——————-——————-———————————————————————————————-——————————————————————
CHANGELOG:
March 21, 2017 - Fixed mekanism compatibility. added positive/negative change in stored energy. Modified how the currently stored energy is displayed
(may look into Zetta Industries mod compatibility)
I made a small computer program for advanced computers that tells you your power levels for energy cells(TE3), mfe,mfsu,batbox(ic2), enderIO capacitor banks and mekanism energy cubes.
-note: currently only works with single EnderIO capacitors . doesnt work with the EnderIO multiblock
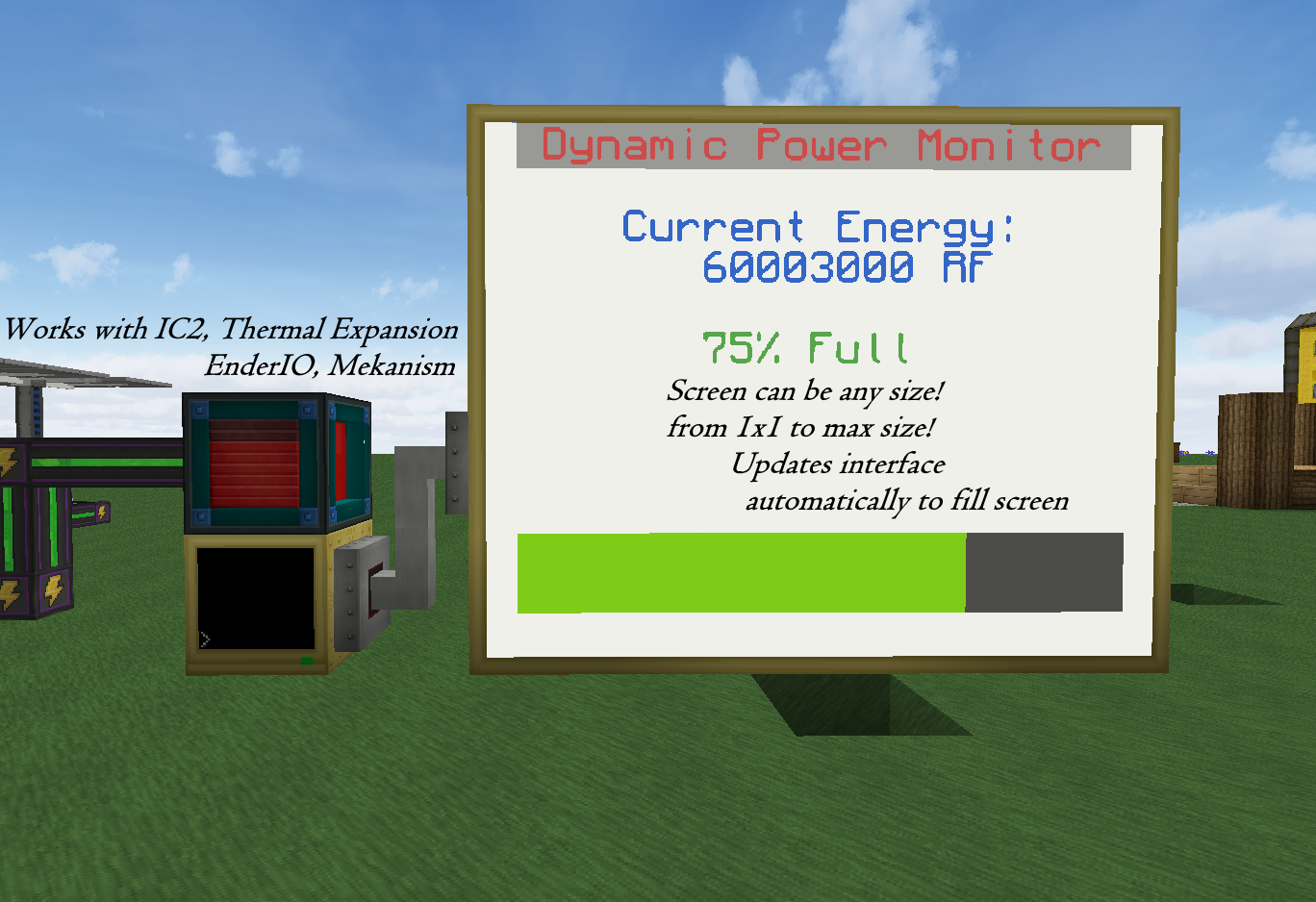
the program could work with any block that stores energy
To use the code just type: pastebin get 9b6m3ym1 install.lua in the terminal. note: must be advanced computer and advanced monitors!
