Anyways, i assumed the computer craft forums would be the best place to look. The game I am coding is a galaga clone. I have all of the move and fire code set up. However, how do i make a bullet damage a ship?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
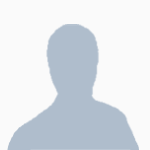
Need lua help for 2d game!
Started by Kron, 31 March 2013 - 08:12 AMPosted 31 March 2013 - 10:12 AM
Hey guys. Im coding a 2d game. Sorry for any grammar errors. When your taking a 2 hour ride using your cell phones 4g, you cant make everything look perfect.
Anyways, i assumed the computer craft forums would be the best place to look. The game I am coding is a galaga clone. I have all of the move and fire code set up. However, how do i make a bullet damage a ship?
Anyways, i assumed the computer craft forums would be the best place to look. The game I am coding is a galaga clone. I have all of the move and fire code set up. However, how do i make a bullet damage a ship?
Posted 31 March 2013 - 12:48 PM
NitrogenFingers has a GameUtils API. Do a search for it. It has pixel-based and box-based collision detection.
Posted 31 March 2013 - 04:00 PM
That's a really tricky part of any game. it is rarely simple, and will sometimes be tedious. I agree with Dicruz129 that is probaly te best way, or you could just look into the Gameutils src code and see how. (i dont know either :P/>/>)
Posted 31 March 2013 - 04:24 PM
It really depends on how you're organizing your game, and I really wouldn't recommend overhauling everything with another engine.
You would do this under your game "update" loop, where everything is moved and/or created.
http://pastebin.com/MjdR7TcS
Usually, people store their "game objects" as separate tables within tables with different properties, so I'm going to assume that's how you're doing things, as that's probably the best way of doing it anyway.
This method is for if all of your game objects are stored in a grid.
EDIT: This seems to have been my thousandth post. Woo.
You would do this under your game "update" loop, where everything is moved and/or created.
http://pastebin.com/MjdR7TcS
Usually, people store their "game objects" as separate tables within tables with different properties, so I'm going to assume that's how you're doing things, as that's probably the best way of doing it anyway.
Spoiler
-- go through the "enemies" table
-- backwards, so we can efficiently remove objects, and there will be no skips
for enum = #enemies, 1, -1 do
local enemy = enemies[enum]
-- all of your enemy creation/moving code
-- go through whatever table holds the bullets
for bnum = #bullets, 1, -1 do
local bullet = bullets[bnum]
-- check if they are touching
-- for this, I assume that everything takes up only one character
-- use whatever method fits you, however
if bullet.x == enemy.x
and bullet.y == enemy.y then
-- destroy both the enemy and the bullet
-- by removing them from their respective tables
table.remove(enemies, enum)
table.remove(bullets, bnum)
break
-- alternatively, you could use a health system for enemies.
--[[
if enemy.health > 0 then
enemy.health = enemy.health - 1
else
-- the removing code above
end
--]]
end
end
end
This method is for if all of your game objects are stored in a grid.
Spoiler
-- go through all of the grid squares
for y=1, #grid do
for x=1, #grid[y] do
-- we assume that each grid space has a "type" property that tells us what is there
if grid[y][x].type == 'enemy' then
-- for this, we assume that the player is shooting bullets from the bottom
-- therefore, any bullets will be below us
-- so we check that space, which is at x, y + 1
if grid[y + 1] and grid[y + 1][x] and grid[y + 1][x].type == 'bullet' then
-- then, erase both the enemy and the bullet from the grid if they are both in the right position
grid[y][x] = nil
grid[y + 1][x] = nil
break
end
end
end
end
EDIT: This seems to have been my thousandth post. Woo.
Posted 01 April 2013 - 05:15 AM
you could have a ship with a health value. This would determine how much health it has.
E.G:
If will not work tell me. I am new to codding and do not know everything. I do know that this would work if the code is how –> I <– would have codded it.
