This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
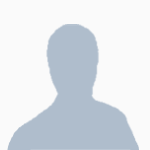
Need help with rednet
Started by Pebbe19, 17 March 2013 - 10:50 AMPosted 17 March 2013 - 11:50 AM
I want to make a program where I can type in a message and ID to send the message to, and the computer will send this message. But how can i get this same program to recieve a message while waiting for a text input?
Posted 17 March 2013 - 12:01 PM
If you are using os.pullEvent, there is an event called "rednet_message".
Posted 17 March 2013 - 12:16 PM
Any example fo how to use that?
Posted 17 March 2013 - 12:17 PM
Use a parallel with two functions; one waiting for an input and the other waiting for a rednet_message event.
Posted 17 March 2013 - 12:24 PM
while true do
evt, arg1, arg2, arg3 = os.pullEvent()
if evt == "rednet_message" then
print(arg1, ": ", arg2)
elseif evt == "key" then
-- add char to input line.
end
end
That should help you along the way, and will use less resources than parallel.
Posted 17 March 2013 - 12:27 PM
Without using the parallel it wouldn't capture the rednet message if the user was typing something to send
Posted 17 March 2013 - 12:32 PM
Without using the parallel it wouldn't capture the rednet message if the user was typing something to send
?
I don't think you can click a key while a rednet message is fired.
No event can occur the same time as anotherone.
Posted 17 March 2013 - 12:35 PM
we are not firing two events, we are comparing the event returned after it is fired.Without using the parallel it wouldn't capture the rednet message if the user was typing something to send
?
I don't think you can click a key while a rednet message is fired.
No event can occur the same time as anotherone.
RemiX, explain! I am on my phone…
Posted 17 March 2013 - 12:39 PM
Without using the parallel it wouldn't capture the rednet message if the user was typing something to send
?
I don't think you can click a key while a rednet message is fired.
No event can occur the same time as anotherone.
If you use os.pullEvent, then I'm quite sure you can
Posted 17 March 2013 - 12:51 PM
Talk to me like I'm an idiot, I can't make any sense out of these replies. If i want to do this: Computer waits for either the user to type something, or to recieve a message. If the computer recieves a message, then I want it to display the message, If the computer gets a tex input from the user, then i want it to be able to use it to send a message to an ID.
Posted 17 March 2013 - 12:54 PM
@remiX: But then using parallel has the same effect. So in any way, suicidalSTDz statement that only parallel would work is false.
Parallel will resume the coroutine with the new event, so it will only pull the next event after it has resumed and waited for all the coroutines it handled. Therefor it results in the same effekt as Omega's programm.
@Pebbe19: OmegaVest posted one of the solution you can use.
Parallel will resume the coroutine with the new event, so it will only pull the next event after it has resumed and waited for all the coroutines it handled. Therefor it results in the same effekt as Omega's programm.
@Pebbe19: OmegaVest posted one of the solution you can use.
Posted 17 March 2013 - 12:55 PM
I am not here to talk to people with an idiotic tone. I am here to help people with problems in a logical way. With this being said, I am going to opt out of this conversation. o/Talk to me like I'm an idiot, I can't make any sense out of these replies. If i want to do this: Computer waits for either the user to type something, or to recieve a message. If the computer recieves a message, then I want it to display the message, If the computer gets a tex input from the user, then i want it to be able to use it to send a message to an ID.
Edit: I said the parallel would capture the event when a user is typing
Posted 17 March 2013 - 01:00 PM
Depends if you want to have a long code with your own 'read' function, if not than you use parallel.
local input, sID, msg
local id = 9
term.clear() term.setCursorPos( 1, 1 )
local function waitForInput()
term.setCursorPos( 1, 1 )
write( ' > ' )
input = read()
rednet.send( id, input )
print( '\nSent ' .. input .. ' to ID: ' .. id )
end
local function waitForRednet()
sID, msg = rednet.recieve()
print( '\nReceived: ' .. msg .. ' from ID: ' .. sID )
end
parallel.waitForAny( waitForInput, waitForRednet )
Posted 17 March 2013 - 01:01 PM
Edit: I said the parallel would capture the event when a user is typing
Look at the code of the parallel api. Coroutines are not running at the same time. If one will be resumed, all others are stopped until that routine yields.
