Posted 03 February 2013 - 11:27 PM
Title:[Lua][Question] Table not printing correctly
Ok, so after staring/derping around with this code for about 4 hours I can't figure out why my table won't work.
Before I start here's the code:
Pastebin: http://pastebin.com/XgM0eSv5
Problem 1: Table won't print correclty. The third button(Test3) prints above Test1 and Test2. Obviously, it needs to be below them. D:
If you can't tell. The buttons have a blue background when not active.
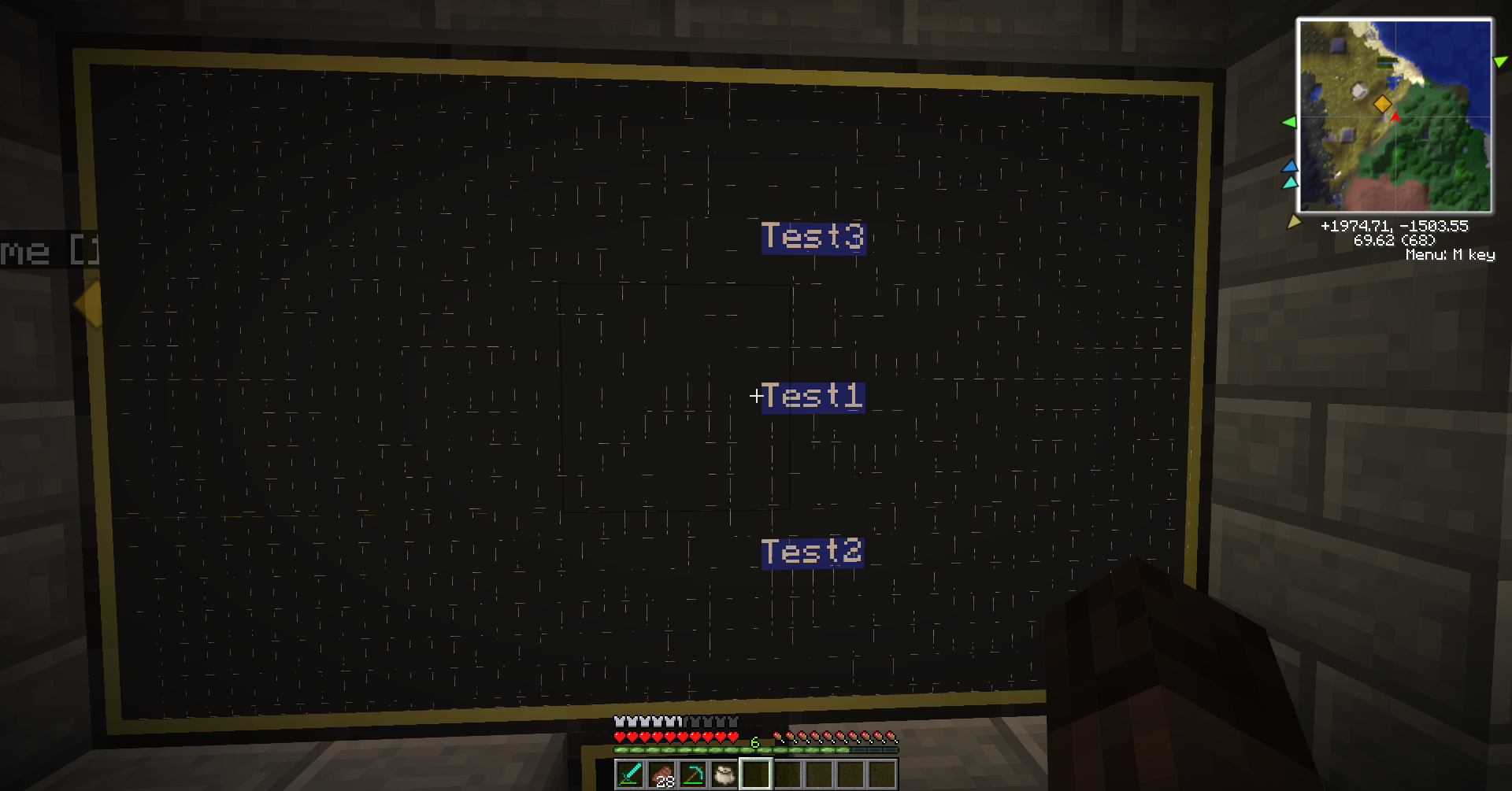
Problem 2: Right click (monitor_touch) on the 'Test3' button activates 'Test1' button. All other events work correctly so I don't understand how it got messed up.