E.G:
ship-one-health = 3
--if statement to find if the projectile is in the same
x,y of the ship--
ship-1-health =(ship-one-health -1)
If will not work tell me. I am new to codding and do not know everything. I do know that this would work if the code is how –> I <– would have codded it.
Posted 01 April 2013 - 07:45 AM
that would not work. the logic is off.you could have a ship with a health value. This would determine how much health it has.
E.G:ship-one-health = 3 --if statement to find if the projectile is in the same x,y of the ship-- ship-1-health =(ship-one-health -1)
If will not work tell me. I am new to codding and do not know everything. I do know that this would work if the code is how –> I <– would have codded it.
shipHealth = 3
--if statment blabla
shipHealth = shipHealth - 1
Posted 01 April 2013 - 07:48 AM
that would not work. the logic is off.you could have a ship with a health value. This would determine how much health it has.
E.G:ship-one-health = 3 --if statement to find if the projectile is in the same x,y of the ship-- ship-1-health =(ship-one-health -1)
If will not work tell me. I am new to codding and do not know everything. I do know that this would work if the code is how –> I <– would have codded it.shipHealth = 3 --if statment blabla shipHealth = shipHealth - 1
With ship-one-health and ship-1-health he meant "insert a variable name here".
Posted 01 April 2013 - 07:51 AM
that would not work. the logic is off.you could have a ship with a health value. This would determine how much health it has.
E.G:ship-one-health = 3 --if statement to find if the projectile is in the same x,y of the ship-- ship-1-health =(ship-one-health -1)
If will not work tell me. I am new to codding and do not know everything. I do know that this would work if the code is how –> I <– would have codded it.shipHealth = 3 --if statment blabla shipHealth = shipHealth - 1
With ship-one-health and ship-1-health he meant "insert a variable name here".
Yes.
Posted 01 April 2013 - 08:41 AM
I suppose if it helps, I can upload the code I have so far
print("Welcome to SpaceGame 0.1 BETA.")
print("What is your game name?")
username = read()
print("Hello, '..username'!")
sleep(2)
term.clear()
function on.arrowLeft = arrowleft
function.on.arrowRight = arrowright
function.on.spacebar = space
-- Note to self, create function for gethealth()
term.setCursorPos(1,1)
if gethealth =5 then
term.setCursorPos(12,12)
print("5/5")
if gethealth = 4 then
term.setCursorPos(12,12)
print("4/5")
if gethealth = 3 then
term.setCursorPos(12,12)
print("3/5")
if gethealth = 2 then
term.setCursorPos(12,12)
print("2/5")
if gethealth = 1 then
term.setCursorPos(1,1)
print("1/5")
display.newImage("os/spacegame/sprites/lowhp.png,12,12,true")
if gethealth = 0 then
term.setCursorPos(6,6)
print(" GAME OVER ")
-- Note to self, create function for restart
display.newImage("os/spacegame/sprites/lvl1 ship.png,1,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,1,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,2,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,2,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,1,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,2,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,3,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,3,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,2,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,3,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,4,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,4,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,3,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,4,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,5,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,5,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,4,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,5,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,6,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,6,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,5,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,6,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,7,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,7,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,6,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,7,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,8,1 ,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,8,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,7,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,8,1,false")
display.newimage("os/spacegame/sprites/lvl1 ship.png,9,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,9,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,8,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,9,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,10,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,10,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,9,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,10,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,11,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,11,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,10,1,true")
if arrowright = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,11,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,12,1,true")
if arrowleft = pressed then
display.newImage("os/spacegame/sprites/lvl1 ship.png,12,1,false")
display.newImage("os/spacegame/sprites/lvl1 ship.png,11,1,true")
if space = pressed then
