This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
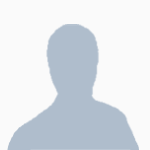
[Lua][Question] Multiple inputs in code? (IDK correct terminology)
Started by wookiederk, 08 April 2013 - 01:19 PMPosted 08 April 2013 - 03:19 PM
I want an input to have multiple arguments (not sure if that's the right way to put it) but I'm not sure how to do it. Lets say if input == "star" then print("star") end. That's a simple one word input. But how would you do something where your input was "star 3" where the three means you do print("star") three times? Ex. you type "star 2" then it would print "star" two times, or if you type "star 16" it would print "star" 16 times. At the same time, if you type just "star" with no number after it would only print "star" once by default. Of course in my real code print "star" would be replaced with something else. How would I do this?
Posted 08 April 2013 - 03:27 PM
local args = {...}
for i = 1,tonumber(tArgs[1]) do
print("star")
end
Or inside a script:
local times = read()
local valid = tonumber(times)
if type(valid) ~= "number" then
print("This is not a number")
return
else
for i = 1,valid do
print("star")
end
end
Posted 08 April 2013 - 03:29 PM
I'm not positive this would work, but it should. I think io.read() can get more than one word, for instance "star 3". That will be stored to whatever variable you set io.read() to. The string.sub(string, starting character, ending character) function could be used to differentiate the words. For instance:
variable = io.read() – the first word being "star" is assumed.
if string.sub(variable, 6, 6) == nil then – string.sub(variable, 6, 6) returns either the number or nil.
number = 1
else
number = tonumber(string.sub(variable, 6, 6))
end
for i = 1, number do
print("star")
end
(Sorry about no code container, I'm working off a mobile device.)
That should do what you want. Hope that helps!
Edit: Suicidal's is much cleaner!
variable = io.read() – the first word being "star" is assumed.
if string.sub(variable, 6, 6) == nil then – string.sub(variable, 6, 6) returns either the number or nil.
number = 1
else
number = tonumber(string.sub(variable, 6, 6))
end
for i = 1, number do
print("star")
end
(Sorry about no code container, I'm working off a mobile device.)
That should do what you want. Hope that helps!
Edit: Suicidal's is much cleaner!
Posted 08 April 2013 - 03:56 PM
Although it looks clean, the code needs to run when you type a string then number, that is just typing a number to run the code that many times. Quantum's works great, only that when you type the string with no number after it returns "for" limit must be a number error. Thanks for both your help so far! Edit: Can you explain what string.sub(variable, 6, 6) means and how to tonumber(string.sub(variable,6,6) works?local args = {...} for i = 1,tonumber(tArgs[1]) do print("star") end
Or inside a script:local times = read() local valid = tonumber(times) if type(valid) ~= "number" then print("This is not a number") return else for i = 1,valid do print("star") end end
Posted 08 April 2013 - 04:23 PM
I'm not entirely sure why typing no number would error it, maybe somebody can explain why my code does that?
The command 'string.sub(string, starting character, ending character)' returns a sub-string or portion of the string you input, starting at the second argument and going to the third. For instance:
variable = "Test"
print(variable)
print(string.sub(variable, 1, 4))
This code will print "Test" twice. The first time is normal, then it prints a sub-string of variable starting at character 1 and going to character 4, which, in this case, is the entire string.
variable = "Test"
print(variable)
print(string.sub(variable, 1, 3))
This code will print "Test" and then "Tes." If you put the same number in the second and third arguments, it will give you the character at that point. print(string.sub(variable, 1, 1)) would print: "T".
'tonumber' is quite simple. It converts whatever is in its parentheses into a number if it can. The same works for 'tostring,' except it converts whatever is in its parentheses to a string. Very useful functions for when you are trying to convert input, a string, to a function that needs a number.
If a variable = "4", tonumber(variable) = 4.
In the code, variable = "star #". string.sub(variable, 6, 6) returns the 6th character of variable, which is the number. That is a string, and we need a number, so we use 'tonumber' to fix it.
I hope that clears things up!
The command 'string.sub(string, starting character, ending character)' returns a sub-string or portion of the string you input, starting at the second argument and going to the third. For instance:
variable = "Test"
print(variable)
print(string.sub(variable, 1, 4))
This code will print "Test" twice. The first time is normal, then it prints a sub-string of variable starting at character 1 and going to character 4, which, in this case, is the entire string.
variable = "Test"
print(variable)
print(string.sub(variable, 1, 3))
This code will print "Test" and then "Tes." If you put the same number in the second and third arguments, it will give you the character at that point. print(string.sub(variable, 1, 1)) would print: "T".
