Posted 09 January 2016 - 09:51 AM
What it does:
It modifies the default Turtle's API's functions to add a tracking functionnality.
It adds four new variables that you may use in any program:
To correct that, you could ask the user where the turtle is currently located and modify them upon startup.
These variables are updated in real time when you use turtle.forward, turtle.back, turtle.up, turtle.down, turtle.turnRight or turtle.turnLeft.
If you are a cool person, you could save the content of the variables upon shutdown with bwhodle's great .ini config files API on this very forum
Details for advanced users
A fifth variable was also added, it is a function called
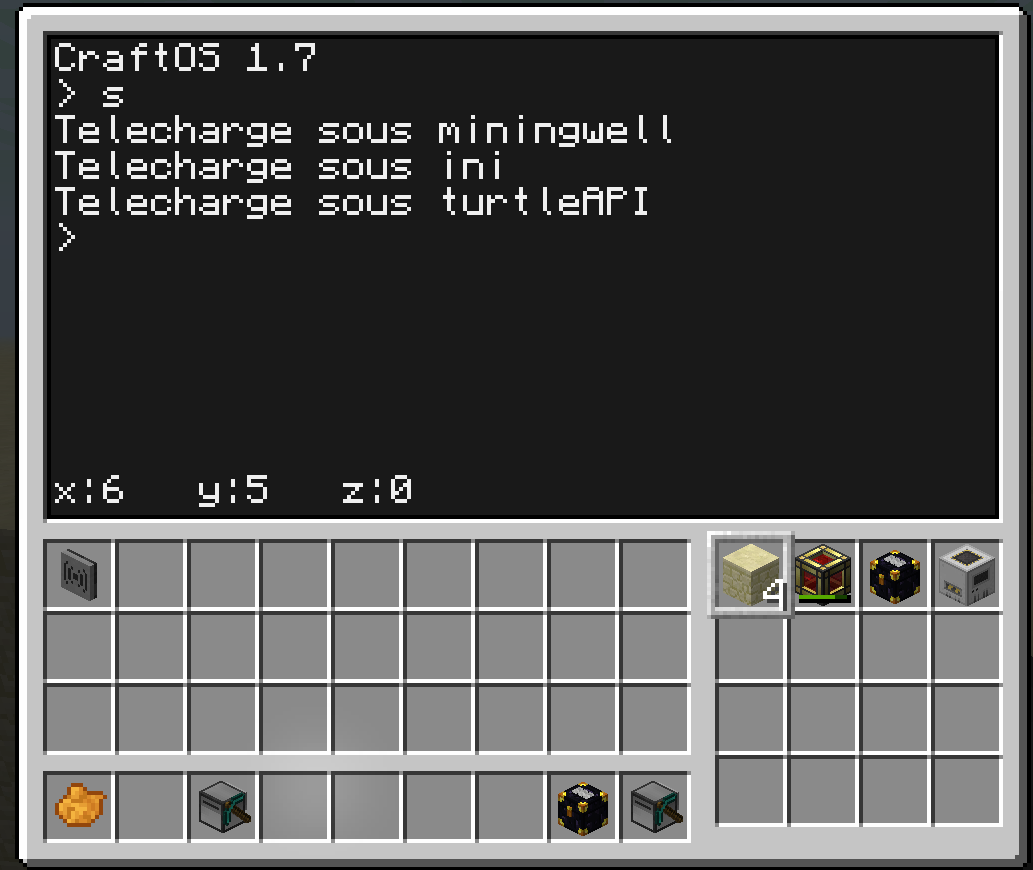
How do I use it ?
The API must be launched before the turtle turns or moves, for accuracy.
It can be launched at startup automatically by using
That's it.
Installing
I made two versions, one with regular coordinates (north is x+1, east is y+1, up is z+1) for sane people
pastebin get GXABjDpr turtleAPI
And one with real minecraft coordinates (north is z-1, east is x+1, up is y+1) so that the turtle's mental position and its real coordinates will match up, for those who might need it
pastebin get Su3BWPb8 turtleAPI
It modifies the default Turtle's API's functions to add a tracking functionnality.
It adds four new variables that you may use in any program:
- turtle.x
- turtle.y
- turtle.z
- turtle.facing
- turtle.goto(x,y,z)
- y being the height if you choose to install the version with the good ole' weird minecraft coordinates
- It's not optimized. See this post
- turtle.turnTo(direction)
- It's optimized ! It will never turn thrice.