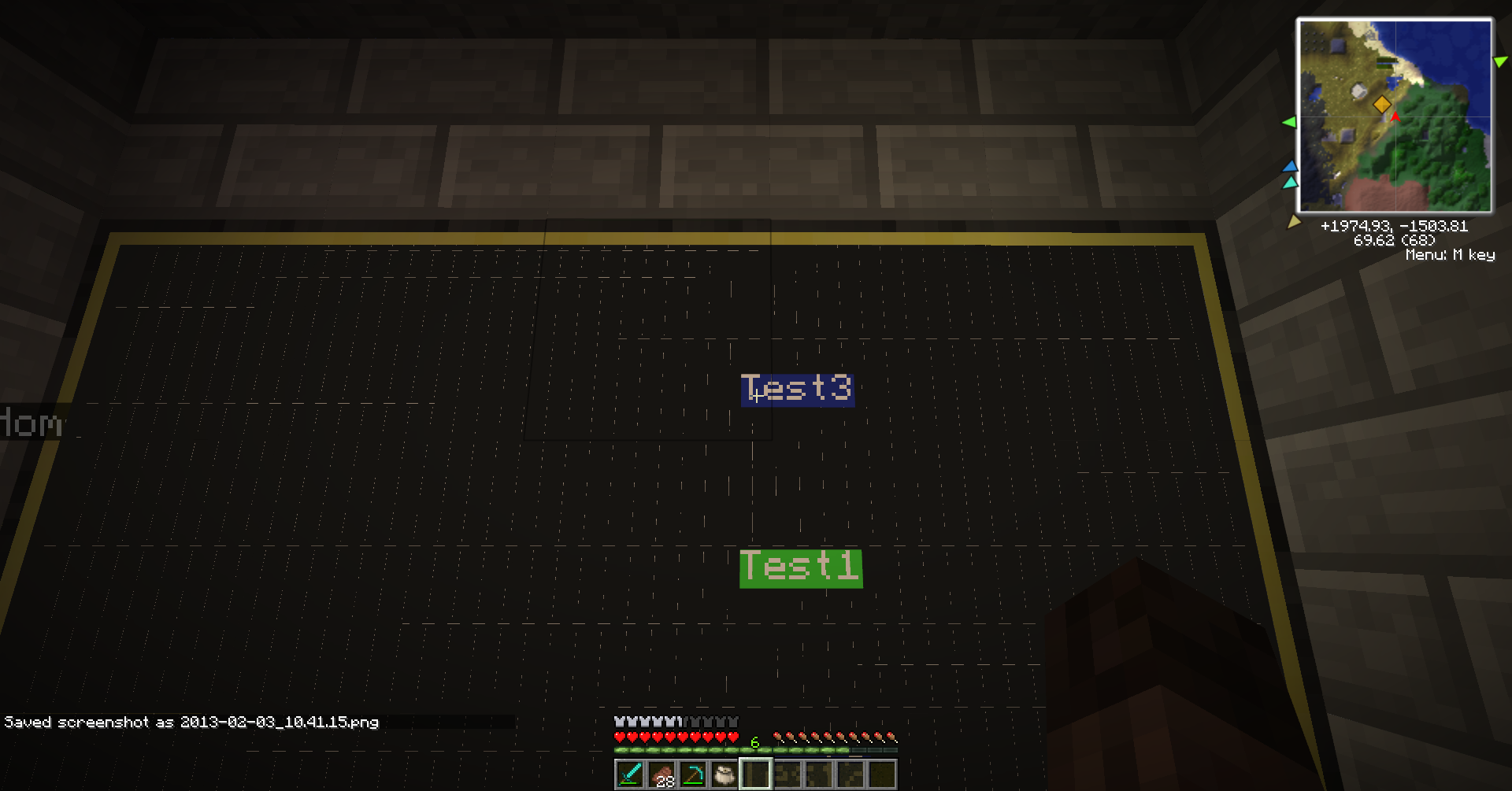
So, in conclusion:
Wat?
Ok, so after staring/derping around with this code for about 4 hours I can't figure out why my table won't work.
