Intro
Hello everyone! Almost a year ago I released MonAPI which was an API that allowed you to make small sections within monitors that acted more or less like a regular monitor object. I had originally made this API for a project I was working on and decided to polish it up and release it. It worked but the code wasn't very pretty and it was missing some features (scroll, setCursorBlink). I had plans to implement these features but one thing I really wanted to see in the API was support for multiple monitors. Anyway, 1.6 was released and it came with a window API that basically did what my API did but it was a lot more efficient, feature-complete and better coded in general. It however did not have support for multiple monitors. Seeing this, it motivated me to work on my API again, however I wanted to just make a new API that did multiple monitors and nothing else. So that is why I am releasing multiMon.
Documentation
The API comes with 1 function:
create( name, monitors, [, wide [, tall [, width [, height ]]]] )
This functions creates a virtual monitor (that is fully compatible with the peripheral API and emits monitor_touch events) out of multiple monitor objects stitched together. It accepts the following arguments:- name Name of the virtual monitor.
- monitors A table in format: { { object [, …] } [, …] } containing the monitor objects to be stitched.
- wide Optional (default: determined by 'monitors' argument). Amount of monitor objects stacked horizontally.
- tall Optional (default: determined by 'monitors' argument). Amount of monitor objects stacked vertically.
- width Optional (default: the width of the first object in 'monitors' argument). Width of each monitor object.
- height Optional (default: the height of the first object in 'monitors' argument). Height of each monitor object.
Side notes:
1. All monitors should be of the width and height and the monitors stitched together should form a rectangle.
2. You must load the API [os.loadAPI("multiMon")] before wrapping to the actual monitors otherwise touch events may not work.
3. This API is meant to be used with monitors however it should be compatible with terminal and window objects.
Examples
Setup:
Spoiler
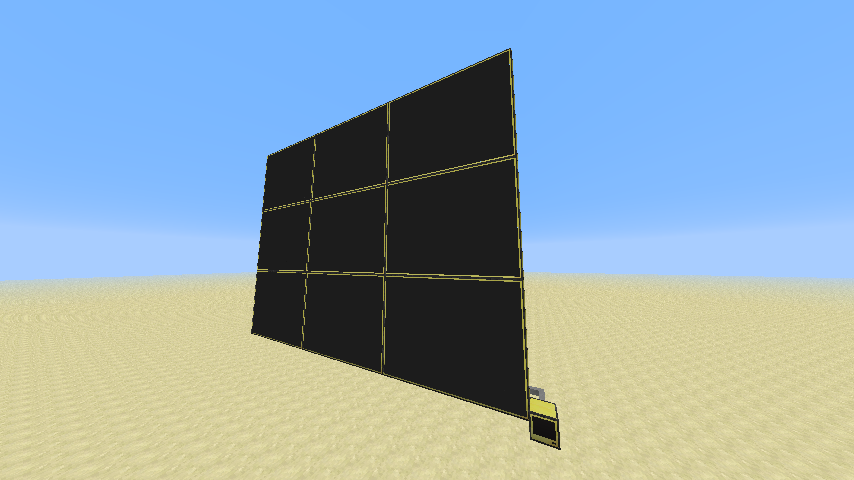
Yeah, that's right 9 full size monitors. Here is what the back looks like:
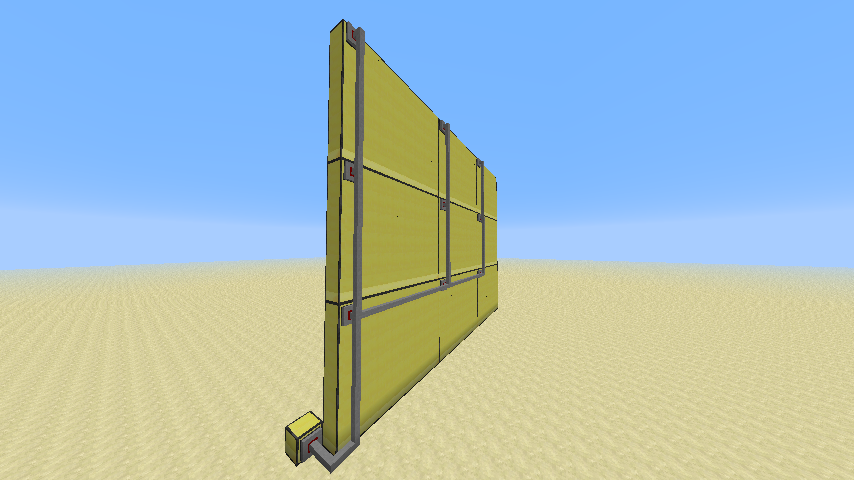
Simple example:
Spoiler
--# Load the API
os.loadAPI('/multiMon')
--# Create the monitors object (the monitors are arranged in the world the same way you see them in the table below).
