Posted 18 May 2015 - 12:40 AM
Object API
[indent=1]This API allows you to create buttons, check boxes, texts, images and processes as objects.[/indent]
You can download via Pastebin by dint of the command:
pastebin get 8bF64AVh APIs/object
Than you need to load this API to your program. You can do this with the standart function:
os.loadAPI( "APIs/object" )
General Functions
Spoiler
The Object API have some general functions.Creating a new object:
Button = object.New.Button( table term, number xPos, number yPos, number width, number height [, string textOnTheButton, number textColor, number buttonColor, number activeButtonColor, number inactiveButtonColor, boolean visible] )
CheckBox = object.New.CheckBox( table term, number xPos, number yPos [, string textNearCheckBox, number textColor, number backgroundTextColor, number checkBoxColor, number activeCheckBoxColor, number inactiveCheckBoxColor, boolean state, boolean visible] )
Image = object.New.Image( table term, number xPos, number yPos, number width, number height, string Path or table image [, number backgroundColor, string borderStyle, number borderColor, number xOffset, number yOffset, boolean visible] )
Text = object.New.Label( table term, number xPos, number yPos [, string text, number textColor, number backgroundColor, string stateNearPoint, boolean visible] )
Process = object.New.Process( table term, number xPos, number yPos, number width, number height, string pathToProgram, shell [, multishell, string path to workingDirectory, argumentsForProgram] )
All functions in the table object.New return objects.Function that returns the type of an object or nil if parameter is not an object:
object.Type( table object )
Function that returns the amount of created objects:
object.Count( [string typeOfTheObject] )
If you fill the parameter in this function you will get the amount of specific objects.All kinds of objects have their own functions.
Button Object
Spoiler
At first you need to create the button object:
Button = object.New.Button( table term, number xPos, number yPos, number width, number height [, string textOnTheButton, number textColor, number buttonColor, number activeButtonColor, number inactiveButtonColor, boolean visible] )
You will get the button as result. Let's analyze all parameters in this function:- term is a term object where the button will be put. It can be the default term, monitor or window,
- xPos and yPos are coordinates,
- width and height are width and height of course,
- textOnTheButton is text on the button,
- textColor the color of the text,
- buttonColor is button color,
- activeButtonColor is clicked button color,
- inactiveButtonColor is color for inactive buttons that can't be clicked,
- visible is the special state. If it is false object won't be drawed on the term or monitor.
Button.Draw( [string state] )
This finction draws the button. If you write "active" in the state parameter it draws clicked button. If you write "inactive" it draws button in inactive state.The next is
Button.GetVisibility()
It returns the visibility state.Another function sets the visibility state.
Button.SetVisibility( boolean state )
Other function is
Button.SetPosition( number xPos, number yPos [, number width, number height] )
It sets the button position, width and height.
Button.GetPosition()
Returns the button position, width and height.
Button.GetText()
Returns the button's text.
Button.SetText( [string buttonText] )
Sets the text on the button.
Button.SetColors( [number textColor, number buttonColor, number activeButtonColor,number inactiveButtonColor] )
Sets the colors of the button.
Button.GetColors()
Returns the button's colors in order like in Button.SetColors() function.
Button.Detect( string action, number mouseButton [, number x, number y] )
This function detects clicking, dragging, scrolling and touching. It have several parameters:- action is the os.pullEvent() action. It can be "mouse_click", "mouse_drag", "mouse_scroll" and "monitor_touch".
- mouseButton needs for "mouse_click" and "mouse_drag" for detecting right mouse button.
- x and y are very usefull if you have many buttons or check boxes. If you have this parameters Button.Detect() will not use os.pullEvent() function inside.
Spoiler
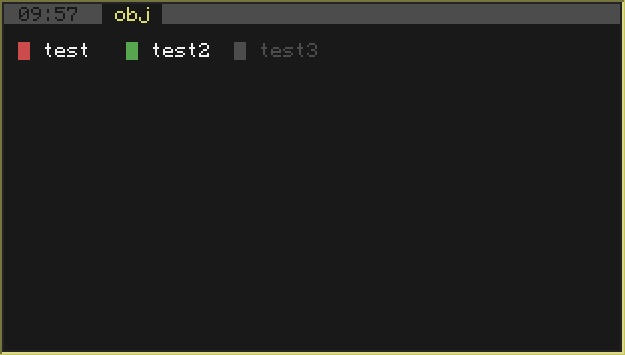
Three buttons: standart, activated, and inactive.
