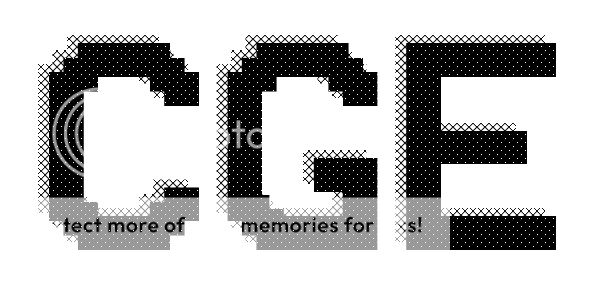
CGE is ComputerCraft Game Engine it is an API which can vastly reduce the amount of code needed to made a mini game (or other applications) and simplify the code needed. It does not do lots currently but I hope for it to grow, with hopefully the communities help. So for this reason I ask you to please reply to say either what you think of it, what your first impressions are, a suggestion for it or anything of that sort. Thank you.
Functions:
Spoiler
constructMap(map)returns a formatted version of the map you gave which CGE can read and use,
so you would input something like {"+++","+++","+++"} and CGE would read a 3x3
cube made out of the "+" symbol only
constuctSprite(sx, sy, sprite, name, maxani)
returns a formatted version of the sprite you gave which CGE can read and use,
so you would input something like {"#","##"} and CGE would read a 2x2 L shape
made out of the "#" symbol only
sx and sy are the starting x/y coordinates of the sprite (the upper left hand corner)
name is the name of the sprite
maxani is the maximum frame of animation that the sprite has; set to 1 if left as nil.
createSprite(xtable, ytable, ttable, name, maxani)
returns a formatted version of the sprite you gave which CGE can read and use,
so you would input something like {0,0,1}{0,1,1}{"#","#","#"} and CGE would read a 2x2 L shape
made out of the "#" symbol only, exactly the same as the constructSprite example
name is the name of the sprite
maxani is the maximum frame of animation that the sprite has; set to 1 if left as nil.
changeSprite(sprite, nsprite)
if sprite is found in the games sprite list then it is replaced with nsprite.
sprite is the original sprite
nsprite is the replacement sprite
addSprite(sprite)
adds the chosen sprite in to the game
deleteSprite(sprite)
deletes the chosen sprite from the game
getSprite(name)
returns the first sprite found with that name
getSprites()
returns a table containing all sprites currently in the game
addSprites(sprites)
adds that list of sprites to the game.
setSprites(sprites)
sets the table containing all the sprites in the game to this new table
getMap()
returns the current map
setMap(map)
sets the map currently being used by the game to the chosen one
setMapLoc(x, y, type)
sets the character at the x, y position on the map to the character chosen (type)
type is the character chosen to replace the one found at the x, y coordinate
moveSprite(sprite, dirx, diry, smart)
returns a new sprite which is the same as the one chosen in the function but has
been moved along the x coordinate by dirx and along the y coordinate by diry
smart is true/false value, if true then the sprite is only moved when it did not collide after movement
checkCollision(sprite, sprites)
returns true/false depending on whether it detected a collision between any of the sprites
in the sprites table and the sprite you chose, ignoring the sprite you chose if it happens
to be in the sprites table
setVelocity(sprite, dir, momentum)
this sets the velocity of the sprite in question
sprite is the sprite having it's velocity set.
dir is the direction of the velocity as a table containing {x,y}, x and y can be any integer
momentum is the force it travels at, if this is greater than zero then the sprite moves when
the velocity is updated.
getVelocity(sprite)
returns the velocity in the format:
"momentum,dirx,diry"
updateVelocity(sprite, resistance, smart)
this updates the velocity of the sprite.
resistance is the amount taken away from the momentum (if the momentum is >0)
smart works the same way as it does in moveSprite()
getCurAnimation(sprite)
gets the current animation frame of the sprite.
sprite is the sprite in question
animateSprite(sprite, forwards)
this loops through the sprites animation frames, moving one back or forwards every call depending
on whether the forwards variable is equals to true or false
sprite is the sprite being animated
forwards is whether or not the animation is scrolling through it's frames forwards or backwards
animateSprites(sprites, forwards)
does the same as animateSprite() but animates every sprite in the 'sprites' table variable, so that
you can easily animate multiple sprites at once.
