Recently I got an urge to do this for no apparent reason, even though I've gotten bored of both ComputerCraft and Minecraft.
It is a JavaScript that will take the URL to a ComputerCraft image file and display it on the webpage.
It should be compatible across all devices (computers, tablets, smartphones) and browsers as it is purely JavaScript based.
At the moment I feel this is pretty stable, but I haven't really tested it much, so report any bugs you find.
Oh, and also, the images that it renders aren't actual .png files or anything, just a bunch of HTML markup. And it works for all sizes of images. Although, unexpected results will be caused if the image goes further than the browser width.
Changelog:
[indent=1]1.0: Initial release.[/indent]
[indent=1]1.1: Make the coloring the same as ComputerCraft. Thanks theoriginalbit for the hex codes. Use the same Pastebin link for the latest version.[/indent]
Current Setbacks:
- Takes about a second to render the image.
- Can't load images from your hard drive - most features will only work when uploaded to a webserver.
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="cc_render.js"></script>
</head>
<body>
<div id="canvas1" class="canvas">
<!-- The rendered image will be put in here -->
</div>
<script type="text/javascript">
var canvas1 = document.getElementById("canvas1");
renderImageFromFile(canvas1, "testImage");
</script>
</body>
</html>
Extra Functions:
[indent=1]renderPixel(canvas, color) - Outputs a pixel on the specified canvas, with the specified color.[/indent]
[indent=1]renderBlankPixel(canvas) - Outputs a blank, transparent pixel on the specified canvas.[/indent]
[indent=1]nextLine(canvas) - Goes down a row on the specified canvas.[/indent]
[indent=1]renderImageRow(canvas, image) - Takes in a row of image code (a line from a CC image file) and outputs it onto the specified canvas.[/indent]
[indent=1]renderImageFromFile(canvas, fileStr) - Reads the file from the specified URL and outputs the contents on the canvas. Probably the main function.[/indent]
[indent=1]clearCanvas(canvas) - Clears the specified canvas.[/indent]
[indent=1]In place of the canvas parameter - you need to put the element which you'd most likely retrieve using the document.getElementById function.[/indent]
Tweaks in the JS file:
[indent=1]Most things that you would want changed are clear variables at the top of the JavaScript file.[/indent]
[indent=1]Here is what each variable/config thingy does:[/indent]
[indent=1]pixelWidth. Width of a pixel. Remember ComputerCraft pixels are taller than they are wide.[/indent]
[indent=1]pixelHeight. Height of a pixel. ^[/indent]
[indent=1]zoom. Zoom on the pixels. I recommend 2.[/indent]
[indent=1]debug. Outputs to the JavaScript console when pixels and drawn and on what canvas.[/indent]
[indent=1]pixelBorders. Shows black borders around all the pixels. A sort of debug option. Partially mis-aligns all the pixels.[/indent]
[indent=1]Further down you'll also see a large array called colors. This here basically is all the character codes - and what HTML color to convert them to. You shouldn't need to modify this.[/indent]
Live Demo:
[indent=1]You can have a look at a demo here: http://mitchfizz05.n..._imagerenderer/[/indent]
Pictures:
[indent=1]You could probably guess what it will look like, but meh. The sayin' goes "no pics, no clicks" - so I'll put some pics.[/indent]
[indent=1]
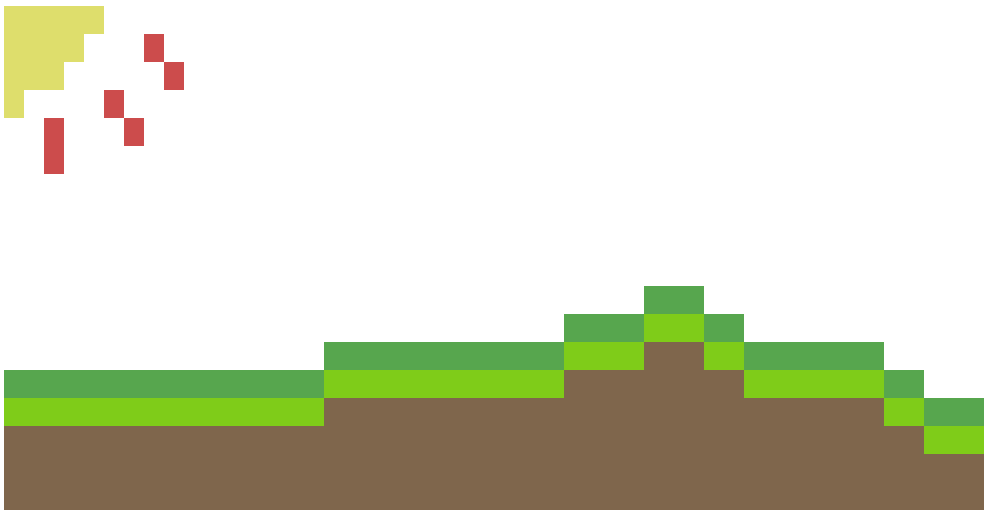
Using in your own projects:
[indent=1]You can use this in your own projects if you want, however, please give credit in some form.[/indent]
Oh, and apologies if I put this in the wrong section, but I suppose this is a program, so yeah. I'm a bit rusty cause I haven't posted here for months.
So, yeah. Enjoy.