38 posts
Location
Somewhere near keyboard
Posted 15 November 2015 - 05:32 PM
Hello. I've made simple application framework to make UI coding a bit easier.
For example, it does all drawing and event-handling functions for you.
Also, UI drawing functions are run on separate thread (kind of semi-multitasking) from main application code.
What have I implemented so far:- Application class, it can run both console and GUI code
- Screen class, which represents UI container
- Button
- Label
- EditText
- ProgressBar
- ComboBox
- CheckBox
- ScrollView
- ImageView
- Dialogs:
- Nested containers
- Application title bar at bottom
Downloads:latest (stable) version - pastebin get
FYAvjNKm libAAF
nightly version - download from
my GitHubDocumentation:https://github.com/A...ork-for-CC/wikiCode examples:Simple GUI application:
Spoiler
-- Load app framework API
os.loadAPI("/libAAF")
-- Create application instance
local app=libAAF.Application.new()
-- Create window
local win=libAAF.Screen.new()
-- Make our application know which window to use
app.appWindow=win
-- Main application code function
AppMain = function()
-- Create new label
local labelHello = libAAF.Label.new()
-- Set label's text
labelHello.text="Hello from Application!"
-- Attach label to window
win:add(labelHello)
-- Then, we'll create a simple button
local buttonExit = libAAF.Button.new()
-- Set its text
buttonExit.text="Exit"
-- Set its position, so it won't overlap our label
buttonExit.x=3
buttonExit.y=3
-- Add click listener to it, so when we click it, application will close
buttonExit.onMouseClick=function(self)
app:dispose()
end
-- And finally, attach button to our window
win:add(buttonExit)
end
-- Start application
app:start(AppMain)
Simple console application:
Spoiler
-- Load API
os.loadAPI("/libAAF")
-- Create application instance
local app=libAAF.Application.new()
-- So, we won't add screen to app, it'll only run this code
AppMain=function()
print("Hello from Application!")
end
-- Start our app
app:start(AppMain)
I would be very glad if you tell me your opinion on it.
Edited on 19 November 2015 - 05:00 PM
797 posts
Posted 15 November 2015 - 06:20 PM
Huh, this is structured very similarly to my own UI framework, which at least to me is really great. I'd love to see some documentation though, it's quite hard to tell what this can actually do just from what you've said here. I'd recommend getting the documentation done, and then maybe adding more elements in.
I can't tell if you can have nested components, but if you can, good job! If not, it's pretty essential when making big layouts, so I'd work on that. Also, not sure if you can move elements round at any time, or if you need to tell it to manually redraw. Are things buffered? So many unanswered questions! This certainly has potential though, just work on getting the documentation done.
Edited on 15 November 2015 - 06:13 PM
38 posts
Location
Somewhere near keyboard
Posted 15 November 2015 - 06:29 PM
Okay, I haven't started working on documentation yet, just because I haven't added many features.
It doesn't have buffering, and it is redrawn automatically: I have while loop, in which I draw my components, then pull events from OS. Also, there's event to do manual redraw (can be sent from parallel thread).
At the moment it doesn't have nested components, but I think it's easy to implement (maybe something like Panel or Container). Thanks for feedback.
38 posts
Location
Somewhere near keyboard
Posted 15 November 2015 - 07:07 PM
Okay, now I've added ComboBoxes, now working to add nested components, or containers.