then just run install.lua in the console. the installer will run and download the program for you
[Lua] Power Monitor Installer V:30 - Pastebin.com
[Lua] Power Monitor setup V:30 - Pastebin.com (note: you dont need to pastebin get this one. it is done automatically with the installation program)
[Lua] Power Monitor Program V:30 - Pastebin.com (note: you dont need to pastebin get this one. it is done automatically with the installation program)
Pictures Of Terminal
Spoiler
This is the setup walkthrough i made for it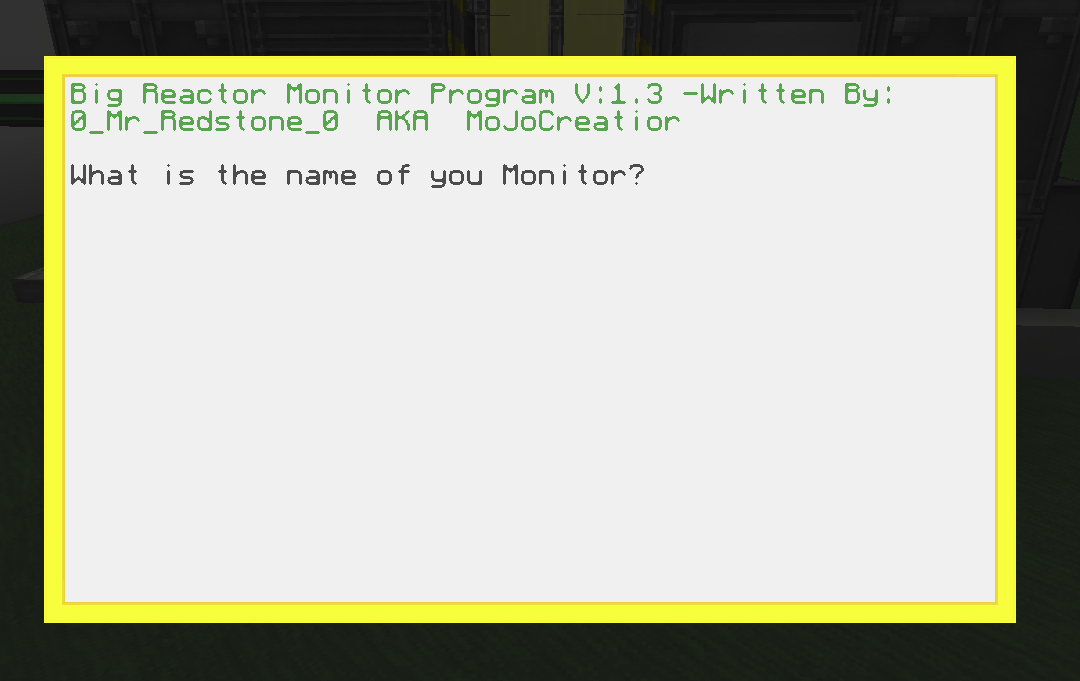
this is the information panel
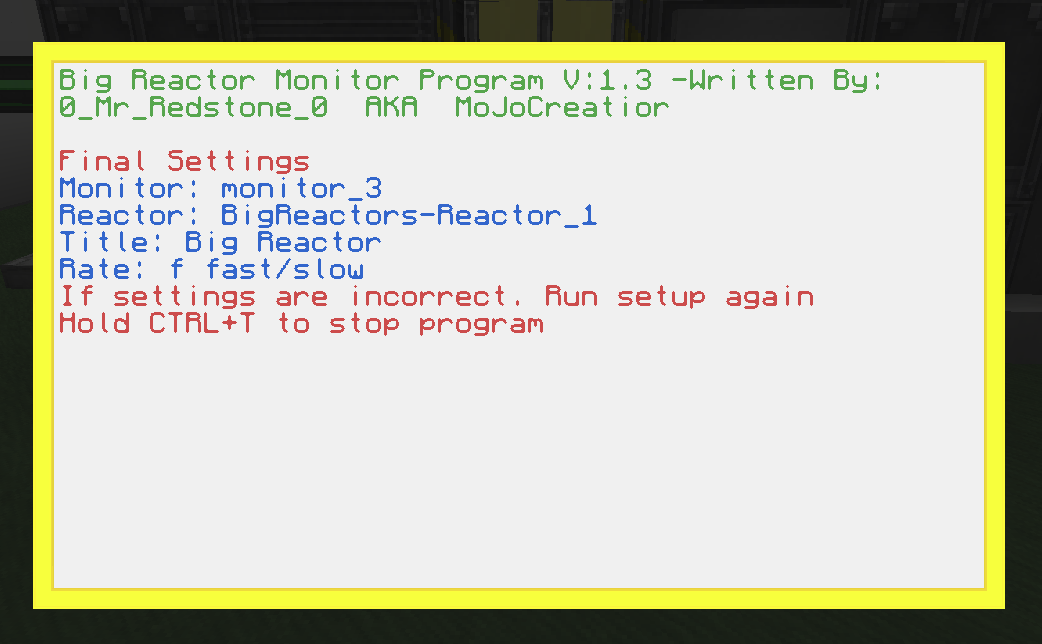
this is a basic setup.
Spoiler
note:
Spoiler
Setup Code
--[[ Power Monitoring Program Written By: 0_Mr_Redstone_0 AKA MoJoCreatior
This code is considered free for use both commercial and private and can be redistributed so long as it meats following criteria:
1: 0_Mr_Redstone_0 and MoJoCreatior are credited as the original authors
2: You do not try to take full ownership of the code/written program
3: If you modify the code for re-upload you must provide it under the same conditions. as in- Open Source, and credit me as original author]]
program = "Power Monitor"
setup = "f2ty2kg3"
program = "trrwXhMX"
--functions
function intro()
term.setBackgroundColour(colors.white)
term.clear()
term.setCursorPos(1,1)
term.setTextColour(colors.green)
print(program.." -Written By: 0_Mr_Redstone_0 AKA MoJoCreatior\n")
end
function install()
fs.delete("setup.lua")
fs.delete("program.lua")
fs.delete("config/")
shell.run("pastebin get "..setup.." setup.lua")
shell.run("pastebin get "..program.." program.lua")
fs.delete("startup")
startup = fs.open("startup","w")
startup.write('shell.run("setup.lua")')
startup.close()
if fs.exists("config/update") == true then print("Updated")
else print("Installed")
end
end
function configSave(name,data)
cfg = fs.open("config/"..name,"w")
cfg.write(data)
cfg.close()
end
function RFAPI()
rfAPI = false
if peripheral.find("tile_thermalexpansion_cell_resonant_name") == nil then
else cube = peripheral.find("tile_thermalexpansion_cell_resonant_name")
rfAPI = true
end
if peripheral.find("tile_blockcapacitorbank_name") == nil then
else cube = peripheral.find("tile_blockcapacitorbank_name")
rfAPI = true
end
end
function IC2Check()
IC2 = false
if peripheral.find("mfsu") == nil then
else cube = peripheral.find("mfsu")
IC2 = true
end
if peripheral.find("mfe") == nil then
else cube = peripheral.find("mfe")
IC2 = true
end
if peripheral.find("cesu") == nil then
else cube = peripheral.find("cesu")
IC2 = true
end
if peripheral.find("batbox") == nil then
else cube = peripheral.find("batbox")
IC2 = true
end
end
function mekanismCheck()
mekanism = false
if peripheral.find("Basic Energy Cube") == nil then
else cube = peripheral.find("Basic Energy Cube")
mekanism = true
end
if peripheral.find("Advanced Energy Cube") == nil then
else cube = peripheral.find("Advanced Energy Cube")
mekanism = true
end
if peripheral.find("Elite Energy Cube") == nil then
else cube = peripheral.find("Elite Energy Cube")