display.newImage("os/spacegame/sprites/lazer.png,1,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,1,false")
display.newImage("os/spacegame/sprites/lazer.png,1,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,2,false")
display.newImage("os/spacegame/sprites/lazer.png,1,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,3,false")
display.newImage("os/spacegame/sprites/lazer.png,1,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,4,false")
display.newImage("os/spacegame/sprites/lazer.png,1,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,5,false")
display.newImage("os/spacegame/sprites/lazer.png,1,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,6,false")
display.newImage("os/spacegame/sprites/lazer.png,1,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,7,false")
display.newImage("os/spacegame/sprites/lazer.png,1,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,8,false")
display.newImage("os/spacegame/sprites/lazer.png,1,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,9,false")
display.newImage("os/spacegame/sprites/lazer.png,1,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,10,false")
display.newImage("os/spacegame/sprites/lazer.png,1,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,1,11,false")
display.newImage("os/spacegame/sprites/lazer.png,1,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,2,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,1,false")
display.newImage("os/spacegame/sprites/lazer.png,2,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,2,false")
display.newImage("os/spacegame/sprites/lazer.png,2,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,3,false")
display.newImage("os/spacegame/sprites/lazer.png,2,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,4,false")
display.newImage("os/spacegame/sprites/lazer.png,2,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,5,false")
display.newImage("os/spacegame/sprites/lazer.png,2,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,6,false")
display.newImage("os/spacegame/sprites/lazer.png,2,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,7,false")
display.newImage("os/spacegame/sprites/lazer.png,2,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,8,false")
display.newImage("os/spacegame/sprites/lazer.png,2,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,9,false")
display.newImage("os/spacegame/sprites/lazer.png,2,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,10,false")
display.newImage("os/spacegame/sprites/lazer.png,2,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,2,11,false")
display.newImage("os/spacegame/sprites/lazer.png,2,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,3,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,1,false")
display.newImage("os/spacegame/sprites/lazer.png,3,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,2,false")
display.newImage("os/spacegame/sprites/lazer.png,3,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,3,false")
display.newImage("os/spacegame/sprites/lazer.png,3,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,4,false")
display.newImage("os/spacegame/sprites/lazer.png,3,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,5,false")
display.newImage("os/spacegame/sprites/lazer.png,3,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,6,false")
display.newImage("os/spacegame/sprites/lazer.png,3,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,7,false")
display.newImage("os/spacegame/sprites/lazer.png,3,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,8,false")
display.newImage("os/spacegame/sprites/lazer.png,3,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,9,false")
display.newImage("os/spacegame/sprites/lazer.png,3,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,10,false")
display.newImage("os/spacegame/sprites/lazer.png,3,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,3,11,false")
display.newImage("os/spacegame/sprites/lazer.png,3,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,4,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,1,false")
display.newImage("os/spacegame/sprites/lazer.png,4,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,2,false")
display.newImage("os/spacegame/sprites/lazer.png,4,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,3,false")
display.newImage("os/spacegame/sprites/lazer.png,4,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,4,false")
display.newImage("os/spacegame/sprites/lazer.png,4,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,5,false")
display.newImage("os/spacegame/sprites/lazer.png,4,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,6,false")
display.newImage("os/spacegame/sprites/lazer.png,4,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,7,false")
display.newImage("os/spacegame/sprites/lazer.png,4,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,8,false")
display.newImage("os/spacegame/sprites/lazer.png,4,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,9,false")
display.newImage("os/spacegame/sprites/lazer.png,4,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,10,false")
display.newImage("os/spacegame/sprites/lazer.png,4,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,4,11,false")
display.newImage("os/spacegame/sprites/lazer.png,4,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,5,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,1,false")
display.newImage("os/spacegame/sprites/lazer.png,5,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,2,false")
