Posted 26 August 2012 - 03:41 AM
Hi, this is my first attempt on CC programming. I know there's a lot of similiar programs but this one is quite complex and (I hope) extremely secure. Plus I made its huge part out of pure boredom when my internet connection went down, so the only api reference I had was ingame.
It was improved since the initial release (here as the official CC forums were down at the time).
You will need
eg. my permanent card
Okay, we've got a card, now we need a terminal:
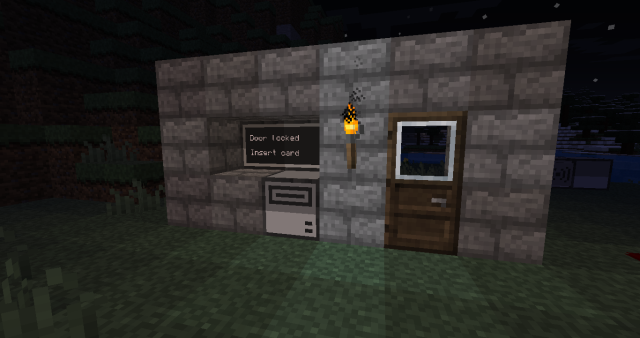
(please ignore it's a wooden door :P/>/>)
As of the version 1.1 the program uses a little api that you have to save as api/devices if you want to use the code without any changes:
Create a startup file on the computer and use this code:
To be able to activate "guest cards" quickly I also created a little program, but notice it was made rather for testing purposes and is highly insecure (until you run it on password protected terminal or something alike).
This is the code:
And /files/id content:
Notes
It was improved since the initial release (here as the official CC forums were down at the time).
You will need
- a computer :D/>/>
- disk drive
- double monitor (optional but recommended)
- redstone output (default: bottom)
- floppy disk
username (only for welcoming message)
password (the same for every user, different between single and permanent mode)
eg. my permanent card
Jahmaican
dupa.8
eg. guest card (one use only)guest
admin1
Okay, we've got a card, now we need a terminal:
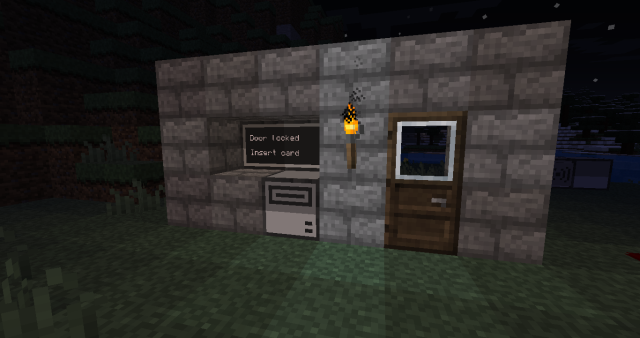
(please ignore it's a wooden door :P/>/>)
As of the version 1.1 the program uses a little api that you have to save as api/devices if you want to use the code without any changes:
function find(name,prior)
local sides = {
"top";
"front";
"left";
"right";
"back";
"bottom" }
if prior==nil then
p=1
else
p=prior
end
for n=1,6 do
if peripheral.getType(sides[n])==name then
if p==1 then
return sides[n]
else
p=p-1
end
end
end
return "none"
end
(also, it's very useful!)Create a startup file on the computer and use this code:
-- CONFIG for your convenience :P/>/> --
rside="bottom" -- redstone output side
itime=2 -- "access denied" message timeout
vtime=3 -- "access granted" message timeout
idfile="/disk/id" -- ID filename
vpass="dupa.8" -- permanent access password
spass="admin1" -- single access password
trm=true -- allow the program to be terminated with Ctrl-T
-- please read further thoughts in "Notes" section of this post
-- END OF CONFIG --
os.loadAPI("api/devices")
dside=devices.find("drive")
function Main()
print("Security access v1.1nby Jahmaicannrunning...")
Monit()
end
function Ismon() -- allows to destroy or connect the monitor
local mside=devices.find("monitor") -- at any time without crashing the program
if mside~="none" then
mon=peripheral.wrap(mside)
ismon=true
else
ismon=false
end
return ismon
end
function Monit()
rs.setOutput(rside,false)
if Ismon()==true then
mon.clear()
mon.setCursorPos(2,2)
mon.write("Door locked")
mon.setCursorPos(2,4)
mon.write("Insert card")
end
while event~="disk" do
event=os.pullEvent()
end
event=nil
Check()
end
function Check()
if Ismon()==true then
mon.clear()
end
if fs.exists(idfile)==false then
Invalid()
end
plik=assert(fs.open(idfile,"r"))
name=plik.readLine()
pass=plik.readLine()
plik.close()
if pass==vpass then
Valid()
single=false
elseif pass==spass then
Valid()
single=true
else
Invalid()
end
end
function Invalid()
if Ismon()==true then
mon.clear()
mon.setCursorPos(2,2)
mon.write("Access denied")
mon.setCursorPos(2,4)
mon.write("Invalid card")
end
disk.eject(dside)
sleep(itime)
Monit()
end
function Valid()
if Ismon()==true then
mon.clear()
mon.setCursorPos(2,2)
mon.write("Access granted")
mon.setCursorPos(2,4)
mon.write("Hi "..name.."!")
end
if single=="true" then -- erases the single access card
if fs.isReadOnly(idfile)==false then
fs.delete(idfile)
disk.setLabel(dside,"Expired_card")
end
end
disk.eject(dside)
rs.setOutput(rside,true)
sleep(vtime)
Monit()
end
function os.pullEvent()
local event, p1, p2, p3, p4, p5 = os.pullEventRaw()
if event == "terminate" then
if trm==true then
print("Terminated")
error()
else
print("Look out, we got a badass over here!")
end
end
return event, p1, p2, p3, p4, p5
end
Main()
To be able to activate "guest cards" quickly I also created a little program, but notice it was made rather for testing purposes and is highly insecure (until you run it on password protected terminal or something alike).
This is the code:
-- CONFIG --
vpass="haslo" -- card admin access password
orfile="/files/id" -- original ID filename
idfile="/disk/id" -- where to copy original file
-- END OF CONFIG --
os.loadAPI("api/devices")
dside=devices.find("drive")
function Main()
print("Guest card validator v1.0nby Jahmaicann")
print("Password needed!") -- obviously password protection here is quite pointless
pass=read("*") -- apart from looking neat :)/>/>
if pass==vpass then
Correct()
else
Incorrect()
end
end
function Correct()
print("Password correct!")
print("Validating guest card...")
if disk.isPresent(dside)==true then
if fs.exists(idfile) then
if fs.isReadOnly(idfile)==false then
fs.delete(idfile)
else
print("[ID file is read only - card may not work]")
end
end
fs.copy(orfile,idfile)
disk.setLabel(dside,"Single_access")
disk.eject(dside)
print("All set!")
else
print("Disk error!")
end
end
function Incorrect()
print("Incorrect password!")
end
Main()
And /files/id content:
guest
admin1
Notes
- Single-access cards shouldn't be trusted too much as they are very easy to duplicate
- The whole system is pointless if other players have permissions to break any blocks around :)/>/>
- I didn't test what will happen when you insert a disk with a "startup" file and reboot the computer with Ctrl-S or Ctrl-R, though these shortcuts are easy to disable the same way I did with Ctrl-T
- As I recently joined a nice tekkit server plus it somewhat interferes with my educational path, I might expand this one into a complete API and a set of programs to suit all your CC security needs!