)
RightFace()
DigForward(12)
turtle.back()
LeftFace()
DigForward(10)
turtle.back()
turtle.back()
RightFace()
DigForward(3)
turtle.turnLeft()
turtle.dig()
turtle.turnRight()
DigForward(3)
end
local function DigTierFour()
turtle.turnLeft()
turtle.turnLeft()
DigForward(7)
LeftFace()
DigForward(9)
RightFace()
DigForward(11)
LeftFace()
DigForward(13)
RightFace()
DigForward(14)
LeftFace()
DigForward(14)
RightFace()
DigForward(14)
LeftFace()
DigForward(14)
RightFace()
DigForward(14)
LeftFace()
DigForward(14)
RightFace()
DigForward(14)
turtle.back()
LeftFace()
DigForward(12)
turtle.back()
RightFace()
DigForward(10)
turtle.back()
LeftFace()
DigForward(8)
turtle.back()
RightFace()
DigForward(6)
end
local function MoveToTierFive()
turtle.back()
turtle.back()
turtle.turnLeft()
turtle.back()
turtle.turnLeft()
GoUp()
end
local function DigTierFive()
DigForward(4)
LeftFace()
DigForward(7)
RightFace()
DigForward(9)
LeftFace()
DigForward(10)
RightFace()
DigForward(11)
LeftFace()
DigForward(12)
RightFace()
DigForward(12)
LeftFace()
DigForward(12)
turtle.back()
RightFace()
DigForward(10)
LeftFace()
DigForward(10)
turtle.back()
RightFace()
DigForward(8)
turtle.back()
LeftFace()
DigForward(6)
turtle.back()
turtle.back()
RightFace()
DigForward(2)
end
local function FinalDump()
turtle.select(16)
turtle.placeDown()
for i=1,15,1 do
turtle.select(i)
turtle.dropDown()
end
turtle.select(16)
turtle.digDown()
end
--=========== Main Program ===========
--Make sure we have enough fuel to begin
ClearTerminal()
if turtle.getFuelLevel()<minFuel then
print("WARNING: Insufficient fuel; please add fuel to slot 1")
end
turtle.select(1)
while turtle.getFuelLevel()<minFuel do
if turtle.refuel()==true then
print("Fuel level now " .. turtle.getFuelLevel() .. " of " .. minFuel .. " needed")
end
sleep(0.1)
end
--Make sure we have an ender chest to
--route material back to our base
if turtle.getItemCount(16)~=1 then
print("Place an ender chest in slot 16")
end
while turtle.getItemCount(16)~=1 do
sleep(0.1)
end
--Proceed with the geode mining
ClearTerminal()
print("Digging geode, check back in 22 minutes")
print("Initial fuel level is " .. turtle.getFuelLevel())
DigTierOne()
DigTierTwo()
GoUp()
DigTierThree()
GoUp()
DigTierFour()
MoveToTierFive()
DigTierFive()
FinalDump()
print("Final fuel level is " .. turtle.getFuelLevel())
Finally the pastebin ID is agGfpAbX so the command line would be: rom/programs/http/pastebin get agGfpAbX geode
I hope others find this as useful as I have!
Edited on 20 November 2013 - 05:04 PM