2 posts
Posted 24 April 2013 - 09:42 AM
Having multiple players in an if player == ?
This is my code
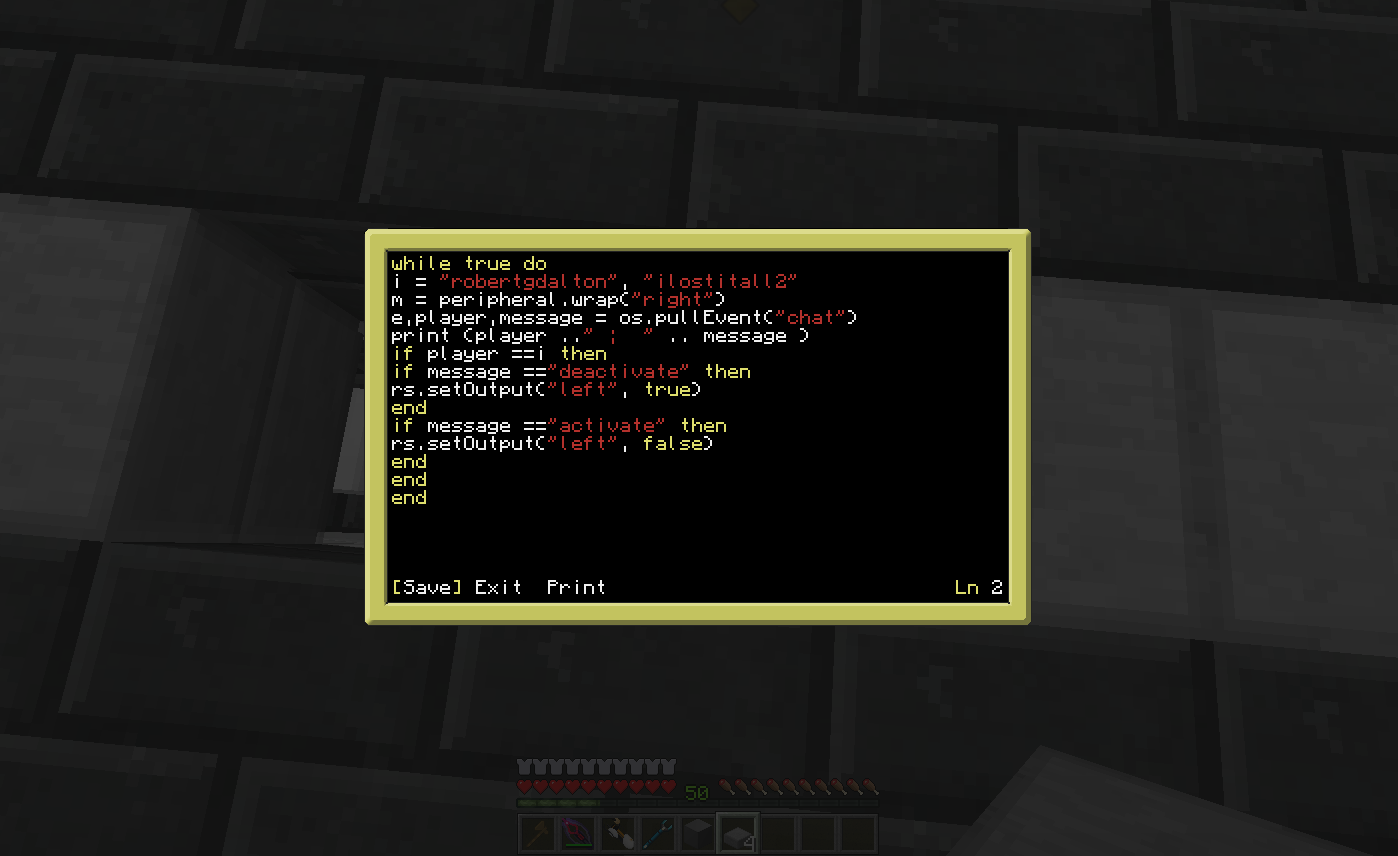
The first person in the variable works as in me but for ilostitall2 he can't activate the the rs output.
