To make it easier to use these characters in an effective way I've created this simple to use API:
http://pastebin.com/TFFJwxvp
It contains two classes, the character class and the canvas class.
Character Class (Please note that this class isn't really ment to be used outside of the API):
textColor :: color :: The foreground color
backgroundColor :: color :: The background color
pixelMode :: bool :: true if the character is made out of seperate pixels
pixel :: bool[2][3] :: a two dimensionay array, each element represents a pixel. A character consist out of 2 by 3 pixels
character :: char :: the actual character
invert :: bool :: wether or not the text- and backgroundcolor are switched around (required for some pixel combinations)
create() :: returns a new character object
update() :: sets the "character" and "invert" member based on the values in the "pixel" member array
draw() :: writes the character (or pixels) with the right text and background color
Canvas Class:
x :: number :: x position of the canvas on the terminal
y :: number :: y position of the canvas on the terminal
character :: Character[<canvasWidth>][<canvasHeight>] :: an two-dimensional array containing all the characters
create() :: returns a new canvas object.
setSize() :: set the size of the canvas. Clears all the characters.
setPixel(number x, number y, bool value) :: set a pixel within the canvas. Please note that these positions are in pixels and not in character row/columns. For example, to set the right most pixel the X would have to be twice the canvas width. The lowest pixel would be three times the canvas height (each pixel consist out of 2x3 pixels).
setCharacter(number x, number y, char char) :: set a character on the canvas.
draw() :: draws the canvas to the screen.
Example:
local canvas = Canvas.create()
for i=1,20 do
canvas:setPixel(i, i, true)
canvas:setPixel(28 - i, i + 4, true)
canvas:setPixel(26 - i, i + 2, true)
canvas:setPixel(8, i + 8, true)
canvas:setPixel(i + 8, 28, true)
end
canvas:draw()
term.setCursorPos(1, 18)
which would draw: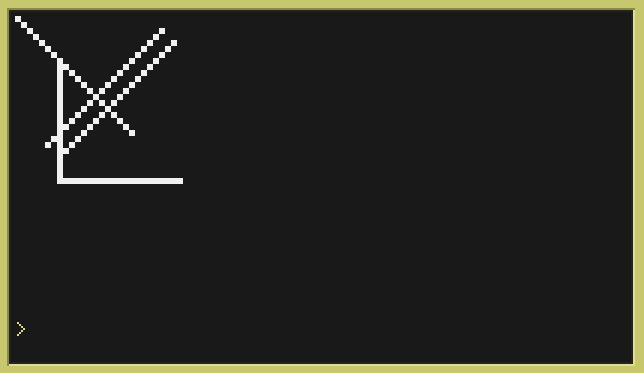
And that's it, hope you like it :-)
-Oli414