This is what I made from your explanation:
--[[ This like a shorthand notation for each color ]]
local a = colors.white
local b = colors.orange
local c = colors.magenta
local d = colors.lightBlue
local e = colors.yellow
local f = colors.lime
local g = colors.pink
--[[ This table will map each value to a table containing all colors that should be enabled ]]
local mapping = {
[0] = {a,b,c,d,e,f},
[1] = {b,c},
[2] = {a,b,d,e,g},
[3] = {a,b,c,d,g},
[4] = {b,c,f,g},
[5] = {a,c,d,f,g},
[6] = {a,c,d,e,f,g},
[7] = {a,b,c},
[8] = {a,b,c,d,e,f,g},
[9] = {a,b,c,d,f,g},
}
--[[ This function will output the right number on the bundled cable according to 'val', which is a figure (0-9). ]]
local function set( val )
local color = colors.combine( unpack( mapping[val] ) ) -- unpack the colors in the table for this figure and pass it to colors.combine
rs.setBundledOutput("back", color) -- output the combined color values on the bundled cable
end
It does use tables, but in a slightly different manner than you do. I don't see why you'd use rs.getBundledInput like pixletoast said, maybe to find it out manually. Anyway, this should work, make sure that a,b,c,e,… match the correct colors. Here is a drawing that shows where a,b,c,d,e,f and g should go according to my program:
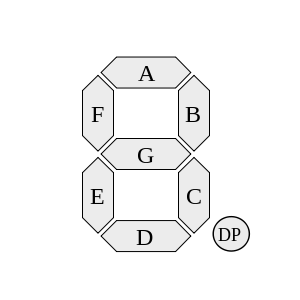
Edit: I added some comments into the code.
Edit2: fixed some syntax errors