194 posts
Location
Spain
Posted 15 March 2013 - 07:48 AM
Hello,
With HTML, PHP and MySQL you can make a website where people can create accounts and then login to do something.
I know how to make a form in HTML and connect it to a MySQL database, so people can login in a web browser, but I want to implement this in ComputerCraft.
My questions are:
-How can I make a program in ComputerCraft where people can fill a HTML form ingame?
-Will this work with a standard HTML form or do I need to do something special?
-Can this be used to upload files to a server?
Thanks in advance.
42 posts
Posted 15 March 2013 - 08:20 AM
you won't be able to use an HTML form. instead, you'll have to prompt for a username and password, or whatever other information you need.
files are a little more difficult. if you're uploading text files only, you can use a POST request using http.post.
example php:
file_put_contents('somefile.txt', $_POST['file']);
edit:
firewolf's wiki page on
making a website might be of some use if you're targeting advanced computers/firewolf.
2088 posts
Location
South Africa
Posted 15 March 2013 - 08:26 AM
-How can I make a program in ComputerCraft where people can fill a HTML form ingame?
In-order for a user to be able to fill out forms, you would need to use php scripts.
It's fairly simple if you know http and php well.
I just finished the my login script for my ComputerCraft YouTube program and it looks something like this
CC Code: ( Used this as a test at first :P/> )
Spoiler
local serverURL = "http://yourserver.com"
term.clear() term.setCursorPos( 1, 1 )
write( " Name: " )
local name = read()
write( " Email: " )
local email = read()
write( "Username: " )
local user = read()
write( "Password: " )
local pass = read()
local res = http.post( serverURL .. "/register.php/",
"name=" .. textutils.urlEncode( name ) .. "&" ..
"email=" .. textutils.urlEncode( email ) .. "&" ..
"username=" .. textutils.urlEncode( user ) .. "&" ..
"password=" .. textutils.urlEncode( pass ) )
local info = res.readAll():match( '<INFO>(.-)</INFO>' ) -- get the text that was printed, to check if succesfullor failed etc.
res.close()
print( info )
PHP CodeSpoiler
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Untitled Document</title>
</head>
<?php
function email_is_valid($email) { // useful function to check if inputed email is valid
return preg_match('/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i',$email);
}
$salt = "41233465qwea13d189ere98413o21o32plk132"; // Random salt for hashing passwords
$username = trim($_POST["username"]); // trim is important, removes whitespaces etc .. http://php.net/manual/en/function.trim.php
$password = trim($_POST["password"]);
$password_hashed = $salt . md5(trim($_POST["password"]));
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$now = date("m/d/Y h:i:s a", time()); // The current time in month/day/year hour:minute:second pm/am format
if ( isset($username) and isset($password) and isset($name) and isset($email) ) { // checks to see if these variables were filled out using POST ( ie http.post for CC )
// Make some verifications of the inputted variables if needed
if ( strlen( $name ) > 25 ) {
echo '<INFO>Name too long.</INFO>';
return false;
} elseif ( strlen( $email ) > 0 and !email_is_valid($email) ) {
echo '<INFO>Invalid email address.</INFO>';
return false;
} elseif ( strlen( $username ) <= 4 ) {
echo '<INFO>Username too short.</INFO>';
return false;
} elseif ( strlen( $username ) >= 25 ) {
echo '<INFO>Username too long.</INFO>';
return false;
} elseif ( strlen( $password ) <= 4 ) {
echo '<INFO>Password too short.</INFO>';
return false;
}
// Code to do stuff the with names/emails/username/passwords
// to add to a mysql database or whatnot.
} else { // if no post was used, error!
echo "Invalid post. Requires some data.";
mysql_close( $con );
return false;
}
?>
<body>
</body>
</html>
-Will this work with a standard HTML form or do I need to do something special?
PHP
-Can this be used to upload files to a server?
Yes, you can upload files to a FTP. I currently use MySQL databases to store videos/accounts etc.
194 posts
Location
Spain
Posted 15 March 2013 - 08:34 AM
Thanks remiX, your answer helped me a lot. Didn't know http.post() works in that way.
42 posts
Posted 15 March 2013 - 08:38 AM
@remiX, your salt is done wrong. consider the following hypothetical scenario:
i've compromised your database and dumped your user table.
function strip_salt($hash) {
$salt = "41233465qwea13d189ere98413o21o32plk132";
return substr($hash, strlen($salt));
}
for($i = 0; $i < count($passwords); $i++) {
echo strip_salt($passwords[i])."
