As the name suggest, this is a set of peripherals oriented to managing inventories, it comes with 2 peripherals:
- Player Manager
- Inventory Manager
The player manager is a plate, connected to a computer on the side, and an inventory under it, that can detect players walking on it and manipulate their inventory.
Recipe:
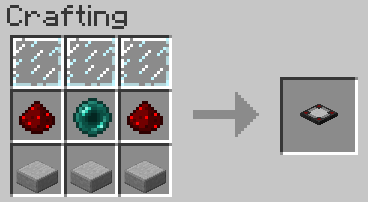
Screenshots showing respectively the offline (no computer nearby), online (computer nearby but no player on plate) and active (player on the plate with computer nearby) states of the plate:
Spoiler
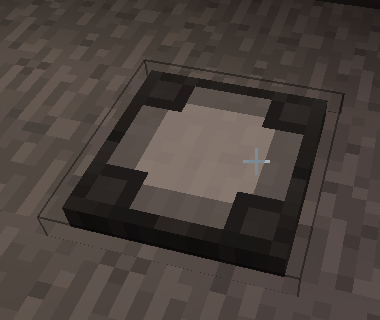
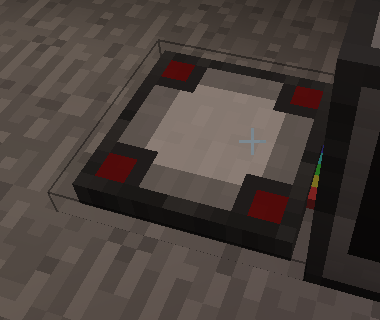
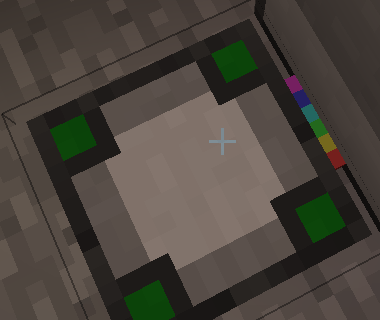
With this, you can do stuff like automatically detecting ores the player and send them to smelting, here is a video of a small contraption I made (click on the image):
Spoiler
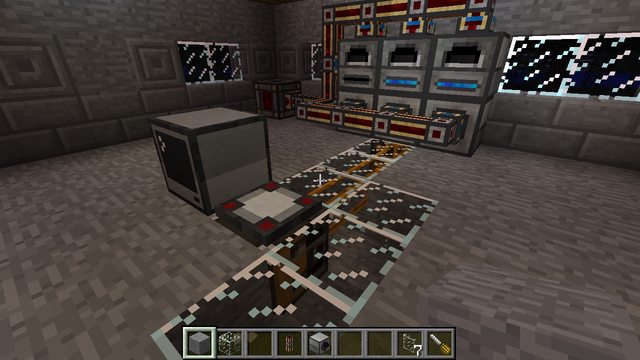
The program used in the video is the following (with more comments) [TERRIBLY OUT OF DATE, PLEASE SEE API DESCRIPTION]:
Spoiler
m = peripheral.wrap("right")
while true do
-- wait for a player to step on the plate
os.pullEvent("playerAvailable")
-- retrieve the inventory size
size = m.size("player")
-- iterate on it
for i=0,size-1 do
-- read item in inventory at slot i, for the player inventory, slots 0 to 8 are the hotbar, 9 to 39 are the real inventory, starting from the top left
-- the "item" object contains 5 infos: id, name, display, amount, damage
-- id is the item id
-- name is the name inside the MC engine, like ore.copper, or tile.oreGold
-- display is the beautified name, like "Copper Ore" or "Gold Ore"
-- amount is the amount of items in this slot
-- damage is the damage on the item (or for some mods, the energy)
item = m.read("player", i)
-- if there is no item in this slot, the function returns 0
if item ~= 0 then
-- do a bunch of checks on the name, and call the export function, that function takes whatever is in the slot i of the player, and send it to the inventory
-- under the plate, or a buildcraft compatible pipe, in this demo I use a redpower relay that then takes care of throwing the items in the rp tubes
-- this function returns an object with the same infos then the read function, but amount says how much items were not exported, and a new variable
-- "exported" says how many items where succesfully exported
if string.sub(item.name,0,4) == "ore." or string.sub(item.name,0,8) == "tile.ore" then
res = m.move("player", i, "down")
print("exported ", res.moved, " units of ", item.display)
end
end
end
end
Automatic Player Manager
This version of the player manager doesn't connect to computers, but can still be used by BuildCraft pipes, RedPower Sorting Machines and other mods manipulating inventories.
Recipe:
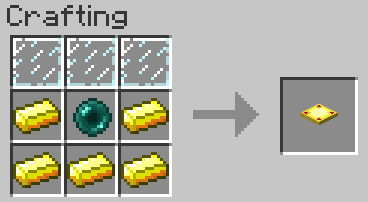
Inventory Manager
This block can connect to any inventory and pipe in 6 directions (beside the direction is connected from).
It has a 1 slot sized buffer for piped in items, accessible through a special inventory name: "buffer".
Recipe:
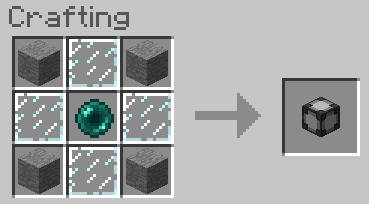
API
Ok so there is the current state of the API, if you have any remark please feel free to make a comment
APIs:
The mod adds an API in rom/apis named "invmanager" with a single method wrap() that will check the wrapped device type and map methods through a special wrapper.
