It uses the least amount of drawing calls possible to reduce lag and tearing.
It is compatible with all displays, but if the display only supports black and white
then you can only use colors.black and colors.white.
A surface is a texture that you can draw to.
It has 3 layers: the character, background color and text color layer.
The data of those 3 layers are stored in one sequential buffer so it uses the least amount of RAM.
Surfaces are wrapped in tables with the width, height, buffer and functions in it.
There are many useful functions, such as: clearing the surface, drawing text, pixels, lines, rectangles, scaling surfaces and more!
You can also load and save surfaces with the NFP format for the built-in paint,
or the more advanced NFT format which also supports text for programs such as NPaintPro and Sketch.
This is a complete replacement for the build-in paintutils API and maybe even the term API.
There is no error checking to keep the performance high, so if you get errors check if you have correct inputs.
You can download the source here: (34.35kb)
pastebin get 5YWfPd8Z surface
All functions are documented here:
https://github.com/CrazedProgrammer/SurfaceAPI/wiki (click on the function name to get to the documentation)
And then you can simply load it using os.loadAPI:
os.loadAPI("surface")
This program will display Hello, world! for two seconds with an orange background and blue text:
-- Loads the surface API
os.loadAPI("surface")
-- Creates a new surface to draw on:
-- surface.create(width, height, char, backcolor, textcolor)
local surf = surface.create(51, 19, " ", colors.orange, colors.blue)
-- Draws text at the desired location:
-- surf:drawText(x, y, text, backcolor, textcolor)
surf:drawText(3, 3, "Hello, world!")
-- Draws the surface to the screen:
-- surface.render(surface, display, x, y, sx1, sy1, sx2, sy2)
surf:render(term)
-- Waits for two seconds
os.sleep(2)
Screenshot: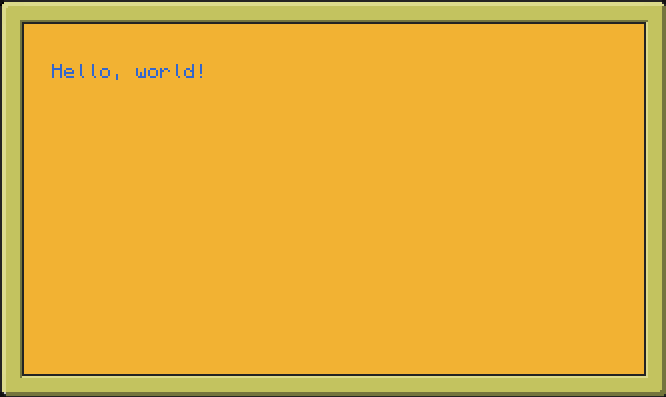
Surfaces also support transparency.
Because surfaces have 3 layers, you can choose which layers you change by nil-ing out the ones you don’t.
For example if you only want to change the background color:
surf:drawPixel(3, 3, nil, colors.black)
If you want to overwrite all layers then you can set surf.overwrite to true.
surf.overwrite = true
Here is an example of what you can do with the scaling and rotating algorithm:
-- Loads the surface API
os.loadAPI("surface")
-- Creates an orange surface
local surf = surface.create(51, 19, " ", colors.orange, colors.white)
-- Loads a picture of mario from the file mario.nft
local surf2 = surface.load("mario.nft")
-- Draws a small mario
surf:drawSurfaceScaled(2, 2, 7, 9, surf2)
-- Draws a normal mario
surf:drawSurface(11, 2, surf2)
-- Draws a rotated mario
surf:drawSurfaceRotated(33, 10, 7, 9, 0.4, surf2)
-- Draws the surface to the computer display
surf:render(term)
-- Waits for two seconds
os.sleep(2)
Screenshot: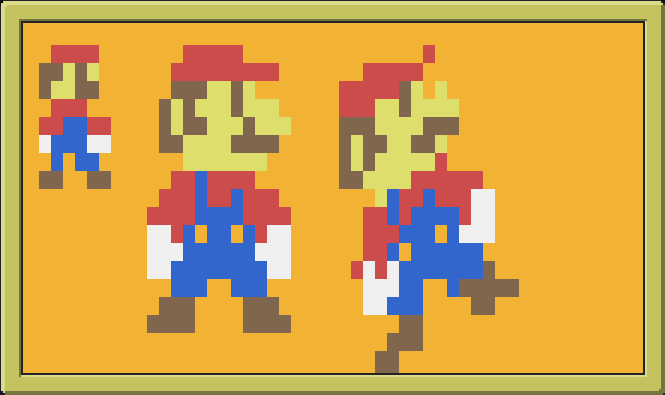
If you want to save disk space, you can delete functions that you don’t use in the API code.
However, some functions require other functions to function.
You can view the dependencies in the function documentation.
Changelog:
Version 1.6.2:
- Fixed term.scroll, term.clearLine and term.setCursorBlink from surf:getTerm
Version 1.6.0:
- Added surf:drawHLine and surf:drawVLine
- Added the ability to remove functions from the API code to save disk space (look at the function documentation for dependencies)
- Removed all global references (surface.*) so you can load the API as something else than "surface" or load it locally
Version 1.5.3:
- Fixed surf:render function
Version 1.5.2:
- Big performance improvements (about 80% on surf:render)
Version 1.5.1:
- Fixed surf:drawArc, surf:drawPie and surf:fillPie using inverted angles
Version 1.5.0:
- Added surf:getBounds
- Added surf:copy
- Added surf:getTerm
- Added surf:drawRoundedRect and surf:fillRoundedRect
- Added surf:drawArc
- Added surf:drawPie and surf:fillPie
- Added surf:shader
- Added surf:shift
- Fixed a couple bugs
Version 1.4.0:
- Completely rewritten and optimized
- New version numbering and variable: surface.version
- Added surf:drawRoundRect and surf:fillRoundRect
Version 1.3:
- Added term.blit support
- surf:render no longer throws an error with transparent surfaces
- Added a new image format: SRF (only uses hex characters and underscores)
- Added surface.loadString and surf:saveString
- Added surf:drawLines
- Added surf:drawTriangles and surf:fillTriangles
Version 1.2:
- Added surf:drawSurfaceRotated
Version 1.1:
- Replaced surf:drawToDisplay with surf:render
- Added surf:setBounds
- Added surf:drawTriangle and surf:fillTriangle
- Added surf:drawEllipse and surf:fillEllipse
- Added surf:floodFill
Older version downloads:
1.5.3: pastebin get J2Y288mW surface
1.4.0: pastebin get vm1y156i surface
1.3: pastebin get ajFj7yNK surface
1.2: pastebin get hwAbAgLD surface
1.1: pastebin get AmmVZusd surface
1.0: pastebin get g87xZ4dA surface
If you have any suggestions, bug reports or questions then please leave a reply or send a PM.