Can someone help me with this?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
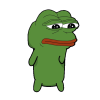
Cursor howto?
Started by nolongerexistant, 06 April 2012 - 01:32 AMPosted 06 April 2012 - 03:32 AM
I've been trying to create an cursor all night, but I can't get it working and I didn't find any info on internet; except whole API's with shitloads of code and it's kinda hard to find the cursor-only code in there.
Can someone help me with this?
Can someone help me with this?
Posted 06 April 2012 - 03:55 AM
Are you looking for a cursor that moves in the menu to select the option you want to perform?
If so look at the menu tutorial in the tutorial section of the forums.
If so look at the menu tutorial in the tutorial section of the forums.
Posted 06 April 2012 - 04:03 AM
If you wan't to make a cursor, you need term.setCursorPos() and some variables. Here is a example:
If you put all this code together like this:
It will create a cursor that can move left and right.
If you wan't to make options in a menu like above, remove the left and right and keep up/down and make it so if the cursor is at the same position of the button, make it open the button.
This is how I did it in my WinOS. You can look at that source for more information
local currentX = 1
local currentY = 1
These will set the cursor position.
function drawCursor()
term.clear()
term.setCursorPos(currentX, currentY)
write(">")
end
This will draw the cursor at the current x/y, now that we got that, we need to call drawCursor() and add controls like this:
while true do
drawCursor()
local e,key = os.pullEvent( "key" )
if key == 17 or key == 200 then --up
currentY = currentY -1
elseif key == 31 or key == 208 then --down
currentY = currentY +1
elseif key == 203 or key == 30 then --left
currentX = currentX -1
elseif key == 205 or key == 32 then --right
currentX = currentX +1
end
end
If you put all this code together like this:
local currentX = 1 --Saves the current X of the cursor
local currentY = 1 --Saves the current Y of the cursor
function drawCursor()
term.clear() --Clears the screen
term.setCursorPos(currentX, currentY) --Draws a cursor at the current X/Y
write(">")
end
while true do
drawCursor()
local e,key = os.pullEvent( "key" )
if key == 17 or key == 200 then --up
currentY = currentY -1
elseif key == 31 or key == 208 then --down
currentY = currentY +1
elseif key == 203 or key == 30 then --left
currentX = currentX -1
elseif key == 205 or key == 32 then --right
currentX = currentX +1
end
end
It will create a cursor that can move left and right.
If you wan't to make options in a menu like above, remove the left and right and keep up/down and make it so if the cursor is at the same position of the button, make it open the button.
This is how I did it in my WinOS. You can look at that source for more information
Posted 06 April 2012 - 04:45 AM
@ComputerCraftFan Thanks, but if I put it in my code everything dissapears except the cursor, I tried finding a way around the term.clear()but it didn't work. Also, i'm trying to block the cursor from going outside the selectbox (Y: 7 to Y: 10)
Here's my code so far:
Also, don't mind the commented code, i'm trying to let it display the number of accounts registered and logged in today.
Here's my code so far:
Local Author = "Snakybo inc."
Local Version = "1.0"
-- Local Registered = numRegistered()
-- Local LoggedInToday = numLoggedInToday()
Local currentX = 13
Local currentY = 7
function drawCursor()
term.clear()
term.setCursorPos(currentX, currentY)
write("<--")
end
--[[
function numRegistered()
print "6"
end
function numLoggedInToday()
print "2"
end
]]--
shell.run("clear")
print " --------------------------------------------------"
print("| "..Author.." "..Version.." |")
print " --------------------------------------------------"
print ""
--[[
print " --------------------------------------------------"
print("| Registered users: "..Registered)
print("| Users logged in today: "..LoggedInToday)
print " --------------------------------------------------"
]]--
sleep(.5)
print "What do you want to do?"
print ""
print " -----------"
print "| Login |"
print "| Register|"
print "| Shutdown|"
print " -----------"
while true do
drawCursor()
local e, key = os.pullEvent("key")
if key == 17 or key == 200 then
currentY = currentY -1
elseif key == 31 or key == 208 then
currentY = currentY +1
end
end
-- shell.run("Login")
-- shell.run("Register")
Also, don't mind the commented code, i'm trying to let it display the number of accounts registered and logged in today.
Posted 06 April 2012 - 05:19 AM
@ComputerCraftFan Thanks, but if I put it in my code everything dissapears except the cursor, I tried finding a way around the term.clear()but it didn't work. Also, i'm trying to block the cursor from going outside the selectbox (Y: 7 to Y: 10)
Here's my code so far:Local Author = "Snakybo inc." Local Version = "1.0" -- Local Registered = numRegistered() -- Local LoggedInToday = numLoggedInToday() Local currentX = 13 Local currentY = 7 function drawCursor() term.clear() draw() term.setCursorPos(currentX, currentY) write("<--") end --[[ function numRegistered() print "6" end function numLoggedInToday() print "2" end ]]-- --shell.run("clear") function draw() print " --------------------------------------------------" print("| "..Author.." "..Version.." |") print " --------------------------------------------------" print "" --[[ print " --------------------------------------------------" print("| Registered users: "..Registered) print("| Users logged in today: "..LoggedInToday) print " --------------------------------------------------" ]]-- sleep(.5) print "What do you want to do?" print "" print " -----------" print "| Login |" print "| Register|" print "| Shutdown|" print " -----------" end while true do drawCursor() local e, key = os.pullEvent("key") if key == 17 or key == 200 then currentY = currentY -1 elseif key == 31 or key == 208 then currentY = currentY +1 end end -- shell.run("Login") -- shell.run("Register")
Also, don't mind the commented code, i'm trying to let it display the number of accounts registered and logged in today.
Try the code now
You can edit the while true do to this:Also, i'm trying to block the cursor from going outside the selectbox (Y: 7 to Y: 10)
local e, key = os.pullEvent("key")
if key == 17 or key == 200 then
if currentY > 7 then
currentY = currentY -1
end
elseif key == 31 or key == 208 then
if currentY < 10 then
currentY = currentY +1
end
end
end
Posted 06 April 2012 - 06:06 PM
Thanks alot! But do you happen to know the Keyboard Code for the enter key? So I can make it open a program
Posted 06 April 2012 - 06:20 PM
Thanks alot! But do you happen to know the Keyboard Code for the enter key? So I can make it open a program
28