To correct that, you could ask the user where the turtle is currently located and modify them upon startup.
These variables are updated in real time when you use turtle.forward, turtle.back, turtle.up, turtle.down, turtle.turnRight or turtle.turnLeft.
If you are a cool person, you could save the content of the variables upon shutdown with bwhodle's great .ini config files API on this very forum
Details for advanced users
A fifth variable was also added, it is a function called
- turtle.onEachMove
local function myFunc()
--do stuff
end
turtle.onEachMove=myFunc
You can use it for various things, for instance, displaying the coordinates of the turtle in real time: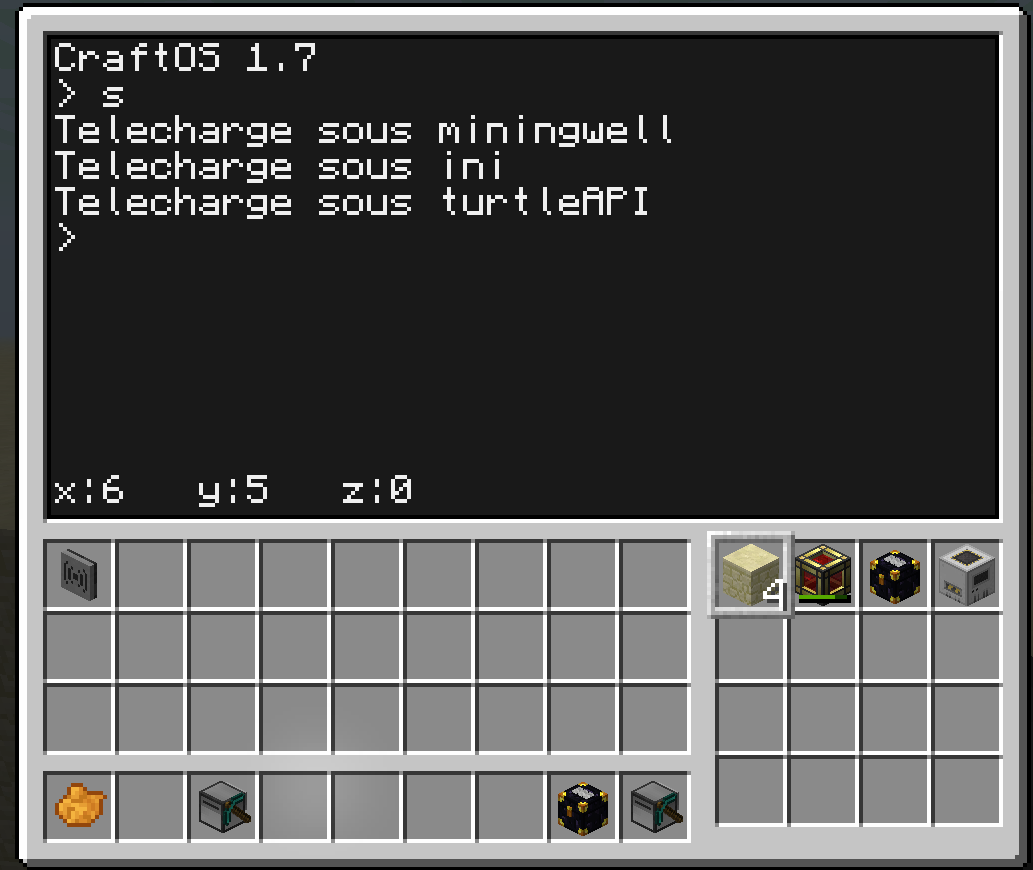
How do I use it ?
The API must be launched before the turtle turns or moves, for accuracy.
It can be launched at startup automatically by using
shell.run("turtleAPI")
That's it.
Installing
I made two versions, one with regular coordinates (north is x+1, east is y+1, up is z+1) for sane people
pastebin get GXABjDpr turtleAPI
And one with real minecraft coordinates (north is z-1, east is x+1, up is y+1) so that the turtle's mental position and its real coordinates will match up, for those who might need it
pastebin get Su3BWPb8 turtleAPI
Edited on 11 January 2016 - 05:15 PM