Code Examples
Spoiler
The program on the screen below.
term.clear()
os.loadAPI("apis/object")
local B1=object.New.Button(term.current(), 2, 2, 7, 2,"test")
local B2=object.New.Button(term.current(), 11, 2, 7, 2,"test2")
local B3=object.New.Button(term.current(), 20, 2, 7, 2,"test3")
B2.Draw("active")
B3.Draw("inactive")
if B1.Detect("mouse_click", 1) then
print("Everything is working!")
end
Check Box
Spoiler
At first you need to create the check box object:
CheckBox = object.New.CheckBox( table term, number xPos, number yPos [, string textNearCheckBox, number textColor, number backgroundTextColor, number checkBoxColor, number activeCheckBoxColor, number inactiveCheckBoxColor, boolean state, boolean visible] )
You will get the check box as result. Let's analyze all parameters in this function:- term is a term object where the check box will be put. It can be the default term, monitor or window,
- xPos and yPos are coordinates,
- textNearCheckBox is text near the check box of course,
- textColor is the color of the text,
- backgroundTextColor is the text background color,
- checkBoxColor is the standart check box color,
- activeCheckBoxColor is the color of checked check box,
- inactiveCheckBoxColor is the color of useless check box,
- state is the state of check box. It can be true or false,
- visible is the button's visibility state.
CheckBox.Draw( [string state] )
This function draws the check box. If you write "active" in the state parameter it draws checked check box. If you write "inactive" it draws useless check box.The next is
CheckBox.GetVisibility()
It returns the visibility state.Another function sets the visibility state.
CheckBox.SetVisibility( boolean state )
Other function is
CheckBox.SetPosition( number xPos, number yPos )
It sets the check box position.
CheckBox.GetPosition()
Returns the check box position.
CheckBox.GetText()
Returns the check box's text.
CheckBox.SetText( [string buttonText] )
Sets the text on the check box.
CheckBox.ChangeState( [boolean state] )
Switches the check box's state. If the state parameter filled with true or false the state will be switched to true or false respectively.
CheckBox.GetState()
Returns the check box's state.
CheckBox.SetColors( [number textColor, number backgroundTextColor, number checkBoxColor, number activeCheckBoxColor, number inactiveCheckBoxColor] )
Sets the colors of the check box.
CheckBox.GetColors()
Returns the check box's colors in order like in CheckBox.SetColors() function.
CheckBox.Detect( string action, number mouseButton [, number x, number y] )
This function detects clicking, dragging, scrolling and touching. It have several parameters:- action is the os.pullEvent() action. It can be "mouse_click", "mouse_drag", "mouse_scroll" and "monitor_touch".
- mouseButton needs for "mouse_click" and "mouse_drag" for detecting right mouse button.
- x and y are very usefull if you have many buttons or check boxes. If you have this parameters Button.Detect() will not use os.pullEvent() function inside.
Spoiler
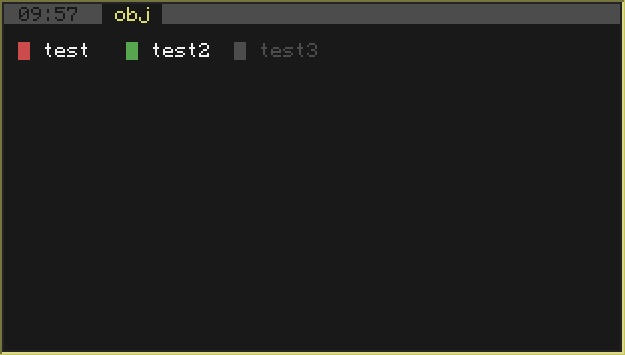
Three check boxes in three states.
