Posted 22 May 2016 - 04:32 AM
pastebin get hR8p9ML4 partTerm
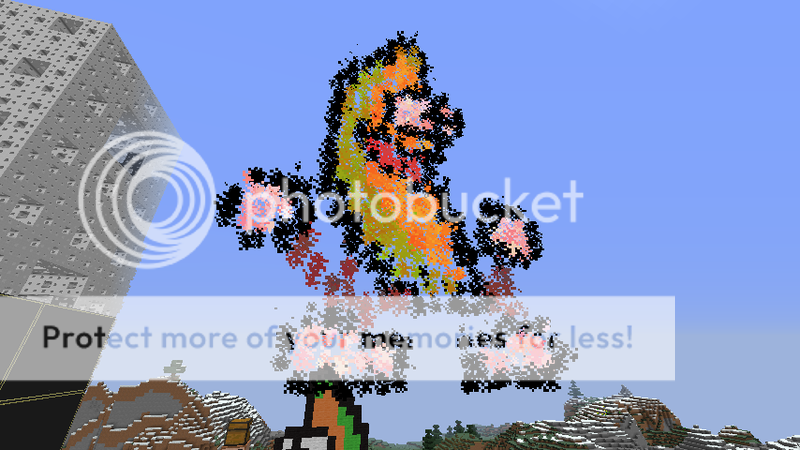
So I discovered /particle. Time for another terminal object, then.
Returns a terminal object you can redirect to, then draw to with eg the paintutils API.
The object will be centered on the co-ords specified by xPos/yPos/zPos unless the final parameter is specifically false (in which case the co-ords pinpoint the top-left of the terminal instead, and rotations act around that position). Its size is based on its width/height multiplied by the "spacing" value, which defaults to 0.2 - higher values move the particles in the object further apart. For example, a 5x5 object with the default spacing covers one square block.
Rotation settings are in degrees. In terms of horizontal rotation, 0 degrees faces north, 90 faces east, 180 south, and 270 west. In terms of vertical, 0 is upright, 90 aims the object down, 180 flips it upside down, and 270 aims it upwards.
Particle types may be any listed here, but only "reddust", "mobSpell" and "mobSpellAmbient" may be coloured. The default is "reddust".
The terminal objects have the following functions (in addition to the regular ones):
Allows or disallows rendering of the terminal object.
termObject.setSpacing(number spacing)
Makes the terminal object larger or smaller by increasing the distance between particles. Default is 0.2.
termObject.setParticle(string particleType)
Particle types may be any listed here, but only "reddust", "mobSpell" and "mobSpellAmbient" may be coloured. The default is "reddust".
termObject.reposition([number x] [, number y] [, number z] [, number width], [, number height])
Moves the terminal object around the world and / or re-defines its bounds.
termObject.getPosition() => number x, number y, number z
Returns the world-location of the center of the terminal object.
termObject.setAngles([number horizontalRotation] [, number verticalRotation])
Alters the orientation of the terminal object. Angles are in degrees.
termObject.getAngles() => number horizontalRotation, number verticalRotation
Returns the current orientation of the terminal object, in degrees.
termObject.center(boolean)
If set to true (as is the default), the terminal is positioned and rotated around its center point. If false, its top-left point is used instead.
partTerm.render()
Runs indefinitely, and executes the particle commands that draw any terminal objects you've created. If you want to alter their content while rendering, then use eg the parallel API to run the rendering function alongside the rest of your code.
A limitation of this function is that if it is halted, it may not function correctly when restarted until either partTerm.setDensity() is called or the API is reloaded.
partTerm.remove(table termObject)
De-registers a given terminal object from the renderer, preventing it from being drawn within the world anymore. Do not call this while rendering - use termObject.setVisible(false) if you really need to hide a terminal while drawing is in progress.
partTerm.removeAll()
De-registers all terminal objects from being rendered. Make the same thing can be done by re-loading the whole API. Do not call this while rendering.
partTerm.setDensity(number particles)
The higher this value is set, the faster the renderer will attempt to draw particles. Minimum is one, maximum is 250, default is 50.