local monitorSetup = {
{peripheral.wrap('monitor_22'), peripheral.wrap('monitor_23'), peripheral.wrap('monitor_24')},
{peripheral.wrap('monitor_25'), peripheral.wrap('monitor_26'), peripheral.wrap('monitor_27')},
{peripheral.wrap('monitor_19'), peripheral.wrap('monitor_20'), peripheral.wrap('monitor_21')},
}
--# Create the virtual monitor (will also return the handle).
local disp = multiMon.create('myMonitor', monitorSetup)
--# Showcasing some basic functions.
disp.setTextScale(0.5)
if disp.isColour() then
disp.setTextColour(colours.white)
disp.setBackgroundColour(colours.black)
end
disp.setCursorBlink(false)
disp.clear()
disp.setCursorPos(1, 1)
local width, height = disp.getSize()
--# Writes random characters onto the screen.
for y = 1, height do
disp.setCursorPos(1, y)
disp.write(string.rep(string.char(math.random(32, 126)), width))
end
Here is what it looks like on the monitor: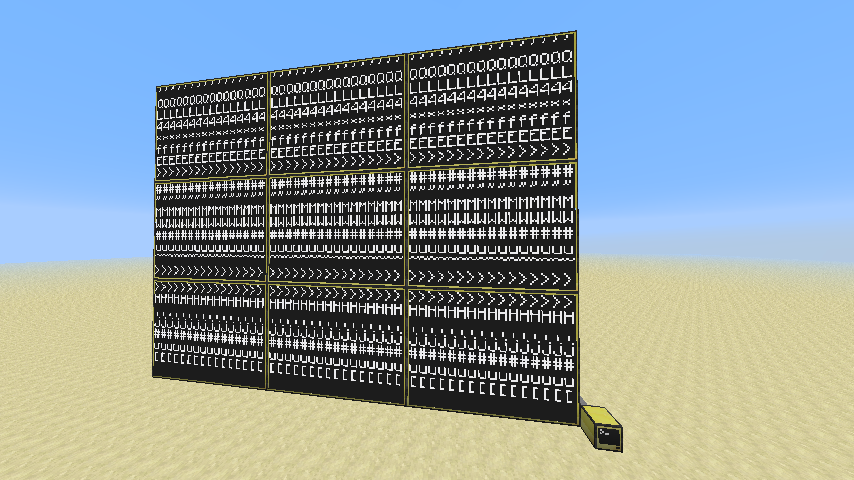
Here it is with 0.5 text scale instead of 5:
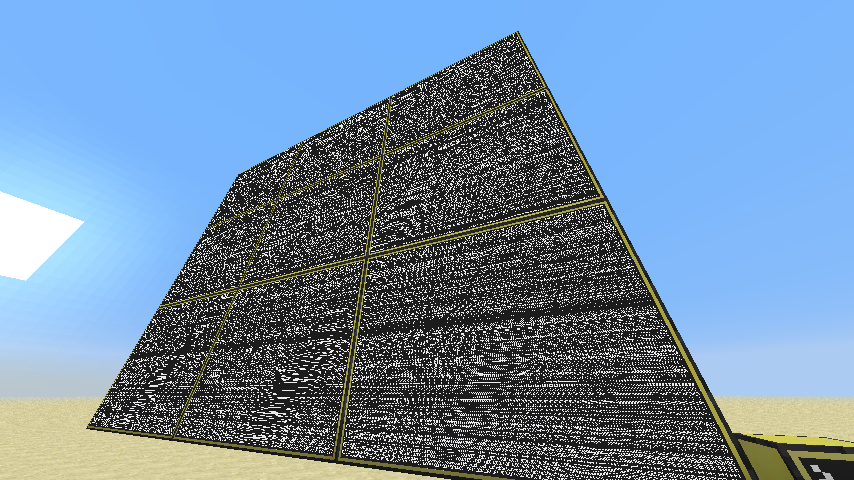
Closer up:
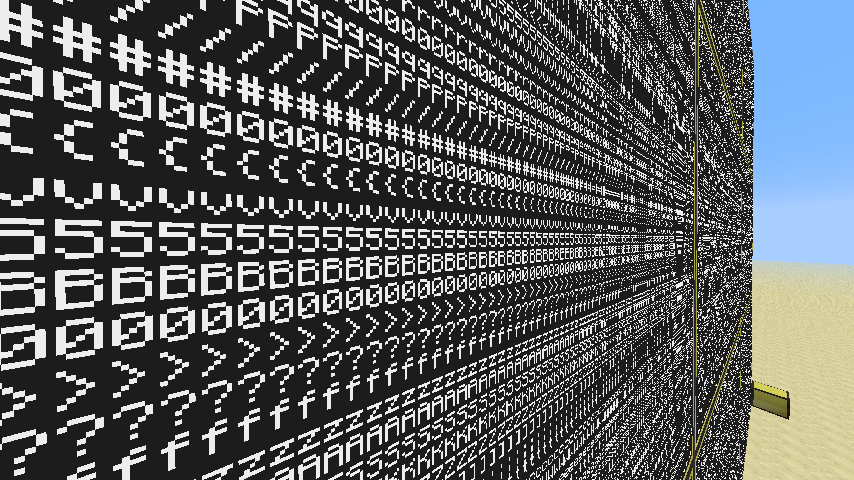
Running paint (on the same setup):
Spoiler
--# Load the API
os.loadAPI('/multiMon')
--# Create the monitors object (the monitors are arranged in the world the same way you see them in the table below).
