This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
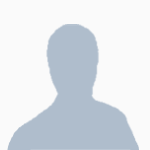
Deleting a certain line
Started by HurricaneCoder, 18 April 2013 - 04:16 PMPosted 18 April 2013 - 06:16 PM
I was wondering if there is a code that delete a line in a file that is open by io.open() or fs.open(). I already know that you can insert the line read into a table and remove it there but there is one problem. As soon as my server restart the computer just gonna run the program again and the table will return to it original state.
Posted 18 April 2013 - 06:24 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Edit: Or better yet, use unpack and write to the file like this:
Edit: Or better yet, use unpack and write to the file like this:
local content = { --You've got your table with the contents of the file in it like this
"line 1";
"line 2";
"line 3";
}
table.remove(content, 2) --You remove line 2
local f = fs.open("yourfile", "w")
f.write(unpack(content, "\n")) --This will unpack the table and put it into string form, with a newline character after each entry.
f.close()
Posted 18 April 2013 - 06:29 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
Posted 18 April 2013 - 06:32 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
You should do something like this:
1) Open the file with all the users. Read it line by line and insert it into a table.
2) If a new user is added, add it to the table and to the file
3) If a user is removed, delete it from the table and rewrite the content to the file, overwriting everything that is already in there.
Posted 18 April 2013 - 06:33 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Edit: Or better yet, use unpack and write to the file like this:local content = { --You've got your table with the contents of the file in it like this "line 1"; "line 2"; "line 3"; } table.remove(content, 2) --You remove line 2 local f = fs.open("yourfile", "w") f.write(unpack(content, "\n")) --This will unpack the table and put it into string form, with a newline character after each entry. f.close()
I am a noob at lua so can you explain to me what is \n like what it do because I only know "a" "r" and "w". Also what is unpack the table name content do. THX
Posted 18 April 2013 - 06:35 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
You should do something like this:
1) Open the file with all the users. Read it line by line and insert it into a table.
2) If a new user is added, add it to the table and to the file
3) If a user is removed, delete it from the table and rewrite the content to the file, overwriting everything that is already in there.
what do you mean rewrite the content to the file? Do I have to manually edit it and rewrite it?
Posted 18 April 2013 - 06:38 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Edit: Or better yet, use unpack and write to the file like this:local content = { --You've got your table with the contents of the file in it like this "line 1"; "line 2"; "line 3"; } table.remove(content, 2) --You remove line 2 local f = fs.open("yourfile", "w") f.write(unpack(content, "\n")) --This will unpack the table and put it into string form, with a newline character after each entry. f.close()
I am a noob at lua so can you explain to me what is \n like what it do because I only know "a" "r" and "r". Also what is unpack the table name content do. THX
Essentially, the \n sequence denotes that the file should begin writing content after the \n on the next line. It is not a file mode. Here is an example:
local f = fs.open("file", "w")
f.write("This is line 1\nThis is line 2\nThis is line 3")
f.close()
That'll create a file with three lines in it.What unpack() does is take an entire table and put it into string form, with the second argument you give it being the separator. For example:
local myTable = {
"This", "Is", "a", "table", "with", "stuff"
}
print(unpack(myTable, ", "))
--This prints out: This, is, a, table, with, stuff
--I could also do this:
print(unpack(myTable, " "))
--Which prints: This Is a table with stuff
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
You should do something like this:
1) Open the file with all the users. Read it line by line and insert it into a table.
2) If a new user is added, add it to the table and to the file
3) If a user is removed, delete it from the table and rewrite the content to the file, overwriting everything that is already in there.
what do you mean rewrite the content to the file? Do I have to manually edit it and rewrite it?
No. Opening a file in "w" mode will erase the contents of the file and allow you to add content from the start.
Posted 18 April 2013 - 06:43 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Edit: Or better yet, use unpack and write to the file like this:local content = { --You've got your table with the contents of the file in it like this "line 1"; "line 2"; "line 3"; } table.remove(content, 2) --You remove line 2 local f = fs.open("yourfile", "w") f.write(unpack(content, "\n")) --This will unpack the table and put it into string form, with a newline character after each entry. f.close()
I am a noob at lua so can you explain to me what is \n like what it do because I only know "a" "r" and "r". Also what is unpack the table name content do. THX
Essentially, the \n sequence denotes that the file should begin writing content after the \n on the next line. It is not a file mode. Here is an example:That'll create a file with three lines in it.local f = fs.open("file", "w") f.write("This is line 1\nThis is line 2\nThis is line 3") f.close()
What unpack() does is take an entire table and put it into string form, with the second argument you give it being the separator. For example:local myTable = { "This", "Is", "a", "table", "with", "stuff" } print(unpack(myTable, ", ")) --This prints out: This, is, a, table, with, stuff --I could also do this: print(unpack(myTable, " ")) --Which prints: This Is a table with stuff
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
You should do something like this:
1) Open the file with all the users. Read it line by line and insert it into a table.