mekanism = true
end
if peripheral.find("Ultimate Energy Cube") == nil then
else cube = peripheral.find("Ultimate Energy Cube")
mekanism = true
end
if peripheral.find("Induction Matrix") == nil then
else cube = peripheral.find("Induction Matrix")
mekanism = true
end
end
RFAPI()
IC2Check()
mekanismCheck()
intro()
if peripheral.find("monitor") == nil then
print("Connect Monitor!") print("terminating") sleep(2.5) os.reboot()
end
if rfAPI == true then
elseif IC2 == true then
elseif mekanism == true then
else print("Connect Energy Cell!") print("terminating") sleep(2.5) os.reboot()
end
install()
os.reboot()
Program Code
--[[ Power Monitoring Program Written By: 0_Mr_Redstone_0 AKA MoJoCreatior
This code is considered free for use both commercial and private and can be redistributed so long as it meats following criteria:
1: 0_Mr_Redstone_0 and MoJoCreatior are credited as the original authors
2: You do not try to take full ownership of the code/written program
3: If you modify the code for re-upload you must provide it under the same conditions. as in- Open Source, and credited to me as original author]]
program = "Power Monitoring Program V:30"
--Function
function intro()
term.setBackgroundColour(colors.white)
term.clear()
term.setCursorPos(1,1)
term.setTextColour(colors.green)
print(program.." -Written By: 0_Mr_Redstone_0 AKA MoJoCreatior")
print(" ")
end
function centerText(text,yVal)
length = string.len(text)
minus = math.floor(monX-length)
x = math.floor(minus/2)
mon.setCursorPos(x+1,yVal)
mon.write(text)
end
function centerTextC(text,line,colorB,colorT)
mon.setBackgroundColour(colorB)
mon.setTextColour(colorT)
length = string.len(text)
minus = math.floor(monX-length)
x = math.floor(minus/2)
mon.setCursorPos(x+1,line)
mon.write(text)
end
function clearScreen()
mon.setBackgroundColour(convert(colorMB))
mon.clear()
end
function config(name)
if fs.exists("config/"..name) then
cfg = fs.open("config/"..name,"r")
name = cfg.readAll()
cfg.close()
return name
else
fs.delete("startup")
startup = fs.open("startup","w")
startup.write('shell.run("setup.lua")')
startup.close()
term.setTextColour(colors.red)
print("ERROR! 404")
print(name.." config not found")
term.setTextColour(colors.blue)
print("rebooting into setup")
print("3") sleep(1)
print("2") sleep(1)
print("1") sleep(1)
os.reboot()
end
end
function textScale()
mon.setTextScale(0.5)
monX,monY = mon.getSize()
if monX <= 16 or monY <= 11 then mon.setTextScale(0.5) elseif
monX <= 37 or monY <= 25 then mon.setTextScale(1) elseif
monX <= 58 or monY <= 39 then mon.setTextScale(1.5) elseif
monX <= 80 or monY <= 53 then mon.setTextScale(2) elseif
monX <= 101 or monY <= 68 then mon.setTextScale(2.5) else
mon.setTextScale(3)
end
end
function IC2Check()
IC2 = false
if peripheral.find("mfsu") == nil then
else cube = peripheral.find("mfsu")
IC2 = true
end
if peripheral.find("mfe") == nil then
else cube = peripheral.find("mfe")
IC2 = true
end
if peripheral.find("cesu") == nil then
else cube = peripheral.find("cesu")
IC2 = true
end
if peripheral.find("batbox") == nil then
