7508 posts
Location
Australia
Posted 14 January 2013 - 05:02 AM
Thread Closed in favour of merging to a single, easier to manage, thread and websiteSpoiler
File System Iterator
Spoiler
This is a simple little utility with one function call that can be used in your programs to iterate the files and folders on your computer and store their paths into a table… You can also tell it sequences or paths to ignore all together ( handy for those with OSes and want to hide their OS files from their file managers )…
Initial version ( stores file system data in a supplied table ):
Spoiler
Function:
function iterateFileSystem( startPath, tableToStoreInto, debug, tableOfIgnores )
This is the only function to use in this utility. It requires the following parameters:- A start path
- A table to write the file paths into
- A boolean as to display console messages (normally id suggest false, unless it doesn't seem to be working like you expected)
- A table of all the paths and or sequences to ignore
Usage Code Example (also a nice way to debug what its gathering) :
local files = {}
iterateFileSystem( "/", files, false, { "/rom", ".git" } )
textutils.pagedPrint( table.concat( files, "\n" ) )
This will gather all the files that aren't in the /rom folder or contain the key .git in it… this includes folders and files ( set debug to true to see what its ignoring, if anything matches of course )…
Please note that as this uses recursion to iterate the file system if the file system has enough subfolders, in subfolders, in subfolders, ( etc, etc, etc) it may cause a stack overflow error…
It can support a large amount of files/folders… Currently in my test environment I have 852 files and it processes in less than 1 second… So HAVE FUN!
Download here
Get it in-game with pastebin get nQsArDRi iterator
New update thanks to Eric ( requires use of an iterator to iterate file system ) :
Spoiler
Function:
function fileSystemIter( startPath, tableOfIgnores, debug )
This is the only function to use in this utility. It requires the following parameters:- A start path
- A table of all the paths and or sequences to ignore
- A boolean as to display console messages (normally id suggest false, unless it doesn't seem to be working like you expected)
Usage Code Example (also a nice way to debug what its gathering) :
for fullPath, filename in fileSystemIter("/", { "rom", ".git" }, false) do
print(fullPath, filename)
end
This will gather all the files that aren't in the /rom folder or contain the key .git in it… this includes folders and files ( set debug to true to see what its ignoring, if anything matches of course )…
Download here
Get it in-game with pastebin get 9Fg3uYAd iterator
A big thanks to Eric who beat me to fixing my solution and did the iterators solution…
File Hex Dump
Spoiler
Why you might ask? Because I can I would say… honestly though I was bored and was looking through old uni assignments, saw this and wondered if I could implement this in Lua… Turns out I can… ;)/>
Functions:
There is only one function in this and it is
function hex_dump( inputFile, outputFile )
The parameters are fairly self explanatory, give it a file path for input and output…
Example Usage:
hex_dump( "startup", "hexd_startup" )
Download here
Get it in-game with pastebin get rFx9Jd9G hexdump
Circle Drawing Function
Spoiler
Nothing much to explain with this one… call the function with a centre x, centre y, radius and a color ( optional, also can draw on CC1.3 and CC1.4 non-advanced computers )
And it will output the closest thing to a circle that can be drawn with rectangular pixels… You can also call the solid function with the same parameters for a solid circle to be drawn.
