Posted 16 March 2013 - 05:36 AM
Title: For-Do loop bad math?
I'm writing a script that has a user variable and multiple nested for loops that have a step of "1/<var>", and while I was bug testing I noticed that I was getting unexpected results from my for loop. Because it is simple math to determine how many times the for loop executes the code, I decided to pre-calculate it as it would use less memory, and less time (1000s of calculations add up) than a nested counter variable. However, my results were off, At first I thought I did my math wrong somewhere, but I hadn't. So I distilled the code down to just the loop and executed it line by line in a terminal. Here are the results:
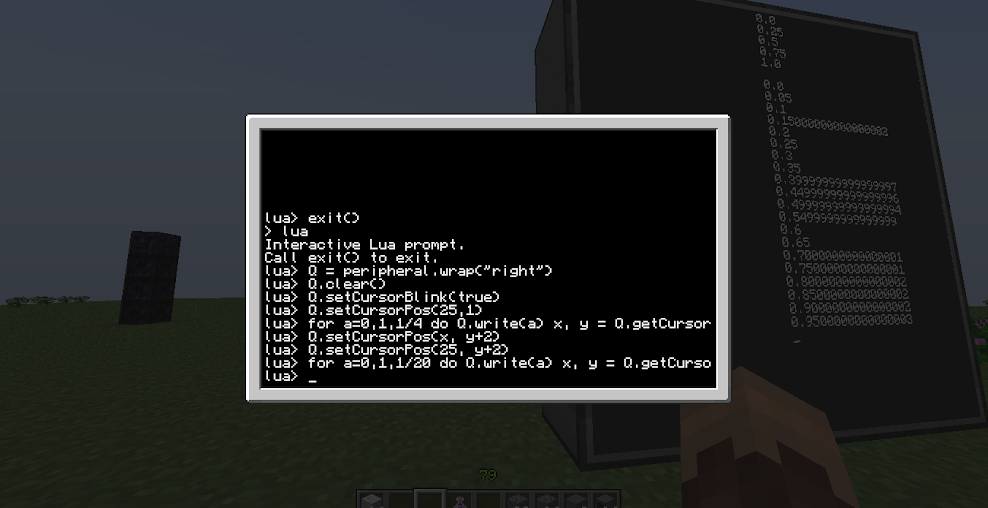
For those curious, the full code input here is:
If you can't see the problem, count the number of times the code is executed. It is 5 for step size 1/4 and 20 for 1/20.
I would like to keep my code as for loops for convenience, but this problem is almost making me switch to repeat-until loops.
Can somebody please explain what is happening here?
I'm writing a script that has a user variable and multiple nested for loops that have a step of "1/<var>", and while I was bug testing I noticed that I was getting unexpected results from my for loop. Because it is simple math to determine how many times the for loop executes the code, I decided to pre-calculate it as it would use less memory, and less time (1000s of calculations add up) than a nested counter variable. However, my results were off, At first I thought I did my math wrong somewhere, but I hadn't. So I distilled the code down to just the loop and executed it line by line in a terminal. Here are the results:
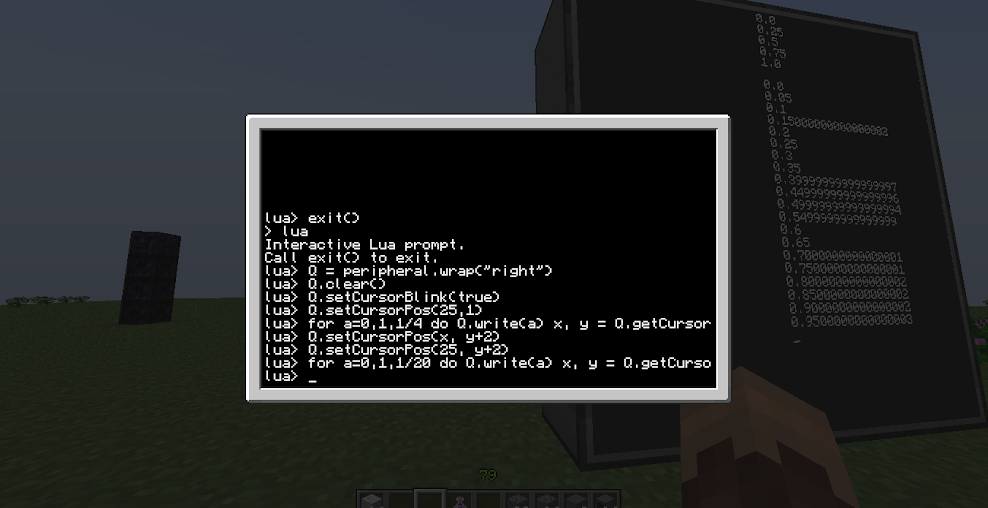
For those curious, the full code input here is:
Q = peripheral.wrap("right")
Q.clear()
Q.setCursorBlink(true)
Q.setCursorPos(25,1)
for a=0,1,1/4 do Q.write(a) x, y = Q.getCursorPos() Q.setCursorPos(25,y+1) end
Q.setCursorPos(x, y+2) --this was a screw up
Q.setCursorPos(25, y+2)
for a=0,1,1/20 do Q.write(a) x, y = Q.getCursorPos() Q.setCursorPos(25,y+1) end
If you can't see the problem, count the number of times the code is executed. It is 5 for step size 1/4 and 20 for 1/20.
I would like to keep my code as for loops for convenience, but this problem is almost making me switch to repeat-until loops.
Can somebody please explain what is happening here?