setSpriteAnimationFrame(sprite, frame)
sets the current animation from of the sprite in question to the frame, if out of frame boundaries then it
is set to the highest/lowest frame boundry, which ever is relevant.
sprite is the sprite having its animation frame edited
frame is the number frame that you are switching to.
getSpriteCode(sprite)
returns a single string containing all information on this sprite
sprite is the sprite being encoded
readSpriteCode(code)
returns a sprite based on the code given
code is the encoded version of the sprite
readSprite(sx, sy, dir, name, maxani, colored)
this returns a sprite created by reading the defined file
sx is the starting x coordinate for the sprite
sy is the starting y coordinate for the sprite
dir is the directory for the sprite to be read from
maxani is the max animation frame (kinda irrelavant for this)
colored is whether or not the file is reading a sprite made of colors or of strings
readAnimatedSprite(sx, sy, dirs, name, maxani, colored)
this is exactly the same as readSprite() however it returns a sprite
with an animation, the only variable difference is:
dirs is a table containing all directors to be read as animation frames, in order of frame 1 to tablesize.
readMap(dir)
returns a map read from a file
addGravity(x, y, force)
adds a gravitational center
x is the x coordinate for the gravitational center
y is the y coordinate for the gravitational center
force is the force (range) for the gravitation center, force uses the formula:
(x + y)/2 = f, where x, y are the coordinates of a sprite and f is the required force
removeGravity(grav)
removes this gravitational center
removeGravity(x, y, force)
removes any gravitational center which has those x, y, and force values.
getGravityPoints()
returns a table containing all gravitiation centers
updateGravity(sprite, smart)
updates the sprite so it is affected by gravity (as long as it's in range of a gravitational center)
sprite is the sprite being updated
smart is whether or not smart movement is allowed (checks for collisions)
getSpriteBounds(sprite)
returns a table containing the x,y coordinates of upper left and lower right corners of the box surrounding the sprite
(an imaginary box)
sprite is the sprite having its bounds taken
flipSprite(sprite, flipx, flipy)
flips the sprite along the chosen axis.
sprite is the sprite being flipped
flipx is true/false depending on whether you want to flip along the x axis
flipy is true/false depending on whether you want to flip along the y axis
colorDraw(offx, offy)
does the exact same as draw() but also checks to see if the sprite is using color tags,
if so then it will color the sprite in the correct colors, if not it will act as normal
(credit to marumaru for the setPixel and getColor functions!)
draw(offx, offy)
draws the current game, offset by the x and y coordinates (the position of every x and y coordinate
is changed by the offx and offy)
Heres a paste of CGE:
Spoiler
http://pastebin.com/r2UReK1MHeres a download:
Spoiler
v1.0 - [attachment=530:cge.zip]v1.1 - [attachment=539:cge.zip]
v1.2 - [attachment=553:cge.zip]
Heres an example program paste:
Spoiler
http://pastebin.com/TwUAkG0gHeres a tutorial (v1.1)
Spoiler
CGE Tutorial:This is a tutorial which will guide you through using GME and all it's functions, I recommened downloading the programs Notepad++ and
CCEmulater in order to code and test your programs easier before starting this tutorial, if you wish, however, you can use your computer
the tutorial will not rely on using any form of coding your program.
To start, make a new program called "pacman"
(note some of the allignment of the code is messed up, however the code still works and should be understandable.)
Step 1: Making a map
Spoiler
You should have nothing but an empty screen when you start programming your program, if not delete everything thats on it (or start a newprogram) before starting this tutorial…
Now, our map could be whatever we want it to be, but for our program we want it to be blank with a border.