local monitorSetup = {
{peripheral.wrap('monitor_22'), peripheral.wrap('monitor_23'), peripheral.wrap('monitor_24')},
{peripheral.wrap('monitor_25'), peripheral.wrap('monitor_26'), peripheral.wrap('monitor_27')},
{peripheral.wrap('monitor_19'), peripheral.wrap('monitor_20'), peripheral.wrap('monitor_21')},
}
--# Create the virtual monitor (will also return the handle).
local disp = multiMon.create('myMonitor', monitorSetup)
--# Showcasing some basic functions.
disp.setTextScale(0.5)
if disp.isColour() then
disp.setTextColour(colours.white)
disp.setBackgroundColour(colours.black)
end
disp.setCursorBlink(false)
disp.clear()
disp.setCursorPos(1, 1)
local width, height = disp.getSize()
--# Some setup is needed for paint.
parallel.waitForAny(
function()
--# Since paint is meant to work only on the terminal, we need to catch all touch events and turn them into mouse_click events.
--# This happens in the background while paint is running.
while true do
local ev = {os.pullEvent('monitor_touch')}
if ev[2] == 'myMonitor' then
os.queueEvent('mouse_click', 1, ev[3], ev[4])
end
end
end,
function()
--# Since paint uses 'term' internally, we need to set 'disp' to act as 'term'.
term.redirect(disp)
--# Run the program with 'image' as the argument.