Screenshots
Spoiler
Circle:
Spoiler
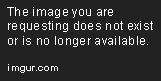
Solid circle:
Spoiler
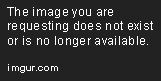
Download here
Get it in-game with pastebin get SwEFYCxw circle
Touchscreen Monitor Right-Click
Spoiler
I just threw this together in about 1 minute ( doing so many other projects atm that have little time :P/> ) so there is one bug and one little quirk, if you can figure either of these out just tell me in the comments below…
Are you developing a program for a monitor and want to have it so that the user can left-click and-right click? Well if you use this little script as the main loop of your program, just adding in anything else you want, you can do that*…
* there is a bug where occasionally a right-click event will be sent as 2 separate, left-click events… also if they are quick enough they can register a right-click by touching different parts of the monitor
Screenshot
Spoiler
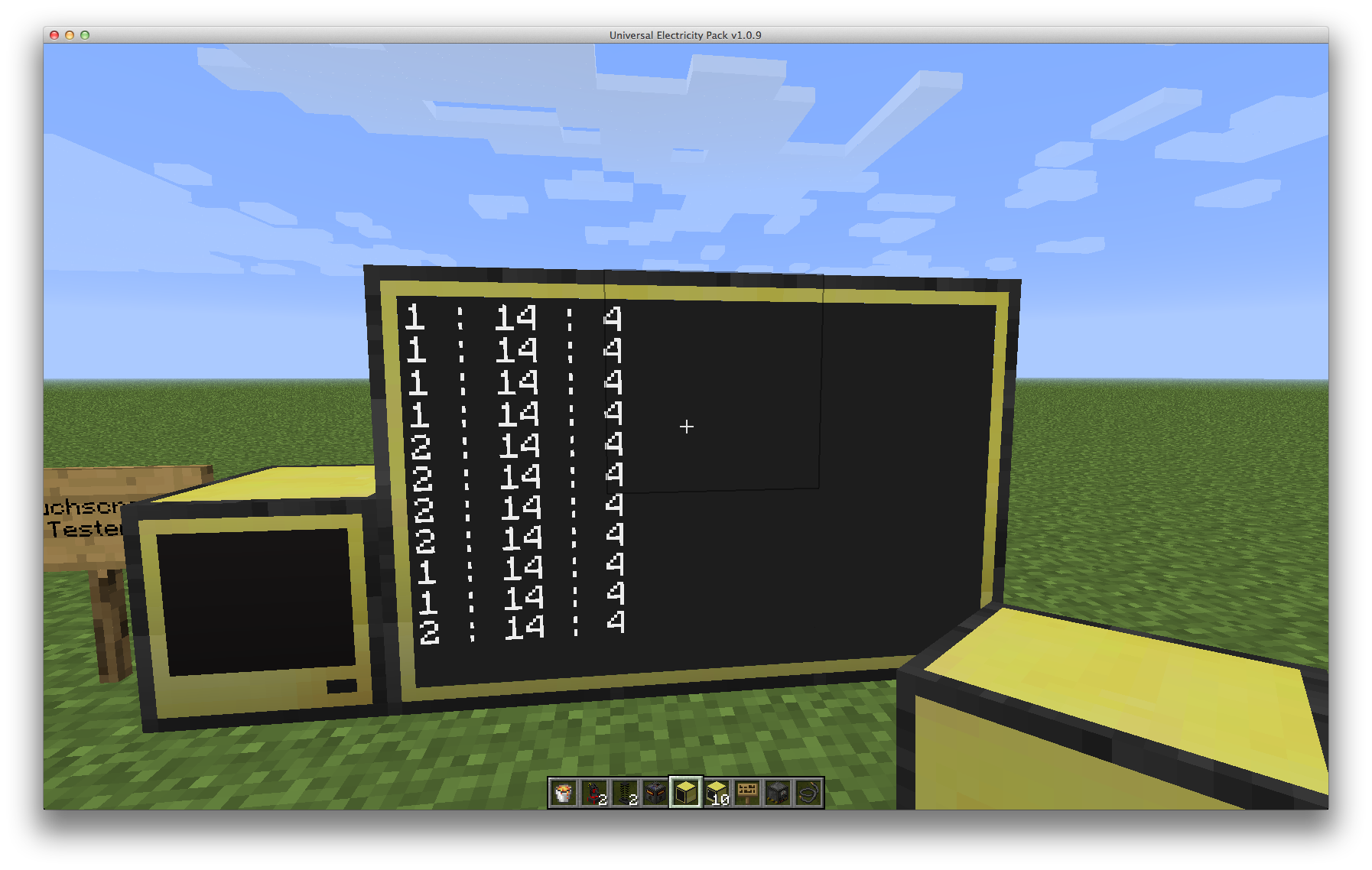
Download here
Get it in-game with pastebin get LkfDRrAt touch
Vigenere Cipher
Spoiler
For information on a Vigenere Cipher read this wikipedia article: http://en.wikipedia....Vigenère_cipher
Functions:
function encodeString( aString, aKey )
Encodes a supplied string with a supplied key, key must consist of ASCII alphabetic characters
function decodeString( aString, aKey )
Decodes a supplied string with a supplied key, key must consist of ASCII alphabetic characters
Download here
Get it in-game with pastebin get N5fGgKLD vegenere
Edited on 23 January 2013 - 06:29 PM
88 posts
Location
UK
Posted 14 January 2013 - 09:15 AM
A few tips for your code:
- Instead of if not startPath then startPath = "/" end consider startPath = startPath or "/"
- fs.combine handles trailing/leading slash problems
- There are nicer ways to write iterators in lua than passing in a table and having your code populate it. Check out PIL 7 and 9.3.