To make a map you need to make a new table, lets call our table "map",
local map = {}
now we need to define the map, the map is a table (as I previously stated) and inside the table is what the map (or background) will looklike, each line is one line of the map. If that makes sense, if not look at the code and figure it out (or ask if you still don't understand)
local map = {"+-----------+",
"| |",
"| |",
"| |",
"| |",
"| |",
"| |",
"+-----------+"}
So thats our map it should be pretty obvious what that outputs…
now we need to quickly use the contstructMap function to put it in to CGE format:
local map = {"+-----------+",
"| |",
"| |",
"| |",
"| |",
"| |",
"| |",
"+-----------+"}
local cgemap = cge.constructMap(map)
we also need to add the map in to the game, so to do that we just use the setMap function, very simple:
local map = {"+-----------+",
"| |",
"| |",
"| |",
"| |",
"| |",
"| |",
"+-----------+"}
local cgemap = cge.constructMap(map)
cge.setMap(cgemap)
the function should be easy to understand what it does.Step 2: Making a sprite
Spoiler
In the latest update our sprites can be animate, so lets take advantage of this and create our animated packman:lets make a function which creates a 2 frame animated sprite and call it "makeSprite", so fist create the function
local function makeSprite()
end
now we want a few variables in the makeSprite() parenthesis, those are:
sx - the starting x coordinate of the sprite
sy - the starting y coordinate of the sprite
name - the name of the sprite
c - a table containing all of the frames of the animations
so lets add these in:
local function makeSprite(sx, sy, name, c)
end
now we want to turn all of the characters in c in to one string, each character being split up but a ',', so lets add a table
loop to loop through all of the characters and add them to a string (called type)
we also want to get the size of c, so lets throw in another variable called sz which we will set to i on every loop
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
end
type is the type of string that every string in the sprite is going to be, for each frame.
sz is the size of the c table, I will explain why this is needed a little bit later
now we need to make our x,y,t tables to create (not construct, that doesn't support animations as well) our sprite.
these are going to be rather simple as our sprite is only 1x1 large, so lets quickly create those
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
local xsprite = { sx }
local ysprite = { sy }
local tsprite = {type}
end
now we are ready to create our sprite and then add it to the game, for this we are going to use two CGE
functions,
createSprite(xsprite, ysprite, tsprite, name, maxani)
addSprite(sprite)
(look them up in functions to see what they do if you are unsure of what they do)
as you can see, maxani is required for this function (maximum animation frame), this is where we are going
to use the size of c, as the size of c must equal the amount of frames.
so lets quickly define a new variable as sprite and set it to equal createSprite with our variables plugged in
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
local xsprite = { sx }
local ysprite = { sy }
local tsprite = {type}
local sprite = cge.createSprite(xsprite, ysprite, tsprite, name, sz)
end
now that we have created our sprite we need to add it in to the game, otherwise it won't be drawn…
so for that we just add in our second function, which only requires one variable, sprite.
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
local xsprite = { sx }
local ysprite = { sy }
local tsprite = {type}
local sprite = cge.createSprite(xsprite, ysprite, tsprite, name, sz)
cge.addSprite(sprite)
return sprite
end
this function also returns the sprite we have created, just in case.and thats it, when you call this function with the correct variable it will automatically create your sprite
for you!
Step 3: creating the walls
Spoiler
The walls that pacman is going to collide with must be a sprite, so that we can easily use the "checkCollision" function in CGE.however, as the sprite doesnt need to be animated and is going to be large we can just use constructSprite for this one and create it
in a very similar way to how we created out map.
so first lets initiate a new variable called "prewalls" which will be a table
prewalls = {}
now lets create a blank square which is the same size as the map but -2 in both dimensions (to fit inside the walls
local prewalls = {" ",
" ",
" ",
" ",
" ",
" ",}
now we need to design our walls, we are going to use the 'X' charcter as the character for out walls, so lets first surround the whole
with X
local prewalls = {"XXXXXXXXXXX",
"X X",
"X X",
"X X",
"X X",
"XXXXXXXXXXX",}
now we can fill the insides however we want, I'll give an example here but feel free to design your walls however you want (you can also
make the map bigger if you wish, though you will need to understand the implications it will have on things I will be going through later,
like placing pacman)
local prewalls = {"XXXXXXXXXXX",
"X X X",
"X X X X X",
"X X X X X",
"X X X",
"XXXXXXXXXXX",}
Now we need to create our sprite using the construct method, we will call the sprite "walls" for both its name and variable:
we are also going to make it start at x=1, y=1 so that it is inside the map, as we designed it to do so. the maxani is going to be
1 as there is no animation
local prewalls = {"XXXXXXXXXXX",
"X X X",
"X X X X X",
"X X X X X",
"X X X",
"XXXXXXXXXXX",}
local walls = cge.constructSprite(1, 1, prewalls, "walls", 1)
now lets quickly throw in the addSprite method to add it to the game as well.
local prewalls = {"XXXXXXXXXXX",
"X X X",
"X X X X X",
"X X X X X",
"X X X",
"XXXXXXXXXXX",}
local walls = cge.constructSprite(1, 1, prewalls, "walls", 1)
cge.addSprite(walls)
and we are done! the walls are added in to the game and will obstruct pacman when we get round to making him.
