Another "just for giggles"-type-script. This one's an API for Command Computers; somewhat similar to the window API, it offers one function, "create", which returns a terminal object.
The object in concern is the sky.
For now, only your current background colour is rendered (using wool blocks), though this still allows for some interesting "drawings" to be made.
Usage
skyTerm.create(number x, number y, number z, string facing, number width, number height [, number transCol]) => table terminal objectThe x/y/z co-ords indicate the world position of the top-left-most point of the display. "facing" must be a compass direction (eg, "north", "south", etc). The "transCol", if specified, will be rendered as air.
Because you'll typically want to be able to see your main display while using this, you'll probably want to create an aggregated terminal object out of it, eg:
Example
os.loadAPI("skyTerm")
local x, y, z, facing = -330, 94, 298, "east"
do
local displays = {term.current(), skyTerm.create(x, y, z, facing, 51, 19)}
local multiTerm = {}
for funcName,_ in pairs(displays[1]) do
multiTerm[funcName] = function(...)
for i=2,#displays do displays[i][funcName](unpack(arg)) end
if displays[1][funcName] then return displays[1][funcName](unpack(arg)) end
end
end
term.redirect(multiTerm)
end
term.clear()
term.setCursorPos(1,1)
shell.run("shell")
It does have a rather notable flaw in that, because commands.execAsync() can't be trusted to execute asynchronously, it needs to yield on all writes. This means you'll need the likes of the parallel API to use it reliably alongside eg timers and the like.
Screenshots
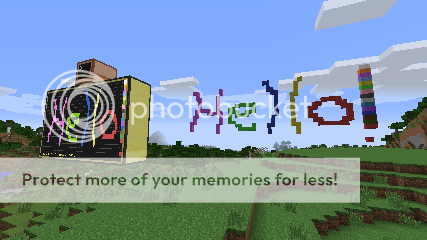
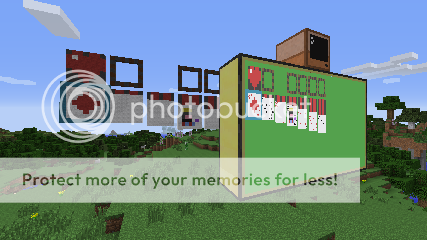
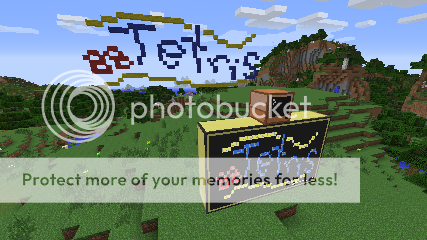