Screenshots of ComboBox (also featuring Alert window):
Spoiler
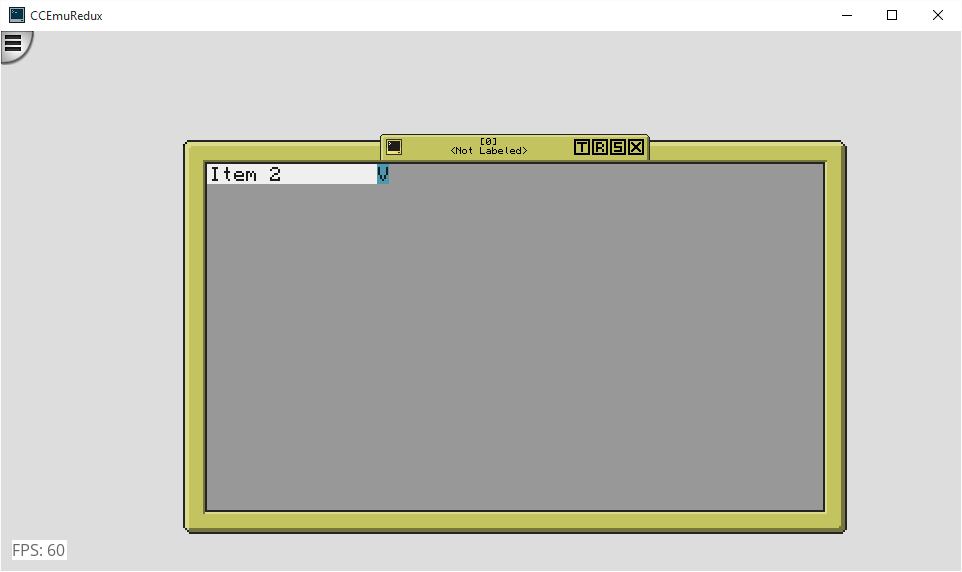
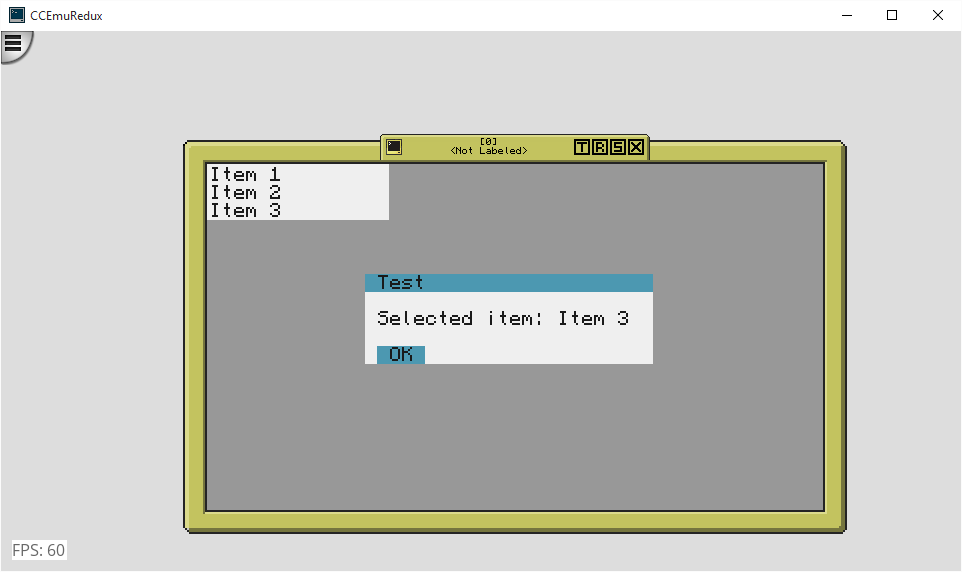
797 posts
Posted 15 November 2015 - 07:14 PM
Lack of features isn't a reason to delay the documentation. I know it's a pain to write, but it's essential if you want people to use your stuff.
The combo box looks cool, any chance of some screenshots of the other components?
38 posts
Location
Somewhere near keyboard
Posted 15 November 2015 - 07:18 PM
Okay, actually, I've taken your suggestion into my consideration, so now I'm writing documentation, it'll be done soon, in my GitHub repo.
38 posts
Location
Somewhere near keyboard
Posted 16 November 2015 - 04:58 PM
Okay, documentation is done now.
Also, I've finally got nested components working:
You can add Button inside a Container inside a Container inside a Container inside a Screen inside an Application.
Edit: now doing some screenshots, as you've asked.
Edited on 16 November 2015 - 04:02 PM
38 posts
Location
Somewhere near keyboard
Posted 16 November 2015 - 08:07 PM
Added ImageView (can be used as canvas too, as it has own graphics), CheckBox. Also, now AAF uses Surface API by CrazedProgrammer, because it has buffering and sub-surfaces.
Images loaded into ImageView can be transparent.
