Posted 19 March 2016 - 12:36 AM
So I was recently looking at bytecode in lua and reading A No-Frills Introduction to Lua 5.1 VM Instructions.I was thinking of what I could make with it when I found out chunkspy does not work with LuaJ's bytecode because it uses big endian numbers rather than little endian. This is my WIP api for reading the bytecode.
GitHub page
Installation
I used Github Repo downloader by max96at, mainly because it was the first I found. If you want to use the same do
pastebin run wPtGKMam Gorzoid chunkLib
Documentation
to get an chunk object call the class table. It takes one argument, the string dump of a function.
It may looking something like this:
header
I won't bother telling you what each field is for, just refer to the Nofrills guide above.
func
name –This is the functions name, or whatever was put in there
line_defined –Self explanatory
last_defined –again…
num_upvalues –The number of upvalues from upper functions
num_params –Number of parameters
is_vararg – idfk what this is really, i think its for functions with … check nofrills
stack_size – Number of registers
Instructions – Finally something interesting List of these things:
value –The numerical 4byte value
opcode –The opcodes number, to get the name use Instruction.getInstruction(opcode)[1]
format –You don't really need this but it just says if the instruction is using one of 3 formats
args –This is a table of the arguments, depending on the format there may be a,b,c or just a,b. Note that some instructions use the last bit to tell if it is a register or a constant.
This class also has a _tostring function to turn it into a basic printable string. just do print(instruction)constants – List of constants, the constant is a table with type(0-4) and value.
functions –TIme to get inception style, this contains all nested functions
Now that's all the regular data in function… BUT WAIT! There's more!
If you happen to have debug data in your dump, you got some nice little tables to play with.
sourcelinepos –A list of numbers, the index = instruction number and value = source line number
locals –In bytecode, locals loose their names and become registers, but this tells you the orginal name of each register
upvals –List of names of all the values the function took from uppper functions.
I will now document some of the other classes, which are use by alot of my classes:
byteStruct
byteBuff
The function is byteBuff:readBytes(n) btw.
ScreenShot of the dump of textutils.serialize:
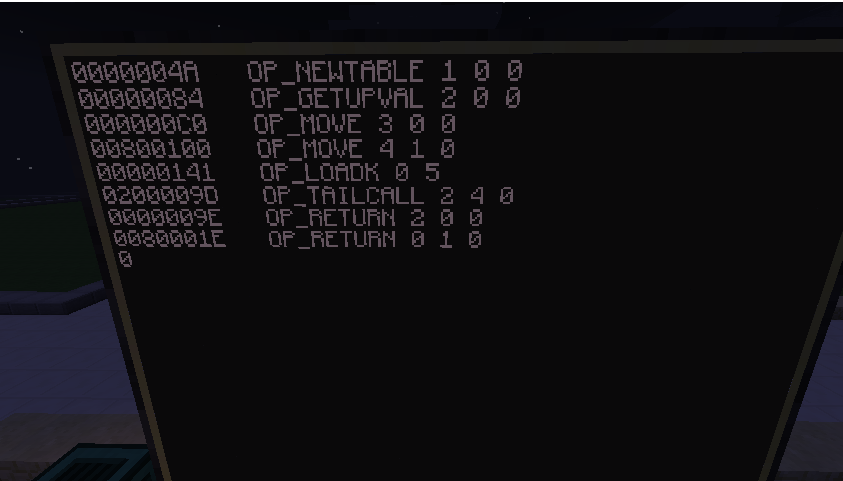
Suggestions, opinions and criticism(but keep it mild and constructive) are greatly welcomed. Hope this helps you on your pr0 lua h4x endeavors.
Btw mods, I did not put this in releases because I do not consider it a finished product.
I plan to "release" this once I have atleast polished up the code.
I also planned on making this reversible, so you could edit the tables then recompile it into bytecode and loadstring() it.
GitHub page
Installation
I used Github Repo downloader by max96at, mainly because it was the first I found. If you want to use the same do
pastebin run wPtGKMam Gorzoid chunkLib
Documentation
Spoiler
We start of with the lua chunk class Chunkto get an chunk object call the class table. It takes one argument, the string dump of a function.
