This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
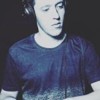
How to create a lua-compatible site
Started by FuuuAInfiniteLoop(F.A.I.L), 06 February 2013 - 07:09 AMPosted 06 February 2013 - 08:09 AM
I want to create a webpage (000webhost) that returns a table for a program but i dont know how to make it
Posted 06 February 2013 - 08:29 AM
It depends on what you want to achieve. But the best (well, most typical) way to achieve anything of this sort is using PHP (or some other server side scripting language). Anyway, my is to go with PHP. You can easily search online for some good and easy tutorials to learn it. Then you basically just have to print the text you want to receive in CC on the screen. If you want it to return the same table at all times, you can just paste the table into a file and fetch that through http.
If you want to construct the table from a database, I suggest learning MySQL as well.
If you want to construct the table from a database, I suggest learning MySQL as well.
Posted 06 February 2013 - 11:57 AM
If you simply want to load a static table from a webserver however you could also use pastebin and the pastebin api. If the table should be dynamic you should use PHP or Pearl. However I suggest using PHP.
Posted 06 February 2013 - 12:54 PM
I would suggest first looking at the textutils API, specifically the functions "serialize" and "unserialize"
These commands will let you convert a table to text and back.
The most plain, bare-bones, simple way to do it is to serialize your table, and copy/paste the output into a file that you upload to your web host.
You can then use the http API to retrieve that page and give the contents to unserialize. Now you have your table from the website!
If this is for your own uses, this might be enough - as long as you don't mind creating, editing, and uploading such files to the website.
If you want either
A ) to store the table from lua, or
b ) allow others to create this data
then you will need a more dynamic page. The PHP suggestion from Orwell and xuma202 are more along those lines.
(Personally I use TCL on the server side - but PHP is by far the most common pre-processor out there, and there is a ton of resources available to help you out)
If you build a big table in Lua, using lots of variable types and sub-tables and different indexes (both numbers and strings) and then toss it into textutils.serialize() - study the output, as this will be what you need to recreate to get data from a database into a Lua table directly.
It's a pretty straight forward format.
{[1]="one",[2]="two",["three"]="snarf!",}
If that was in a file called "mytable.txt" that you upload to the website, it will probably have a full URL like "ht tp://www.example.com/~user/mytable.txt"
Some example code to read in the above file on the website into a table named "tTable"
These commands will let you convert a table to text and back.
The most plain, bare-bones, simple way to do it is to serialize your table, and copy/paste the output into a file that you upload to your web host.
You can then use the http API to retrieve that page and give the contents to unserialize. Now you have your table from the website!
If this is for your own uses, this might be enough - as long as you don't mind creating, editing, and uploading such files to the website.
If you want either
A ) to store the table from lua, or
b ) allow others to create this data
then you will need a more dynamic page. The PHP suggestion from Orwell and xuma202 are more along those lines.
(Personally I use TCL on the server side - but PHP is by far the most common pre-processor out there, and there is a ton of resources available to help you out)
If you build a big table in Lua, using lots of variable types and sub-tables and different indexes (both numbers and strings) and then toss it into textutils.serialize() - study the output, as this will be what you need to recreate to get data from a database into a Lua table directly.
It's a pretty straight forward format.
{[1]="one",[2]="two",["three"]="snarf!",}
If that was in a file called "mytable.txt" that you upload to the website, it will probably have a full URL like "ht tp://www.example.com/~user/mytable.txt"
Some example code to read in the above file on the website into a table named "tTable"
local sData = http.get("http://www.example.com/~user/mytable.txt")
local sText = sData.readLine()
sData.close()
local tTable = {}
tTable = textutils.unserialize(sText)
print(tTable["three"])
snarf!