Spoiler
Working check box.
term.clear()
os.loadAPI("apis/object")
local CheckBox=object.New.CheckBox(term.current(), 2, 2,"test")
while true do
local a, b = CheckBox.Detect("mouse_click", 1)
if b then
CheckBox.SetText("checked ")
else
CheckBox.SetText("unchecked")
end
end
Image
Spoiler
At first you need to create the check box object:
Image = object.New.Image( table term, number xPos, number yPos, number width, number height, string Path or table image [, number backgroundColor, string borderStyle, number borderColor, number xOffset, number yOffset, boolean visible] )
You can see many parameters in this function:- term is a term object where the image will be put. It can be the default term, monitor or window,
- xPos and yPos are coordinates,
- width and height are width and height,
- path or image is used for image. You can write there a path to an image or table with image,
- If image is transparent somewhere the parameter backgroundColor will be used for that places,
- borderStyle parameter is used for border style. It can be "none", "box" and "lines",
- borderColor is color of the border.
- xOffset is parameter for moving image along the x axis,
- yOffset is parameter for moving image along the y axis,
- visible is the visibility parameter.
Functions.
Image.Draw()
Draws the image object.
Image.GetVisibility()
Returns the visibility state.
Image.SetVisibility( boolean state )
Sets the visibility state.
Image.SetPosition( number xPos, number yPos [, number width, number height] )
Sets the image position, width and height.
Image.GetPosition()
Returns the image position, width and height.
Image.SetColors( [number backColor, number borderColor])
Sets the images colors.
Image.GetColors()
Returns the colors in order like in Image.SetColors() function.
Image.SetBorderStyle( string borderStyle )
Sets the image's border style. It can be "none", "box" and "lines".
Image.GetBorderStyle()
Returns the border style.
Image.GetImage( string )
Returns image as a table if the parameter is "table" or nil. Returns path to the image if the parameter is "path".
image.SetImage( string path or table image )
Sets a new image for the image object.
Image.GetOffset()
Returns the image's offset.
Image.SetOffset( number xOffset, number yOffset )
Sets a new offset.
Image.AddOffset( number xOffset, number yOffset )
Adds numbers to the image's offset.
Image.Detect( string action, number mouseButton [, number x, number y] )
Works like Button.Detect().Screenshots
Spoiler
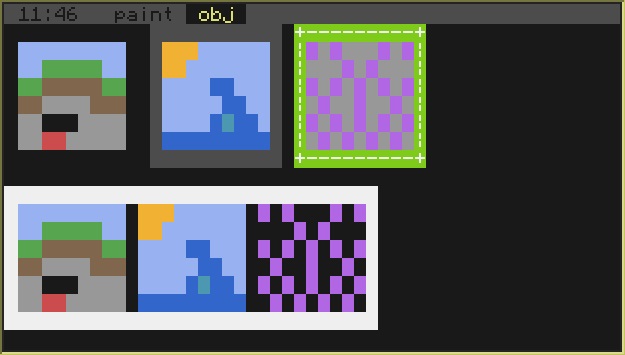
Four images with different parameters.
Spoiler
term.clear()
os.loadAPI("apis/object")
local t = term.current()
local Image1 = object.New.Image(t, 2, 2, 8, 5, "1")
local Image2 = object.New.Image(t, 14, 2, 8, 5, "1", nil, "box", nil, 10)
local Image3 = object.New.Image(t, 26, 2, 8, 5, "1", colors.lightGray, "lines", colors.lime, 20)
local Image4 = object.New.Image(t, 2, 11, 28, 5, "1", nil, "box", colors.white)
local action = "mouse_click"
local event, param1, param2, param3 = os.pullEvent(action)
print(Image1.GetOffset()," "..Image2.GetOffset(),"")
if Image1.Detect("mouse_click", 1, param2, param3) then
Image1.AddOffset(1,1)
Image1.Draw()
end
if Image2.Detect("mouse_click", 1, param2, param3) then
Image2.AddOffset(1,1)
Image2.Draw()
end
if Image3.Detect("mouse_click", 1, param2, param3) then
Image3.AddOffset(1,1)
Image3.Draw()
end
This program load all images from the one file.Label
Spoiler
At first you need to create a new label object.
Label = object.New.Label( table term, number xPos, number yPos [, string text, number textColor, number backgroundColor, string stateNearPoint, boolean visible] )
- term is a term object where the label object will be put. It can be the default term, monitor or window,
- xPos and yPos are coordinates,
- text is a text on the label object,
- textColor is a color of the text,
- backgroundColor is a background text color,
- stateNearPoint is the label location near its coordinates point. It can be "right", "left" or "middle",
- visible is the visibility state.
Label.Draw()
Draws the label.
Label.GetVisibility()
Returns the visibility state.
