Posted 24 August 2012 - 08:51 AM
This is my variant on the tree farm turtle. As well as my first real Computer Craft program, Please provide feedback as I can not improve it with out other viewpoints.
What my program offers is:
The basis behind my method is that a sapling will not conduct a red-stone current but a tree will, This allows the turtle to detect when it passes by a tree instead of a sapling.
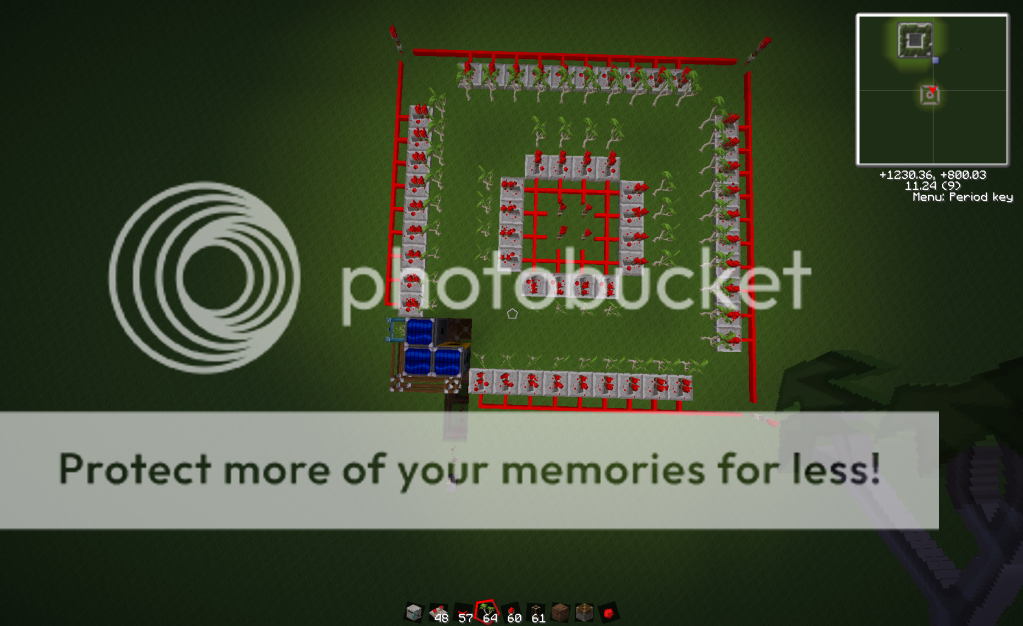
Shown above is the basic layout for what the farming area looks like It has more set up them other programs but should have less downtime. The farming area is scalable to fit your needs as well.
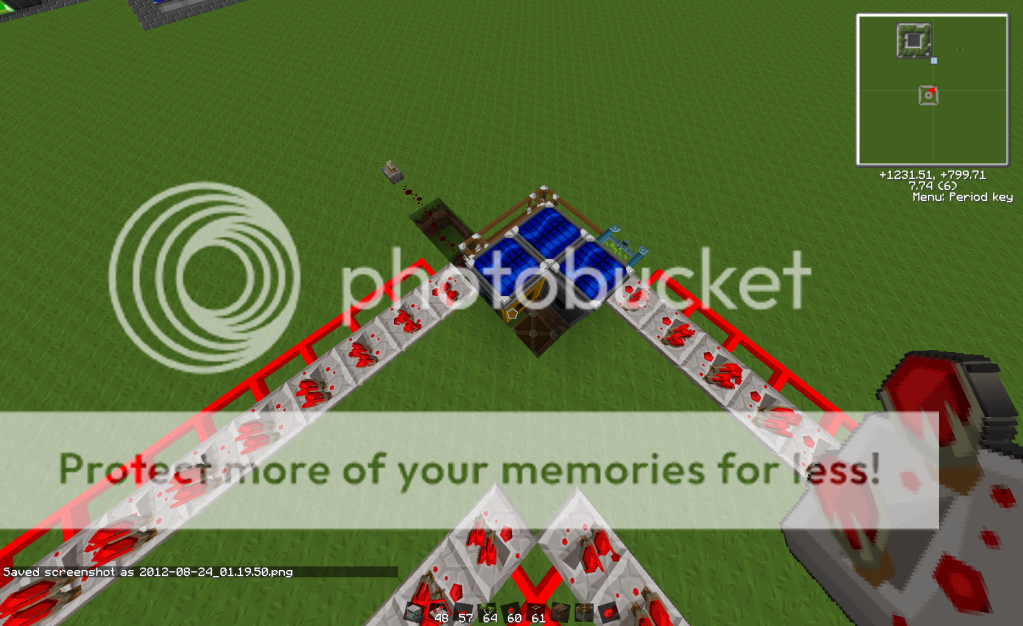
This image shows the setup for the auto collection and stock point.
