The main feature is that it is very simple to use. There are no complicated server/client/router networks you have to set up, only a minimum of 3 computers to have a working network where 2+ computers can communicate. It also works with wireless/wired modems (both on the same network).
You need to host( ) on one computer, then other computers can connect to that, or other connected computers.
For example, this setup:
Computer 1:
network.host( )
Computer 2:
network.connect( "1" )
Computer 3:
network.connect( "2" )
local message = network.receive( )
print( "Got message from "..message:getSender( )..":" ) --> Got message from 3:
print( message:getMessage( ) ) --> Hello world!
message:respond( "Received!" )
Computer 4:
network.connect( "1" )
local response = network.send( "2>3", "Hello world!" )
print( "Got response: "..tostring( response:waitFor( 1 ) ) ) --> Got response: Received!
The encryption is *very secure* because it re-encrypts multiple times along it's route with keys of the IDs. This means only 1 computer at a time can read the message, not even the destination computer will be able to read it until it has travelled the full route. This does however add in an issue: it can lead to long messages causing lag because they are encrypted/decrypted multiple times. I may add in some fixes for this in the future (basically simplifying the encryption/decryption).
In addition to the network API, I have created some programs to use with it, and a couple of other APIs.
The other APIs:
- GUI - A very small GUI library aimed for simple web scripts.
- Script - A small script API that is formatted similar to HTML. It can only be used with the GUI API.
- Server - Act as a main server and allow other computers to communicate.
- Router - Act as a router, allowing computers to connect and route messages through it.
- Send - Send a string data to another computer.
- Receive - Receive messages and print their sender and data.
- DNS - Host a DNS server. Send {protocol = "DNS", request = "lookup" or "register", name = [the name for your address]}.
- FTP - Host a ftp server, with read and write commands. Send {protocol = "FTP", request = "read" or "write", path = [path to file], content = [content of file in write mode]}.
- WebServer - Host a website (websites are folders containing files to be loaded with the script API).
- Browser - A basic web browser, connect to a website and view/run it's contents.
Now I guess the main attraction of a networking API is websites (or emails I guess), but for making websites, use the following syntax:
<type param:value param2:value2>Content</type>
<row param:value...> children... </row>
<column param:value...> children... </column>
For example, to make an address bar:
<row size:n1>
<input id:n1 size:n47>web address</input>
<button size:n4 bcol:cyan tcol:white onClick:l"
local page = getAttribute( 1, "text" )
browser.gotopage( page )
">goto</button>
</row>
<text size:n3 bcol:lightBlue halign:centre>Type in the name of the page you want to visit then press goto</text>
This would output:Spoiler
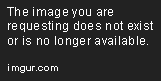
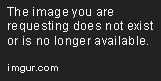
I will be making an interactive installer at some point, which will allow you to download different components individually or download everything at once, and also add in list and image elements into the GUI/script. I might also make a website creator and I will definitely improve the browser/web server programs to have a GUI, but that will probably come later.
Edit: made the installer: pastebin get yynKGXy1 install