'tonumber' is quite simple. It converts whatever is in its parentheses into a number if it can. The same works for 'tostring,' except it converts whatever is in its parentheses to a string. Very useful functions for when you are trying to convert input, a string, to a function that needs a number.
If a variable = "4", tonumber(variable) = 4.
In the code, variable = "star #". string.sub(variable, 6, 6) returns the 6th character of variable, which is the number. That is a string, and we need a number, so we use 'tonumber' to fix it.
I hope that clears things up!
Posted 09 April 2013 - 12:27 AM
The code is giving an error cause you tell him that the number he should loop to is at position 6 to 6 of the string.
when you not give him a number there he will have a nil and he can not loop to a nil
to fix this just use this
other problem with the provided function is that it will error everytime there is no number at position 6 of the string.
if you type in "HelloKittySucks" 2
he will error or if you have fixed the error by adding or 1 he will just print it once not 2 times like you typed
Greets Loki
when you not give him a number there he will have a nil and he can not loop to a nil
to fix this just use this
number = tonumber(string.sub(variable, 6, 6)) or 1 -- or 1 sets number to 1 if the other condition is nil
other problem with the provided function is that it will error everytime there is no number at position 6 of the string.
if you type in "HelloKittySucks" 2
he will error or if you have fixed the error by adding or 1 he will just print it once not 2 times like you typed
Greets Loki
Posted 09 April 2013 - 12:53 AM
Wrote a short code that should fit your needs. Put some comments in if you have further Questions just ask.
number can be as big as you like. if you put in "Bimmelbahn 2132" he will print "Bimmelbahn" 2132 times :D/>
number can be as big as you like. if you put in "Bimmelbahn 2132" he will print "Bimmelbahn" 2132 times :D/>
variable = read()
space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence
counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop
variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string
number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false
if not number then -- if number false Print error
print "the second parameter must be a number" -- print error
else
for i = 1, number do
print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different
end
end
Posted 09 April 2013 - 04:35 AM
Thanks for the help I understand how this works. However, the code still throws an error if you leave the second parameter blank: attempt to perform arithmetic __add on nil and number. Also, if I was to create a detector for the first word, would this code work: if string.sub(variable, 1, 4) == "star" then? Edit: I see the reason why it throws the error. When you type the string, it checks the second space after the word if its a number (and catches if its not). But if you simply leave the string with no spaces after then it will throw the error….Any idea how to fix?
Posted 09 April 2013 - 04:53 AM
local args = {...} for i = 1,tonumber(tArgs[1]) do print("star") end
-snip-
I'd use SuicidalSTDz code. It's, by far, the simplest and easiest.
The only thing I would add would be to make it print once without any 2nd argument.
local tArgs = {...}
tArgs[1] = tArgs[1] or 1 -- if no 2nd argument is given, tArgs[1] is 1.
for i = 1,tonumber(tArgs[1]) do
print("star")
end
This way you can just type whatever word you want printed, and if you don't supply it with a number it automatically does it once.
Posted 09 April 2013 - 04:55 AM
I'm on the mobile at the moment will paste code later if needed.
For your question about if substring etc..
Yes this would work.
To capture the error you can do the same thing I did for number to the space1 variable
Simply add or false
Then check if false and throw error message
For your question about if substring etc..
Yes this would work.
To capture the error you can do the same thing I did for number to the space1 variable
Simply add or false
Then check if false and throw error message
Posted 09 April 2013 - 05:30 AM
Yeah im not sure if I follow, so It would be great to see what you mean.I'm on the mobile at the moment will paste code later if needed.
For your question about if substring etc..
Yes this would work.
To capture the error you can do the same thing I did for number to the space1 variable
Simply add or false
Then check if false and throw error message
Posted 09 April 2013 - 08:59 AM
There is no need to use the string library in my opinion. Capture the args called with the file or capture user input with the read function and print that x amount of times.
Posted 09 April 2013 - 06:41 PM
@wookie ill add the code later. And maybe it would help us if you tell us for what you want to use this code. Maybe there is a more easy way like the one from suicidal
@suicidal
Somehow I agree with you when you want to have printed sth x number of times best thing is write a programm catching the arguments and print it.
Inside a running programm you could use a function with 2 times read first read for the text 2nd for the number of repeats.
But if you don't want to ask the user 2 times for entering text I don't see how you can do it without use of string libary.
Greets Loki
@suicidal
Somehow I agree with you when you want to have printed sth x number of times best thing is write a programm catching the arguments and print it.
Inside a running programm you could use a function with 2 times read first read for the text 2nd for the number of repeats.
But if you don't want to ask the user 2 times for entering text I don't see how you can do it without use of string libary.