\n";
}
if i grab the user information from your database, i can just get the raw hash for each user's password.
edit: in the future, i'll use a PM or something. it didn't occur to me at the time.
7508 posts
Location
Australia
Posted 15 March 2013 - 08:41 AM
Thanks remiX, your answer helped me a lot. Didn't know http.post() works in that way.
One thing that remiX does that I advise you against is using the md5 function to secure the password. instead use something like
$password_hashed = hash("sha256", $salt . trim($_POST["password"]));
Also it is better (if you want) to use a random salt for each password (don't forget to remember it, so you can use it later to check passwords) so that the hashes for even passwords that are the same, are still different.
2088 posts
Location
South Africa
Posted 15 March 2013 - 08:41 AM
Thanks remiX, your answer helped me a lot. Didn't know http.post() works in that way.
It's amazing what you can find out :)/>
EDIT:
@remiX, your salt is done wrong. consider the following hypothetical scenario:
i've compromised your database and dumped your user table.
function strip_salt($hash) {
$salt = "41233465qwea13d189ere98413o21o32plk132";
return substr($hash, strlen($salt));
}
for($i = 0; $i < count($passwords); $i++) {
echo strip_salt($passwords[i])."
\n";
}
if i grab the user information from your database, i can just get the raw hash for each user's password.
edit: in the future, i'll use a PM or something. it didn't occur to me at the time.
I just gave a random salt for that one, and I'm not going to be using md5 either.
TOBIT gave me better advice as he said above which I have mostly incorporated.
194 posts
Location
Spain
Posted 15 March 2013 - 08:50 AM
One thing that remiX does that I advise you against is using the md5 function to secure the password. instead use something like
$password_hashed = hash("sha256", $salt . trim($_POST["password"]));
Also it is better (if you want) to use a random salt for each password (don't forget to remember it, so you can use it later to check passwords) so that the hashes for even passwords that are the same, are still different.
I will look into this. I don't want someone stealing users' passwords.
7508 posts
Location
Australia
Posted 15 March 2013 - 08:51 AM
I will look into this. I don't want someone stealing users' passwords.
Thats good :)/> because we definitely don't want another NDFJay experience.
42 posts
Posted 15 March 2013 - 08:54 AM
@remiX, what i mean is that your salt doesn't have any effect on the resulting hash. it's just appended to the beginning. for it to make a difference, you would need to hash both the salt and the password, together.
TOBIT is correct that you should use a different salt for each password– using something like the registration time, and also correct that md5 isn't particularly secure.
however, sha256 is not much better. sha256 and md5 are both cryptographic hashes, so they're meant to be very fast. they were never meant for storing passwords. it's better to use a function such as bcrypt which, though still using a cryptographic hash, will hash the password, with a salt, several times in a row.
7508 posts
Location
Australia
Posted 15 March 2013 - 09:00 AM
TOBIT is correct that you should use a different salt for each password– using something like the registration time, and also correct that md5 isn't particularly secure.
Why thank you sir, it is good to see we can agree on something :P/>
however, sha256 is not much better.
According to an article I read a few weeks? … maybe a month or two ago … NIST stated there have been no significant attack demonstrated on SHA-2 (even though they decided to do SHA-3) … According to NIST there have been successful attacks on MD5, SHA-0 and theoretical attacks on SHA-1 (which spawned the need for SHA-2)
42 posts
Posted 15 March 2013 - 09:08 AM
here, this will explain better than i can:
http://codahale.com/how-to-safely-store-a-password/i do wish it had hard data on the speed of generating sha256, but its points are valid regardless of the specifics.
2088 posts
Location
South Africa
Posted 15 March 2013 - 09:10 AM
@remiX, what i mean is that your salt doesn't have any effect on the resulting hash. it's just appended to the beginning. for it to make a difference, you would need to hash both the salt and the password, together.
TOBIT is correct that you should use a different salt for each password– using something like the registration time, and also correct that md5 isn't particularly secure.
however, sha256 is not much better. sha256 and md5 are both cryptographic hashes, so they're meant to be very fast. they were never meant for storing passwords. it's better to use a function such as bcrypt which, though still using a cryptographic hash, will hash the password, with a salt, several times in a row.