shell.run('/rom/programs/advanced/paint', 'image')
end
)
Empty canvas: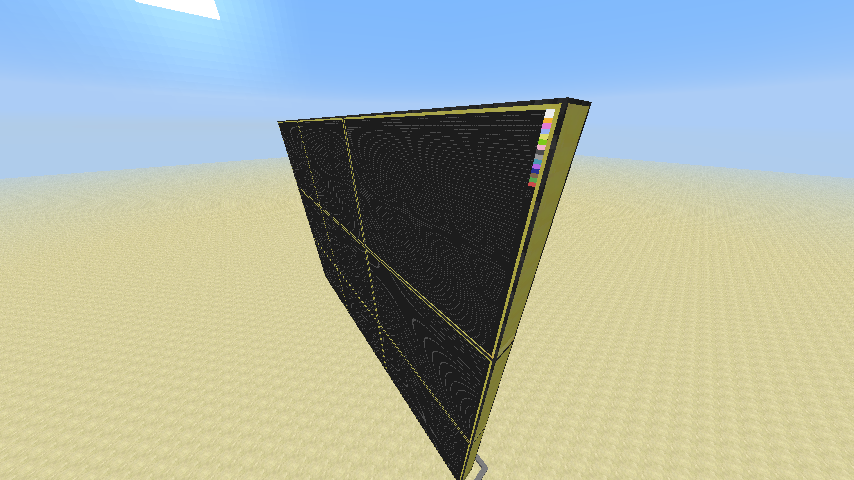
After some random brush strokes:
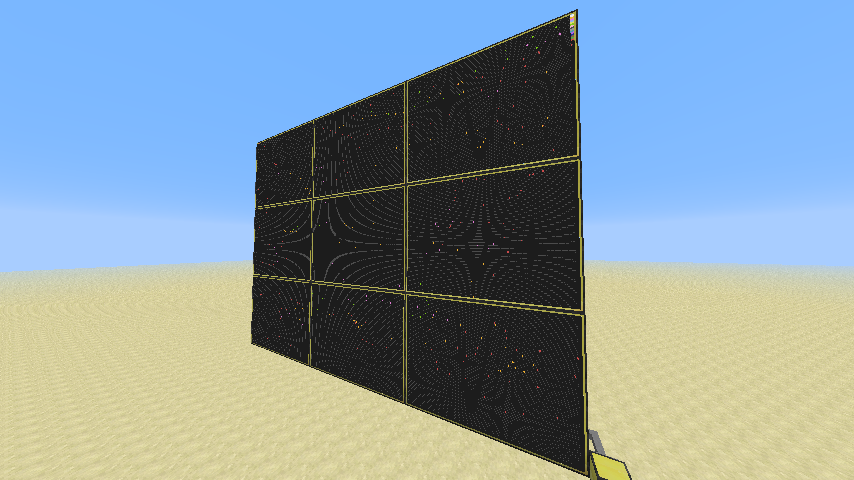
Playing worm (on the same setup):
Spoiler
--# Load the API
os.loadAPI('/multiMon')
--# Create the monitors object (the monitors are arranged in the world the same way you see them in the table below).
local monitorSetup = {
{peripheral.wrap('monitor_22'), peripheral.wrap('monitor_23'), peripheral.wrap('monitor_24')},
{peripheral.wrap('monitor_25'), peripheral.wrap('monitor_26'), peripheral.wrap('monitor_27')},
{peripheral.wrap('monitor_19'), peripheral.wrap('monitor_20'), peripheral.wrap('monitor_21')},
}
--# Create the virtual monitor (will also return the handle).
local disp = multiMon.create('myMonitor', monitorSetup)
--# Showcasing some basic functions.
disp.setTextScale(2)
if disp.isColour() then
disp.setTextColour(colours.white)
disp.setBackgroundColour(colours.black)
end
disp.setCursorBlink(false)
disp.clear()
disp.setCursorPos(1, 1)
local width, height = disp.getSize()
--# Since worm uses 'term' internally, we need to set 'disp' to act as 'term'.
term.redirect(disp)
--# Run the program.
shell.run('/rom/programs/fun/worm')
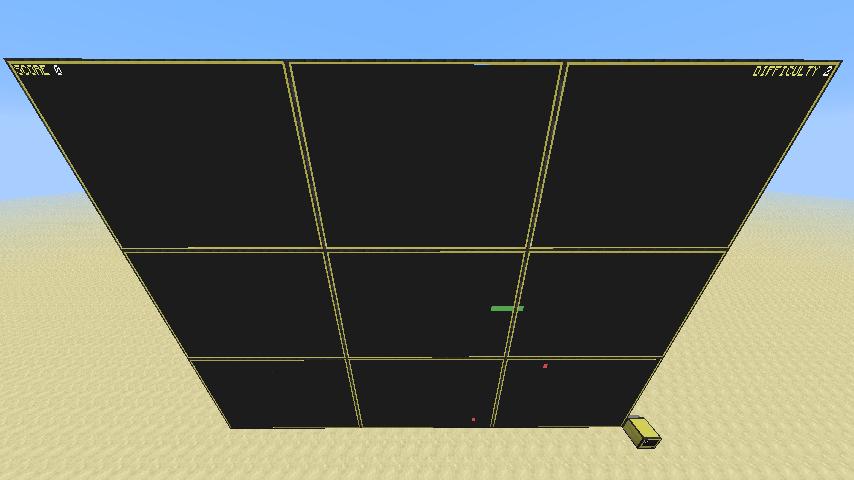
Download
pastebin get Yu9jAVuk multiMon
Changelog
Spoiler
v1.0- Initial release