else cube = peripheral.find("batbox")
IC2 = true
end
end
function mekanismCheck()
mekanism = false
if peripheral.find("Basic Energy Cube") == nil then
else cube = peripheral.find("Basic Energy Cube")
mekanism = true
end
if peripheral.find("Advanced Energy Cube") == nil then
else cube = peripheral.find("Advanced Energy Cube")
mekanism = true
end
if peripheral.find("Elite Energy Cube") == nil then
else cube = peripheral.find("Elite Energy Cube")
mekanism = true
end
if peripheral.find("Ultimate Energy Cube") == nil then
else cube = peripheral.find("Ultimate Energy Cube")
mekanism = true
end
if peripheral.find("Induction Matrix") == nil then
else cube = peripheral.find("Induction Matrix")
mekanism = true
end
end
function RFAPI()
if peripheral.find("tile_thermalexpansion_cell_resonant_name") == nil then
else cube = peripheral.find("tile_thermalexpansion_cell_resonant_name")
end
if peripheral.find("tile_blockcapacitorbank_name") == nil then
else cube = peripheral.find("tile_blockcapacitorbank_name")
end
end
function convert(color)
if color == "white" then color = colors.white end
if color == "orange" then color = colors.orange end
if color == "magenta" then color = colors.magenta end
if color == "lightBlue" then color = colors.lightBlue end
if color == "yellow" then color = colors.yellow end
if color == "lime" then color = colors.lime end
if color == "pinkgray" then color = colors.pinkgray end
if color == "gray" then color = colors.gray end
if color == "lightGray" then color = colors.lightGray end
if color == "cyan" then color = colors.cyan end
if color == "purple" then color = colors.purple end
if color == "blue" then color = colors.blue end
if color == "brown" then color = colors.brown end
if color == "green" then color = colors.green end
if color == "red" then color = colors.red end
if color == "black" then color = colors.black end
return color
end
function eChange()
newE = curE
local difference = (newE-oldE)
oldE = curE
if rate == "f" then dividend = 20 elseif rate == "s" then dividend = 100 end
local change = math.floor(difference/dividend)
return(change)
end
--PreFace
--Color Coneverter
--Load Settings
title = config("title")
rate = config("rate")
dynamic = config("dynamic")
colorTB = config("colorTB")
colorTT = config("colorTT")
colorMB = config("colorMB")
colorST = config("colorST")
colorBT = config("colorBT")
colorBE = config("colorBE")
colorBF = config("colorBF")
colorPR = config("colorPR")
colorPos = config("colorPos")
colorNeg = config("colorNeg")
redstone = config("redstone")
side = config("side")
bundled = config("bundled")
options = config("options")
IC2Check()
mekanismCheck()
RFAPI()
--Shows Settings inside control computer
intro()
term.setTextColour(colors.red)
print("Final Settings")
term.setTextColour(colors.blue)
print(" Title: "..title)
if rate == "f" then print(" Refresh Rate: fast") else print(" Refresh Rate: slow") end
if mekanism == true then print(" Mekanism: true") else print(" Mekanism: false") end
if IC2 == true then print(" IC2: true") else print(" IC2: false") end