Greets Loki
Posted 09 April 2013 - 09:38 PM
Here is the fixed code
variable = read()
space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence
if not space1 then
counter = 1
else
counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop
variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string
end
number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false
term.clear()
if not number then -- if number false Print error
print "the second parameter must be a number" -- print error
else
if variable == "star" then
for i = 1, number do
print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different
end
else
print "wrong word"
end
end
Posted 10 April 2013 - 04:17 PM
I fixed this code as well :P/> there was still errors. But still, 1 please and I really can not stress this more to people, SPACE YOUR CODE!!! Alright now that I got that out of the way, when you putHere is the fixed codevariable = read() space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence if not space1 then counter = 1 else counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string end number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false term.clear() if not number then -- if number false Print error print "the second parameter must be a number" -- print error else if variable == "star" then for i = 1, number do print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different end else print "wrong word" end end
else
if blah == "blah" then
for i =1, number do
-- whatever was in the rest of this if and for.
end
else
-- Code
end
You cannot do that…. its should be
elseif
not
else
if
AND PLEASE SPACE!!!! Oh yeah and open this spoiler for the spaced codeSpoiler
variable = read()
space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence
if not space1 then
counter = 1
else
counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop
variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string
end
number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false
term.clear()
if not number then -- if number false Print error
print "the second parameter must be a number" -- print error
elseif variable == "star" then
for i = 1, number do
print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different
end
else
print "wrong word"
end
end
Posted 10 April 2013 - 06:10 PM
^ Couldn't have said it better.
Posted 10 April 2013 - 07:20 PM
I fixed this code as well :P/>/> there was still errors. But still, 1 please and I really can not stress this more to people, SPACE YOUR CODE!!! Alright now that I got that out of the way, when you putHere is the fixed codevariable = read() space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence if not space1 then counter = 1 else counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string end number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false term.clear() if not number then -- if number false Print error print "the second parameter must be a number" -- print error else if variable == "star" then for i = 1, number do print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different end else print "wrong word" end end
You cannot do that…. its should beelse if blah == "blah" then for i =1, number do -- whatever was in the rest of this if and for. end else -- Code end
notelseif
AND PLEASE SPACE!!!! Oh yeah and open this spoiler for the spaced codeelse if
Spoiler
variable = read() space1 = string.find(variable, "%s") -- searches the string for space and returns the first occurence if not space1 then counter = 1 else counter = string.sub(variable,space1+1,#variable) -- we cut the string starting at the point where he found the space and suggest that everything after the space is the number for the loop variable = string.sub(variable, 1, space1-1) -- now we cut the number of the inital string end number = tonumber(counter) or false -- here we convert the entered counter to a number and set false if its not a number or not existing if so set value to false term.clear() if not number then -- if number false Print error print "the second parameter must be a number" -- print error elseif variable == "star" then for i = 1, number do print(variable) -- i print the text you entered before the number you can edit this if you want to print sth different end else print "wrong word" end end
The code i posted was working. Its not true that you can not do
else
if
Maybe not nice but no error
Mistakes happen when you code on the road :)/>
And for the spacing i dont mind. Im not asking for help in this case and im typing a lot from the ipad so if you want spacing in my code put it in or dont read it :)/>
When i want help or post a really big code that is hard to follow i will add spacing.
But i dont care for a 20 lines code typed on the ipad.
Greets
Posted 10 April 2013 - 07:46 PM
Actually since I remember recalling from a error I keept getting while trying to do
else
if
It didn't work and would give a error, so thats why you have to do (Or at least for me my programs never work unless I do a else if like this "elseif")
elseif
Posted 10 April 2013 - 08:15 PM
as i mentioned before the way i did the elseif was stupid for multiple reasons.
1. Its Inefficient
2. Its looking bad
3. its more code cause the second if has to be ended as well
but it is defenetly working. When it was not working for you, you maybe treated the else if like an elseif without using a second "end". then you are right will not work. else it will work.
1. Its Inefficient
2. Its looking bad
3. its more code cause the second if has to be ended as well
but it is defenetly working. When it was not working for you, you maybe treated the else if like an elseif without using a second "end". then you are right will not work. else it will work.
Posted 11 April 2013 - 12:17 AM
If I understood it right, maybe this code will work for you?
local tArgs = { ... }
if #tArgs ~= 2 then
print( "Usage: text count" )
return
end
local text = tostring( tArgs[1] )
local count = tonumber( tArgs[2] )
for i = 1, count do
print(text)
end
Posted 11 April 2013 - 01:09 AM
You indeed can do:Actually since I remember recalling from a error I keept getting while trying to doIt didn't work and would give a error, so thats why you have to do (Or at least for me my programs never work unless I do a else if like this "elseif")else if
elseif
if something then
--something
else
if not foo then
--something
end
end