local mon = peripheral.wrap("right")
mon.setTextScale(1)
mon.setTextColor(colors.white)
local button={}
mon.setBackgroundColor(colors.black)
function setTable(name, func, xmin, xmax, ymin, ymax)
button[name] = {}
button[name]["func"] = func
button[name]["active"] = false
button[name]["xmin"] = xmin
button[name]["ymin"] = ymin
button[name]["xmax"] = xmax
button[name]["ymax"] = ymax
end
function farmControl()
redstone.setOutput("back")
end
function fillTable()
setTable("Farm Control", farmControl, 2, 28, 2, 4)
end
function fill(text, color, bData)
mon.setBackgroundColor(color)
local yspot = math.floor((bData["ymin"] + bData["ymax"]) /2)
local xspot = math.floor((bData["xmax"] - bData["xmin"] - string.len(text)) /2) +1
for j = bData["ymin"], bData["ymax"] do
mon.setCursorPos(bData["xmin"], j)
if j == yspot then
for k = 0, bData["xmax"] - bData["xmin"] - string.len(text) +1 do
if k == xspot then
mon.write(text)
else
mon.write(" ")
end
end
else
for i = bData["xmin"], bData["xmax"] do
mon.write(" ")
end
end
end
mon.setBackgroundColor(colors.black)
end
function screen()
local currColor
for name,data in pairs(button) do
local on = data["active"]
if on == true then
currColor = colors.lime
else
currColor = colors.lightBlue
end
fill(name, currColor, data)
end
end
function checkxy(x, y)
for name, data in pairs(button) do
if y>=data["ymin"] and y <= data["ymax"] then
if x>=data["xmin"] and x<= data["xmax"] then
data["func"]()
data["active"] = not data["active"]
print(name)
end
end
end
end
function heading(text)
w, h = mon.getSize()
mon.setCursorPos((w-string.len(text))/2+1, 1)
mon.write(text)
end
fillTable()
while true do
mon.clear()
heading("Wither Skeleton Farm")
screen()
local e,side,x,y = os.pullEvent("monitor_touch")
checkxy(x,y)
sleep(.1)
end
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
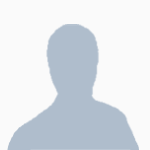
Advanced Monitor
Started by TechGuy427, 12 April 2013 - 05:48 AMPosted 12 April 2013 - 07:48 AM
This is Direwolf's slightly modified code for a button with computer craft, How can i configur it so when the Farm Control Button is Green the redstone signal out the back is on and when its light blue, its off? Answers much apreciated! x
Posted 12 April 2013 - 09:14 AM
Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:
I have not had the chance to test it but it should work :)/>
Here is an example code for a single button to do as you ask:
m = periheral.wrap("right")
m.setCursorPos(2,2)
m.setBackgroundColor(colors.blue)
m.write(" ")
m.setCursorPos(2,3)
m.write(" Farm ON ")
m.setCursorPos(2,4)
m.write(" ")
function button()
event, button, x, y = os.pullEvent("mouse_click")
end
if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then
rs.setOutput("<side>", true)
else
os.reboot()
end
function green()
m.setCursorPos(2,2)
m.setBackgroundColor(colors.green)
m.write(" ")
m.setCursorPos(2,3)
m.write(" Farm OFF ")
m.setCursorPos(2,4)
m.write(" ")
end
I have not had the chance to test it but it should work :)/>
Posted 12 April 2013 - 10:54 AM
Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
Here is my version of the revised code:
local monitor = peripheral.wrap("right")
local state = false --This will keep track of the redstone signal state. Also will control coloring.
local monX, monY = mon.getSize() --We'll need the monitor size for centering text '
local function drawButton()
mon.setBackgroundColor(colors.black) --Just reset the monitor color to black and clear the screen
mon.clear()
mon.setBackgroundColor(state and colors.blue or colors.green) --If the state is true, set the button color to blue. Otherwise, set it to green.
for yPos = -1, 1 do --This will just draw a background for the button that is 3 units high
mon.setCursorPos((monX/2 - 12), monY/2+yPos)
mon.write(string.rep(" ", 24))
end
local button_str = "Redstone output: "..tostring(state)
mon.setCursorPos((monX/2 - #button_str/2), monY/2)
mon.write(button_str)
end
while true do
drawButton()
rs.setOutput("back", state)
local e = {os.pullEvent()}
if e[1] == "monitor_touch" then
if e[3] >= (monX/2 - 12) and e[3] <= (monX/2 + 12) and e[4] <= (monY/2 + 1) and e[4] >= (monY/2 - 1) then --Just check to see if the click was within the boundaries of the button
state = not state
end
end
end
Posted 12 April 2013 - 10:56 AM
I know, I dont really like loops as the times I try them I often loop it so I cant terminate it or stop it :D/>Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
Posted 12 April 2013 - 11:07 AM
Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
Here is my version of the revised code:local monitor = peripheral.wrap("right") local state = false --This will keep track of the redstone signal state. Also will control coloring. local monX, monY = mon.getSize() --We'll need the monitor size for centering text ' local function drawButton() mon.setBackgroundColor(colors.black) --Just reset the monitor color to black and clear the screen mon.clear() mon.setBackgroundColor(state and colors.blue or colors.green) --If the state is true, set the button color to blue. Otherwise, set it to green. for yPos = -1, 1 do --This will just draw a background for the button that is 3 units high mon.setCursorPos((monX/2 - 12), monY/2+yPos) mon.write(string.rep(" ", 24)) end local button_str = "Redstone output: "..tostring(state) mon.setCursorPos((monX/2 - #button_str/2), monY/2) mon.write(button_str) end while true do drawButton() rs.setOutput("back", state) local e = {os.pullEvent()} if e[1] == "monitor_touch" then if e[3] >= (monX/2 - 12) and e[3] <= (monX/2 + 12) and e[4] <= (monY/2 + 1) and e[4] >= (monY/2 - 1) then --Just check to see if the click was within the boundaries of the button state = not state end end end
I know, I dont really like loops as the times I try them I often loop it so I cant terminate it or stop it :D/>Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
I don't think you understand, my monitor is only 3 blocks wide and 1 tall, so this dosen't work for starters and i want to the color of the button to be red on startup with no rs signal and when pressed turns to green and sends a rs signal out the back off the computer, sorry for not explaining well
Posted 12 April 2013 - 11:11 AM
I know, I dont really like loops as the times I try them I often loop it so I cant terminate it or stop it :D/>
That's easy to solve.