Screenshot of ImageView (tested with .nft, .nfp):
Spoiler
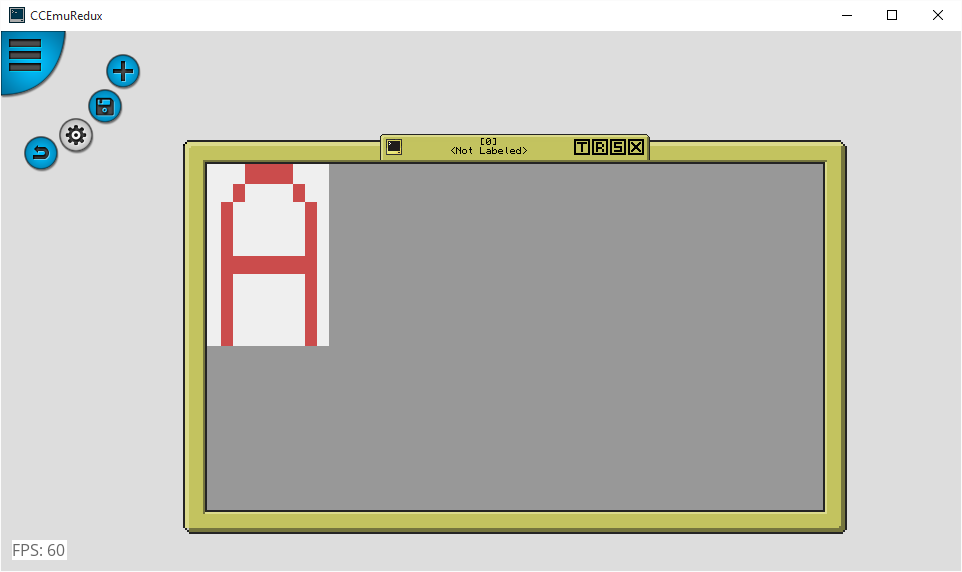
Screenshots of CheckBox:
Spoiler
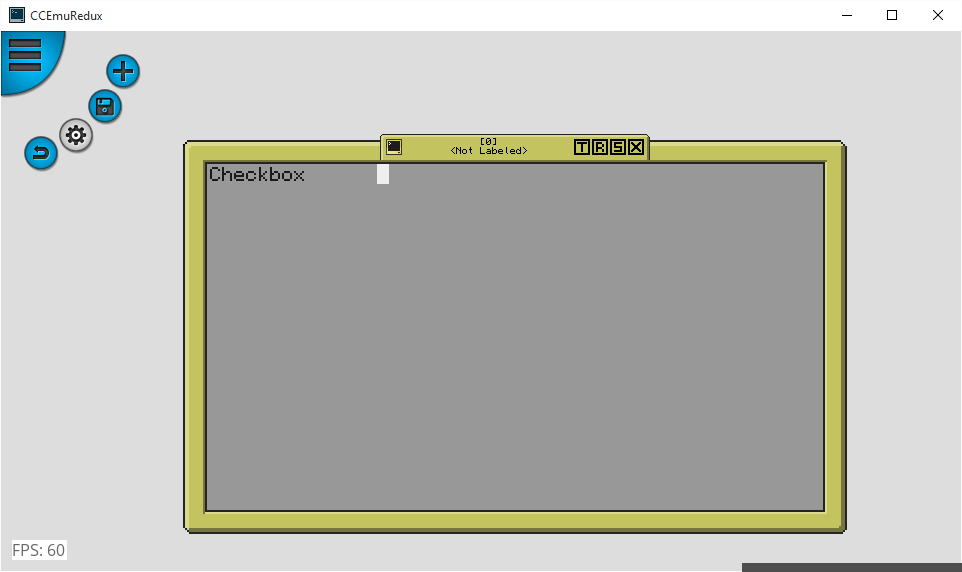
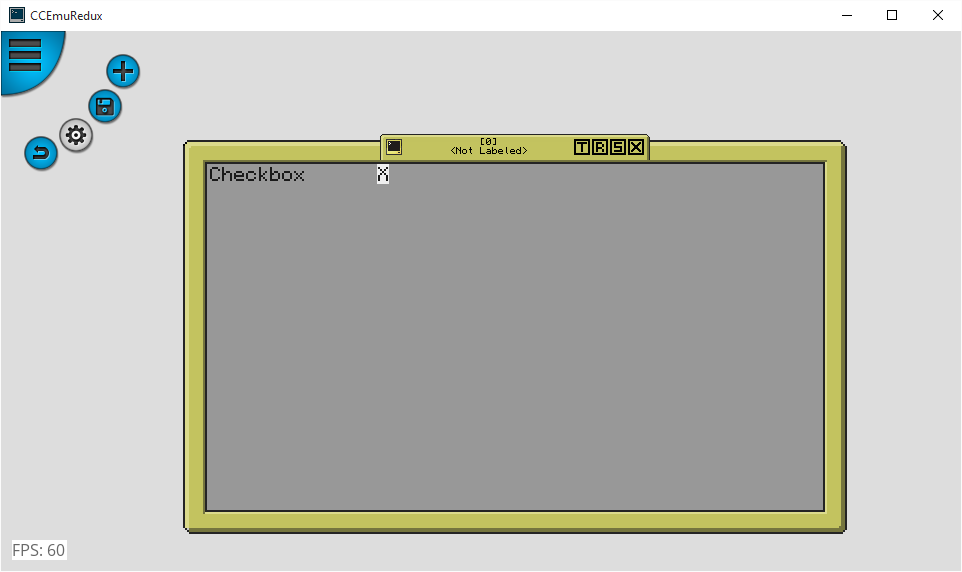
Edited on 16 November 2015 - 07:19 PM
2679 posts
Location
You will never find me, muhahahahahaha
Posted 16 November 2015 - 08:30 PM
Sweet! Like real nice. Keep up the good effort.
797 posts
Posted 16 November 2015 - 08:33 PM
This is a nice go-between for things like touchpoint to things like Bedrock and Flare. Perfect for medium sized programs that don't want to use a huge 100KB API, and it adds in more than just buttons.
