Background info: I play on ftb and I am making an automated bar. What they do is throw their payment in, and if the item is the right payment it sends a redstone pulse to the computer. I want the computer to count those pulses and wait for the person to say "strong" or "thick" and when they do, send the same amount of pulses to another machine that will send you the beer.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
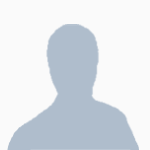
How to count redstone pulses?
Started by ComputerCrafter, 09 February 2013 - 05:09 AMPosted 09 February 2013 - 06:09 AM
What I want to do is have my computer count the number of redstone pulses it recieves, then send those same amount of pulses through another wire, but only when a certain word is said to the computer. How could this work?
Background info: I play on ftb and I am making an automated bar. What they do is throw their payment in, and if the item is the right payment it sends a redstone pulse to the computer. I want the computer to count those pulses and wait for the person to say "strong" or "thick" and when they do, send the same amount of pulses to another machine that will send you the beer.
Background info: I play on ftb and I am making an automated bar. What they do is throw their payment in, and if the item is the right payment it sends a redstone pulse to the computer. I want the computer to count those pulses and wait for the person to say "strong" or "thick" and when they do, send the same amount of pulses to another machine that will send you the beer.
Posted 09 February 2013 - 06:19 AM
This should work, but it's untested.
local pulses = 0
while true do
os.pullEvent('redstone') -- stop until a redstone input has changed
if rs.getInput('left') then -- check the left side for pulses
pulses = pulses + 1 -- add to pulses
rs.setOutput('right', true) -- send the pulse through the right side
sleep(0.1)
rs.setOutput('right', false)
term.clear()
term.setCursorPos(1,1)
term.write(pulses) -- displays the pulse count - you can display the data however you want.
end
end
Posted 09 February 2013 - 06:26 AM
Hmm… It looks right. But the problem is, it looks like this code only sends one pulse through the side. Although this is helpful and almost there, I need it to send the amount of pulses recieved. What about:
Please correct me if I am wrong, I know lots of javascript so it interferes with lua :P/>
This way it checks how many pulses it gets, and increases i by one every time the loop has ran, and when i is larger than pulses, then stop the loop.
Please correct me if I am wrong, I know lots of javascript so it interferes with lua :P/>
for(i = 0; i > pulses; i++) do
rs.setOutput('right', true)
sleep(0.1)
rs.setOutput('right', false)
end
This way it checks how many pulses it gets, and increases i by one every time the loop has ran, and when i is larger than pulses, then stop the loop.
Posted 09 February 2013 - 06:31 AM
for(i = 0; i > pulses; i++) do rs.setOutput('right', true) sleep(0.1) rs.setOutput('right', false) end
Just forget using the FOR statement like that. Lua's FOR is entirely different, like
for i = 1, 5 do ... end
And unfortunately, there is no ++ operator in Lua.
So what do you want? A computer that's waiting for both user input (read) and counting redstone pulses?
Posted 09 February 2013 - 06:31 AM
Yep. Sorry about that :P/> It waits for both of the inputs and then it sends off the signals depending on how many it recieved.
EDIT: I can post the code I already have if you want.
EDIT: I can post the code I already have if you want.
Posted 09 February 2013 - 06:35 AM
Ah, I think I see what you're trying to do. You want it to count the pulses, then send them on a certain keyword. A little less simple.
Was rather lazy with this one, but there you go. It counts pulses, then sends them when you type "send". Would probably be easier if you sent them on a keypress or mouse click rather than waiting for an input from read().
local pulses = 0
function getPulses()
while true do
os.pullEvent 'redstone'
if rs.getInput 'left' then
pulses = pulses + 1
repeat
os.pullEvent 'redstone'
until not rs.getInput 'left'
pulses = 0
end
end
end
function waitForKeyword()
while true do
local input = read()
if input == 'send' then
for i=1, pulses do
rs.setInput('right', true)
sleep(0.1)
rs.setInput('right', false)
end
end
end
end
parallel.waitForAny(waitForKeyword, getPulses)
Was rather lazy with this one, but there you go. It counts pulses, then sends them when you type "send". Would probably be easier if you sent them on a keypress or mouse click rather than waiting for an input from read().
Posted 09 February 2013 - 06:38 AM
I'm wondering, could I do this:
local pulses = 0
function getPulses()
while true do
os.pullEvent 'redstone'
if rs.getInput 'left' then
pulses = pulses + 1
repeat
os.pullEvent 'redstone'
until not rs.getInput 'left'
pulses = 0
end
end
end
function waitForKeyword()
while true do
local input = read()
if input == 'strong' then
for i=1, pulses do
rs.setInput('right', true)
sleep(0.1)
rs.setInput('right', false)
elseif input == 'thick' then
for i=1, pulses do
rs.setInput('left', true)
sleep(0.1)
rs.setInput('left', false)
end
end
end
end
parallel.waitForAny(waitForKeyword, getPulses)
Posted 09 February 2013 - 06:38 AM
Try this:
(Yes, I'm using parallel API, that's not a big deal)
EDIT: Sorry I'm late :D/>
(Yes, I'm using parallel API, that's not a big deal)
local pulsesReceived
local side = "left"
local beerType = ""
parallel.waitForAny(function()
local gotType = false
while not gotType do
if #beerType > 0 then print("We don't sell " .. beerType) end
print("What kind of beer do you want?")
