Typical BASIC Functions and Keywords
So to see all C64 functions, see this website: link.
- FRE
- JOY
- USR
- VAL
- PEEK
- PEN
- POS
- POT
New Implemented Functions
- BTN
- CLEAR
- COLOR
- CURSOR
- WRITE
- STOREN
- HEX
- HIRES
- LORES
- PIXEL
- SPRITE
- REBOOT
- SHUTDOWN
- LABEL
- FOPEN
- FREAD
- FREADLN
- FWRITE
- FWRITELN
- FCLOSE
- FEXISTS
- FLIST
- FRDONLY
- FDEL
- FISDIR
- FLOAD
- FCONEXT
- FCOPREV
- EXIT
- DISPOSE
- CREATETIMER
- SETTIMER
- GETTIMER
- NETCREATE
- NETOPEN
- NETMYIP
- NETRECEIVE
- NETSEND
- NETCLEARBUFFER
- NETTYPE
- NETMESSAGE
- NETGETTYPE
- NETGETMESSAGE
- NETGETID
- NETGETIP
- NETCLOSE
- LET –assigns a value (which may be the result of an expression) to a variable.
- DATA –holds a list of values which are assigned sequentially using the WRITE command.
- IF … THEN –used to perform comparisons or make decisions.
- FOR .. TO … {STEP} … NEXT –repeat a section of code a given number of times. A variable that acts as a counter is available within the loop.
- GOTO –jumps to a numbered or labelled line in the program.
- GOSUB –jumps to a numbered or labelled line, executes the code it finds there until it reaches a RETURN keyword.
- PRINT –displays a message on the screen.
- INPUT –asks the user to enter the value.
- STORE –store a value to a defined data array.
- READ –read from the defined data array.
- RETURN –return from a subroutine.
- DEF –makes a lambda function
Stock BASIC function descriptions
GOTO( number/label position )- jumps to a numbered or labelled line in the program
- jumps to a numbered or labelled line, executes the code it finds there until it reaches a RETURN keyword
- displays a message on the screen
- asks the user to enter the value
- write to a defined data array
- store a number to a growing array
- read from the defined data array
- returns a value at that index in that array
- return from a subroutine
DEF COMMAND
DEF name( vararg ) = expression- makes a lambda function
- Example:
print func( 2 )
def func( x ) = x * x
Rem OUTPUTS 4
let y = 0
set 18
print y
def set( x ) = y = x
Rem OUTPUTS 18
Implemented variable descriptions
cycles- returns executed cycles per CCFrame
- holds the current typed keyboard input!
Implemented function descriptions
BTN( number value )- returns (1) if a button is pressed or (0) if not pressed.
- 1 - arrow left
- 2 - arrow right
- 3 - arrow up
- 4 - arrow down
- 5 - Z
- 6 - X
- clears the screen.
- sets the current colors
- sets built-in terminal cursor position.
- prints a message on the screen, without the new line.
- converts hexadecimal to decimal value
- Switches from Low Resolution Mode ( char column graphics ) to High Resolution Mode ( char pixel graphics ).
- Switches from High Resolution Mode ( char pixel graphics ) to Low Resolution Mode ( char column graphics ).
- Puts a character on the screen in Low Resolution Mode ( char is optional ) or
- Puts a pixel on the screen ( High Resolution Mode )
- Displays the sprite on the screen at the given location
- Reboots the system
- Shutdown the system
- Sets the computers name( label )
- Opens a file return (1) if succeeded or (0) if failed
- Reads all of current opened file
- Reads a line of current opened file returns (0) if end of file
- Writes data to current opened file
- Writes a line of data to the current file
- Closes current opened file
- Checks if the file is present on the computer, returns (1) if it is otherwise returns (0)
- Returns an array list of files and folders present in that path
- Deletes the file specified from the computer
- Returns (1) if the path is a directory or (0) if not
- Stops the program and exits to SHELL or BASIC Terminal
- Simple garbage collection
- Load a file to the program memory!
- Compatible extensions: *.o | *.bas
- Returns the ID of the timer
- Sets the time to the current timer
- Gets current timers time
- Increments the file stack by one, next time you read or write it will write to a different file buffer!
- Decrements the file stack by one
- Creates a network object
- Initializes and opens the network to work on
- Closes and frees the network
- Returns your IP adress, use it only when you open the network
- Receives any messages sent and stores to a buffer
- Returns senders ID
- Returns senders IP
- Returns senders Message Type
- Returns senders Message
- Clears the send buffer
- Stores the type of the message to send buffer
- Stores the message to the send buffer
- Sends a message to that IP adress( computer )
BASIC programs
Hello World
10 print "Hello, world"
20 goto 10
10 print "Hello, world" : goto 10
For Loop
10 for x = 1 to 10
20 print "I farted", x, "times!"
30 next
10 for x = 0 to 10 step 5
20 print "I farted", x, "times!"