This is running on a mindcrack ftb server.
3790 posts
Location
Lincoln, Nebraska
Posted 24 April 2013 - 09:53 AM
Split to new topic.
You would actually want to define a new variable for the second player. What you would do is check if either player has entered their name.
i = "robertgdalton"
g = "ilositall2"
if player == i or player == g then
--success
end
2 posts
Posted 24 April 2013 - 10:05 AM
Split to new topic.
You would actually want to define a new variable for the second player. What you would do is check if either player has entered their name.
i = "robertgdalton"
g = "ilositall2"
if player == i or player == g then
--success
end
Works perfectly thank you.
My very limited lua knowledge has expanded thank you.
1583 posts
Location
Germany
Posted 24 April 2013 - 10:43 AM
If you want an easier way to add new names just use this:
local users = {
"Name1",
"Name2",
"Name3"
}
local input = read()
for i = 1, #users, 1 do
if input == users[i] then
--Do something
else
print"You aren't on the list"
end
end
If you want to add a new name, go into the users table and make a comma after the last entry then go into a new line and add a new name in quotation marks ^_^/>
92 posts
Posted 24 April 2013 - 10:46 AM
If you want an easier way to add new names just use this:
local users = {
"Name1",
"Name2",
"Name3"
}
local input = read()
for i = 1, #users, 1 do
if input == users[i] then
--Do something
else
print"You aren't on the list"
end
end
If you want to add a new name, go into the users table and make a comma after the last entry then go into a new line and add a new name in quotation marks ^_^/>
In a way, there's an even easier way to do that.
local people =
{
["Player1"] = true,
["Player2"] = false,
["Player3"] = true
}
local input = read()
if people[input] then
--Do something
else
print("Sorry, you're not on the list.")
end
1583 posts
Location
Germany
Posted 24 April 2013 - 10:51 AM
If you want an easier way to add new names just use this:
local users = {
"Name1",
"Name2",
"Name3"
}
local input = read()
for i = 1, #users, 1 do
if input == users[i] then
--Do something
else
print"You aren't on the list"
end
end
If you want to add a new name, go into the users table and make a comma after the last entry then go into a new line and add a new name in quotation marks ^_^/>/>
In a way, there's an even easier way to do that.
local people =
{
["Player1"] = true,
["Player2"] = false,
["Player3"] = true
}
local input = read()
if people[input] then
--Do something
else
print("Sorry, you're not on the list.")
end
There is always someone who can do it better than the previous :]
92 posts
Posted 24 April 2013 - 10:55 AM
If you want an easier way to add new names just use this:
local users = {
"Name1",
"Name2",
"Name3"
}
local input = read()
for i = 1, #users, 1 do
if input == users[i] then
--Do something
else
print"You aren't on the list"
end
end
If you want to add a new name, go into the users table and make a comma after the last entry then go into a new line and add a new name in quotation marks ^_^/>/>
In a way, there's an even easier way to do that.
local people =
{
["Player1"] = true,
["Player2"] = false,
["Player3"] = true
}
local input = read()
if people[input] then
--Do something
else
print("Sorry, you're not on the list.")
end
There is always someone who can do it better than the previous :]
Indeed. And I believe that's probably the most efficient way to do it, using only one IF statement. You could even implement it in a function, like so:
function isAllowed(text)
return (people[text] == true and "") or "Sorry, you're not allowed."
end
local people =
{
["Player1"] = true,
["Player2"] = false,
["Player3"] = true
}
local input = read()
print(isAllowed(input))
I think I did the AND/OR operators correctly… Not sure though.
1688 posts
Location
'MURICA
Posted 24 April 2013 - 12:26 PM
If you want to get even easier:
local allowed = "Player1 Player2 Player3"
local input = read()
if allowed:find(input) then
--success
end