beerType = read()
gotType = (beerType == "strong" or beerType == "thick")
end
end, function()
while true do
os.pullEvent("redstone")
if rs.getInput(side) then pulsesReceived = pulsesReceived + 1 end
end
end)
EDIT: Sorry I'm late :D/>
Posted 09 February 2013 - 06:42 AM
local pulses = 0 function getPulses() while true do os.pullEvent 'redstone' if rs.getInput 'left' then pulses = pulses + 1 repeat os.pullEvent 'redstone' until not rs.getInput 'left' pulses = 0 end end end function waitForKeyword() while true do local input = read() if input == 'strong' then for i=1, pulses do rs.setInput('right', true) sleep(0.1) rs.setInput('right', false) elseif input == 'thick' then for i=1, pulses do rs.setInput('left', true) sleep(0.1) rs.setInput('left', false) end end end end parallel.waitForAny(waitForKeyword, getPulses)
Yes, but you would never know the "beer type". Declare "input" right outside the functions. Like…
local pulses = 0
local input = ""
function getPulses()
while true do
os.pullEvent 'redstone'
if rs.getInput 'left' then
pulses = pulses + 1
repeat
os.pullEvent 'redstone'
until not rs.getInput 'left'
pulses = 0
end
end
end
function waitForKeyword()
while true do
input = read()
if input == 'strong' then
for i=1, pulses do
rs.setInput('right', true)
sleep(0.1)
rs.setInput('right', false)
elseif input == 'thick' then
for i=1, pulses do
rs.setInput('left', true)
sleep(0.1)
rs.setInput('left', false)
end
end
end
end
parallel.waitForAny(waitForKeyword, getPulses)
Posted 09 February 2013 - 06:43 AM
Hmm. The problem with your code is that you are only counting the pulses, not sending them back… Am I correct? If not, I'm gonna go study lua and computercraft language for 2 hours.
Posted 09 February 2013 - 06:44 AM
Hmm. The problem with your code is that you are only counting the pulses, not sending them back… Am I correct? If not, I'm gonna go study lua and computercraft language for 2 hours.
Of course, but I'm sure you can handle writin that on your own :)/>
Posted 09 February 2013 - 06:46 AM
Isnt that what I'm doing right here though? (I will bold it):
Edit: Bold didnt work. It is red.
Edit Edit: Neither did red. It is the one that has html code on it.
Edit Edit Edit: Not html, nevermind. You know what I mean.
local pulses = 0
function getPulses()
while true do
os.pullEvent 'redstone'
if rs.getInput 'left' then
pulses = pulses + 1
repeat
os.pullEvent 'redstone'
until not rs.getInput 'left'
pulses = 0
end
end
end
function waitForKeyword()
while true do
[color=#ff0000]local input = read()[/color]
if input == 'strong' then
for i=1, pulses do
rs.setInput('right', true)
sleep(0.1)
rs.setInput('right', false)
elseif input == 'thick' then
for i=1, pulses do
rs.setInput('left', true)
sleep(0.1)
rs.setInput('left', false)
end
end
end
end
parallel.waitForAny(waitForKeyword, getPulses)
Edit: Bold didnt work. It is red.
Edit Edit: Neither did red. It is the one that has html code on it.
Edit Edit Edit: Not html, nevermind. You know what I mean.
Posted 09 February 2013 - 06:48 AM
Yes, but you're declaring it INSIDE the function. This means when the function returns, input will be garbage-collected. It's like declaring "pulses" inside getPulses.
Posted 09 February 2013 - 06:52 AM
local pulses = 0
local input = read()
function getPulses()
while true do
os.pullEvent 'redstone'
if rs.getInput 'left' then
pulses = pulses + 1
repeat
os.pullEvent 'redstone'
until not rs.getInput 'left'
pulses = 0
end
end
end
function waitForKeyword()
while true do
if input == 'strong' then
print("Thank you for your payment! Your beer(s) will be right out!")
for i=1, pulses do
rs.setInput('right', true)
sleep(0.1)
rs.setInput('right', false)
elseif input == 'thick' then
print("Thank you for your payment! Your beer(s) will be right out!")
for i=1, pulses do
rs.setInput('left', true)
sleep(0.1)
rs.setInput('left', false)
end
end
end
end
parallel.waitForAny(waitForKeyword, getPulses)
Would this work? It is hard enough to build the machine that gives you the beer, but all this fuss with the computers is taking up a bit of time xD
EDIT: Dont forget the nice thank yous from the computer :)/>
Posted 09 February 2013 - 06:55 AM
Yes, this would, but if the costumer is drunk and can't type in "strong" or "thick"… You know what I mean. So declare "input" right after declaring "pulses", then read() it in waitForKeyword until it's something like "strong" or "thick".
Posted 09 February 2013 - 07:10 AM
Ok. Thanks for your help :)/> Now I will spend the rest of my time thinking why virgin would open up an airline called virgin america… <<Just saw an ad for it :/