I see what you mean but the salt is saved on the PHP side and no one has access to it, so goodluck guessing a very long salt :P/>
I know TOBIT is correct, which is why I'm going to be doing what he suggested :)/>
7508 posts
Location
Australia
Posted 15 March 2013 - 09:14 AM
I see what you mean but the salt is saved on the PHP side and no one has access to it, so goodluck guessing a very long salt :P/>
Are you 100% sure no one has access to the php script? :P/> also if they see a common denominator to all the passwords (i.e. concat to the start instead of hashing it in) they would just removed it, there is no need to seeing the php file.
42 posts
Posted 15 March 2013 - 09:15 AM
if they see a common denominator to all the passwords (i.e. concat to the start instead of hashing it in) they would just removed it, there is no need to seeing the php file.
exactly.
2088 posts
Location
South Africa
Posted 15 March 2013 - 09:16 AM
I see what you mean but the salt is saved on the PHP side and no one has access to it, so goodluck guessing a very long salt :P/>
Are you 100% sure no one has access to the php script? :P/> also if they see a common denominator to all the passwords (i.e. concat to the start instead of hashing it in) they would just removed it, there is no need to seeing the php file.
They would need access to my database then -,-
And I don't use a single salt, like I said I did what you suggested to me :P/>
42 posts
Posted 15 March 2013 - 09:23 AM
They would need access to my database then -,-
if you trust that no one will be able to access your database, why bother with a hash? the whole point of protecting passwords is to keep them safe in the event of a breach.
7508 posts
Location
Australia
Posted 15 March 2013 - 09:27 AM
They would need access to my database then -,-
if you trust that no one will be able to access your database, why bother with a hash? the whole point of protecting passwords is to keep them safe in the event of a breach.
That being said, best method to protect your users is to try stop the attack from getting even close to your data. Never just rely on having a good hash. Protect
and obstruficate.
On a side note, a few weeks ago NeverCast and I had a bot trying to attack the CCTube server. It was interesting watching all its attempts and the ways it was trying to bypass our security.
2088 posts
Location
South Africa
Posted 15 March 2013 - 05:53 PM
They would need access to my database then -,-
if you trust that no one will be able to access your database, why bother with a hash? the whole point of protecting passwords is to keep them safe in the event of a breach.
True :P/>
194 posts
Location
Spain
Posted 16 March 2013 - 09:26 AM
On a side note, a few weeks ago NeverCast and I had a bot trying to attack the CCTube server. It was interesting watching all its attempts and the ways it was trying to bypass our security.
I'm going to make a test before coding the actual program. When I finish coding the test, can you try to hack the server to improve security?
This would be very helpful.
2088 posts
Location
South Africa
Posted 16 March 2013 - 09:34 AM
On a side note, a few weeks ago NeverCast and I had a bot trying to attack the CCTube server. It was interesting watching all its attempts and the ways it was trying to bypass our security.
I'm going to make a test before coding the actual program. When I finish coding the test, can you try to hack the server to improve security?
This would be very helpful.
When will you be done with your test code?
194 posts
Location
Spain
Posted 17 March 2013 - 05:01 AM
When will you be done with your test code?
I just finished my test code:
http://pastebin.com/LEZDqjDc
2088 posts
Location
South Africa
Posted 17 March 2013 - 08:44 AM
Well, it works :P/> Nice job
194 posts
Location
Spain
Posted 18 March 2013 - 01:02 AM
Well, it works :P/> Nice job
Thanks, you helped me a lot with your first post.
Lol, there are 899 users. I need to prevent this, maybe with a capcha.
Also all of the users are registered the 00-00-0000. But I think I know how to fix this.
EDIT: I couldn't fix the date :(/>
PS: Nice email: spam@spam.spam
2088 posts
Location
South Africa
Posted 18 March 2013 - 01:29 AM
Well, it works :P/>/> Nice job
Thanks, you helped me a lot with your first post.
Lol, there are 899 users. I need to prevent this, maybe with a capcha.
Also all of the users are registered the 00-00-0000. But I think I know how to fix this.