Label.SetVisibility( boolean state )
Sets the visibility state.
Label.SetPosition( number xPos, number yPos )
Sets the label's position.
Label.GetPosition()
Returns the label position.
Label.SetColors( [number textColor, number backgroundColor] )
Sets the colors of the label object.
Label.SetText( string text )
Sets a new text for label object.
Label.GetText()
Returns the text from the label object.
Label.SetState( string state )
Sets a new state near point. It can be "middle", "right" or "left".
label.GetState()
Returns the label state.Screenshots
Spoiler
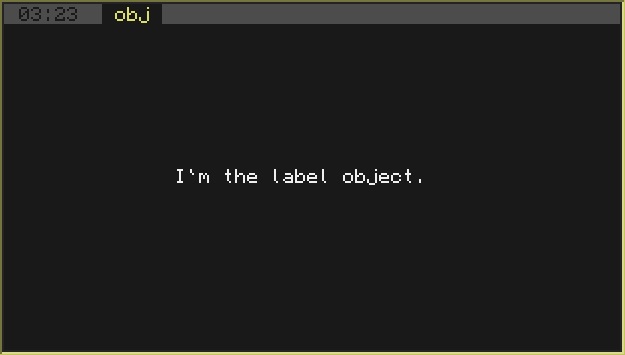
The standart label with text.
Spoiler
term.clear()
os.loadAPI("apis/object")
local t = term.current()
local w, h = term.getSize()
local Text1 = object.New.Label(t, w/2, h/2, "I'm the label object.")
os.sleep(1)
term.clear()
Text1.SetState("right")
os.sleep(1)
term.clear()
Text1.SetState("left")
The simple text changes its position near its point.Process
Spoiler
At first you need to create a process object.
Process = object.New.Process( table term, number xPos, number yPos, number width, number height, string pathToProgram, shell [, multishell, string workingDirectory, arguments] )
- term is a parent term for the process's window,
- xPos and yPos are coordinates for the process's window,
- width and height are window's width and height,
- pathToProgram is a path to a program,
- shell is the shell API. Don't asking a questions. Just write there shell,
- multishell is the multishell API. If you use the multishell in your program write there multishell.
- workingDirectory is a path to a working directory. If not stated the process will use "/Temp" directory.
- arguments are arguments for program.
Process.Start( boolean state )
Starts the process and returns success as boolean. if you fill the parameter with state false process will start in the background mode. If the process have not any arguments it will also return error.
Process.SetPosition( number xPos, number yPos [, number width, number height] )
Changes the process's window position, width and height.
Process.GetPosition()
Returns the process's position, width and height.
Process.SetProcess( string pathToProgram, arguments )
Sets a program path and its arguments.
Process.GetProcess()
Returns the path to the program and its arguments.
process.GetRunning()
Returns the process's running state. It might be very usefull if you run the process as coroutine.
process.SetWorkDir( string workingDirectory )
Sets a new working directory.
Process.GetWorkDir()
Returns the process's working directory.Screenshots
Spoiler
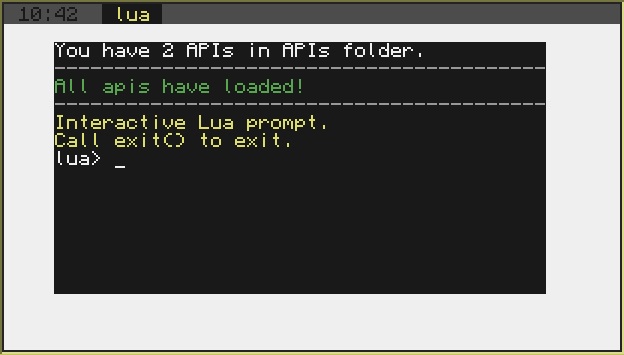
My program works via object API on the white field.
Spoiler
term.setBackgroundColor(colors.white)
term.clear()
os.loadAPI("apis/object")
local w, h = term.getSize()
local t = term.current()
local Process = object.New.Process(t, 5, 2, w-10, h-4, "<program>", shell, multishell, "/")
local ok, err = Process.Start()
if not ok then
error(err)
end
term.setBackgroundColor(colors.black)
term.clear()
term.setCursorPos(1,1)
Starts a program on the white field.Planed features
- Text box object
- Image list object
- If you starts one process many times you will get many lags. I don't know why.