Before I start here's the code:
Spoiler
m = peripheral.wrap("top")
--Creates table 'menu'
local menu = {}
--Function to define 'menu' options
--'name' = name of button
--'active' determines background color
function setTable(name, xmin, xmax, ymin, ymax)
menu[name] = {}
menu[name]["active"] = false
menu[name]["xmin"] = xmin
menu[name]["xmax"] = xmax
menu[name]["ymin"] = ymin
menu[name]["ymax"] = ymax
end
--Function to define table information.
function fillTable()
setTable("Test1", 30, 40, 5,10)
setTable("Test2", 30, 40, 15, 20)
setTable("Test3", 30, 40, 25, 30)
end
--Function to fill the desired space
function fill(x,y,color,text)
m.setBackgroundColor(color)
m.setCursorPos(x,y)
m.write(text)
m.setBackgroundColor(colors.black)
end
--Function to change button background
--from one color to another
function bColor()
local y = 5
local currColor
for name,data in pairs(menu) do
local on = data["active"]
--If then for background color
if on == true then
currColor = colors.lime
else
currColor = colors.blue
end
fill(10,y,currColor,name)
y = y + 5
end
end
--Function to change 'active' value if
--a right click is confirmed
function check(x,y)
for name, data in pairs(menu) do
if (y>=data["ymin"] and y<=data["ymax"]) then
if (x>=data["xmin"] and x<=data["xmax"]) then
data["active"] = not data["active"]
print(name)
end --closes 'x' if
end --closes 'y' if
end --closes 'for' loop
end --ends function
--On to the juicy stuff!
fillTable()
while true do
m.clear()
bColor()
local event,side,x,y = os.pullEvent("monitor_touch")
print(x..":"..y)
check(x,y)
sleep(.1)
end
Problem 1: Table won't print correclty. The third button(Test3) prints above Test1 and Test2. Obviously, it needs to be below them. D:
If you can't tell. The buttons have a blue background when not active.
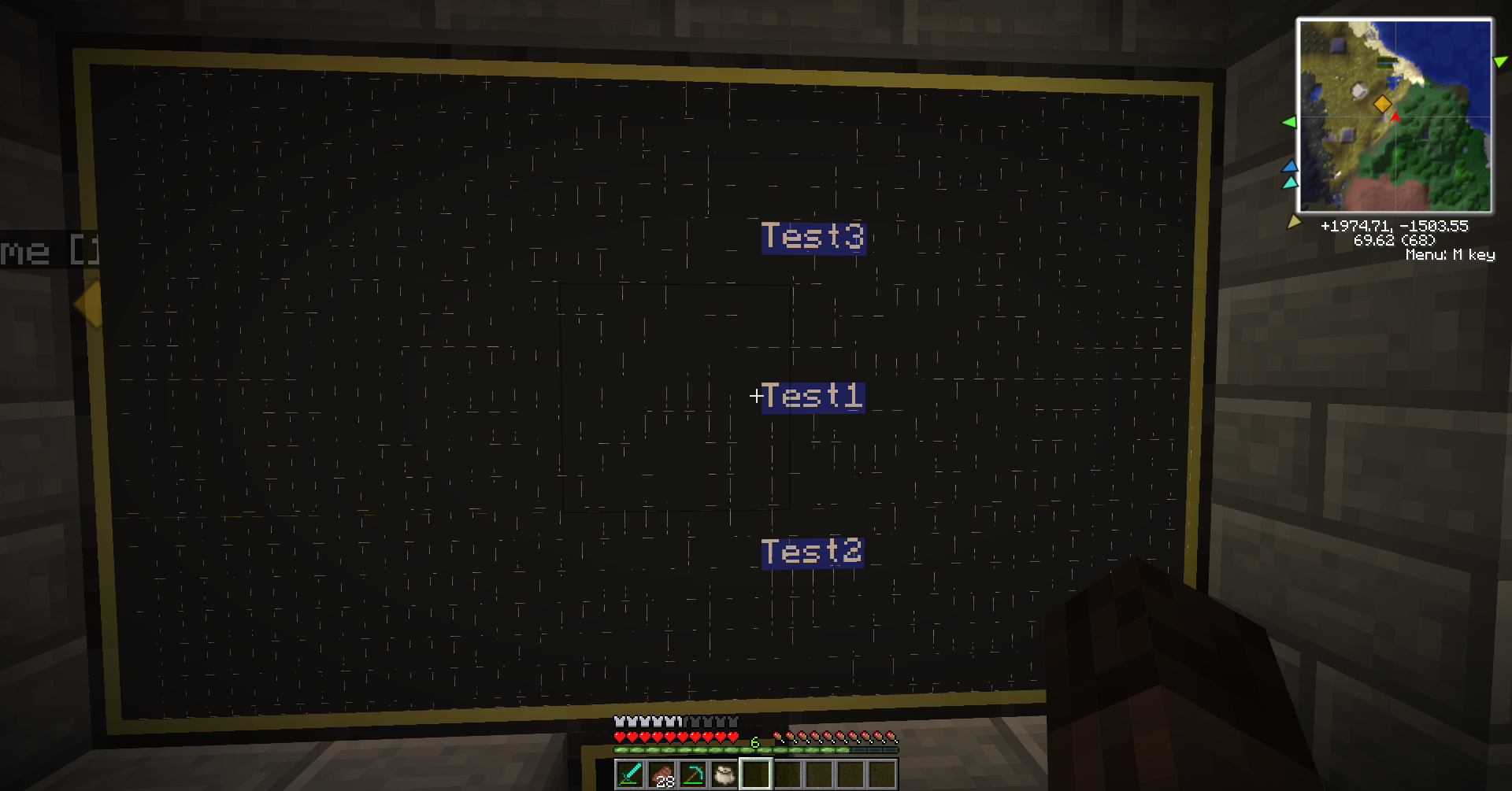
Problem 2: Right click (monitor_touch) on the 'Test3' button activates 'Test1' button. All other events work correctly so I don't understand how it got messed up.