EDIT: I couldn't fix the date :(/>/>
PS: Nice email: spam@spam.spam
Lol o_O
Dat email…
To prevent spam like this, you can use the global $_SESSON variable
Test this code for example:
Spoiler
<?php
if(!isset($_SESSION)){ session_start(); }
$time_between_refresh = 5;
$last_entered = $_SESSION['now'] or 0;
if ($last_entered != 0 and time()-$last_entered < $time_between_refresh) {
echo 'Go away fowl demon!<br>';
$secs = $time_between_refresh-(time()-$last_entered);
echo 'You can come back in '.$secs.' seconds :)/>';
return false;
} else {
echo 'Hi :D/>';
$_SESSION['now'] = time();
return false;
}
?>
For the date, use the date() function to format the current time()
$now = date("m/d/Y h:i:s a", time());
echo 'Time: '.time().'<br>';
echo 'Formatted: '.$now;
Output:
Time: 1363523618
Formatted: 03/17/2013 01:33:38 pm
42 posts
Posted 18 March 2013 - 02:22 AM
To prevent spam like this, you can use the global $_SESSON variable
this won't work in CC. a quick script in lua could register a thousand accounts because CC doesn't support cookies. with that said, it's pretty trivial to make http requests with no cookie anyway.
2088 posts
Location
South Africa
Posted 18 March 2013 - 02:26 AM
To prevent spam like this, you can use the global $_SESSON variable
this won't work in CC. a quick script in lua could register a thousand accounts because CC doesn't support cookies. with that said, it's pretty trivial to make http requests with no cookie anyway.
Hmm, weird. I'm sure it worked for me when I tested it about a month ago.
EDIT: Ah, you're right.
The way I did it with my ComputerCraft YouTube program is that it saves the last time you upload a video or whatever into a mysql table and then checks from there if the time between uploads is valid.
42 posts
Posted 18 March 2013 - 02:47 AM
you might be able to implement an alternative to cookies by saving a file to the CC disk somewhere and sending its contents with each request, but that only solves the problem of logging in, and not the problem of abuse.
for the issue of nonsense signups, apply some more advanced email validation.
- email verification. send email to users, users must verify their email, or their account is deleted after e.g. a week.
- verify that the email's domain actually exists. no @spam.spam
- make sure the same name isn't being registered repeatedly from the same IP
2088 posts
Location
South Africa
Posted 18 March 2013 - 03:10 AM
you might be able to implement an alternative to cookies by saving a file to the CC disk somewhere and sending its contents with each request, but that only solves the problem of logging in, and not the problem of abuse.
for the issue of nonsense signups, apply some more advanced email validation.
- email verification. send email to users, users must verify their email, or their account is deleted after e.g. a week.
- verify that the email's domain actually exists. no @spam.spam
- make sure the same name isn't being registered repeatedly from the same IP
1. The thing is that most CC Users would get irritated by the fact that they need to verify their email.
2. Is there a php method of actually doing this? Or will you need to check itself using an array or something containing variables like gmail, hotmail, yahoo, webmail etc.
3. Yeah that can be done easily.
42 posts
Posted 18 March 2013 - 03:22 AM
- then don't require validation to start using the account unless there have been bulk registrations from that IP
- an array is unreliable because there's the possibility of a personal email address, or an unknown email.
- which reminds me, it's necessary to blacklist temporary emails like the emails from 10minutemail.com.
for verifying the existence of a domain, you could probably use a whois service that offers an API.
edit: i don't know for certain, but it might be good enough to ping a domain to see if it responds. there's probably a better way. i'll see what i can find.
2088 posts
Location
South Africa
Posted 18 March 2013 - 03:26 AM
- then don't require validation to start using the account unless there have been bulk registrations from that IP
- an array is unreliable because there's the possibility of a personal email address, or an unknown email.
- which reminds me, it's necessary to blacklist temporary emails like the emails from 10minutemail.com.
for verifying the existence of a domain, you could probably use a whois service that offers an API.
edit: i don't know for certain, but it might be good enough to ping a domain to see if it responds. there's probably a better way. i'll see what i can find.
1. That could work, but that's more complicated code.
2. Yeah true, didn't think of that. How would you go about this then?
I'm googling now if there is a way to see if an email is a valid one.
42 posts
Posted 18 March 2013 - 03:35 AM
that's actually
less complicated code. you just add a new column to the database table, with e.g. a 0 or a 1. tell the user they must verify within a week. if they haven't (i.e. the column is set to 0), delete the account.
the query might look like
DELETE FROM users WHERE verified=0 AND registration_date > CURDATE() - 7;
i'd forgotten, but php has this. there may not be any effective way to do this.
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
//valid
}
a better way of verifying an account might be to determine whether it's actually been in use since registration. the process of logging in would act as verification, and any accounts unused for more than a week are deleted.