If set too low, your terminals may flicker. However, setting it higher will put more stress on your server. Do not call this while rendering.
partTerm.getHorizontalAngle(number x1, number z1, number x2, number z2) => number angle
Given two points in the world, returns the angle between them on the horizontal plane. For example, you might use this to aim a terminal displaying an arrow at another point in the world:
partTerm.getVerticalAngle(number x1, number y1, number z1, number x2, number y2, number z2) => number angle
Similar to partTerm.getHorizontalAngle(), though it measures the angle between two points on the vertical plane.
Floats a Bomberman over your Command Computer, which turns to watch you.
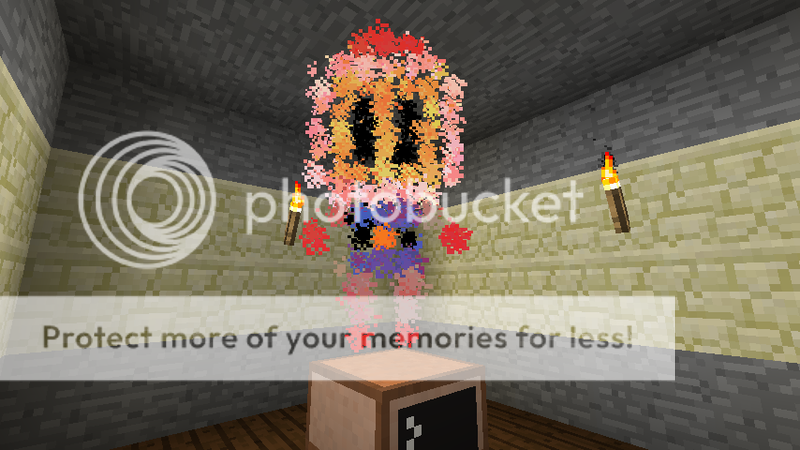
1.0.0
Initial release.
2015/05/30
1.0.1
Fixed bug where terminal objects without a transparent colour set wouldn't render.
Added partTerm.getHorizontalAngle() / partTerm.getVerticalAngle().
New option to have terminal co-ords act relative to their top-left point instead of their center.
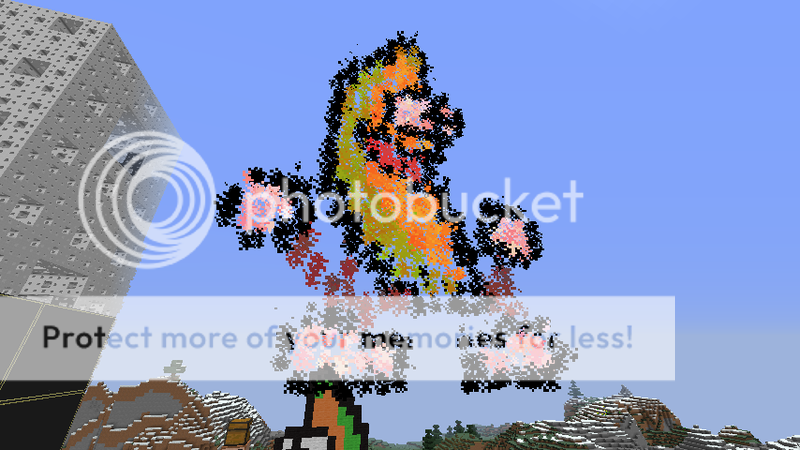
So I discovered /particle. Time for another terminal object, then.
Usage
partTerm.createWindow(number xPos, number yPos, number zPos, number width, number height [, number transparentColour] [, number horizontalRotation] [, number verticalRotation] [, boolean visible] [, number spacing] [, string particleType] [, boolean centered]) => table terminalObjectReturns a terminal object you can redirect to, then draw to with eg the paintutils API.
The object will be centered on the co-ords specified by xPos/yPos/zPos unless the final parameter is specifically false (in which case the co-ords pinpoint the top-left of the terminal instead, and rotations act around that position). Its size is based on its width/height multiplied by the "spacing" value, which defaults to 0.2 - higher values move the particles in the object further apart. For example, a 5x5 object with the default spacing covers one square block.
Rotation settings are in degrees. In terms of horizontal rotation, 0 degrees faces north, 90 faces east, 180 south, and 270 west. In terms of vertical, 0 is upright, 90 aims the object down, 180 flips it upside down, and 270 aims it upwards.
Particle types may be any listed here, but only "reddust", "mobSpell" and "mobSpellAmbient" may be coloured. The default is "reddust".
