[indent=13]v 2.4 Fixed a bug with [button]:remove()[/indent]
[indent=13]v 2.3 Added functions [button]:remove() and [bar]:setup()[/indent]
[indent=13]v 2.0 Added Dialogue Boxes[/indent]
[indent=13]v 1.2 Added Text-boxes[/indent]
Spoiler
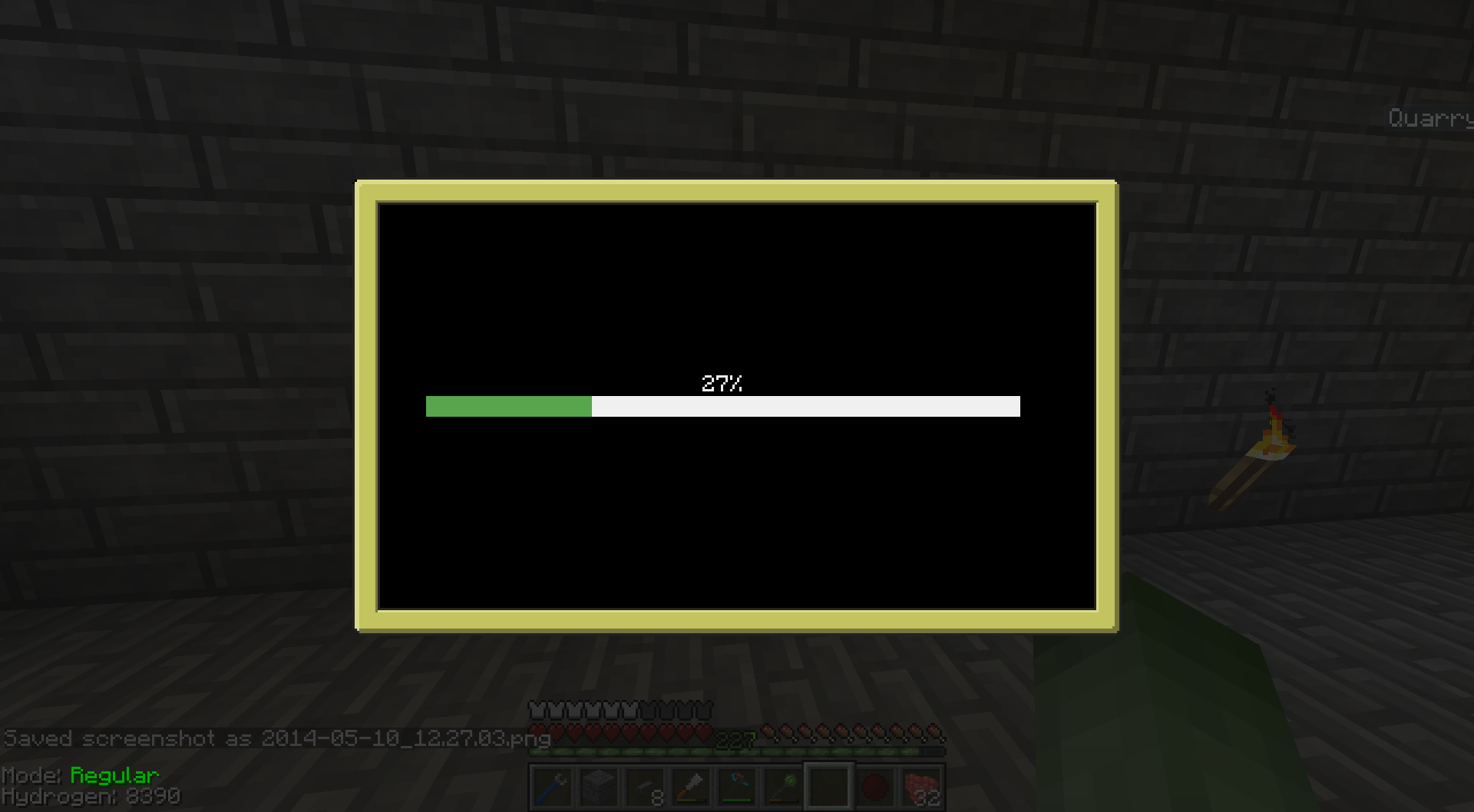