Below is my paste bin like for easy transferring to computer craft as well as my code
http://pastebin.com/ak5RVpQc
What my program offers is:
- Continues Operation ( No need to start it each time you want it to search or trees )
- Automated Drop off of lumber as well as sapling restock
- An on turtle display showing real time stats
- The ability to enter the turtle into a standby mode
The basis behind my method is that a sapling will not conduct a red-stone current but a tree will, This allows the turtle to detect when it passes by a tree instead of a sapling.
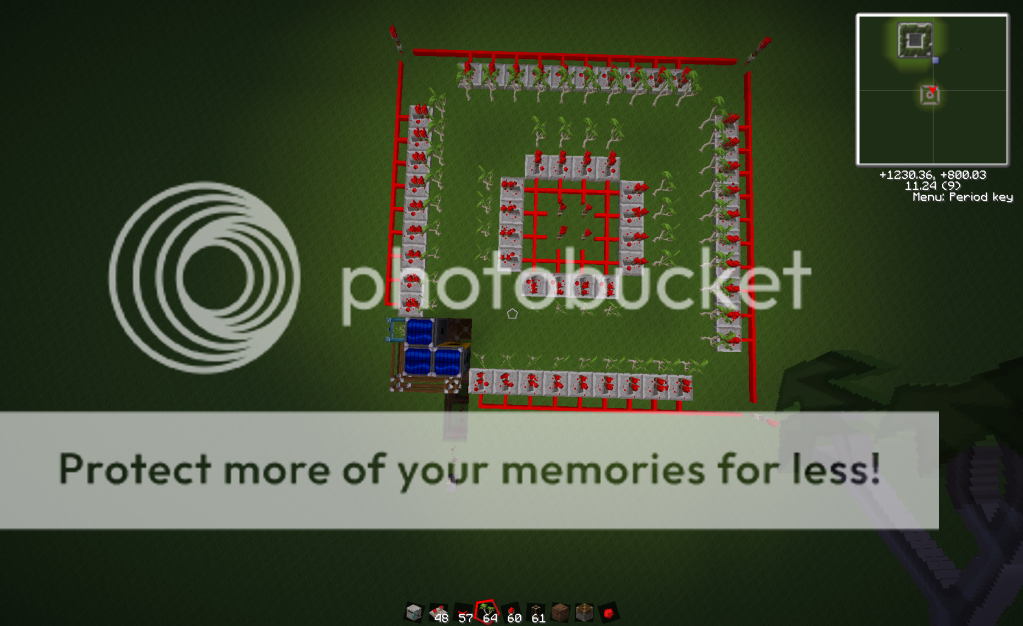
Shown above is the basic layout for what the farming area looks like It has more set up them other programs but should have less downtime. The farming area is scalable to fit your needs as well.