if dynamic == "y" then print(" Dynamic Scaling: true") else print(" Dynamic Scaling: false") end
if options == "y" then
term.setTextColour(colors.red)
print("\nAdvanced Options")
term.setTextColour(colors.blue)
print(" Title Text Color: "..colorTT)
print(" Title Background: "..colorTB)
print(" Background Color: "..colorMB)
print(" Statistics Color: "..colorST)
print(" Bar Percent Text: "..colorBT)
print(" Bars Empty Color: "..colorBE)
print(" Bar Filled Color: "..colorBF)
print(" Positive Change: "..colorPos)
print(" Negative Change: "..colorNeg)
end
term.setTextColour(colors.gray)
print("Million < Billion < Trillion < Quadrillion")
term.setTextColour(colors.red)
print("\nIf settings are incorrect. Run setup.lua")
print("Hold CTRL+T to stop program")
--RF/EU label
if IC2 == true then energyAPI = " EU" else energyAPI = " RF" end
if IC2 == true then energyAPI_ = "EU" else energyAPI_ = "RF" end
--Wraps the Monitor
mon = peripheral.find("monitor")
monX,monY = mon.getSize()
textScale()
monX,monY = mon.getSize()
oldE,newE,curE,maxE = 0,0,0,0
while true do
if dynamic == "y" then textScale() end
--Main Program
--Variables for Calculations
if mekanism == true then maxE = cube.getMaxEnergy() or 1
elseif IC2 == true then maxE = cube.getEUCapacity() or 1
else maxE = cube.getMaxEnergyStored() or 1
end
if mekanism == true then curE = cube.getEnergy() or 1
elseif IC2 == true then curE = cube.getEUStored() or 1
else curE = cube.getEnergyStored() or 1
end
if mekanism == true then
maxE = math.floor(maxE/2.5)
curE = math.floor(curE/2.5)
end
percent = math.floor(((curE/maxE)*100)+0.5)
bar = math.floor(((curE/maxE)*(monX-2))+0.5)
stats = curE
--Redstone Output
if redstone == "y" then
if bundled == "1" then
if percent >= 99 then
rs.setBundledOutput(side,convert(colorPR))
else rs.setBundledOutput(side,0)
end
else
if percent >= 99 then
rs.setOutput(side,true)
else rs.setOutput(side,false)
end
end
end
--Centers and Displays Title On Monitor
clearScreen()
mon.setBackgroundColour(colors.black)
mon.setTextColour(colors.lightGray)
mon.setCursorPos(1,1)
centerTextC(string.rep(" ",string.len(title)+2),1,convert(colorTB),convert(colorTT))
centerTextC(title,1,convert(colorTB),convert(colorTT))
--Monitor Statistics
--[[if curE >= 1000000 and curE <= 1000000000 then
stats = math.floor(stats/1000)
stats = (stats/1000)
mbtq = " M"
else]]if curE >= 1000000000 and curE <= 1000000000000 then
stats = math.floor(stats/100000)
stats = (stats/1000)
mbtq = " B"
elseif curE >= 1000000000000 and curE <= 1000000000000000 then
stats = math.floor(stats/100000000)
stats = (stats/1000)
mbtq = " T"
elseif curE >= 1000000000000000 then
stats = math.floor(stats/100000000000)
stats = (stats/1000)
mbtq = " Q"
elseif curE <= 1000000 then mbtq = " "
end
mon.setBackgroundColour(convert(colorMB))
mon.setTextColour(convert(colorST))
if curE >= 1000000 then eAPI = energyAPI_ else eAPI = energyAPI end
centerText(stats..mbtq..eAPI,3)
--[[
if monX >= math.floor(string.len(stats)+9)
then
centerText("Total:"..stats..mbtq..eAPI,3)
else
centerText("Total:",3)
centerText(stats..mbtq..eAPI,4)