2) If a new user is added, add it to the table and to the file
3) If a user is removed, delete it from the table and rewrite the content to the file, overwriting everything that is already in there.
what do you mean rewrite the content to the file? Do I have to manually edit it and rewrite it?
No. Opening a file in "w" mode will erase the contents of the file and allow you to add content from the start.
Oh wait your right I felt a little stupid. Turn out all I need was the "w" mode which I already know but I don't know how to use it in this scenario. After testing some code with "w" I got it. THX A LOT FOR YOUR HELP!!!
Posted 18 April 2013 - 06:47 PM
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Edit: Or better yet, use unpack and write to the file like this:local content = { --You've got your table with the contents of the file in it like this "line 1"; "line 2"; "line 3"; } table.remove(content, 2) --You remove line 2 local f = fs.open("yourfile", "w") f.write(unpack(content, "\n")) --This will unpack the table and put it into string form, with a newline character after each entry. f.close()
I am a noob at lua so can you explain to me what is \n like what it do because I only know "a" "r" and "r". Also what is unpack the table name content do. THX
Essentially, the \n sequence denotes that the file should begin writing content after the \n on the next line. It is not a file mode. Here is an example:That'll create a file with three lines in it.local f = fs.open("file", "w") f.write("This is line 1\nThis is line 2\nThis is line 3") f.close()
What unpack() does is take an entire table and put it into string form, with the second argument you give it being the separator. For example:local myTable = { "This", "Is", "a", "table", "with", "stuff" } print(unpack(myTable, ", ")) --This prints out: This, is, a, table, with, stuff --I could also do this: print(unpack(myTable, " ")) --Which prints: This Is a table with stuff
Can you not remove it from the table and then rewrite the table to the file using a for loop?
Well the thing here is I am creating a system password login and there is user and password that correspond to each other so I want to have the option delete user and add user. The add user I basically store the user name and pass word in a file using io.open(). So if I rewrite the table it would get all the file from the io.open() and write exactly the same thing there fore that username will just comeback again.
You should do something like this:
1) Open the file with all the users. Read it line by line and insert it into a table.
2) If a new user is added, add it to the table and to the file
3) If a user is removed, delete it from the table and rewrite the content to the file, overwriting everything that is already in there.
what do you mean rewrite the content to the file? Do I have to manually edit it and rewrite it?
No. Opening a file in "w" mode will erase the contents of the file and allow you to add content from the start.
Oh wait your right I felt a little stupid. Turn out all I need was the "w" mode which I already know but I don't know how to use it in this scenario. After testing some code with "w" I got it. THX A LOT FOR YOUR HELP!!!
One more thing to use \n do I have to be in the "\n" mode or the "w" mode?
Posted 18 April 2013 - 06:50 PM
One more thing to use \n do I have to be in the "\n" mode or the "w" mode?
There is no such thing as "\n" mode. There are only three file modes in ComputerCraft: "w", "r", and "a". You need to be in a writing mode ("w" or "a") in order to write things to a file and use "\n" as a line separator.
Posted 18 April 2013 - 07:16 PM
One more thing to use \n do I have to be in the "\n" mode or the "w" mode?
There is no such thing as "\n" mode. There are only three file modes in ComputerCraft: "w", "r", and "a". You need to be in a writing mode ("w" or "a") in order to write things to a file and use "\n" as a line separator.
I try using \n but it won't work it said number expected, got string so I am not so sure why it is expecting number. I use this code file:write(unpack(content,"\n")
Posted 18 April 2013 - 07:43 PM
One more thing to use \n do I have to be in the "\n" mode or the "w" mode?
There is no such thing as "\n" mode. There are only three file modes in ComputerCraft: "w", "r", and "a". You need to be in a writing mode ("w" or "a") in order to write things to a file and use "\n" as a line separator.
I try using \n but it won't work it said number expected, got string so I am not so sure why it is expecting number. I use this code file:write(unpack(content,"\n")
My apologies. I used the wrong function. Try this instead:
file.write(table.concat(content, "\n"))
Note that it is file.write, not file:write. The result will be two different things.