30 next
Input Program
10 let name = input( "What is your name?" )
20 print "Nice to meet you,", name
Rectangle Program
10 for y = 1 to 9
20 for x = 1 to 21
30 pixel x, y, " "
40 next
50 next
60 goto 10
Sprite Program
05 rem VARIABLE DECLARATIONS
10 let back = hex( "f" )
20 let front = 0
30 let canClear = 1
35 hires
39 rem INIT SPRITE
40 gosub 100
49 rem DRAW SPRITE
50 if canClear = 0 then goto 80
60 color back, front
70 clear
75 canClear = 0
80 sprite spr, 5, 5, 8, 8
90 goto 50
99 rem SPRITE DATA
100 data spr
110 storen spr, back
111 storen spr, back
112 storen spr, front
113 storen spr, front
114 storen spr, front
115 storen spr, front
116 storen spr, back
117 storen spr, back
118 storen spr, back
119 storen spr, front
120 storen spr, front
121 storen spr, front
122 storen spr, front
123 storen spr, front
124 storen spr, front
125 storen spr, back
126 storen spr, front
127 storen spr, front
128 storen spr, back
129 storen spr, front
130 storen spr, front
131 storen spr, front
132 storen spr, front
133 storen spr, front
134 storen spr, front
135 storen spr, front
136 storen spr, front
137 storen spr, front
138 storen spr, front
139 storen spr, front
140 storen spr, front
141 storen spr, front
142 storen spr, front
143 storen spr, front
144 storen spr, front
145 storen spr, front
146 storen spr, back
147 storen spr, back
148 storen spr, back
159 storen spr, back
150 storen spr, front
151 storen spr, front
152 storen spr, front
153 storen spr, front
154 storen spr, front
155 storen spr, front
156 storen spr, front
157 storen spr, front
158 storen spr, back
159 storen spr, front
160 storen spr, front
161 storen spr, front
162 storen spr, front
163 storen spr, front
164 storen spr, front
165 storen spr, back
166 storen spr, back
167 storen spr, back
168 storen spr, front
169 storen spr, front
170 storen spr, front
171 storen spr, front
172 storen spr, back
173 storen spr, back
180 return
Guess game
10 let y = rnd( 1, 10 )
20 let x
30 x = num( input( "Guess the number?" ) )
40 if x = y then goto 70
50 print "Wrong!"
60 goto 30
70 print "RIGHT!"
80 if input( "Want to play again?" ) = "y" then goto 10
90 print "BYE!"
Moving pixel
1 Rem VARIABLE DECLARATIONS
5 Let timer = createTimer()
10 Let x = 1
20 Let y = 1
21 Rem MAIN LOOP
30 Color 15, 0
40 Clear
41 Rem USERS INPUT
50 If Btn( 1 ) = 1 Then x = x - 1
60 If Btn( 2 ) = 1 Then x = x + 1
70 If Btn( 3 ) = 1 Then y = y - 1
80 If Btn( 4 ) = 1 Then y = y + 1
81 Rem DRAW THE PIXEL
90 Color 5, 0
100 Pixel x, y, " "
101 Rem SKIP SOME CYCLES
110 setTimer timer, cycles
120 If getTimer( timer ) <= 0 Then Goto 30
130 Goto 120
BASIC Loader
Rem Made By: LeDark Lua
Rem NOTE: all programs that will run from
Rem this program share same Enviroment!
__Init:
Rem INITIALIZE HELPER VARIABLES
Let __currSelected = 1
Let __tempFiles
Data __files
Let __fileLen
Let __TempFn
Let __Timer = createTimer()
Let timer = createTimer()
__FetchFiles:
Rem FETCH AND STORE FILES
__tempFiles = FList( "/" )
For i=1 To Len( __tempFiles )
__TempFn = Read( __tempFiles, i )
If __TempFn = "loader.bas" Then Goto __SkipLoaderFile
If Right( __TempFn, 3 ) = "bas" Then Storen __files, __TempFn
If Right( __TempFn, 1 ) = "o" Then Storen __files, __TempFn
__SkipLoaderFile:
Next
__fileLen = Len( __files )
__MainLoop:
Rem MAIN PROGRAM LOOP
Cursor 1, 1
Clear
If Btn( 3 ) = 1 Then __currSelected = __currSelected - 1
If Btn( 4 ) = 1 Then __currSelected = __currSelected + 1
If __currSelected > __fileLen Then __currSelected = 1
If __currSelected < 1 Then __currSelected = __fileLen
For i = 1 To __fileLen
Color 15, 0
if __currSelected = i then Color 15, 5
Print Read( __files, i )
Next
If Btn( 5 ) = 1 then GoSub __RunSelected
setTimer __Timer, 250 - cycles
__Sleep:
Rem SLEEPER
if getTimer( __Timer ) <= 0 then goto __MainLoop
goto __Sleep
__RunSelected:
Rem RUNS A SELECTED PROGRAM!