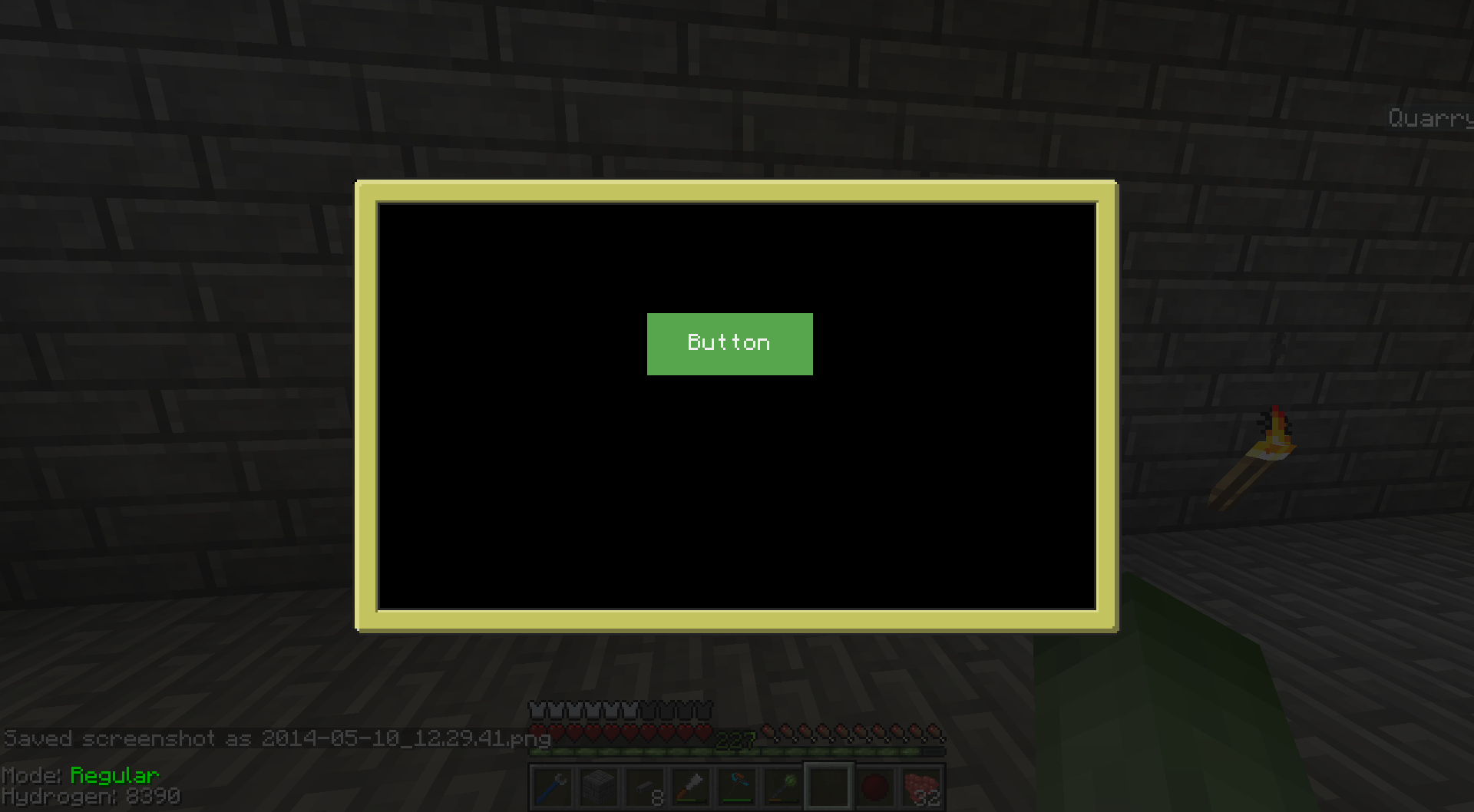
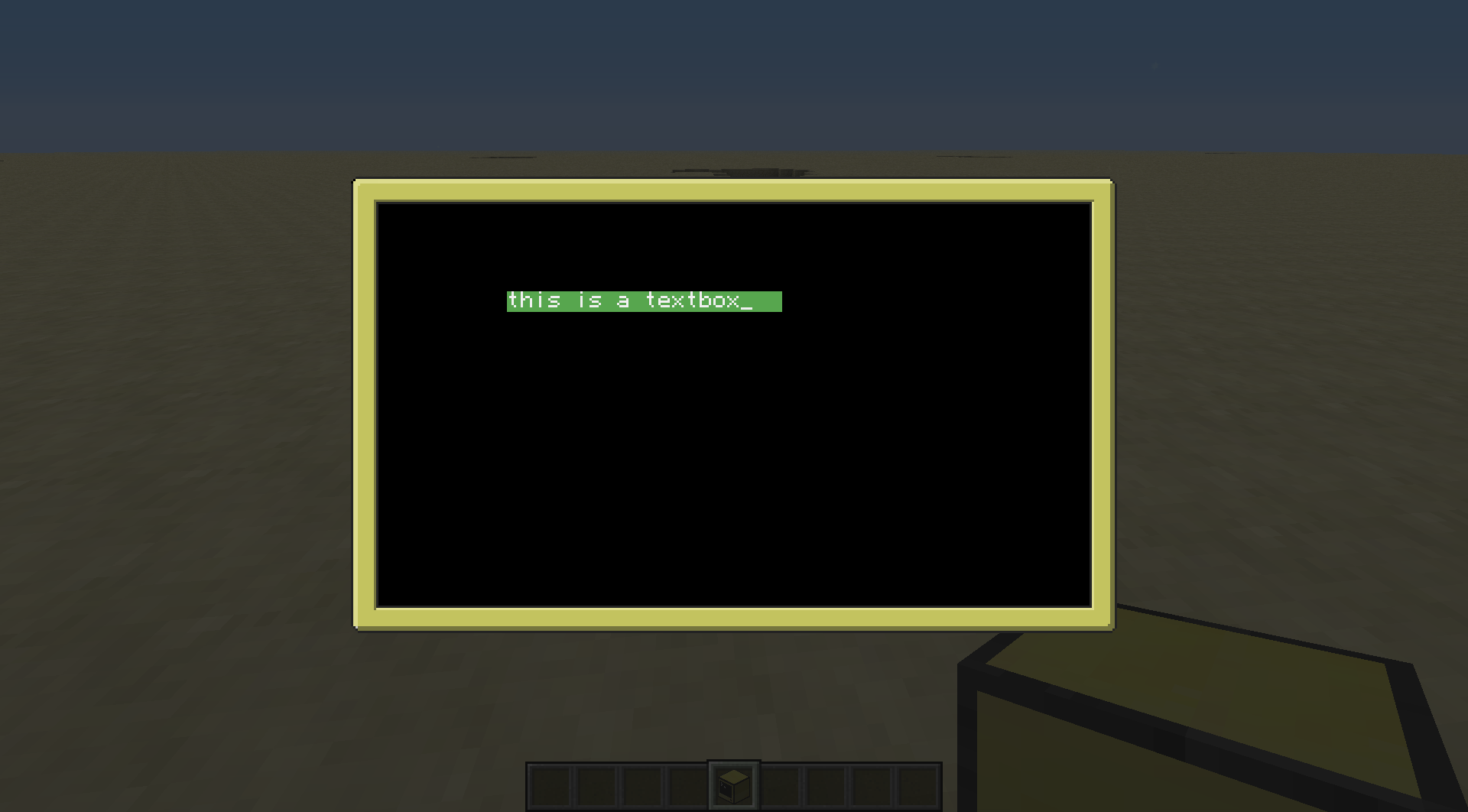
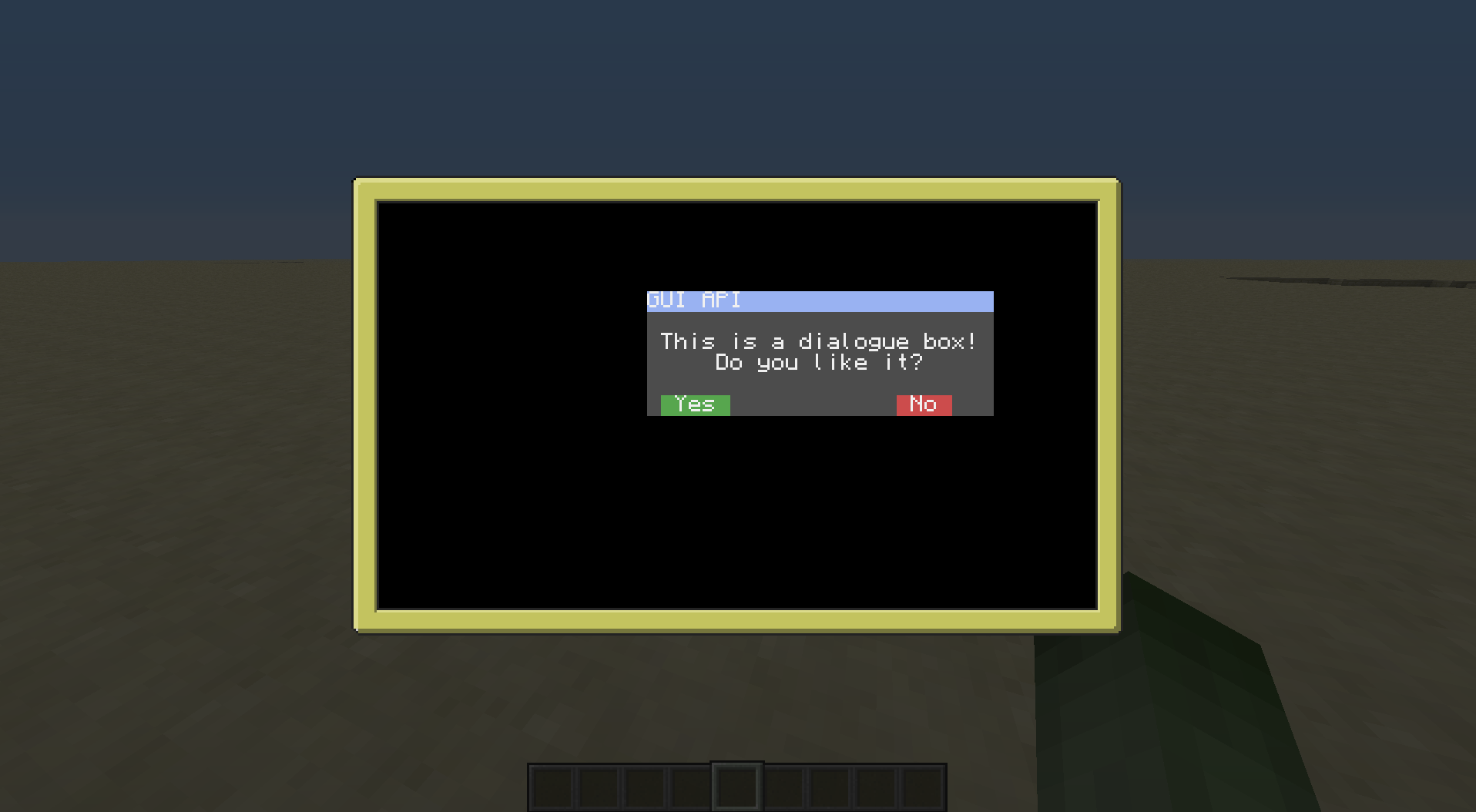
Spoiler
Buttons:
button = gui.createButton( "button", function() print("Hello World!") end ) --#This creates a new button with a function that when triggered will print "Hello World!"
