Posted 24 November 2012 - 06:05 PM
Hello,
I have recently released my AdventureMap peripheral and now that I've sat down to do some lua coding I've encountered a weird issue. This issue only happens in the compiled version (or at least I have not encountered it in the decompiled one).
What happens is this:
I have a peripheral function called "peripheral.findComputer()" which finds the x,y,z coordinates of the computer attached to the peripheral. The function works fine for a while, but for some reason after about five minutes or so the function starts calling "peripheral.playerCoords()", which gets the coordinates of the player. The peripheral.findComputer() function requires no arguments, and the peripheral.playerCoords() function does.
Here are some screenshots:
Working: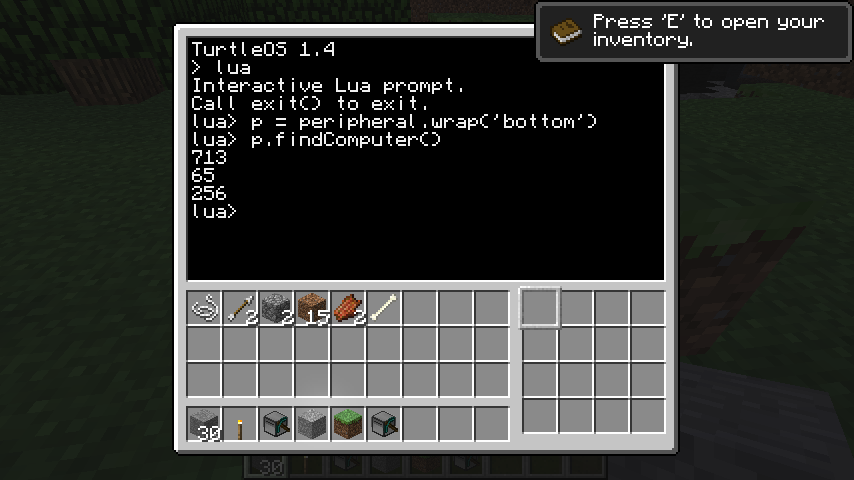
Not working (several minutes later):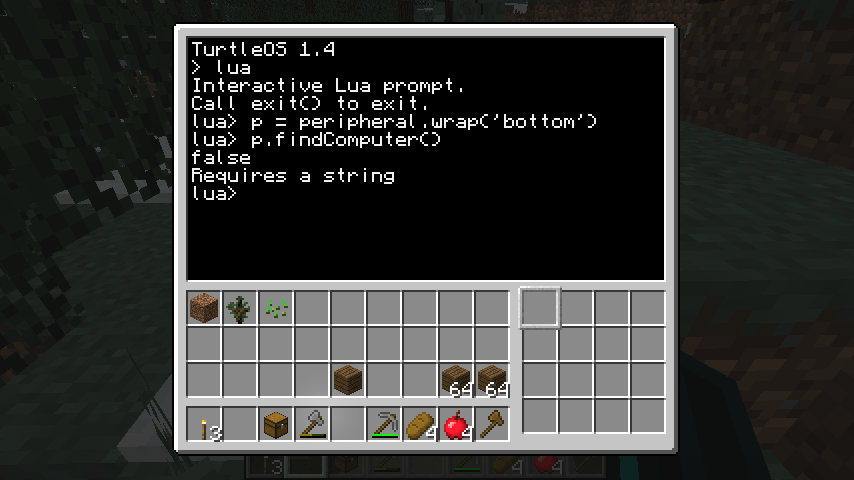
It seems to be a problem localized to each created world as creating a new world fixes the problem for several minutes before it reverts back to the broken state. Here is the relevant java code:
Here is a link to the entire file in case it is for some reason necessary: http://pastebin.com/GFg6b02K
My method of finding the computer's location is terrible (and temporary), but it shouldn't be effecting what method is called when I use these functions. It is especially strange to me that it would work temporarily but then change for no apparent reason.
And before anyone asks, yes I have recompiled the peripheral and done testing in eclipse debugging mode. It worked fine in eclipse debugging mode, but as soon as I compile it and run it the regular way, it does this.
Any help would be greatly appreciated.
I have recently released my AdventureMap peripheral and now that I've sat down to do some lua coding I've encountered a weird issue. This issue only happens in the compiled version (or at least I have not encountered it in the decompiled one).
What happens is this:
I have a peripheral function called "peripheral.findComputer()" which finds the x,y,z coordinates of the computer attached to the peripheral. The function works fine for a while, but for some reason after about five minutes or so the function starts calling "peripheral.playerCoords()", which gets the coordinates of the player. The peripheral.findComputer() function requires no arguments, and the peripheral.playerCoords() function does.