Posted 17 March 2013 - 01:37 PM
Depends if you want to have a long code with your own 'read' function, if not than you use parallel.local input, sID, msg local id = 9 term.clear() term.setCursorPos( 1, 1 ) local function waitForInput() term.setCursorPos( 1, 1 ) write( ' > ' ) input = read() rednet.send( id, input ) print( '\nSent ' .. input .. ' to ID: ' .. id ) end local function waitForRednet() sID, msg = rednet.recieve() print( '\nReceived: ' .. msg .. ' from ID: ' .. sID ) end parallel.waitForAny( waitForInput, waitForRednet )
This was alot easier to understand than the code OmegaVest gave me, although I'll try to be able to input the id without editing the program, i want to be able to send to different computers.
Posted 17 March 2013 - 01:41 PM
I am more than aware how the two work. Look at remiX,s code. That is what I was trying to get at.Edit: I said the parallel would capture the event when a user is typing
Look at the code of the parallel api. Coroutines are not running at the same time. If one will be resumed, all others are stopped until that routine yields.
Posted 17 March 2013 - 02:09 PM
Depends if you want to have a long code with your own 'read' function, if not than you use parallel.local input, sID, msg local id = 9 term.clear() term.setCursorPos( 1, 1 ) local function waitForInput() term.setCursorPos( 1, 1 ) write( ' > ' ) input = read() rednet.send( id, input ) print( '\nSent ' .. input .. ' to ID: ' .. id ) end local function waitForRednet() sID, msg = rednet.recieve() print( '\nReceived: ' .. msg .. ' from ID: ' .. sID ) end parallel.waitForAny( waitForInput, waitForRednet )
I tried this out, I get this: parallel:22: par:15: attempt to call nil
Line 15 is this: sID, msg = rednet,recieve():
Posted 17 March 2013 - 03:19 PM
Line 15 is this: sID, msg = rednet,recieve():
It's rednet.receive() (A '.' and 'receive')
Posted 18 March 2013 - 01:57 AM
Is there no way of replicating read() in a os.pullEvent() ?
Posted 18 March 2013 - 02:23 AM
There is, of course.
[attachment=1080:t.PNG]
Spoiler
local function inputAndRednet( replaceChar, x, y )
local input = ''
term.setCursorBlink(true)
term.setCursorPos( x, y )
replaceChar = replaceChar and replaceChar:sub( 1, 1 ) or nil
while true do
local event, p1, p2, p3 = os.pullEvent()
if event == "char" then
-- Character event
input = input .. p1
write( replaceChar or p1 )
elseif event == "key" then
if p1 == keys.backspace and #rString >= 1 then
-- Backspace
input = input:sub( -2 )
xPos, yPos = term.getCursorPos()
term.setCursorPos(xPos-2, yPos)
write( input:sub( -2 ) .. ' ' )
elseif p1 == keys.enter then
-- Enter key to stop waiting for user input
return 'input', input
end
elseif event == "rednet_message" then
-- Rednet message
return 'rednet.message', { senderID = p1, msg = p2, dist = p3 }
end
end
end
rednet.open( 'right' ) -- side of modem
term.clear() term.setCursorPos( 1, 1 )
local sType, info = inputAndRednet( nil, 3, 3 )
term.clear() term.setCursorPos( 1, 1 )
if sType == 'input' then
print( 'Your inputted text: ' .. info )
elseif sType == 'rednet.message' then
print( 'Message received.' )
print( ' SenderID: ' .. info.senderID )
print( ' Message: ' .. info.msg )
print( ' Distance: ' .. info.dist )
end
[attachment=1080:t.PNG]