2088 posts
Location
South Africa
Posted 18 March 2013 - 04:08 AM
that's actually
less complicated code. you just add a new column to the database table, with e.g. a 0 or a 1. tell the user they must verify within a week. if they haven't (i.e. the column is set to 0), delete the account.
the query might look like
DELETE FROM users WHERE verified=0 AND registration_date > GETDATE() - 7;
i'd forgotten, but php has this. there may not be any effective way to do this.
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
//valid
}
a better way of verifying an account might be to determine whether it's actually been in use since registration. the process of logging in would act as verification, and any accounts unused for more than a week are deleted.
When I said '1. That could work, but that's more complicated code.', it was for your '- then don't require validation to start using the account unless there have been bulk registrations from that IP'
You need to see if the IP already been registered a few times in the system and query the database to delete it.
194 posts
Location
Spain
Posted 18 March 2013 - 04:10 AM
And why not a CAPCHA? In a real webpage this can be done with reCAPCHA and in CC maybe random nfp files with text generated by the server.
For the date, use the date() function to format the current time()
$now = date("m/d/Y h:i:s a", time());
echo 'Time: '.time().'<br>';
echo 'Formatted: '.$now;
Output:
Time: 1363523618
Formatted: 03/17/2013 01:33:38 pm
What variable type do I need in the MySQL database for this?
42 posts
Posted 18 March 2013 - 04:24 AM
'- then don't require validation to start using the account unless there have been bulk registrations from that IP'
oh right.
SELECT ip,registration_time FROM users WHERE registration_date < CURTIME() - 300;
count the returned data, if more than e.g. 10, reject registration.
also, there was a typo in my SQL above; it should be
DELETE FROM users WHERE verified=0 AND registration_date > GETDATE() - 7;
And why not a CAPCHA? In a real webpage this can be done with reCAPCHA and in CC maybe random nfp files with text generated by the server.
For the date, use the date() function to format the current time()
$now = date("m/d/Y h:i:s a", time());
echo 'Time: '.time().'
';
echo 'Formatted: '.$now;
Output:
Time: 1363523618
Formatted: 03/17/2013 01:33:38 pm
What variable type do I need in the MySQL database for this?
reCAPTCHA might work but has gotten notoriously difficult for humans to solve. i'm not sure how you'd implement it, and you'd need an advanced computer.
>What variable type do I need in the MySQL database for this?
this won't work properly due to the lack of cookie support in CC
2088 posts
Location
South Africa
Posted 18 March 2013 - 04:48 AM
'- then don't require validation to start using the account unless there have been bulk registrations from that IP'
oh right.
SELECT ip,registration_time FROM users WHERE registration_date < CURTIME() - 300;
count the returned data, if more than e.g. 10, reject registration
Yeah,
if (mysql_num_rows($result) >= 10) {
// Deny
return false;
}
Could work.
reCAPTCHA might work but has gotten notoriously difficult for humans to solve. i'm not sure how you'd implement it, and you'd need an advanced computer.
>What variable type do I need in the MySQL database for this?
this won't work properly due to the lack of cookie support in CC
Hmm, yeah this won't work.
The way I have done it with storing the time in a php database is probably the best afaik
42 posts
Posted 18 March 2013 - 05:00 AM
The way I have done it with storing the time in a php database is probably the best afaik
yep, registration time can work for that.
so with your input, i've concluded that the following should keep the database relatively clean:
"
- check for bulk registration
- select recent registrations from same IP, prevent registrations if more than X registrations in Y minutes
- verify emails using filter_var($email, FILTER_VALIDATE_EMAIL)
- add a database column named something like 'verified', set to 0 by default, and set to 1 on first login
- delete accounts older than X where verified is 0
"
edit: stupid forum syntax highlighting. something has to be done about this
7508 posts
Location
Australia
Posted 18 March 2013 - 08:59 AM
Btw I was the one that spammed you with spam@spam.spam, was testing something… Your php always returns failed, even when it succeeds.
42 posts
Posted 18 March 2013 - 09:18 AM
Btw I was the one that spammed you with spam@spam.spam, was testing something… Your php always returns failed, even when it succeeds.
for registration? not for me
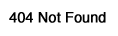
7508 posts
Location
Australia
Posted 18 March 2013 - 09:21 AM
Yeh for registration. Using his script the thing kept printing failed.
194 posts
Location
Spain
Posted 18 March 2013 - 09:34 AM
Yeh for registration. Using his script the thing kept printing failed.