Color 15, 0
Cursor 1, 1
Clear
GoSub FLoad( Read( __files, __currSelected ) )
Dispose
Return
subSleep:
Rem HELPER SUBROUTINE FOR PROGRAMS
If getTimer( timer ) <= 0 Then Return
Goto subSleep
Rem Prints a hello world onto the screen, waits a bit and returns to the loader program!
Print "Hello, world!"
Rem Sleep a bit ( calls other programs subroutine )
timer = 1000
GoSub subSleep
Rem Return to the loader
Return
How to get and run BASIC
To compile BASIC file:
BASIC c <in> <out> --compiles the file and spits out bytecode file
To run the compiled BASIC file:
BASIC r <in> --runs the compiled file
To run just the BASIC file:
BASIC <filename> --runs a file
To run a BASIC terminal:
BASIC --runs a terminal
Terminal commands
new- creates a new file
- clears the screen
- fetch all files and directories
- to see then type list in the terminal
- loads a file
- saves a file
- list all contents of a file or list all loaded files and directories
- or if you want just a block of code to be displayed use:
- list 10 50
- displays all code from line 10 to line 50
- list compiled data of the contents typed in ( DEBUG only )
- runs typed contents
- exits BASIC terminal
Some pictures of BASIC
There are BASIC terminal pictures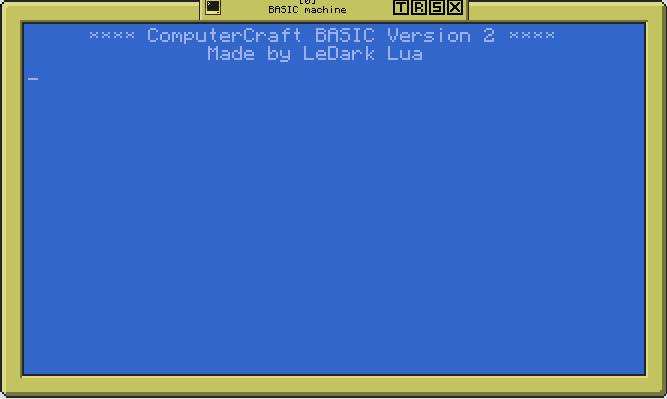
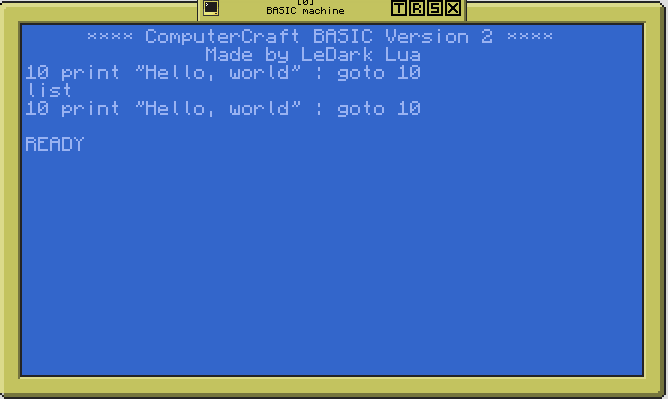
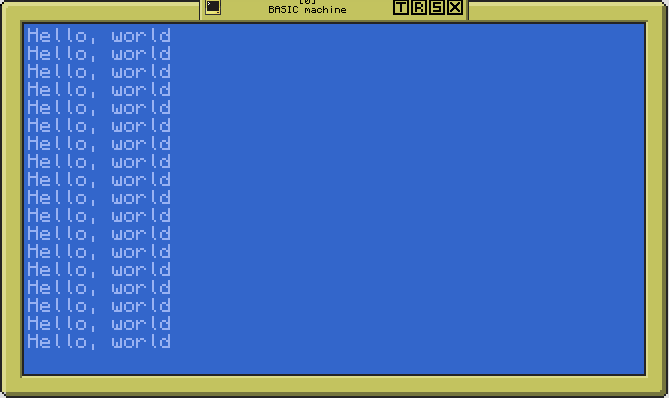
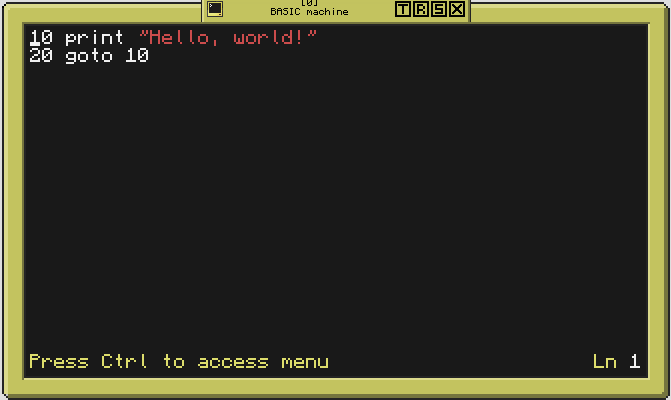
Thanks in advance,
LeDark Lua