Part 4: creating pacman
Spoiler
Firstly, lets create pacman using our previously set makeSprite function;pacmans location will be x=8, y=3 as our map is 13 wide so to put him in the middle x must equal 8 and y=3 moves him down enough so that
he is not hitting anything, his characters will be
"@" and "O" and his name will be "pacman".
local pacman = makeSprite(8, 3, "pacman", {"@","O"}
thats it, I couldn't really think of anything which should go along side this part so it just shows how simply making a sprite is.
However before we move on to part 5, which start to make the game actually run, we should take a look at where we should be.
Please actually complete the tutorial instead of copying this code though:
Spoiler
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
local xsprite = { sx }
local ysprite = { sy }
local tsprite = {type}
local sprite = cge.createSprite(xsprite, ysprite, tsprite, name, sz)
cge.addSprite(sprite)
return sprite
end
local map = {"+-----------+",
"| |",
"| |",
"| |",
"| |",
"| |",
"| |",
"+-----------+"}
local cgemap = cge.constructMap(map)
cge.setMap(cgemap)
local prewalls = {"XXXXXXXXXXX",
"X X X",
"X X X X X",
"X X X X X",
"X X X",
"XXXXXXXXXXX",}
local walls = cge.constructSprite(1, 1, prewalls, "walls", 1)
cge.addSprite(walls)
local pacman = makeSprite(8, 3, "pacman", {"@","O"}
Check your code against this, make sure you have gone through everything correctly!Part 5: creating a game loop
Spoiler
This part actually has nothing to do with CGE, but it is essential in making almosty any game or application which runs of a similar basis.At the bottom of the code we first want to initialize our timer and our on boolean check (as varibles don't have names in Lua you may not know what a boolean is,
a boolean is a variable which can only have one of two possible values, in most cases it is true or false instead of a logical 0 or 1)
so lets create our 2 variables:
local on = true
local timer = os.startTimer(0)
the use of the on variable will be explained a little bit later, the use of the timer varibale is to notify the game every time it should update.At first the timer is set to 0 so that there is little delay between starting up the program and the game starting, however we do want an interval between updates,
however only something small. For pacman 0.5 seconds should be adequet, so lets create a new variable called 'interval' which is defined as 0.5 so that it is easy
to use and change our interval time.
local on = true
local timer = os.startTimer(0)
local interval = 0.5
Now we need to start our while loop, our while loop is going to check every time to see if on = true, if it is false then it will stop the loop and the game will
stop, this is so that we can add an escape function for if the user wishes to quit the game.
So lets start our while loop:
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
end
Inside the while loop we want to use os.pullEvent, this checks for events being triggered to do with the computer, if you don't fully understand what I'm defining
and doing with the function and the variables I am initializing out of it look at this CC wiki article:
http://computercraft...le=Os.pullEvent
or look at this tutorial which explains a bit about os.pullEvent and what it is usually used for
http://www.computerc...__fromsearch__1
so now lets get our event:
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
end
now we want to checks, we want to check is event is equals to the string "timer" (and if p1 is to the variable "timer") and we want a second elseif check
to check if the event is equal to the string "key".
So lets add this if + elseif statement in underneith the os.pullEvent()
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
elseif event == "key" then
end
end
now inside the timer if statement we need to start by doing one thing; reset the timer.
this time we will be using the interval variable we set earlier and doing almost an identical line to how we initiated the timer.
So lets add this line in:
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
elseif event == "key" then
end
end
this is the end of the game loop part, it continues with the same code in the next part.
