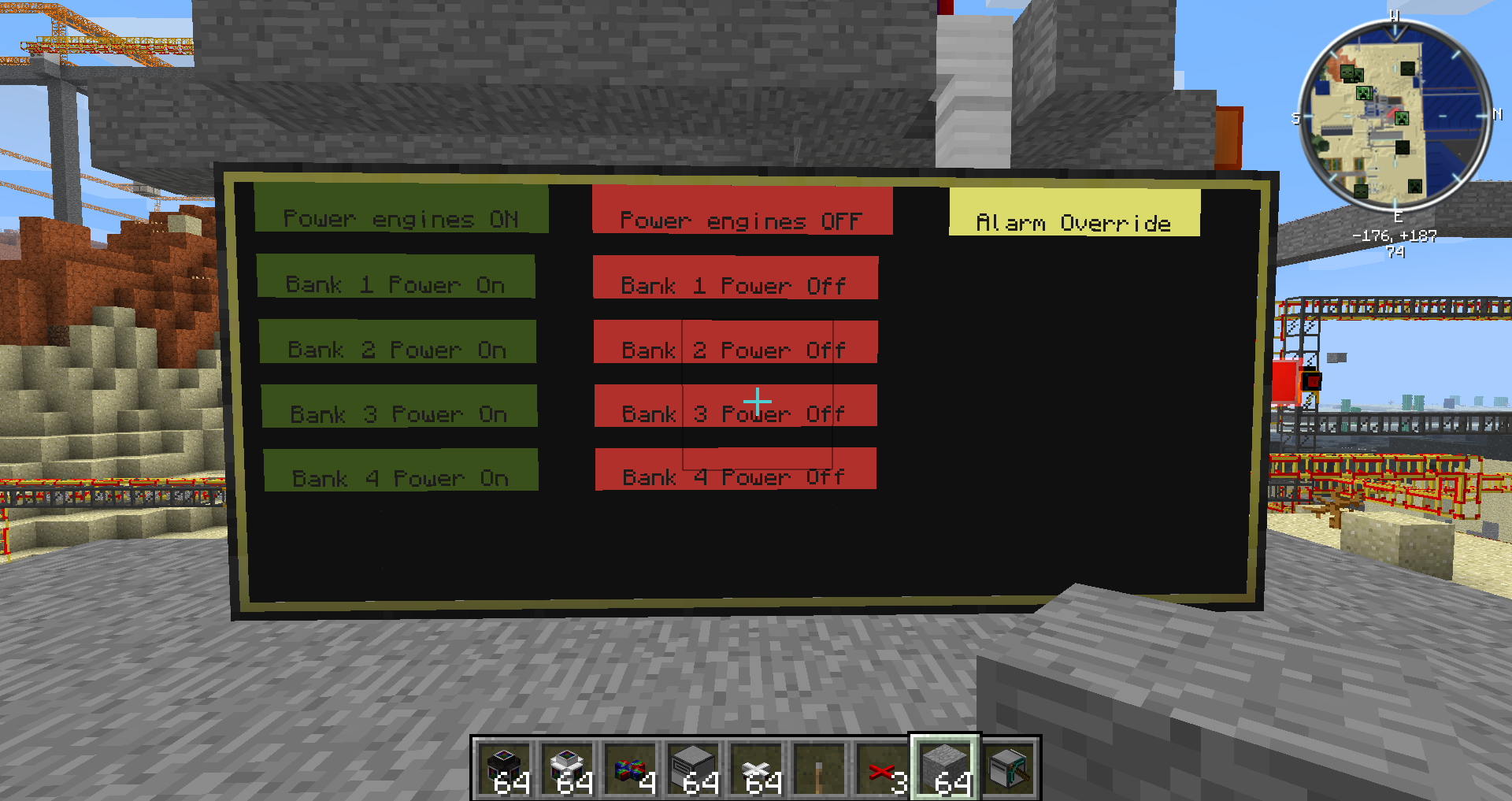
as you can see I finally have something working. I have it based off of
http://www.computercraft.info/forums2/index.php?/topic/11827-video-gui-tutorials-new-video-buttons-metatables/ full credit to Bubba
I'll paste my code in here incase someone has a multi engine bank system like i do.
--[[
You are free to use and distribute this code as your own: I claim no rights to it
This tutorial has a video associated with it, which can be found here: http://youtu.be/ppZMCAF7cWw
]]
mon = peripheral.wrap("back")
local button = { --Our main button table. Contains everything from button draw functions to the button information.
button_defaults = { --This is the metatable which we give set new buttons to. It provides color/size defaults.
__index = {
color_bg = colors.red;
color_cl = colors.green;
color_txt = colors.black;
height = 2;
padding = 2;
isClicked = false;
};
};
mt = { --This is the main metatable of the button table. It changes the behavior of the table to allow for calling and adding new indexes.
__call = function(self) --This allows us to call the table as if it were a function.
for index, btn in pairs(self.buttons) do
local color = btn.isClicked and btn.color_cl or btn.color_bg
mon.setBackgroundColor(color)
mon.setTextColor(btn.color_txt)
for yPos = btn.y, btn.bounds.y2 do
mon.setCursorPos(btn.x, yPos)
mon.write(string.rep(" ", btn.width))
end
local text = btn.isClicked and btn.clickText or btn.text
mon.setCursorPos(btn.x + (btn.width/2 - #text/2), btn.y + (btn.height/2))
mon.write(text)
end
end;
__newindex = function(t, key, value) --This changes the behavior of the table upon adding a new button
assert(type(value)=="table", "Requires a table") --assert will check that a condition is true; if it is not, it will error with the provided text
assert(value.x, "Requires initial x")
assert(value.y, "Requires initial y")
assert(value.text, "Requires text value")
setmetatable(value, t.button_defaults) --Give our new button its defaults with the __index metamethod
value.width = #value.text + (value.padding * 2)
value.bounds = {
x1 = value.x; --I don't use the x1 or y1 vars from the bounds table due to the fact that it's shorter to simply type btn.x than btn.bounds.x, but they are equal to the same thing (obviously)
y1 = value.y;
x2 = value.x + value.width - 1; --In order to draw and detect clicks correctly, you need to subtract 1 from the width and height.