while true do
local e = {os.pullEvent()}
if e[1] == "key" and e[2] == keys.delete then --Insert whatever key you want there...
break
else
print("You can put anything you want here without ending the while loop")
end
end
print("You hit the delete key so I exited the loop!")
Alternatively you could just use ctrl+t which forcibly terminates programs.
Posted 12 April 2013 - 11:12 AM
:D/> It's easy to code; and once you know the basics, you can learn the more complicated stuff. I am creating a range of tutorials on my newly created blog on worpress. In ten minuets (when I have published the first tutorial) It will be linked in my signature; feel free to check it out!Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
Here is my version of the revised code:local monitor = peripheral.wrap("right") local state = false --This will keep track of the redstone signal state. Also will control coloring. local monX, monY = mon.getSize() --We'll need the monitor size for centering text ' local function drawButton() mon.setBackgroundColor(colors.black) --Just reset the monitor color to black and clear the screen mon.clear() mon.setBackgroundColor(state and colors.blue or colors.green) --If the state is true, set the button color to blue. Otherwise, set it to green. for yPos = -1, 1 do --This will just draw a background for the button that is 3 units high mon.setCursorPos((monX/2 - 12), monY/2+yPos) mon.write(string.rep(" ", 24)) end local button_str = "Redstone output: "..tostring(state) mon.setCursorPos((monX/2 - #button_str/2), monY/2) mon.write(button_str) end while true do drawButton() rs.setOutput("back", state) local e = {os.pullEvent()} if e[1] == "monitor_touch" then if e[3] >= (monX/2 - 12) and e[3] <= (monX/2 + 12) and e[4] <= (monY/2 + 1) and e[4] >= (monY/2 - 1) then --Just check to see if the click was within the boundaries of the button state = not state end end end
I know, I dont really like loops as the times I try them I often loop it so I cant terminate it or stop it :D/>Well, I find this code harder than necisary; for what you want to do. If you are only using one button most of this is not necisary.
Here is an example code for a single button to do as you ask:m = periheral.wrap("right") m.setCursorPos(2,2) m.setBackgroundColor(colors.blue) m.write(" ") m.setCursorPos(2,3) m.write(" Farm ON ") m.setCursorPos(2,4) m.write(" ") function button() event, button, x, y = os.pullEvent("mouse_click") end if button == 1 and x >= 2 and x <= 11 and y >= 2 and y <= 4 then rs.setOutput("<side>", true) else os.reboot() end function green() m.setCursorPos(2,2) m.setBackgroundColor(colors.green) m.write(" ") m.setCursorPos(2,3) m.write(" Farm OFF ") m.setCursorPos(2,4) m.write(" ") end
I have not had the chance to test it but it should work :)/>
If that os.reboot is for looping, then this is definitely not an efficient way to go about it. Use a while loop.
Also you should localize everything you can. It's a good habit to get into.
Note to the OP: I would not recommend using Direwolf's code as a prime example of how Lua should be written. He has good ideas, but the execution is lacking.
Thanks loads guys! This helped loads hopefully i will learn to be a good programmer in lua like you guys! :D/> Very Gratefull!!!!
Posted 12 April 2013 - 11:13 AM
I had to learn that after it happended…….I know, I dont really like loops as the times I try them I often loop it so I cant terminate it or stop it :D/>
That's easy to solve.while true do local e = {os.pullEvent()} if e[1] == "key" and e[2] == keys.delete then --Insert whatever key you want there... break else print("You can put anything you want here without ending the while loop") end end print("You hit the delete key so I exited the loop!")
Alternatively you could just use ctrl+t which forcibly terminates programs.
CTRL+T Didn't work in my circumstance.
Posted 12 April 2013 - 06:52 PM
Perhaps a loop wasn't yielding. It has to yield in order for it to handle an event.-snip-
CTRL+T Didn't work in my circumstance.
Posted 12 April 2013 - 09:42 PM
You can also use this to exit to loop:
while true do
local evt = {os.pullEventRaw()}
if evt[1] == "terminate" then
break
end
end
Posted 13 April 2013 - 04:30 AM
The loop had a os.reboot() so it stoped the memory of me holding Ctrl+t and it did this every second so I did not have a chance to terminate itPerhaps a loop wasn't yielding. It has to yield in order for it to handle an event.-snip-
CTRL+T Didn't work in my circumstance.