It may looking something like this:
local chunk = Chunk(string.dump(function()
print("Hi")
end)
)
The Chunk class contains 2 fields:header
Spoiler
The header contains data about the bytecodes format, so you don't really need to read this that much, it is more just to get sizes and the endians of the code and also to verify that we can even read this code at all.I won't bother telling you what each field is for, just refer to the Nofrills guide above.
- signature
- version
- format
- endian
- sizeof_int
- sizeof_size_t
- sizeof_instruction
- sizeof_number
- integral
local info = {
endian = endian
sizeof = {
int = sizeof_int
size_t = sizeof_size_t
}
}
func
Spoiler
This contains all the stuff you want. It has your codes, your constants, and if your lucky, there may be some debug data in there for you. The fields are:name –This is the functions name, or whatever was put in there
line_defined –Self explanatory
last_defined –again…
num_upvalues –The number of upvalues from upper functions
num_params –Number of parameters
is_vararg – idfk what this is really, i think its for functions with … check nofrills
stack_size – Number of registers
Instructions – Finally something interesting List of these things:
Spoiler
The instruction is the actual bytecode. It has 3 fieldsvalue –The numerical 4byte value
opcode –The opcodes number, to get the name use Instruction.getInstruction(opcode)[1]
format –You don't really need this but it just says if the instruction is using one of 3 formats
args –This is a table of the arguments, depending on the format there may be a,b,c or just a,b. Note that some instructions use the last bit to tell if it is a register or a constant.
This class also has a _tostring function to turn it into a basic printable string. just do print(instruction)
functions –TIme to get inception style, this contains all nested functions
Now that's all the regular data in function… BUT WAIT! There's more!
If you happen to have debug data in your dump, you got some nice little tables to play with.
sourcelinepos –A list of numbers, the index = instruction number and value = source line number
locals –In bytecode, locals loose their names and become registers, but this tells you the orginal name of each register
upvals –List of names of all the values the function took from uppper functions.
I will now document some of the other classes, which are use by alot of my classes:
byteStruct
Spoiler
This is a class that most of my objects use as a superclass, it contains alot of functions for reading bytes and patterns, but you probably won't need this.byteBuff
Spoiler
This was a very helpful class which meant I did not have to keep track of how many bytes I had read already, once it reads n bytes it raise it's index by n. this meant i could give my byteBuff to every object and when that object got what it needed, i can just read the next bytes.The function is byteBuff:readBytes(n) btw.
ScreenShot of the dump of textutils.serialize:
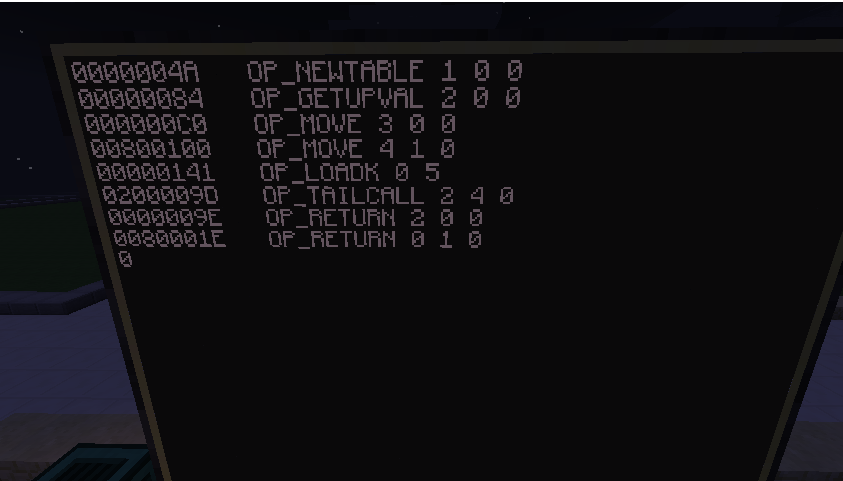
Suggestions, opinions and criticism(but keep it mild and constructive) are greatly welcomed. Hope this helps you on your pr0 lua h4x endeavors.
Btw mods, I did not put this in releases because I do not consider it a finished product.
I plan to "release" this once I have atleast polished up the code.
I also planned on making this reversible, so you could edit the tables then recompile it into bytecode and loadstring() it.