With these changes applied:
local function yieldFileSystem(startPath, ignorePaths, debug)
startPath = startPath or "/"
ignorePaths = ignorePaths or {}
local fsList = fs.list(startPath)
for _, file in ipairs(fsList) do
local path = fs.combine(startPath, file)
if not contains(ignorePaths, path) then
if fs.isDir(path) then
yieldFileSystem(path, ignorePaths, debug)
else
coroutine.yield(path, file)
end
else
if debug then print("Ignoring: "..path) end
end
end
end
function fileSystemIter(startPath, ignorePaths, debug)
startPath = startPath or "/"
ignorePaths = ignorePaths or {}
return coroutine.wrap(function()
yieldFileSystem(startPath, ignorePaths, debug)
end)
end
Which can then be used as:
for fullPath, filename in fileSystemIter("/", { "/rom", ".git" }) do
print(fullPath, filename)
end
7508 posts
Location
Australia
Posted 14 January 2013 - 10:59 AM
A few tips for your code:
- Instead of if not startPath then startPath = "/" end consider startPath = startPath or "/"
- fs.combine handles trailing/leading slash problems
- There are nicer ways to write iterators in lua than passing in a table and having your code populate it. Check out PIL 7 and 9.3.
Thanx for the fixes… I threw this together at about 3am… so was gunna fix up any coding mistakes from being tired when I woke up this morning…
Using the table population over an iterator was a coding choice I made late last night, because of these reasons:
- I was allowing for ease of use on the end where people actually use it
- Even though we know how to use iterators does not mean everyone does, it seems quite a few of the people that are writing the popular OSes and programs that would use this, are fairly new to programming and only know the basics (including only the basics of tables)
- Having a table they don't have to iterate the file system each time they wanted to do something
Continuing on the third point, they could then just access the table and stop when they have found what they are looking for, instead of having a recursive function that has to run to the end and they cant stop it. Granted they could initially iterate and instead of printing the value store it in a table, the just do the intended outcome from that table…. but I didn't think of that until now :P/>
Lastly, did you actually run that solution you made before trying to push it to my git? if you did you would notice that it actually still iterates the ignore paths if its in the root directory for example if I enter "/rom" it WILL iterate rom (and it shouldn't), if I enter "rom" it won't iterate rom… not using fs.combine was a coding choice that allowed it to work EITHER way the user input it… granted it now occurs to me that i could have just used "/"..fs.combine but thats not the point I'm making here :P/>
Again thanx for the fix though :)/>
88 posts
Location
UK
Posted 14 January 2013 - 11:26 AM
Thanx for the fixes… I threw this together at about 3am… so was gunna fix up any coding mistakes from being tired when I woke up this morning…
Using the table population over an iterator was a coding choice I made late last night, because of these reasons:
- I was allowing for ease of use on the end where people actually use it
- Even though we know how to use iterators does not mean everyone does, it seems quite a few of the people that are writing the popular OSes and programs that would use this, are fairly new to programming and only know the basics (including only the basics of tables)
- Having a table they don't have to iterate the file system each time they wanted to do something
Ok, I hadn't considered those things.