See mods/invmanager-lua/invmanager.lua (created when you launch the game with the mod for the first time) for more details.
Methods:
[indent=1]size(direction)[/indent]
[indent=2]Direction is a valid direction or inventory name, see below for a list of directions[/indent]
[indent=1]read(direction, slot)[/indent]
[indent=2]Read the item at the specified slot, returning details about the stack in this slot. Slot IDs start from 1 to the inventory size.[/indent]
[indent=2]See below for the details on the details table.[/indent]
[indent=1]move(directionFrom, directionTo, slotFrom = nil, slotTo = nil, amount = STACK_SIZE)[/indent]
[indent=2]Moves a stack from the source inventory to a target inventory, with an optionally specified source and target slot, as well as a specified amount.[/indent]
[indent=2]If slotFrom is not provided, finds the first item in the inventory and moves it.[/indent]
[indent=2]If slotTo is not provided, finds the first available spot, if specified, try that spot first, then try the other slots.[/indent]
[indent=2]Returns the same details as read() with an additional property moved that contains the amount of items moved by the command.[/indent]
[indent=1]send(directionFrom, directionTo, slotFrom = 1, amount = STACK_SIZE)[/indent]
[indent=2]Send a stack into a pipe, allowing you to chose from which slot and how much to send.[/indent]
[indent=2]If slotFrom is not provided, finds the first item in the inventory and moves it.[/indent]
[indent=2]Returns the same details as read() with an additional property sent that contains the amount of items sent into the pipe.[/indent]
[indent=1]isInventory(direction)[/indent]
[indent=2]Is the block at the given direction is a valid inventory?[/indent]
[indent=1]isPipe(direction)[/indent]
[indent=2]Is the block at the given direction is a valid pipe?[/indent]
Types:
[indent=1]direction is either one of "player", "north", "east", "south", "west", "up", "down", "buffer". The plate only accepts "player" and "bottom", the inventory manager accept all but "player".[/indent]
[indent=1]item A description of a stack, returned by read()[/indent]
[indent=2]id The ID of the item[/indent]
[indent=2]name The raw name of the item in the MC engine[/indent]
[indent=2]display The actual translated name of the item (for display purposes)[/indent]
[indent=2]amount The amount of items in the stack[/indent]
[indent=2]damage The damage of the item[/indent]
Events:
invmanager_in
Called when an item is piped inside an inventory manager. Use move or send with "buffer" as a source to move the received items where you want. The buffer has only 1 slot and the block will refuse any item entering as long as it is not cleared. The event provides the item description, see the example below .
Examples:
Show the details of every items in the player inventory:
-- see the usage of "invmanager" instead of "peripheral"
-- trying to use peripheral will prevent the methods from returning correctly
-- unless you manually listen for the event "invmanager_task"
m = invmanager.wrap("right")
-- read the player inventory size, then iterate on every slots
size = m.size("player")
for i=0,size-1 do
-- read item in slot
item = m.read("player", i)
-- returns 0 if no item in the slot
if item ~= 0 then
print(item.amount, " ", item.display, " (", item.id, ") in slot ", i)
end
end
Move everything from the player inventory to the inventory under the plate:
m = invmanager.wrap("right")
-- read the player inventory size, then iterate on every slots
size = m.size("player")
for i=0,size-1 do
-- move stack in slot i
item = m.move("player", i, "down")
end
Equip the first armor found in the inventory under it:
m = peripheral.wrap("right")
size = m.size("down")
-- armor slots
armor = {
helmet = { slot = 39, equipped = false },
chestplate = { slot = 38, equipped = false },
leggings = { slot = 37, equipped = false },
boots = { slot = 36, equipped = false }
}
-- check if the player already has some equipment
for k, v in pairs(armor) do
if m.read("player", v.slot) ~= 0 then
armor[k]["equipped"] = true
end
end
-- try to equip the player
for i=0,size-1 do
item = m.read("down", i)
if item ~= 0 then
for k, v in pairs(armor) do
if v.equipped == false and string.sub(item.name, 0, 5 + string.len(k)) == "item."..k then
-- move armor from chest to player inventory
res = m.move("down", i, "player", v.slot)
print("Equipped "..item.display)
v.equipped = true
end
end
end
end
Route piped items (like a diamond pipe)
-- wrap manager
m = invmanager.wrap("back")
-- our sorting function, for testing purposes route everything up
function sort(item)
print("Routing "...item.display)
m.send("buffer", "up")
end
-- if the buffer already has items
if m.read("buffer", 1) ~= 0 then
m.send("buffer", "up")
end
-- wait for items to be piped in
while true do
local event, item = os.pullEvent("invmanager_in")
sort(item)
end
Download
Current version may not be 100% bug-free, even though it was tested by a panel of experts, I will not be responsible for lost items, chunk resets, explosions, lost limbs, demon spawning and other side effects that could occur while using the plates.
Current version (1.2.3): http://www.mediafire...ttvmqc10mf4ycfl
Previous versions:
Spoiler
1.0: http://www.mediafire...410wypvzm38aht8Please report any bug you find in this thread, and I'll make sure to fix them as soon as possible.
Changelog
1.2.3 - API rewritten, inventory manager finally added
1.0 - First version, adds the player manager
Source Code
The source code is now hosted on https://github.com/c...ventory-manager
Feel free to do pull-request, assign me issues, etc…