y2 = value.y + value.height - 1;
}
t.buttons[key]=value --In the video, I am aware that I used rawset. However, it is actually not necessary because we are not changing the button table directly, but rather the button.buttons table (which has no newindex metamethod)
end;
};
checkClick = function(self, x,y) --This checks whether you have actually clicked on a table
for index, btn in pairs(self.buttons) do
if x>=btn.x and x<=btn.bounds.x2 and y>=btn.y and y<=btn.bounds.y2 then
btn.isClicked = true --If we have actually clicked the button then set its click value to true
if btn.onClick then --And check if it has an onClick function
btn:onClick() --If so, then execute it and pass the button's table/info into it by using the colon operator
end
return index --Return the index of the button so we can unhighlight it
end
end
end;
buttons = {}; --This is the table that we'll keep all the buttons in
}
setmetatable(button, button.mt) --Set the metatable of button to button.mt
button[1] = {
x = 2;
y = 1;
color_bg = colors.green;
color_cl = colors.red;
text = "Power engines ON";
clickText = "Power Packs on";
onClick = function(self)
rs.setBundledOutput("right",colors.combine(colors.red,colors.blue,colors.white,colors.green,colors.orange,colors.magenta,colors.lightBlue,colors.yellow,colors.lime,colors.pink,colors.gray,colors.lightGray,colors.cyan,colors.purple,colors.brown,colors.black))
rs.setBundledOutput("left", colors.green)
end;
}
button[2] = {
x = 25;
y = 1;
text = "Power engines OFF";
clickText = "Engines Halted";
onClick = function(self) --What is performed upon clicking the button
rs.setBundledOutput("right",0)
end;
}
button[3] = {
x = 50;
y = 1;
color_bg = colors.yellow;
color_cl = colors.red;
text = "Alarm Override";
clickText = "Alarm off. Check Engine";
onClick = function(self)
rs.setBundledOutput("left", 0)
end;
}
button[4] = {
x = 2;
y = 4;
color_bg = colors.green;
color_cl = colors.red;
text = "Bank 1 Power On";
clickText = "Bank 1 Powering up";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.combine( curBun, colors.red,colors.blue,colors.white,colors.green))
rs.setBundledOutput("left", colors.green)
end;
}
button[5] = {
x =2;
y = 7;
color_bg = colors.green;
color_cl = colors.red;
text = "Bank 2 Power On";
clickText = "Bank 2 Powering Up";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.combine( curBun, colors.orange,colors.magenta,colors.lightBlue,colors.yellow ))
rs.setBundledOutput("left", colors.green)
end;
}
button[6] = {
x =2;
y = 10;
color_bg = colors.green;
color_cl = colors.red;
text = "Bank 3 Power On";
clickText = "Bank 3 Powering Up";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.combine( curBun, colors.lime,colors.pink,colors.gray,colors.lightGray ))
rs.setBundledOutput("left", colors.green)
end;
}
button[7] = {
x =2;
y = 13;
color_bg = colors.green;
color_cl = colors.red;
text = "Bank 4 Power On";
clickText = "Bank 4 Powering Up";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.combine( curBun, colors.cyan,colors.purple,colors.brown,colors.black ))
rs.setBundledOutput("left", colors.green)
end;
}
button[8] = {
x = 25;
y = 4;
text = "Bank 1 Power Off";
clickText = "Bank 1 Halted";
onClick = function(self) --What is performed upon clicking the button
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right",colors.subtract( curBun,colors.red,colors.blue,colors.white,colors.green ))
end;
}
button[9] = {
x = 25;
y = 7;
text = "Bank 2 Power Off";
clickText = "Bank 2 Halted";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.subtract( curBun, colors.orange,colors.magenta,colors.lightBlue,colors.yellow))
end;
}
button[10] = {
x = 25;
y = 10;
text = "Bank 3 Power Off";
clickText = "Bank 3 Halted";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.subtract( curBun, colors.lime,colors.pink,colors.gray,colors.lightGray))
end;
}
button[11] = {
x = 25;
y = 13;
text = "Bank 4 Power Off";
clickText = "Bank 4 Halted";
onClick = function(self)
curBun = rs.getBundledOutput("right")
rs.setBundledOutput("right", colors.subtract( curBun, colors.cyan,colors.purple,colors.brown,colors.black))
end;
}
local timer = { --This will keep track of clicked buttons/the timers associated with them
index = false;
timer = false;
}
while true do
button() --Always draw the button first
local e = {os.pullEvent()} --Then pull our events
if e[1] == "monitor_touch" then
local index = button:checkClick(e[3], e[4]) --Check the click: make sure to pass the button table into the checkClick function
if index then
timer.index = index--The index of the button that is clicked
timer.timer = os.startTimer(1)
end
elseif e[1] == "timer" and e[2] == timer.timer then --If we get a timer event and the ID is equal to the timer.timer var then
button.buttons[timer.index].isClicked = false --Deselect the button
timer = {} --This is actually fairly memory inneficient, but for such a small program it doesn't really matter. Rather than do this though, you should probably just manually set the values of timer.index/timer.timer to false
mon.setBackgroundColor(colors.black)
mon.clear()
end
end