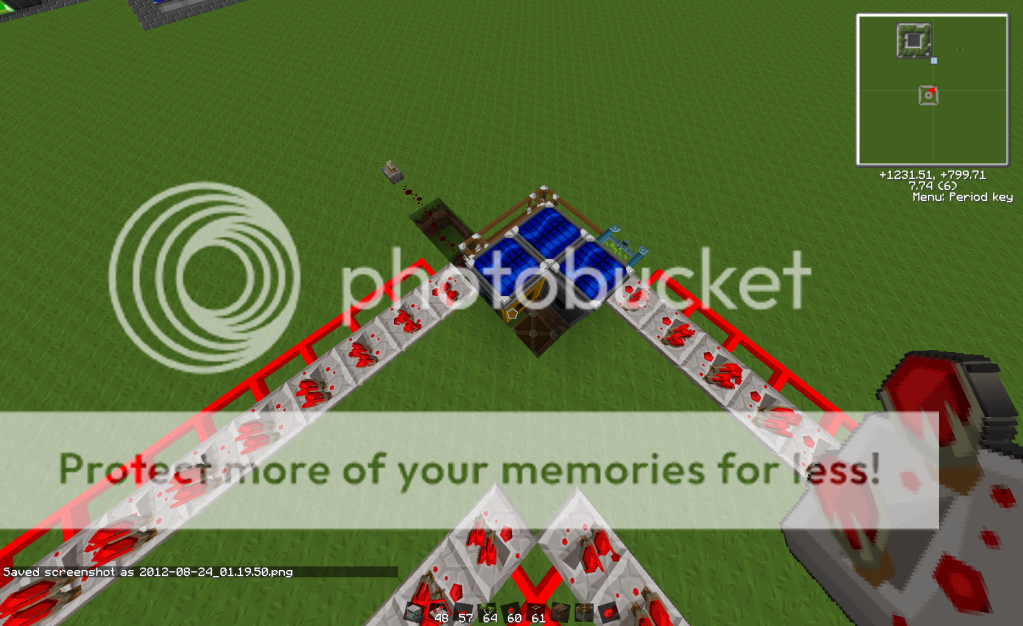
This image shows the setup for the auto collection and stock point.
- It most be located in a corner and keeping in mind that the turtle will always turn right when it encounters something the retriever that it runs into must be retrieving from the chest and the other one from the turtle.
- The one taking from the turtle should be kept blank and the one retrieving from the chest should have 64 sapling in it.
- The one taking from the turtle should have a pipe leading to the chest to allow the sapling taken from the turtle to get reused.
- Under the corner solar panel is a battery box providing the power to the retrievers.
- The turtle will provide the needed red-stone pulse's to power the retrievers
Below is my paste bin like for easy transferring to computer craft as well as my code
http://pastebin.com/ak5RVpQc
Spoiler
--[[
Forestry Turtle 1.0
By: Sayomie555
--]]
local tree = 0
local wood = 0
local height = 0
function data() -- Function to print the logging stats
local sapling = turtle.getItemCount(1) -- Gets the count of saplings in the Turtles inventory (Item slot 1)
local lumber = turtle.getItemCount(2) + turtle.getItemCount(3) + turtle.getItemCount(4) + turtle.getItemCount(5) + turtle.getItemCount(6) + turtle.getItemCount(7) + turtle.getItemCount(8) + turtle.getItemCount(9) -- Counts the ammount of lumber in the turtles inventory (Slots 2-9)
term.clear() -- Clears the screen
term.setCursorPos(1,1) --Sets the cursor position to the start
print("-------Logging Session Stats-------")
print("------------------------------------")
print("Number Of Trees Choped : "..tree)
print("Ammount Of Wood Harvested : "..wood)
print("")
print("Number Of saplings In Stock : "..sapling)
print("Ammount of Lumber In Stock : "..lumber)
print("------------------------------------")
end
function treelocated() -- Function to print that a tree has been found
tree = tree + 1 -- Adds one to the tree counter
data() -- Prints the logging stats
print("Tree Detected Starting Logging")
sleep(0.2)
end
function choplant() -- function to chop down a tree and then replant it
data()
print("Logging")
turtle.dig() -- Chops the base of the tree
turtle.forward() -- Moves the turtle under the tree
wood = wood + 1 -- Counter for the ammount of wood harvested
while turtle.detectUp() do -- Mines upward so long as there is a block above the turtle
turtle.digUp()
turtle.up()
height = height + 1 -- Adds one to the height counter so the turtle knows how far to go back down
data()
print("Logging")
wood = wood + 1
end
wood = wood - 1
data()
print("Returning to Ground")
if not turtle.detectUp() and not turtle.detectDown() then -- Moves the turtle back down
while height ~= 0 do
turtle.digDown() -- Mines under the turtle incase a tree grew around the turtle (Has happened to me :D/>/>)
turtle.down()
height = height - 1
end
end
data()
print("Backing Up")
sleep(0.2)
turtle.back() -- Moves the turtle back
data()
print("Replanting Sapling")
sleep(0.2)
turtle.select(1) -- The turtle selects the first slot in its inventory (The sapling)
turtle.place(1) -- Places the item from the first slot in its inventory
data()
print("Patroling For Trees")
end
function treeleft() -- Function to detect if there is a tree to the left of the turtle
if redstone.getInput("left",true) then -- Checks to see if there is a positive redstone input to the left of the turtle (This is to check if there is a tree vs a sapling)
treelocated() -- Runs the treelocated function
