local i = 0
local r = i * 10
redstone.setOutput("bottom",true)
while true do
sleep(0)
while (redstone.getInput("top")) do
if i < 9 then
sleep(r)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
if i > 8 then
sleep(80)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
end
end
end
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
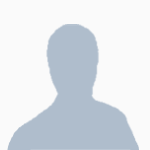
Help with code | Incrementing by one
Started by zBeeHamZ, 25 February 2013 - 09:19 AMPosted 25 February 2013 - 10:19 AM
I'm attempting to make a piston door open up in the floor, but every time you use it, you have to wait 10 more seconds for it to open. I want the increase to cap at 1 minute, 20 seconds (80 seconds). For some reason i won't increase by 1.
Posted 26 February 2013 - 07:03 AM
Split into new topic.
Posted 26 February 2013 - 08:20 AM
The code you've posted shouldn't even start because there are not enough 'end's to close all sections opened using 'do's and 'then's. Do you get any errors or do you only see that it doesn't work?
Posted 26 February 2013 - 10:05 AM
Why do you have two loops? The first loop only contains sleep(0) which is pretty worthless in my opinion. If you want it, just put sleep(0) in the second while loop. Also when the redstone signal is cut, the while loop breaks and will return to the first while loop. After this you will most likely get an error for too long without yielding. Thus exiting your program and setting i to 0. I'm pretty sure this is your problem. If anyone wants to correct me feel free :)/>
So what should the code look like?
So what should the code look like?
local i = 0
local r = i * 10
redstone.setOutput("bottom",true)
while (redstone.getInput("top")) do
sleep(0)
if i < 9 then
sleep(r)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
elseif i > 8 then
sleep(80)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
end
end
Posted 26 February 2013 - 10:08 AM
-snip-
As I've mentioned before, the first IF block has no end, but yes, the code should look like that.
(Maybe elseif instead of the second if?)
Posted 26 February 2013 - 10:10 AM
Yeah, that would be cleaner.-snip-
As I've mentioned before, the first IF block has no end, but yes, the code should look like that.
(Maybe elseif instead of the second if?)
EDIT: Actually that could be the reason i was not incremental.
I can see why you would want another while loop. If you desperately need one, feel free to add one. Just make sure to end the loop like so:
while true do
--some stuff
end
Posted 26 February 2013 - 12:17 PM
Try this?
Uncommented/compressed:
local seconds = 0
while true do
-- stop until we get a signal from the top
while not rs.getOutput('top') do
os.pullEvent('redstone')
end
-- sleep the current amount of time in seconds
sleep(seconds)
-- open the door
rs.setOutput('bottom', true)
sleep(3)
rs.setOutput('bottom', false)
-- increment seconds, only if it is less than 80.
seconds = seconds + (seconds < 80 and 10 or 0)
-- stop until we have no input from the top
-- just to prevent bugginess.
while rs.getOutput('top') do
os.pullEvent('redstone')
end
end
Uncommented/compressed:
local seconds = 0
while true do
while not rs.getOutput('top') do
os.pullEvent('redstone')
end
sleep(seconds)
rs.setOutput('bottom', true)
sleep(3)
rs.setOutput('bottom', false)
seconds = seconds + (seconds < 80 and 10 or 0)
while rs.getOutput('top') do
os.pullEvent('redstone')
end
end
Posted 26 February 2013 - 01:57 PM
none of these are working for me. it still doesn't have an increasing delay on it. here's the code in it's current state:
If needed, I can post pictures of how the computer and piston are set up
local i = 0
local r = i * 10
redstone.setOutput("bottom",true)
while true do
sleep(0)
while (redstone.getInput("top")) do
if i < 9 then
sleep(r)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
elseif i > 8 then
sleep(80)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
end
end
end
If needed, I can post pictures of how the computer and piston are set up
Posted 26 February 2013 - 01:59 PM
This line:
Also, calling sleep(0) over and over is a waste of CPU.
Use os.pullEvent("redstone") instead to wait for a redstone signal to change.
local r = i * 10
doesn't mean that r is always i * 10. It just sets r to that. If i changes later, r will not change automatically.Also, calling sleep(0) over and over is a waste of CPU.
Use os.pullEvent("redstone") instead to wait for a redstone signal to change.
Posted 03 March 2013 - 05:36 AM
a great thanks to immibis for the fix here. I enjoy that you didn't write out the code for me, but instead told me some information and allowed me to solve it myself in that way. i just had to move the r variable into the while loop. the code now reads:
local i = 0
redstone.setOutput("bottom",true)
while true do
sleep(0)
while (redstone.getInput("top")) do
if i < 9 then
r = i * 10
sleep(r)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
i = i + 1
elseif i > 8 then
sleep(80)
redstone.setOutput("bottom",false)
sleep(3)
redstone.setOutput("bottom",true)
end
end
end
Posted 05 March 2013 - 05:46 AM
You might be better served by using the os timer event - my understanding is it's much more CPU friendly than sleep() and would allow you to basically run an event loop independently of your redstone functions.
Posted 05 March 2013 - 11:33 AM
Sleep uses a timer event…You might be better served by using the os timer event - my understanding is it's much more CPU friendly than sleep() and would allow you to basically run an event loop independently of your redstone functions.
Posted 05 March 2013 - 03:08 PM
@Lua God: I read in a couple of places on the boards here over the weekend that it was CPU inefficient. Thanks for dispelling that!
Posted 05 March 2013 - 03:11 PM
His name is immibis not Lua God…@Lua God: I read in a couple of places on the boards here over the weekend that it was CPU inefficient. Thanks for dispelling that!
and sleep is not event friendly, thats all. so if you have a whole heap of events in the queue waiting and you use sleep, you have just lost all those events. that is when you want to use timers so you can preserve your events.
Posted 05 March 2013 - 03:16 PM
Does it really matter what is better CPU wise? CC allows for an infinate amount of memory does it not?
Posted 05 March 2013 - 09:28 PM
Yes, but your world server might not. It doesn't take a lot of badly written programs before lag and CC computer performance become problems.