Continuing on the third point, they could then just access the table and stop when they have found what they are looking for, instead of having a recursive function that has to run to the end and they cant stop it. Granted they could initially iterate and instead of printing the value store it in a table, the just do the intended outcome from that table…. but I didn't think of that until now
But that accesses the entire filesystem first! If you break out of the for loop with my iterator, the entire thing stops working.
Lastly, did you actually run that solution you made before trying to push it to my git? if you did you would notice that it actually still iterates the ignore paths if its in the root directory for example if I enter "/rom" it WILL iterate rom (and it shouldn't), if I enter "rom" it won't iterate rom… not using fs.combine was a coding choice that allowed it to work EITHER way the user input it… granted it now occurs to me that i could have just used "/"..fs.combine but thats not the point I'm making here :P/>
Running things is hard :P/>. My bad.
7508 posts
Location
Australia
Posted 14 January 2013 - 11:32 AM
Ok, I hadn't considered those things.
I might post up both versions so they can choose… :)/>
But that accesses the entire filesystem first!
Which isn't really much, like I said in OP I have 852 files in my file system and its done in less than a second…
If you break out of the for loop with my iterator, the entire thing stops working.
Thats my point, you break from an iterator and it stops working… break from a loop iteration (while searching for a particular path) and it doesn't stop anything from working… minor details though…
Running things is hard :P/>. My bad.
Haha thats ok
EDIT: Oh and also the reason I used
if debug == true then
was also a coding choice at the time so that it will ONLY debug when the param is true, not when its accidental and might be "/rom" because they put an ignore in the wrong place… :)/>
7508 posts
Location
Australia
Posted 16 January 2013 - 01:09 PM
Added some new programs. Most notable: Touchscreen Right-Click!
892 posts
Location
Where you'd least expect it.
Posted 16 January 2013 - 03:11 PM
In the Touchscreen Right Click part it says:
pastebin LkfDRrAt touch
instead of:
pastebin get LkfDRrAt touch
XP
7508 posts
Location
Australia
Posted 16 January 2013 - 03:17 PM
-snip-
Thanx for picking up on my typo :)/>
7508 posts
Location
Australia
Posted 18 January 2013 - 12:25 PM
Added Vigenere Cipher
392 posts
Location
Christchurch, New Zealand
Posted 22 January 2013 - 07:36 PM
encodeString
Decodes a string?
LOLWHAT! CopyPasta fail, T.O-BIT
7508 posts
Location
Australia
Posted 22 January 2013 - 07:39 PM
encodeString
Decodes a string?
LOLWHAT! CopyPasta fail, T.O-BIT
Yeh its was late when i did that… never even noticed it… fixed… thanx
392 posts
Location
Christchurch, New Zealand
Posted 22 January 2013 - 07:41 PM
You're welcome :)/>
Nice work btw, Just been reading about the algorithm, which paved it's way to a frequency analysis topic.. I wonder if that has a place in CC xD
7508 posts
Location
Australia
Posted 22 January 2013 - 07:47 PM
You're welcome :)/>
Nice work btw, Just been reading about the algorithm, which paved it's way to a frequency analysis topic.. I wonder if that has a place in CC xD
Thanx :)/> Yeh its was an interesting topic when we did it at uni…. there is a major flaw to the cypher tho, when encoding a 'Z' with any key char or encoding any char with a 'Z' in the key, the output is no different… its since been shifted a little to not have that happen, however we had to implement the original one ( made it easier to debug ) so thats what I did for this one… We actually had to implement the frequency analysis that was outlined on the wikipedia page… but i saw little point to that one… why would I post a way to crack the cypher on the same post as the cypher. :P/>
actually on second thought it was the shifted one that had the Z flaw… it was the original that had the flaw of encoding a letter with the same letter, yielded no change… yeh that sounds better :P/>
Edited on 22 January 2013 - 06:54 PM
423 posts
Location
AfterLifeLochie's "Dungeon", Australia
Posted 25 January 2013 - 12:47 AM
Locked by request.