button:draw( 1,1 5, colors.green, colors.white ) -- #This draws the button at (1,1) with a width of 5. The first color is what the buttons color will be and the second color is the text color
button:toggle( colors.red,4) --#This toggles the buttons color to red for 4 seconds (If you leave the second argument as nil it will just toggle the button)
button:trigger() --#This triggers the buttons function
gui.detect( 1,3,true ) --#this checks an array of all the buttons you've defined and returns the name of the button. If you also set the third argument to true it will trigger that buttons action
Progress Bars:
bar = gui.createBar( "bar" ) --#This creates a new bar with the name
bar:draw( 5,8,30,colors.white,colors.green,true,colors.black,colors.white ) --#This draws the bar at (5,8) with a length of 30. The first color is the bar color, the second color is the progress color, the next argument is whether or not to display the percent, the third color is the text background color (for displaying the percent) and the fifth is the percent text color.
bar:update( percent ) --#Re-draws the bar with the new percent
Text-boxes:
input = gui.newTextBox(2,5,25,colors.green,colors.white) --#Makes a text box at (2,5) with a length of 25, a green background color and a white text color.
Dialogue Boxes:
box = gui.createDialogueBox("GUI API",{"This is a dialogue box!","Do you like it?"},"yn") --#Creates a dialogue box with the title "GUI API" ,two body lines: "This is a dialogue box!" and "Do you like it?" and the box type is "yn"
box:draw( 20,5,5,colors.gray,colors.lightBlue,colors.white ) --#Makes a text box at (20,5) with a width of 5, a gray background color, white text color, and lightBlue title bar color.
Spoiler
Buttons:createButton( Name , Action [function] )
Creates a button with a name and trigger function.
detect( x, y, trigger? [true/false] )
Uses x and y args from a mouse click event and checks if any buttons were clicked. It can also trigger the buttons function if you set the "trigger?" arg to true.
[button]:draw( x , y, width, color, textColor )
Draws a button at the determined coordinates with the determined colors and length and then adds it to an array of all created and draw buttons.
[button]:toggle( toggleColor, Delay )
Changes the buttons color for a determined delay. If the "delay" argument is left as nil it will toggle and stay the toggle color.
[button]:trigger()
Activates the buttons "action" which is set when the button is created.
[button]:remove()
Removes button for the button array and deletes all info tied to that button.
Progress Bars:
createBar( name )
Creates a bar with a name.
[bar]:setup( x,y,length,barColor,progressColor,displayPercent? [true/false] ,percentBackgroundColor,textColor )
Sets up a bar to be drawn
[bar]:draw( x,y,length,barColor,progressColor,displayPercent? [true/false] ,percentBackgroundColor,textColor )
Draws a bar at the determined coordinates with the determined colors and with the determined length and then display a percent if you put true for the "displayPercent?" arg.
If you leave all the args as nil and have used :setup() it will draw it using what you put for :setup()
[bar]:update( percent )
Draws and updates the bar with the new percent.
Text-boxes:
newTextBox(x,y,length,backgroundColor,textColor)
Draws and selects a textbox at the determined coordinates with the determined colors and with the determined length.
Dialogue Boxes:
createDialogueBox( title,body,boxType )
Creates a dialogue box with a title, body text, and a box type. If you make the body a table you can have multiple lines.
The current box types are "yn" (yes or no returns true or false) or "ok" (returns true).
[box]:draw( x,y,width,backgroundColor,titleBackgroundColor,textColor )
Draws a dialogue box at the determined coordinates with the determined colors.
[box]:clear( color )
Clears a dialogue box of the screen with the determined color.