It works for me :S
42 posts
Posted 18 March 2013 - 09:36 AM
oh, it's his regex.
info = res.readAll():match('<INFO>(.-)</INFO>')
should be
info = res.readAll():match('<INFO>(.+)</INFO>')
7508 posts
Location
Australia
Posted 18 March 2013 - 09:43 AM
ahh. i didn't even look at his pattern. I looked at the php query but didn't even think about the pattern :/ its just WAAAY too early to think.
2088 posts
Location
South Africa
Posted 18 March 2013 - 09:54 AM
Btw I was the one that spammed you with spam@spam.spam, was testing something… Your php always returns failed, even when it succeeds.
It worked for me.
Response for me when I tested it was 'User Created'.
But what you should do is that if it's purely only php code, remove all the HTML code and just echo 'User Created'. and do .readAll() and just print that.
I recently changed that for my code, I don't use <INFO>text</INFO> anymore :P/>
42 posts
Posted 18 March 2013 - 09:58 AM
Yeh for registration. Using his script the thing kept printing failed.
It works for me :S
huh, yeah it works for me too. i don't understand why, though
2088 posts
Location
South Africa
Posted 18 March 2013 - 10:02 AM
Yeh for registration. Using his script the thing kept printing failed.
It works for me :S
huh, yeah it works for me too. i don't understand why, though
why? (.-) works fine for this sort of thing. I have used it a lot.
42 posts
Posted 18 March 2013 - 10:14 AM
why? (.-) works fine for this sort of thing. I have used it a lot.
my initial reaction was "it shouldn't." i read through the PIL. looks like lua pattern matching is not regex.
i assumed .- was a typo for .+ but it turns out to be valid lua.
regex is the norm for pattern matching in programming, so to see a language with an alternative is very shocking.
194 posts
Location
Spain
Posted 18 March 2013 - 10:30 AM
But what you should do is that if it's purely only php code, remove all the HTML code and just echo 'User Created'. and do .readAll() and just print that.
I recently changed that for my code, I don't use <INFO>text</INFO> anymore :P/>
I didn't do that because in the first hosting I tried, it always put some code at the end of the file (I think it was a visit counter). But that server has an outdated PHP version that is not compatible with bcrypt, so I changed my server hoster, and now it doesn't write anything, so I will just echo the info.
42 posts
Posted 18 March 2013 - 10:34 AM
But that server has an outdated PHP version that is not compatible with bcrypt, so I changed my server hoster, and now it doesn't write anything, so I will just echo the info.
+1 for using bcrypt.
out of curiosity, what provider do you use now? i've never found a free host that didn't add something
194 posts
Location
Spain
Posted 18 March 2013 - 10:50 AM
out of curiosity, what provider do you use now? i've never found a free host that didn't add something
I use
http://www.hostinger.es/
2088 posts
Location
South Africa
Posted 18 March 2013 - 05:48 PM
But what you should do is that if it's purely only php code, remove all the HTML code and just echo 'User Created'. and do .readAll() and just print that.
I recently changed that for my code, I don't use <INFO>text</INFO> anymore :P/>
I didn't do that because in the first hosting I tried, it always put some code at the end of the file (I think it was a visit counter). But that server has an outdated PHP version that is not compatible with bcrypt, so I changed my server hoster, and now it doesn't write anything, so I will just echo the info.
Use gsub to remove it.
I use 000webhost (they're brilliant) and in-order to get the correct text, I do this:
local a = res.readAll():gsub( '\n', '' ):gsub( '<%!%-%- Hosting24 Analytics Code %-%-><script type="text/javascript" src="http://stats.hosting24.com/count.php"></script><%!%-%- End Of Analytics Code %-%->', '' )
First remove all the new lines, then remove the other stuff.
out of curiosity, what provider do you use now? i've never found a free host that didn't add something
I use
http://www.hostinger.es/
Look what it says, save it into a file and then use gsub to remove it.
If you can't, give it to me what it says and I'll do it for you.
194 posts
Location
Spain
Posted 19 March 2013 - 04:25 AM
I tried 000webhost because I saw you use it in CC-YouTube. But PHP 5.2 is unsupported since 2011, and it's not compatible with bcrypt, the most secure option to store passwords.
Also, hostinger has more disk space (2000mb instead of 1500), unlimited add-on domains, PHP 5.4, 2 FTP accounts, etc. But only 2 sub-domains (instead of 5 in 000webhost).