Posted 22 July 2018 - 04:17 PM
Aloof
(Another Lua Object Oriented Framework)
2 years in the making (and that's just the name :)/>) and following several rewrites, this Java inspired framework provides a way to write OOP style code in Lua
Features:
- Written completely in Lua, variables and methods are still written in Lua
- Java inspired class structure with static and instance variables and methods, and instance contructors
- Variable and method inheritance by extending other classes
- The 'super' object that allows accessing the methods of the inherited class
- Interface implementation to ensure expected functions exist within classes
- Several included packages which at the moment consist of a type checking class, a basic logging package and a feature packed graphics / UI package called 'nodes'
- Getter and Setter methods to help streamline code
package = "package name", -- string or nil
imports = "full class paths of classes + interfaces to import", -- string_array or string or nil
class = "class_name", -- this is the short_class_name
extends = "name of class this class extends", -- full_class_name or short_class_name or nil
implements = "names of interfaces this class implements", -- array of interface_names or single interface name or nil
static_variables = "string_indexed_table_or_nil" -- static variables
static_getters = "string_to_function_table_or_nil" -- getters for static variables
static_setters = "string_to_function_table_or_nil" -- setters for static variables
static_methods = "string_to_function_table_or_nil" -- static methods
variables = {} -- string_indexed_table or nil - instance variables
getters = {} -- string_to_function_table or nil - getters for instance methods
setters = {} -- string_to_function_table or nil - setters for instance methods
methods = {} -- string_to_function_table or nil - instance methods
constructor = function(self, ...) end, -- instance constructor - has instance passed as first argument
The Interface structure (see Github for working examples):
package = "package name", -- string or nil
imports = "full class paths of classes + interfaces to import", -- string_array or string or nil
interface = "interface name", -- this is the short interface name
extends = "name of interface this interface extends", -- full_interface_name or short_interface_name or nil
static_methods = {}, -- string_array of static function names this interface should implement
methods = {}, -- string_array of instance function names this interface should implement
Github repo:
- Code
- Wiki ('EXTREMELY' WIP)
- Issues + Suggestions
pastebin run J8azvLQg aloof
Saves all files to the 'aloof' directoryDemo Program (Uses the 'nodes' package for the UI):
Code can be found here
Requires installing aloof first - see above
Install by running the following (saves the files to the 'AloofNodesDemo' directory):
pastebin run J8azvLQg aloofnodesdemo
Then run the demo by running:AloofNodesDemo/run.lua
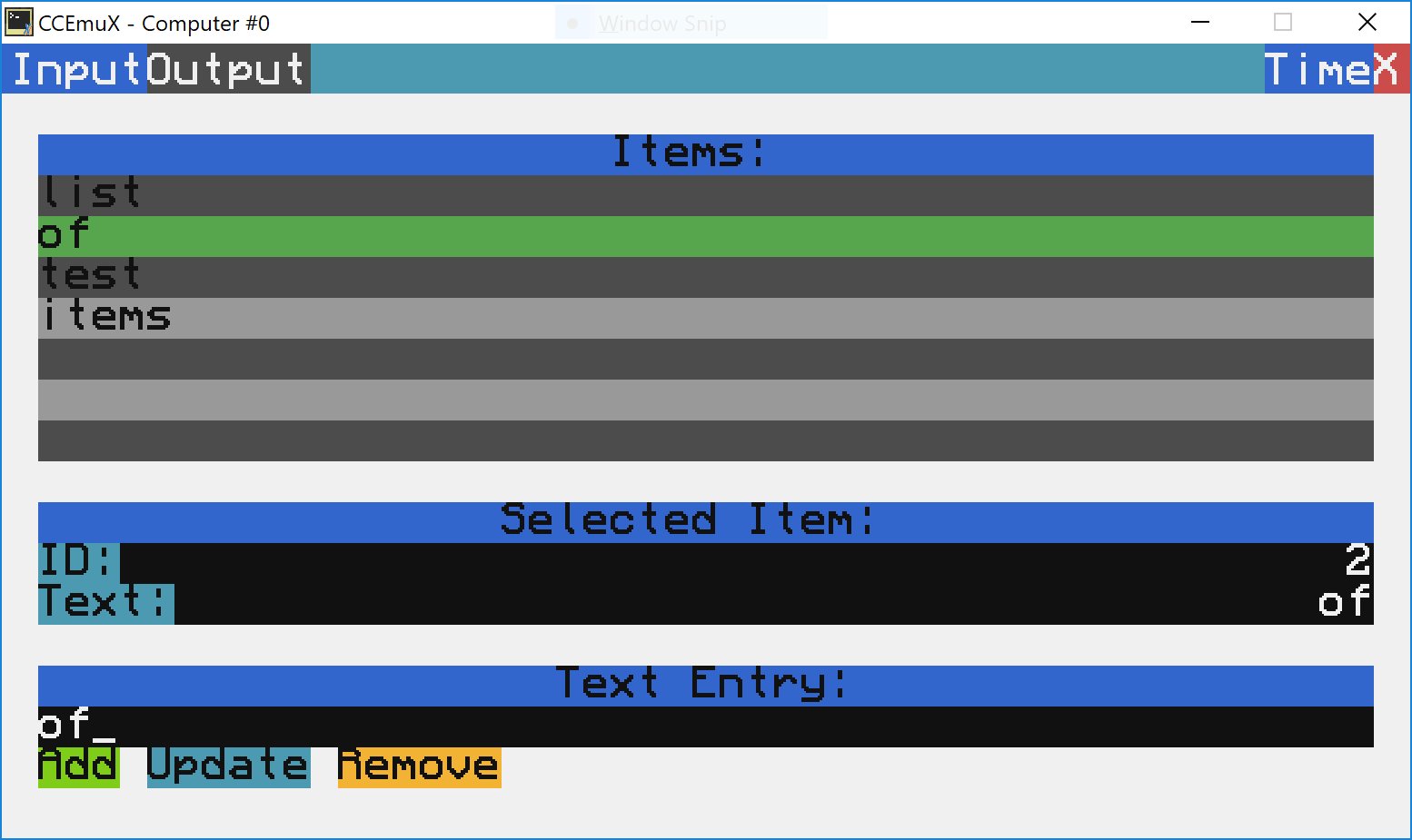
Planned features:
- Auto importing using 'imports' defined in classes - at the moment all classes that need loading are specified in the start arguments
Better class checking implementation to remove the need for the 'NIL' variable in class definitionsImproved class loading to throw errors in more informative places. Can now define nil variables using 'nil', though 'NIL' is still required when bulk defining variables- Remove requirement for Lua 5.1 - calling 'super' currently uses 5.1 environment stuff
- Add static constructors - possibly preInit, init, and postInit to allow setting up inter class dependencies better
Edited on 28 July 2018 - 02:09 PM