Part 6: updating the game
Spoiler
now we need to draw and update the game every time the timer is called, so first lets start of with clearing the console andsetting the cursor position to 1,1, these functions should be known to anyone with adequet enough knowledge of CC to be following
this tutorial…
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
elseif event == "key" then
end
end
so thats simple enough, now we want to draw our map, so lets add in the cge draw function, this is very simple and doesn't
really need much explanation:
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
elseif event == "key" then
end
end
the 1,1 offset the map by x=1 and y=1, this means when drawing both map and sprites all x values have 1 added on to them andall y values have 1 added on to them, these could be 0 or any other number you chose to put in them.
now we want to update two things for our pacman; the pacman's animation and the pacman's velocity.
lets start with the animation, this is very simple:
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
cge.animateSprite(pacman, true)
elseif event == "key" then
end
end
pacman is the sprite we are updating, true is whether you are moving the animation forwards or backwards, true isfor forwards, false is for backwards.
if animating multiple sprites at once you can use cge.animateSprites(sprites, true/false)
this is exactly the same apart from sprites is a table containing all of the sprites you wish to update, to do this
with all sprites in the game you can do:
cge.animateSprites(cge.getSprites(), true/false)
cge.animateSprite() does return a new sprite, however we do not need to use this new sprite as it also updates the
sprite for us.
now, we want to update pacman's velocity.
we will use the smart movement feature on the updateVelocity function so that we don't have to deal with it colliding with our only
other sprites, walls, however if we make it more complex and add things like ghosts, fruits and coins in to the game then we would
have to check the collisions our self as smart wouldn't work.
so lets add in our updateVelocity function then I will explain what it does.
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
cge.animateSprite(pacman, true)
local npacman = cge.updateVelocity(npacman, 0, true)
elseif event == "key" then
end
end
pacman is our sprite, this is the sprite which is having it's velocity updated.0 is our resistance, our momentum is taken away by this everytime the velocity is updated.0 means it carries on moving forwards until
I change it manually.
true means smart movement is on, this means that if it collides with another sprite when it moves it returns it to it's original position.
we created a new variable called 'npacman' which is the updated version of pacman
now we need to change the original pacman to this updated version of pacman.
To do this we use the function: changeSprite()
so lets add this in and then I will explain what it does.
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
cge.animateSprite(pacman, true)
local npacman = cge.updateVelocity(npacman, 0, true)
cge.changeSprite(pacman, npacman)
elseif event == "key" then
end
end
pacman is the original sprite which it will look for when looking through the game's sprites,npacman is the the sprite that will replace the original sprite if it is found in the game's sprites
we also need to refresh our pacman sprite every loop,
so we use the getSprite() function to get a sprite by its name.
Lets add that in at the end of our loop, and also whenever the timer is called (this helps stop bugs occurring):
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
pacman = cge.getSprite("pacman")
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
local npacman = cge.animateSprite(pacman, true)
npacman = cge.updateVelocity(npacman, 0, true)
cge.changeSprite(pacman, npacman)
elseif event == "key" then
end
pacman = cge.getSprite("pacman")
end
now that sit, the has drawn and updated itself and will continue to do so every 0.5 seconds as long as on = true.
Part 7: adding controls
Spoiler
This is the final part of our basic pacman game, we will be using the second if statement to add controls for our pacman.The first thing we need to do is define our key as p1, so lets do that:
(I will only be using this if statement this time, to save space)
elseif event == "key" then
local key = p1
end
now we want to add a check for 5 different keys, up arrow, left arrow, right arrow, down arrow and escape.
You can look these up using this mincraft wiki page: http://www.minecraft.../wiki/Key_Codes
so to check if a key is a certain keycode you simply do
if key == keycode then,
so lets add all 5 of our checks in strait away:
elseif event == "key" then
local key = p1
if key == 1 then --escape key
elseif key == 200 then --up arrow key
elseif key == 208 then --down arrow key
elseif key == 203 then --left arrow key
elseif key == 205 then --right arrow key
end
end
now in the escape key section all we need to add is on = false
this will imidiatly stop the game as it will stop the while loop.