display.newImage("Os/spacegame/sprites/lazer.png,5,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,3,false")
display.newImage("os/spacegame/sprites/lazer.png,5,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,4,false")
display.newImage("os/spacegame/sprites/lazer.png,5,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,5,false")
display.newImage("os/spacegame/sprites/lazer.png,5,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,6,false")
display.newImage("os/spacegame/sprites/lazer.png,5,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,7,false")
display.newImage("os/spacegame/sprites/lazer.png,5,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,8,false")
display.newImage("os/spacegame/sprites/lazer.png,5,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,9,false")
display.newImage("os/spacegame/sprites/lazer.png,5,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,10,false")
display.newImage("os/spacegame/sprites/lazer.png,5,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,5,11,false")
display.newImage("os/spacegame/sprites/lazer.png,5,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,6,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,1,false")
display.newImage("os/spacegame/sprites/lazer.png,6,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,2,false")
display.newImage("os/spacegame/sprites/lazer.png,6,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,3,false")
display.newImage("os/spacegame/sprites/lazer.png,6,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,4,false")
display.newImage("os/spacegame/sprites/lazer.png,6,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,5,false")
display.newImage("os/spacegame/sprites/lazer.png,6,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,6,false")
display.newImage("os/spacegame/sprites/lazer.png,6,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,7,false")
display.newImage("os/spacegame/sprites/lazer.png,6,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,8,false")
display.newImage("os/spacegame/sprites/lazer.png,6,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,9,false")
display.newImage("os/spacegame/sprites/lazer.png,6,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,10,false")
display.newImage("os/spacegame/sprites/lazer.png,6,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,6,11,false")
display.newImage("os/spacegame/sprites/lazer.png,6,12,true")
end
if space == presesed then
display.newImage("os/spacegame/sprites/lazer.png,7,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,1,false")
display.newImage("os/spacegame/sprites/lazer.png,7,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,2,false")
display.newImage("os/spacegame/sprites/lazer.png,7,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,3,false")
display.newImage("os/spacegame/sprites/lazer.png,7,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,4,false")
display.newImage("os/spacegame/sprites/lazer.png,7,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,5,false")
display.newImage("os/spacegame/sprites/lazer.png,7,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,6,false")
display.newImage("os/spacegame/sprites/lazer.png,7,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,7,false")
display.newImage("os/spacegame/sprites/lazer.png,7,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,8,false")
display.newImage("os/spacegame/sprites/lazer.png,7,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,9,false")
display.newImage("os/spacegame/sprites/lazer.png,7,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,10,false")
display.newImage("os/spacegame/sprites/lazer.png,7,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,7,11,false")
display.newImage("os/spacegame/sprites/lazer.png,7,12,true")
end
if space == pressed then
display.newImage("os/spacegame/sprites/lazer.png,8,1,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,1,false")
display.newImage("os/spacegame/sprites/lazer.png,8,2,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,2,false")
display.newImage("os/spacegame/sprites/lazer.png,8,3,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,3,false")
display.newImage("os/spacegame/sprites/lazer.png,8,4,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,4,false")
display.newImage("os/spacegame/sprites/lazer.png,8,5,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,5,false")
display.newImage("os/spacegame/sprites/lazer.png,8,6,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,6,false")
display.newImage("os/spacegame/sprites/lazer.png,8,7,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,7,false")
display.newImage("os/spacegame/sprites/lazer.png,8,8,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,8,false")
display.newImage("os/spacegame/sprites/lazer.png,8,9,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,9,false")
display.newImage("os/spacegame/sprites/lazer.png,8,10,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,10,false")
display.newImage("os/spacegame/sprites/lazer.png,8,11,true")
sleep(0.3)
display.newImage("os/spacegame/sprites/lazer.png,8,11,false")
display.newImage("os/spacegame/sprites/lazer.png,8,12,true")
end
if space == pressed then
Posted 01 April 2013 - 04:34 PM
next time you should use a spoiler or upload it to pastebin…I suppose if it helps, I can upload the code I have so far-code snippet-
theres stuff missing caused of the length of you post…
and you should try to find a way using loops to shorten your code. it looks really repeating.