The terminal objects have the following functions (in addition to the regular ones):
Terminal Object Functions
termObject.setVisible(boolean visible)Allows or disallows rendering of the terminal object.
termObject.setSpacing(number spacing)
Makes the terminal object larger or smaller by increasing the distance between particles. Default is 0.2.
termObject.setParticle(string particleType)
Particle types may be any listed here, but only "reddust", "mobSpell" and "mobSpellAmbient" may be coloured. The default is "reddust".
termObject.reposition([number x] [, number y] [, number z] [, number width], [, number height])
Moves the terminal object around the world and / or re-defines its bounds.
termObject.getPosition() => number x, number y, number z
Returns the world-location of the center of the terminal object.
termObject.setAngles([number horizontalRotation] [, number verticalRotation])
Alters the orientation of the terminal object. Angles are in degrees.
termObject.getAngles() => number horizontalRotation, number verticalRotation
Returns the current orientation of the terminal object, in degrees.
termObject.center(boolean)
If set to true (as is the default), the terminal is positioned and rotated around its center point. If false, its top-left point is used instead.
partTerm.render()
Runs indefinitely, and executes the particle commands that draw any terminal objects you've created. If you want to alter their content while rendering, then use eg the parallel API to run the rendering function alongside the rest of your code.
A limitation of this function is that if it is halted, it may not function correctly when restarted until either partTerm.setDensity() is called or the API is reloaded.
partTerm.remove(table termObject)
De-registers a given terminal object from the renderer, preventing it from being drawn within the world anymore. Do not call this while rendering - use termObject.setVisible(false) if you really need to hide a terminal while drawing is in progress.
partTerm.removeAll()
De-registers all terminal objects from being rendered. Make the same thing can be done by re-loading the whole API. Do not call this while rendering.
partTerm.setDensity(number particles)
The higher this value is set, the faster the renderer will attempt to draw particles. Minimum is one, maximum is 250, default is 50.
If set too low, your terminals may flicker. However, setting it higher will put more stress on your server. Do not call this while rendering.
partTerm.getHorizontalAngle(number x1, number z1, number x2, number z2) => number angle
Given two points in the world, returns the angle between them on the horizontal plane. For example, you might use this to aim a terminal displaying an arrow at another point in the world:
win.setAngles( partTerm.getHorizontalAngle( winXpos, winZpos, tarXpos, tarZpos) )
partTerm.getVerticalAngle(number x1, number y1, number z1, number x2, number y2, number z2) => number angle
Similar to partTerm.getHorizontalAngle(), though it measures the angle between two points on the vertical plane.
Example - Floating Bomberman
pastebin get 8Cp67u6B bomberGhostFloats a Bomberman over your Command Computer, which turns to watch you.
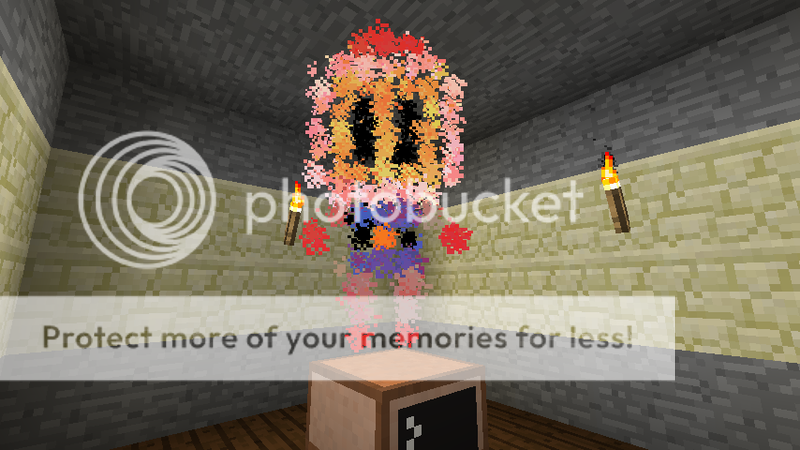
Version History
2015/05/221.0.0
Initial release.
2015/05/30
1.0.1
Fixed bug where terminal objects without a transparent colour set wouldn't render.
Added partTerm.getHorizontalAngle() / partTerm.getVerticalAngle().
New option to have terminal co-ords act relative to their top-left point instead of their center.
Edited on 30 May 2016 - 12:09 PM