end
]]
--Change Per Tick Calculator and Display
e_Change = eChange()
if e_Change >= 0 then sign = "+" elseif e_Change <= 0 then sign = "-" end
if e_Change >= 0 then signC = colorPos elseif e_Change <= 0 then signC = colorNeg end
centerTextC(sign..e_Change..energyAPI.."/t",5,convert(colorMB),convert(signC))
centerTextC(percent.."% Full",monY-3,convert(colorMB),convert(colorBT))
--Loading Bar Code Re-Written Much MUCH simpler than before
mon.setCursorPos(2,monY-2)
mon.setBackgroundColour(convert(colorBE))
mon.write(string.rep(" ",monX-2))
mon.setCursorPos(2,monY-2)
mon.setBackgroundColour(convert(colorBF))
mon.write(string.rep(" ",bar))
mon.setCursorPos(2,monY-1)
mon.setBackgroundColour(convert(colorBE))
mon.write(string.rep(" ",monX-2))
mon.setCursorPos(2,monY-1)
mon.setBackgroundColour(convert(colorBF))
mon.write(string.rep(" ",bar))
if rate == "f" then os.sleep(1)
elseif rate == "s" then os.sleep(5)
else os.sleep(5) end
end
Setup Code
--[[ Power Monitoring Program Written By: 0_Mr_Redstone_0 AKA MoJoCreatior
This code is considered free for use both commercial and private and can be redistributed so long as it meats following criteria:
1: 0_Mr_Redstone_0 and MoJoCreatior are credited as the original authors
2: You do not try to take full ownership of the code/written program
3: If you modify the code for re-upload you must provide it under the same conditions. as in- Open Source, and credit me as original author]]
program = "Power Monitoring Setup V:21"
--functions
function intro()
term.setBackgroundColour(colors.white)
term.clear()
term.setCursorPos(1,1)
term.setTextColour(colors.green)
print(program.." -Written By: 0_Mr_Redstone_0 AKA MoJoCreatior\n")
term.setTextColour(colors.orange)
print("Hold 'CTRL+R' to start over\n")
end
function setup(question,file,binary,subText)
term.setBackgroundColour(colors.white)
term.clear()
intro()
term.setTextColour(colors.gray)
print(question)
if binary == 1 then
term.setTextColour(colors.lightGray)
print(subText)
end
term.setTextColour(colors.cyan)
fs.delete("config/"..file)
cfg = fs.open("config/"..file,"w")
answer = io.read()
cfg.write(answer)
cfg.close()
end
function setupSub(question,file,binary,subText)
term.setTextColour(colors.gray)
print(question)
if binary == 1 then
term.setTextColour(colors.lightGray)
print(subText)
end
term.setTextColour(colors.cyan)
fs.delete("config/"..file)
cfg = fs.open("config/"..file,"w")
answer = io.read()
cfg.write(answer)
cfg.close()
end
function update()
fs.delete("startup")
startup = fs.open("startup","w")
startup.write("shell.run('install.lua')")
startup.close()
end
function config(name)
cfg = fs.open("config/"..name,"r")
name = cfg.readAll()
cfg.close()
return name
end
function configSave(name,data)
cfg = fs.open("config/"..name,"w")
cfg.write(data)
cfg.close()
end
--Setup
if not fs.exists("install.lua") then shell.run("pastebin get 9b6m3ym1 install.lua") end
intro()
if fs.exists("config/update") then setup("Do you want to Update?","update",1," [y/n]") updateV = answer end
configSave("update","true")