turtle.turnLeft() -- Turns the turtle left to face the tree
choplant() -- Runs the Choplant Function
turtle.turnRight() -- Turns the turtle to the right
end
end
function treeright() -- Same as treeleft but check for trees on the right
if redstone.getInput("right",true) then
treelocated()
turtle.turnRight()
choplant()
turtle.turnLeft()
end
end
function treefront() -- Same as tree left but checks for trees infront
if redstone.getInput("front",true) then
treelocated()
choplant()
end
end
function standby() -- Function for when the turtle is to enter standby
local standby = 0
local seconds = 0
local minutes = 0
local hours = 0
while not turtle.detectDown() do -- Checks to see if the turtle should continue on or go into standby
if seconds > 59 then -- Counter to change seconds into minutes
seconds = 0
minutes = minutes + 1
end
if minutes > 59 then -- Counter to change minutes into hours
minutes = 0
hours = hours + 1
end
if standby <= 0 then -- Prints a message saying the turtle is entering standby mode
data()
print("Entering Stadyby Mode")
sleep(2)
seconds = 2
standby = 1
else -- Prints a screen showing the user how long the turtle has been in standby mode
term.clear() -- Clears the screen
term.setCursorPos(1,1) --Sets the cursor position to the start
print("-----------Standby Mode-----------")
print("In standby Mode for")
print("Hours : "..hours)
print("Minutes : "..minutes)
print("Seconds : "..seconds)
print("")
print("Trees Chopped Last Run : "..tree)
print("Wood Collected Last Run : "..wood)
seconds = seconds + 1
sleep(1)
end
end
if standby == 1 then -- Once the turtle is out of standby mode it resets the counters
tree = 0
wood = 0
end
end
function stock() -- Function to drop off the inventory of the turtle and stock up saplings
if not turtle.detectDown() then -- Checks to see if there is a block under the turtle (If not then the turtle will start the stocking procedure)
local slot = 2 -- Resets the Lumber drop off slot number
while slot ~= 10 do -- Cycles through and checks slots 2-9 (The lumber slots)
data()
print("Dropping off Lumber")
while turtle.getItemSpace(slot) ~= 64 do -- Checks the current slot
redstone.setOutput("back",true) -- Sends a redstone pulse to the retriever to collect the lumber in the turtle
sleep(0.2)
redstone.setOutput("back",false) -- Ends the redstone pulse
sleep(0.2)
end
slot = slot + 1 -- Counter for the inventory slots
end
while turtle.getItemSpace(1) ~= 0 do -- Checks to see if the turtle needs more saplings
data()
print("Stocking Up On Saplings")
redstone.setOutput("left",true) -- Sends a pulse to the retriever to send the turtle a sapling
sleep(0.2)
redstone.setOutput("left",false) -- Ends the redstone pulse
sleep(0.2)
end
turtle.down()
data()
print("Checking for off switch")
standby() -- Checks to see if the turtle should go into standby mode
turtle.up() -- Brings the turtle back up
data()
print("Patroling For Trees")
end
end
while true do -- Creates a loop to keep the turtle running
if not turtle.detect() then -- Checks to see if there is a block infront of the turtle and moves the turtle forward if there is not
turtle.forward()
data()
print("Patroling For Trees")
end
treeleft() -- Runs the treeleft function
treeright() -- Runs the treeright function
treefront() -- Runs the treefront function
if turtle.detect() then -- Checks to see if there is a block infront of the turtle and if there is turns the turtle Right
turtle.turnRight()
data()
print("Turning")
end
treeleft()
treeright()
treefront()
stock()
end