Lets add that in:
elseif event == "key" then
local key = p1
if key == 1 then --escape key
on = false
elseif key == 200 then --up arrow key
elseif key == 208 then --down arrow key
elseif key == 203 then --left arrow key
elseif key == 205 then --right arrow key
end
end
now for all of the other keys we need to set pacmans velocity, this is a simple function that I will go through
now then add in to all 4 at once.
the function is:
setVelocity(sprite, dir, momentum)
sprite is the sprite in question
dir is a table containing only {x,y}, this is the amount that the sprite will move in the x direction and the
amount that the sprite will move in the y direction.
momentum is the momentum it has, this is irrrelivant for us as we do not have resistance, as long as it is above 0
then we can set it to whatever number we want.
the function returns a newsprite so we will have to again change the sprite in the game using changeSprite().
so lets add those in.
elseif event == "key" then
local key = p1
if key == 1 then --escape key
on = false
elseif key == 200 then --up arrow key
local npacman = cge.setVelocity(pacman, {0,-1}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 208 then --down arrow key
local npacman = cge.setVelocity(pacman, {0,1}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 203 then --left arrow key
local npacman = cge.setVelocity(pacman, {-1,0}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 205 then --right arrow key
local npacman = cge.setVelocity(pacman, {1,0}, 1)
cge.changeSprite(pacman, npacman)
end
end
the only thing that changes in each of these is the x,y values, making it move in the direction that you choseusing your arrow keys.
and thats it for your controls.
We have finished our basic pacman game now, all we need to do is put all of the code together and add two extra line
to the bottom of the code:
term.clear()
term.setCursorPos(1,1)
this will reset the screen once the game is quit.So here is the finished code,
please do not just copy this if you are following the tutorial as it will not help you nearly as much as following the tutorial will:
Spoiler
local function makeSprite(sx, sy, name, c)
local type = ""
local sz = 0
for i,v in ipairs(c) do
if i == 1 then
type = v
else
type = type..","..v
end
sz = i
end
local xsprite = { sx }
local ysprite = { sy }
local tsprite = {type}
local sprite = cge.createSprite(xsprite, ysprite, tsprite, name, sz)
cge.addSprite(sprite)
return sprite
end
local map = {"+-----------+",
"| |",
"| |",
"| |",
"| |",
"| |",
"| |",
"+-----------+"}
local cgemap = cge.constructMap(map)
cge.setMap(cgemap)
local prewalls = {"XXXXXXXXXXX",
"X X X",
"X X X X X",
"X X X X X",
"X X X",
"XXXXXXXXXXX",}
local walls = cge.constructSprite(1, 1, prewalls, "walls", 1)
cge.addSprite(walls)
local pacman = makeSprite(8, 3, "pacman", {"@","O"})
local on = true
local timer = os.startTimer(0)
local interval = 0.5
while on do
local event, p1, p2 = os.pullEvent()
if event == "timer" and p1 == timer then
pacman = cge.getSprite("pacman")
timer = os.startTimer(interval)
term.clear()
term.setCursorPos(1,1)
cge.draw(1,1)
cge.animateSprite(pacman, true)
local npacman = cge.updateVelocity(pacman, 0, true)
cge.changeSprite(pacman, npacman)
elseif event == "key" then
local key = p1
if key == 1 then --escape key
on = false
elseif key == 200 then --up arrow key
local npacman = cge.setVelocity(pacman, {0,-1}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 208 then --down arrow key
local npacman = cge.setVelocity(pacman, {0,1}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 203 then --left arrow key
local npacman = cge.setVelocity(pacman, {-1,0}, 1)
cge.changeSprite(pacman, npacman)
elseif key == 205 then --right arrow key
local npacman = cge.setVelocity(pacman, {1,0}, 1)
cge.changeSprite(pacman, npacman)
end
end
pacman = cge.getSprite("pacman")
end
term.clear()
term.setCursorPos(1,1)
Thanks for following my tutorial and for using CGE!
Updates:
Spoiler
v1.0^initial release
v1.1
+setSpriteAnimationFrame()
+animateSprites()
+animateSprite()
+getCurAnimation()
+updateVelocity()
+getVelocity()
+setVelocity()
+addSprites()
+changeSprite()
*createSprite()
*constructSprite()
*moveSprite()
^added tutorial
^lots of bug fixes!
v1.2
+getSpriteCode()
+readSpriteCode()
+readSprite()
+readAnimatedSprite()
+readMap()
+addGravity()
+removeGravity()
+removeGravity()
+getGravityPoints()
+updateGravity()
+getSpriteBounds()
+flipSprite()
+colorDraw()
^A few small bug fixes
Please reply like I said earlier :D/>/>
Thanks!