Here are some screenshots:
Working:
Spoiler
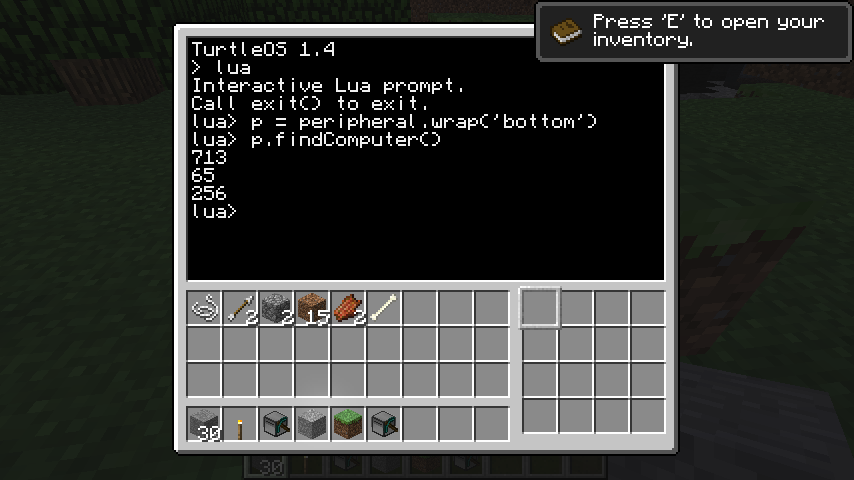
Not working (several minutes later):
Spoiler
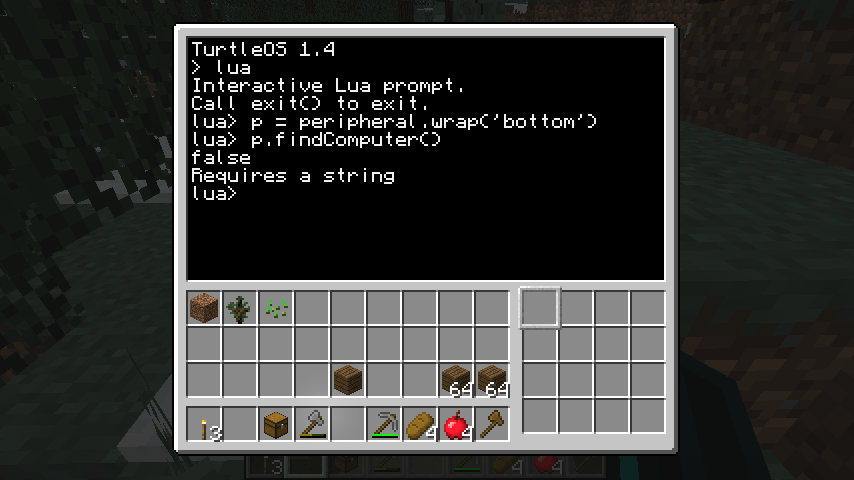
It seems to be a problem localized to each created world as creating a new world fixes the problem for several minutes before it reverts back to the broken state. Here is the relevant java code:
//Get the method names
public String[] getMethodNames()
{
return new String[] { "getBlock", "setBlock", "findPeripheral", "findComputer","playerCoords", "getHealth", "attackPlayer","setPlayerPos", "givePlayer", "getPlayers", "say", "createFireball", "playerLooking", "createExplosion" };
}
//later in the file
case 3: {
int cX = this.xCoord;
int cY = this.yCoord;
int cZ = this.zCoord;
for (int x=cX-3; x<=cX+3; x++) {
for (int y=cY-3; y<=cY+3;y++) {
for (int z=cZ-3;z<=cZ+3; z++) {
if (worldObj.getBlockId(x,y,z) == 210 || worldObj.getBlockId(x,y,z) == 207) {
return new Object[] {x,y,z};
}
}
}
}
//immediately after the previous code
case 4: {
if (arguments.length < 1) {
return new Object[] {false, "Requires a string"};
} else {
World world = this.worldObj;
EntityPlayer player = world.getPlayerEntityByName((String) arguments[0]);
if (player == null) {
return new Object[] {null};
} else {
return new Object[] {player.posX, player.posY, player.posZ};
}
}
}
}
Here is a link to the entire file in case it is for some reason necessary: http://pastebin.com/GFg6b02K
My method of finding the computer's location is terrible (and temporary), but it shouldn't be effecting what method is called when I use these functions. It is especially strange to me that it would work temporarily but then change for no apparent reason.
And before anyone asks, yes I have recompiled the peripheral and done testing in eclipse debugging mode. It worked fine in eclipse debugging mode, but as soon as I compile it and run it the regular way, it does this.
Any help would be greatly appreciated.