if updateV == "y" then update() os.reboot() end
setup("Would you like Advanced Options?","options",1,"[y/n]\n Allows you to set Custom Colors")
setup("What do you want the Title to be?","title",1,"Titles will appear off screen if made too long")
if config("options") == "y" then
setupSub("\nWhat color should the Titles Background be?","colorTB",1," Case Sensitive")
setupSub("\nWhat color should the Titles Text be?","colorTT",1," Case Sensitive")
setup("What color should the Monitor Background be?","colorMB",1," Case Sensitive")
setupSub("\nWhat color should the Energy Statistics be?","colorST",1," Case Sensitive")
setupSub("\nWhat color should the Empty Bar be?","colorBE",1," Case Sensitive")
setupSub("\nWhat color should the Filled Bar be?","colorBF",1," Case Sensitive")
setupSub("\nWhat color should the % Filled be?","colorBT",1," Case Sensitive")
setupSub("\nWhat color should positive change be?","colorPos",1," Case Sensitive")
setupSub("\nWhat color should negative change be?","colorNeg",1," Case Sensitive")
else
configSave("colorTB","black")
configSave("colorTT","white")
configSave("colorMB","white")
configSave("colorST","blue")
configSave("colorBE","gray")
configSave("colorBF","lime")
configSave("colorBT","cyan")
configSave("colorPos","green")
configSave("colorNeg","red")
end
setup("Would you like to enable Redstone Output?","redstone",1," [y/n]")
if config("redstone") == "y" then
setupSub("Are you using -1-Project:Red Bundled Cable or -2-Vanilla Redstone?","bundled",1," [1/2]\n You can choose Bundled Cable Color\n as well the side the output is on")
setupSub("What side of the computer should the output be on?","side",1," right,left,front,back,top,bottom")
if config("bundled") == "1" then
setupSub("What color cable should the output be on?","colorPR",1," Case Sensitive")
else configSave("colorPR","0")
end
else
configSave("bundled","0")
configSave("side","0")
configSave("colorPR","0")
end
setup("Should the Monitor Refresh be fast or slow?","rate",1," [f/s]\n fast is every 1 second\n slow is every 5 seconds")
setup("Would you like Dynamic Text Scaling to be turned on?","dynamic",1,"[y/n]\n Makes Text Scaling update as\n you Change the monitor size")
intro()
print("Auto Restart in 3 seconds!") sleep(1)
print("Auto Restart in 2 seconds!") sleep(1)
print("Auto Restart in 1 seconds!") sleep(1)
print("Restarting...")
startup = fs.open("startup","w")
startup.write('shell.run("program.lua")')
startup.close()
os.reboot()
More Info
using side name such as "top" and "back" in the name input doesn't currently works perfectly. DO NOT USE THE QUOTES!
All peripherals should automatically connect, because of this make sure to only have one of each peripheral connected to the same network, otherwise the program will use the first one it finds and skip the rest.
Feel free to ask questions of needed. or just leave any suggestions for the code. :)/>/&gt;
EDIT: Fixed Images
EDIT 2: Added more documentation
EDIT 13: applied major update
EDIT 15: Added full color support
EDIT 17: Updated spoiler with new code, fixed some documentation