Posted 06 February 2013 - 01:05 PM
The thing that i want to do is when you request website.com/wordlist it return the table(alredy made) but if you put data(ex: blabla = bla2") in website.com/add it can add that value to the table in website.com/wordlist
Posted 06 February 2013 - 01:56 PM
The thing that i want to do is when you request website.com/wordlist it return the table(alredy made) but if you put data(ex: blabla = bla2") in website.com/add it can add that value to the table in website.com/wordlist
definitely PHP then
any sort of dynamic webpage is often best done by creating a wordlist.php file
it would end up being something like website.com/wordlist.php?blabla=bla2
Posted 06 February 2013 - 02:27 PM
can you give me a code for doing that because i dont know PHP, maybe website.com/wordlist is a file and php read it and then adds the line but how????? it can be client-side and the send it to the server, i will work on thatThe thing that i want to do is when you request website.com/wordlist it return the table(alredy made) but if you put data(ex: blabla = bla2") in website.com/add it can add that value to the table in website.com/wordlist
definitely PHP then
any sort of dynamic webpage is often best done by creating a wordlist.php file
it would end up being something like website.com/wordlist.php?blabla=bla2
Posted 06 February 2013 - 03:49 PM
idk PHP, but i know what it's used for and how to send arguments to it through a URL
Posted 06 February 2013 - 09:16 PM
You have to somehow save the table. sure you could go with some PHP and a text file but using a mySQL DB or any other DB (though mySQL is used most often) is better
Posted 07 February 2013 - 08:54 AM
so last night, i learned PHP for my own project, and this is a simplistic example
lua:
lua:
function getData(index)
return http.get("www.yoururl.com/getdata.php?index=" .. textutils.urlEncode(tostring(index)))
end
function sendData(index,value)
return http.post("www.yoururl.com/senddata.php?index=" .. textutils.urlEncode(tostring(index)) .. "&value=" .. textutils.urlEncode(textutils.serialize(value)))
end
getdata.php:
<?php
function getdata($index) {
$connect = @mysql_connect($host,$username,$password);
if (!$connect) die();
elseif (!@mysql_select_db($database,$connect)) {
@mysql_close($connect);
die();
}
$result = @mysql_query("SELECT * FROM yourtable WHERE index='$index'");
@mysql_close($connect);
if (!$result) die();
die($result[2]);
}
if isset($_GET["index"]) getdata($_GET["index"]);
?>
senddata.php:
<?php
function senddata($index,$data) {
$connect = @mysql_connect($host,$username,$password);
if (!$connect) die();
elseif (!@mysql_select_db($database,$connect)) {
@mysql_close($connect);
die();
}
$result = @mysql_query("INSERT * INTO yourtable (index,value) VALUES ('$index','$value')");
@mysql_close($connect);
if (!$result) die();
die("success");
}
if (isset($_GET["index"]) && isset($_GET["value"])) getdata($_GET["index"],$_GET["value"]);
?>
Posted 07 February 2013 - 09:02 AM
thanks for the code, but i need to give the whole table (this is for my ai program so the table is the wordlist) and the tables that need to been set up and a tutorial for setting the up in 000webhostso last night, i learned PHP for my own project, and this is a simplistic example
lua:getdata.php:function getData(index) return http.get("www.yoururl.com/getdata.php?index=" .. textutils.urlEncode(tostring(index))) end function sendData(index,value) return http.post("www.yoururl.com/senddata.php?index=" .. textutils.urlEncode(tostring(index)) .. "&value=" .. textutils.urlEncode(textutils.serialize(value))) end
senddata.php:<?php function getdata($index) { $connect = @mysql_connect($host,$username,$password); if (!$connect) die(); elseif (!@mysql_select_db($database,$connect)) { @mysql_close($connect); die(); } $result = @mysql_query("SELECT * FROM yourtable WHERE index='$index'"); @mysql_close($connect); if (!$result) die(); die($result[2]); } if isset($_GET["index"]) getdata($_GET["index"]); ?>
<?php function senddata($index,$data) { $connect = @mysql_connect($host,$username,$password); if (!$connect) die(); elseif (!@mysql_select_db($database,$connect)) { @mysql_close($connect); die(); } $result = @mysql_query("INSERT * INTO yourtable (index,value) VALUES ('$index','$value')"); @mysql_close($connect); if (!$result) die(); die("success"); } if (isset($_GET["index"]) && isset($_GET["value"])) getdata($_GET["index"],$_GET["value"]); ?>
Posted 07 February 2013 - 10:02 AM
Let me Google that for you..thanks for the code, but i need to give the whole table (this is for my ai program so the table is the wordlist) and the tables that need to been set up and a tutorial for setting the up in 000webhost* snip *
You need to show at least some effort at finding stuff out yourself..