Posted 08 February 2013 - 09:46 AM
Ok, so first of all this isn't my code. I used this to try and get more familiar with manipulating strings.
The code was found here with all credit to the guy who made it: http://www.gammon.co.../forum/?id=7805
Pastebin: http://pastebin.com/xXquxtsL
Code:
Specifically I'm talking about these lines:
I've looked through the lua libraries and I still don't understand why it works.
Help? D:
EDIT: In case anyone was wondering what this did here's a picture of it.
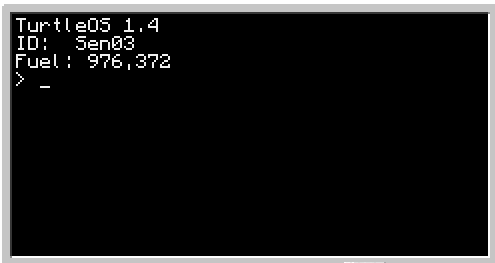
The code was found here with all credit to the guy who made it: http://www.gammon.co.../forum/?id=7805
Pastebin: http://pastebin.com/xXquxtsL
Code:
Spoiler
fuel = turtle.getFuelLevel()
ID = os.getComputerLabel()
function commas (num)
assert (type (num) == "number" or
type (num) == "string")
local result = ""
-- split number into 3 parts, eg. -1234.545e22
-- sign = + or -
-- before = 1234
-- after = .545e22
local sign, before, after =
string.match (tostring (num), "^([%+%-]?)(%d*)(%.?.*)$")
-- pull out batches of 3 digits from the end, put a comma before them
while string.len (before) > 3 do
result = "," .. string.sub (before, -3, -1) .. result
before = string.sub (before, 1, -4) -- remove last 3 digits
end -- while
-- we want the original sign, any left-over digits, the comma part,
-- and the stuff after the decimal point, if any
return sign .. before .. result .. after
end -- function commas
print("ID: "..ID)
print("Fuel: "..commas(fuel))
Specifically I'm talking about these lines:
local sign, before, after =
string.match (tostring (num), "^([%+%-]?)(%d*)(%.?.*)$")
-- pull out batches of 3 digits from the end, put a comma before them
while string.len (before) > 3 do
result = "," .. string.sub (before, -3, -1) .. result
before = string.sub (before, 1, -4) -- remove last 3 digits
end -- while
I've looked through the lua libraries and I still don't understand why it works.
Help? D:
EDIT: In case anyone was wondering what this did here's a picture of it.
Spoiler
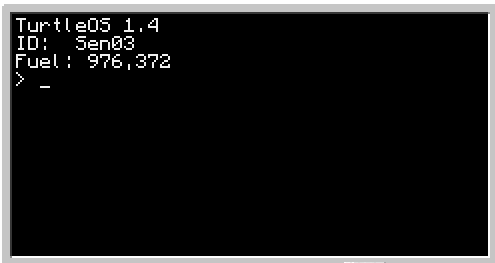