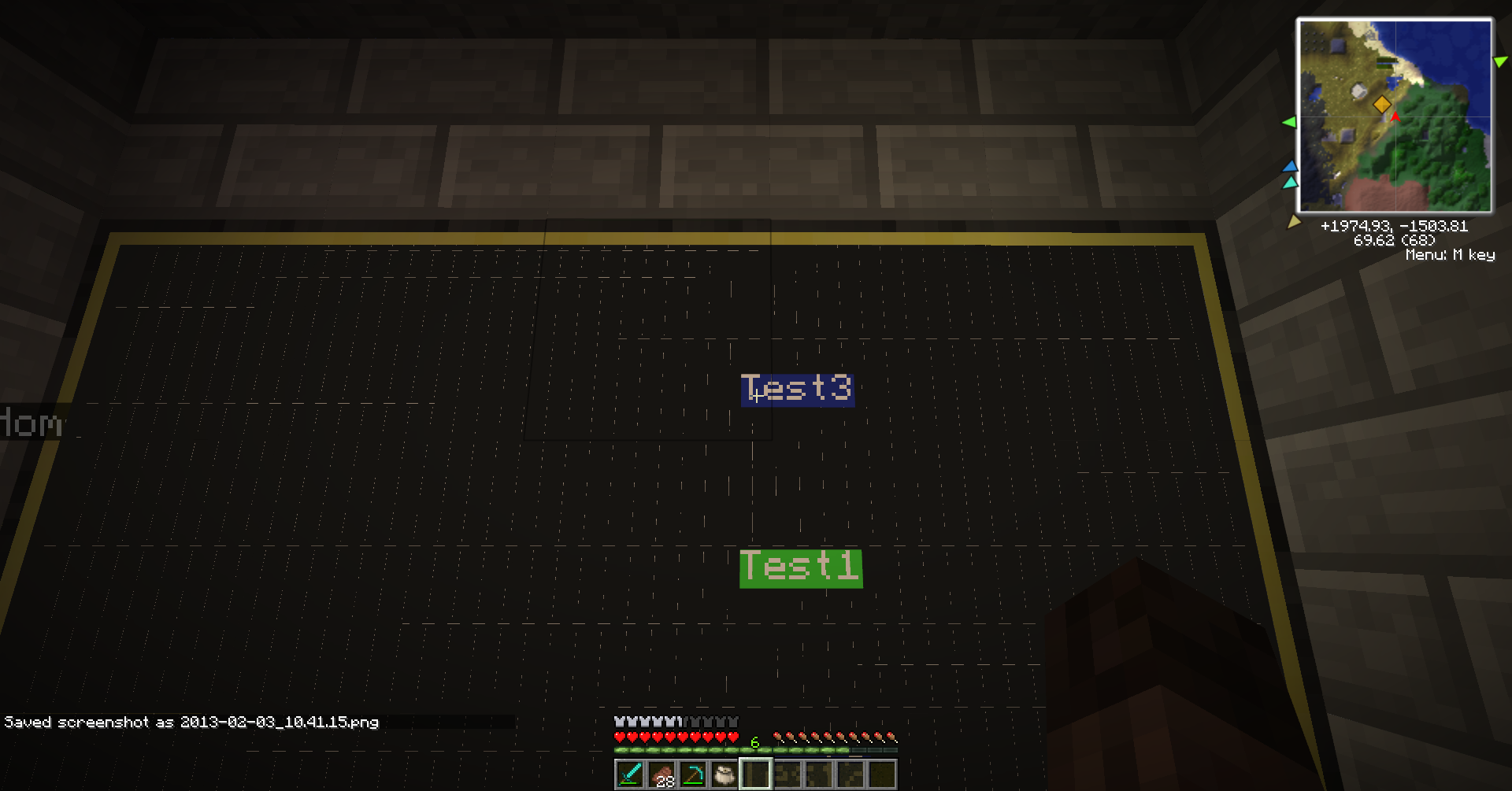
So, in conclusion:
Wat?