Like I said before, I like the Application -> Screen -> Component structure, as it's what I used in Sheets. Something I've added to Sheets is the ability to have multiple screens per application, and each screen can draw to multiple terminals, so you can have different layouts on different monitors, but also share a layout between a couple of monitors. Is that worth implementing here?
As for more components, things with scrollbars never hurt…
38 posts
Location
Somewhere near keyboard
Posted 16 November 2015 - 08:38 PM
Actually, implementing ScrollViews was next point in my roadmap after adding ImageView. As I've finished it, now they are main priority in development.
It's night where I live, so I think I'll implement ScrollViews tomorrow.
Edit: So, well, now I'm proudly presenting ScrollView component (the only problem is that it can only be scrolled vertically):
Spoiler
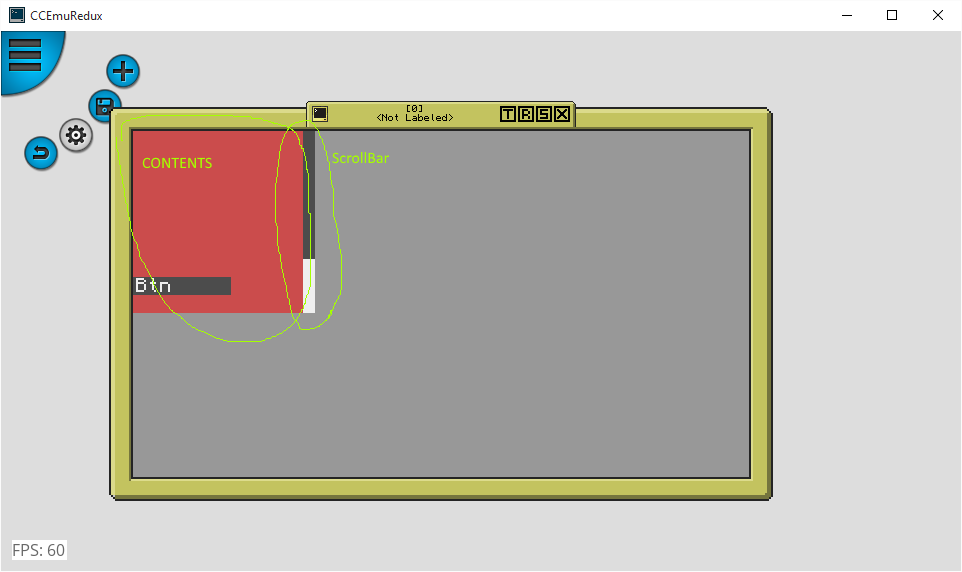
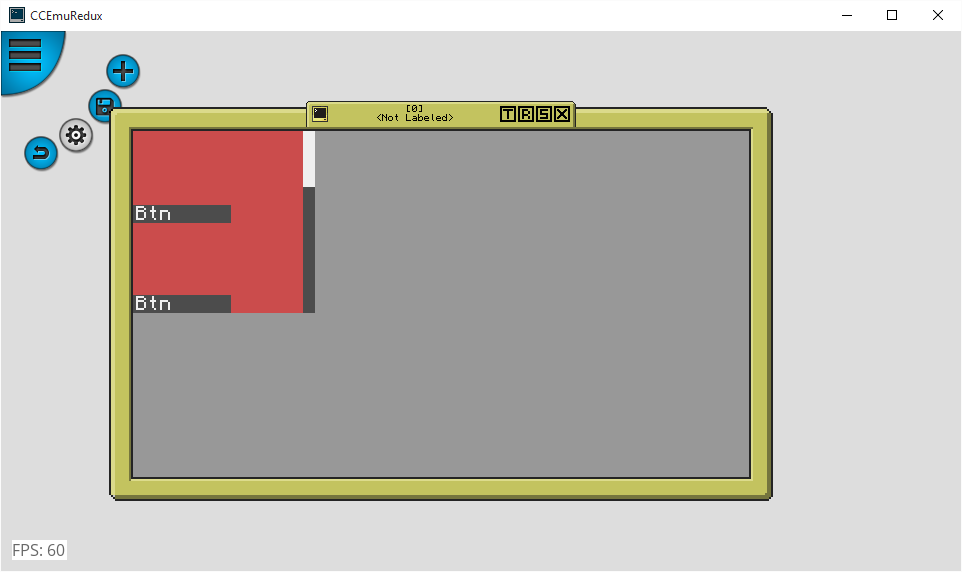
Edited on 17 November 2015 - 05:00 PM
38 posts
Location
Somewhere near keyboard
Posted 19 November 2015 - 06:06 PM
Window application bar (bottom) and ConfirmDialog:
Spoiler
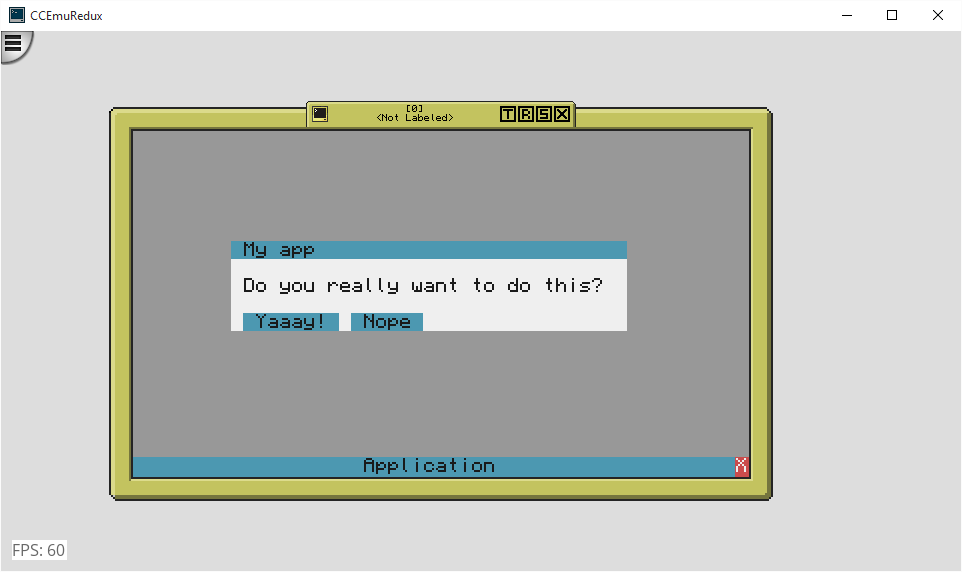