i have finished my program to control turbines of a big reactor (http://big-reactors.com/).
It implements a standard PID controller to change the turbine speed and display some status informations. A second program collect all status informations of any reachable turbine control program and displays a table to console and any attached monitor. This master controller is able to change speed of any turbine controller.
Version history:
- v0.1, 2014-12-29: initial version
- v0.2, 2015-01-02: some improvements (pocket computers) and bugfixes
- v0.3, 2015-01-05: Support terminal glasses and bugfixes
The PID controller switch the turbine between three different states: OFF, 900 RPM and 1800 RPM. It displays some status informations to the console and any attached monitor. A master controller is able to collect any turbine information and it is able to change the turbine status.
1.1 Installation and setup
Attach a computer to the computer port (direct or with a wired modem). Label your computer with some unique name:
label set Turbine_01
Download the program ans save it as "startup":
pastebin get gTEBHv3D startup
old v0.1
pastebin get kfWVibVF startup
Start the control program with "startup". It shows the following screen:

Now, you can type in "m" or "f" to start up the turbine. You may also type in "o" to switch the turbine off. The program tries to reach the desired speed by changing the maximum steam float.
You can attach a monitor to any side of the control computer to show some operation stats. You may also attach a monitor with a wired modem. A 1x1-Monitor will do the work. It then shows the following:


In the first line it shows the operation state: off, 900 RPM or 1800 RPM. The third line shows the actual speed of the turbine. The fourth and fifth line informs about the maximum and actual steam flow.
The maximum steam flow is the configured value of the turbine. The actual steam flow is the real flow the turbine get by the connected reactor at this time. If the actual flow is less than the maximum flow and the turbine does not reach its target speed, the turbine needs more flow to operate.
A normal computer and monitor will work. If you use the advanced ones, the display uses colors. If you use bigger monitors, the program try to scales up the status display:

I you use a newer version of Big Reactor (>= v0.3.4A), the turbines have a disengage coils feature. The controller use this for faster startup. If the coild are disengaged the turbine does not produce energy anymore. If you want to disable this feature, have a look at line 46 of the code and change the variable "useDisengageCoils" to "false":
46: local useDisengageCoils = true
1.2 Networking
The turbine controller is able to send its status informations to a central master controller (see section 2). It also change the operating speed by network request.
By default, the remote information feature is enabled and the remote control is disabled.
1.2.1 Security issues
The networking feature not implemented any security feature at all. If you use this features in a multi player environment, you should trust the other users. By default configuration they can only use the information feature of the controller, if you attach a modem.
1.2.2 Disable remote information feature.
If you want to avoid that other users are able to collect the informations of your turbines, you can disable the sending of this informations. Edit the file "startup" at line 34 and set the variable "stateRequestChannel" to 0:
34: local stateRequestChannel = 0 -- Broadcast channel.
The controller would not send any status informations to attached modems anymore. You don't need to do this, if you don't attach any modem to the computer.
1.2.3 Enable remote control feature
If you want to control the turbine by the master control program (see section 2), you have to edit the file "startup" at line 40 and set the variable "remoteControl" to "true":
40: local remoteControl = true
1.2.4 Use the network
You can simply attach a wired or wireless modem at the controller computer. The controller will use any modem to collect information of control requests.
1.2.5 Use the network feature for your own programs
The network code using the modem API. I don't use the rednet API, because it always use only the first found modem to send messages.
To receive turbine informations, you have to send "BR_turbine_get_state" to the configured broadcast channel (stateRequestChannel = 32768, see line 34 of the code). Use your computerID as reply channel and open this channel to recieve the reply:
peripheral.call(modemSide, "open", os.getComputerID())
peripheral.call(modemSide, "transmit", 32768, os.getComputerID(), "BR_turbine_get_state")
The turbine controller send back the status informations to your reply channel.
You should decode and check the reply:
local rState = textutils.unserialize(tostring(recievedValue))
if rState ~= nil
and type(rState) == "table"
and rState.version ~= nil
and rState.version == "Turbine V0.1"
then
...
end
Have a look at line 590, to see what type of values you will recieve.
To change the turbine speed, you can simply send the new speed with the prefix "BR_turbine_set_speed:" to the controller:
local speed=900
peripheral.call(modemSide, "transmit", turbineComputerId, os.getComputerID(), "BR_turbine_set_speed:" .. speed)
The speed should be 0, 900 oder 1800 to set the turbine target speeds: off, 900 RPM and 1800 RPM. In the example it would send "BR_turbine_set_speed:900". The turbine controller just change the target speed and replies nothing.
1.4 Reconfigure your turbine.
The first time you use the control program, it measures the startup speed of your turbine and save this as a basic configuration into the file "turbine_crtl.save". If you change the setup of your turbine (make it bigger or change the coils), this values are no longer suitable to operate the controller.
Simply remove the file "turbine_crtl.save" after you change your turbine setup. The controller will measure the new startup speed again.
1.5 Customization (Monitor)
If you want to change the informations at the monitor, you can do that by changing the function "displayStateOnMonitor" beginning at line 326.
If you need a bigger screen to display your information, you can change the scale functionality at line 329:
local width, height = scaleMonitor(mon, 15, 16, 5, 5)
This line scales the monitor up and down. You find the documentation of this function beginning at the line 280 of the program.
2. The master controller
The master control program will collect informations of any reachable turbine controller. If the remote control feature of the turbine controller is enabled (see section 1.2.3), you are able to change the operation state of this turbine controller.
2.1 Installation and setup
Place a computer anywhere and set a unique label:
label set Turbine_Master_Control
Download the program and save it as "startup":
pastebin get RcBGNSgb startup
old v0.2
pastebin get rg1auFSE startup
old v0.1
pastebin get F5JPrFUU startup
Then attach a wired ore wireless modem to the master computer and any turbine controller. If you use wired modems, you should connect them by wires, of cause.
After starting the master control program with "startup", it sends broadcast requests to any attached modem. If a turbine controller received this broadcast message, it sends the status informations of the turbine back to the master controller. The master controller displays a table with the collected informations:

The columns:
- Label: The label of the turbine computer. The +/- indicates the status of the turbine (active/deactive) for normal computers without colors
- TRPM: The target speed. This is the operation status of the turbine controller: 900 RPM, 1800 RPM and OFF
- ARPM: The actual speed of the turbine. If it is orange, the turbine does not reach the target speed. If it is red, the turbine is too fast (> 2000 RPM)
- MFlow: The maximum steam flow, the turbine is configured with
- AFlow: The actual steam flow of the turbine (explained in section 1.1)
- kRF/t: Actual energy production of the turbine
- Energy: Load of the internal energy cell

You may also use bigger monitors. The program tries to scale the output:

If you use smaller monitors, the program only shows a few of the columns:


By using an advanced monitor, you can cycle between the different informations by clicking the table header or the last line.
Since v0.2 you can also use a wireless pocket computer as a master controller:

You may also cycle between different informations by clicking the table header or the last line on advanced pocket computers. Or you can simply press the space key, with also works on normal pocket computers.
2.2 Change operating state of turbine controllers
To change the operating state of the displayed turbine controllers, the controllers need the remote control feature enabled (see section 1.2.3). You also need an advanced computer or monitor to change the control state.
To change the state of a turbine controller, simply click with the mouse at the displayed line (right click at monitors, right or left click at the console) and a change dialog box appears:

The last line of the box shows the different operation states of the turbine controller. The actual configured state is marked by a blue background color.
Simply click to any of this states. The box will disappear and the controller will change its control state. If you click outside, the box simply disappears without changing the operation state of the turbine controller.
2.3 OpenPeripheral Terminal Glasses
Since v0.3 the maser supports Terminal Glasses of OpenPeripheral. Simply attach a Terminal Glasses Bridge to your computer and register your terminal glasses by right click with it an the bridge. Then your HUD schould show this:
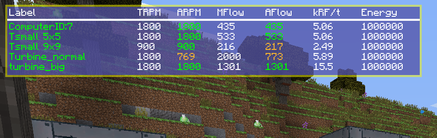
It also supports some chat commands to hide the HUD, change the speed of a turbine or show some detailed informations:
- $$help: Shows a box with the chat commandy you can use
- $$hide: Hide the HUD display
- $$show: With no parameters, it shows the table again. If you use a label of a turbine as parameter it shows a detailed information screen for this turbine.
- $$speed: Set the target speed of the turbine. First parameter ist the new speed (off, 900 or 1800). Second parameter is the label of the turbine computer.
$$help
shows this: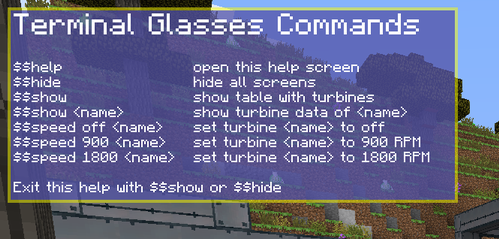
$$show Tsmall 5x5
shows this: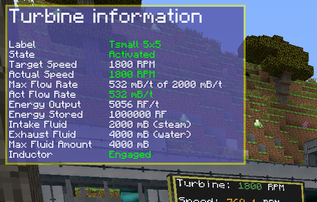
Or simply change the target speed:
$$speed 900 Tsmall 5x5
I you want to place the HUD to another location, have a look at line 37 of the code. If you change the posX and posY values to move the HUD to anjother place. The Terminal Glasses don't give informations about the screen size, so i was not able to align the HUD to the bottom or right side of the screen.
2.4 Customization (Monitor)
If you want to change the output of the table, you can change the function "displayTableOnMonitor" beginning at line 294 of the program. As the turbine control program, it use the function "scaleMonitor" (see line 305) to scale the monitor output. If you want to use bigger or smaller tables, you should change the parameters. The description of "scaleMonitor" can be found at line 230 of the code.
If you change the table header height (two lines) or the position from top, you have to change the calculations in function "changeSpeed" (beginning at line 1203) also. To insert additional informations into the cycle for small monitors, you can change them in function "doCycleInformations" (beginning in line 1174).
The turbine controller send any known status information of the turbine to the controller. So, in most cases, you don't have to change the protocol. Have a look at line 590 of the turbine controller program, to see what type of informations are send. If you need any additional information, simply add it to the "rState" table at this location.
I hope, my poor english skills are not too annoying.
Have fun, kla_sch