while true do
if x == 1 then
term.setCursorPos(0,0)
term.setBackGroundColor(colors.blue)
term.write("Wither Skeletons: "..wstat)
elseif x == not 1 then
term.setCursorPos(0,0)
term.setBackGroundColor(colors.black)
term.write("Wither Skeletons: "..wstat)
end
if x == 2 then
term.setCursorPos(0,1)
term.setBackGroundColor(colors.blue)
term.write("Zombie Pigmen: "..zpstat)
elseif x == not 2 then
term.setCursorPos(0,1)
term.setBackGroundColor(colors.black)
term.write("Zombie Pigmen: "..zpstat)
end
if x == 3 then
term.setCursorPos(0,2)
term.setBackGroundColor(colors.blue)
term.write("Blazes: "..blstat)
elseif x == not 3 then
term.setCursorPos(0,2)
term.setBackGroundColor(colors.black)
term.write("Blazes: "..blstat)
end
function up()
x = x+1
end
function down()
x = x-1
end
local event, key = os.pullEvent("key")
if key == 38 then
up()
end
if key == 40 then
down()
end
if x == 1 and key == 13 then
wsstat = not wsstat
rs.setOutput("back", wsstat)
elseif x == 2 and key == 13 then
zpstat = not zpstat
rs.setOutput("top", zpstat)
elseif x == 3 and key == 13 then
blstat = not blstat
rs.setOutput("left", blstat)
end
end
Mind helping?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
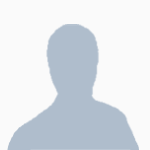
Mob Farm Program Help
Started by popdog15, 28 May 2013 - 05:28 PMPosted 28 May 2013 - 07:28 PM
Okay, so I have a mob farm set up for a number of things, (wither skeletons, zombie pigmen, blazes) to get their resources and xp. Simple turtle killing set up on an attack loop. Now, I've gotten it where I want to control each mob spawner separate. (Soul shard mob spawners.) I'm trying to make it in a way where I can select which mob I want to turn on via up and down arrow keys (keys 38 and 40) and hit enter (key 13) to put out the redstone signal to the back. The screen stays blank whenever I try to run this.
Posted 28 May 2013 - 07:49 PM
"if x == not 1 then" won't work (it'll always be treated as false). Use "if x ~= 1 then" instead.
You've got your functions defined inside your "while" loop. Get them out of there, ideally up to the top of your code.
You've got your functions defined inside your "while" loop. Get them out of there, ideally up to the top of your code.
Posted 28 May 2013 - 08:05 PM
I've done that, and it still doesn't work. It runs the program, but doesn't execute any write lines or anything. Not waiting for events. Just puts me back at the command line where I can run a program."if x == not 1 then" won't work (it'll always be treated as false). Use "if x ~= 1 then" instead.
You've got your functions defined inside your "while" loop. Get them out of there, ideally up to the top of your code.
Posted 28 May 2013 - 09:06 PM
Well, I don't see any way for it to break out of your old "while" loop without at least giving you an error. You might want to link to the current version of your code.
Posted 28 May 2013 - 09:38 PM
Alrighty. Current code is here: http://pastebin.com/gHaRvdtu
Posted 28 May 2013 - 09:52 PM
I still don't see how that would return to the command line, but I also don't see how that'll print anything.
You were using:
I told you to use:
You're now using:
"~=" means "is not equal to". Under other languages, you might use "!=" or "<>" for this.
Another valid (and less redundant) way to go about it would be to simply not bother checking if "x isn't a value".
Is exactly the same as:
Because the "elseif" statement in the first example will only be processed if x is not 1, there's no point in specifically telling it to make that check again.
You were using:
if x == not 1 then
I told you to use:
if x ~= 1 then
You're now using:
if x ~= not 1 then
"~=" means "is not equal to". Under other languages, you might use "!=" or "<>" for this.
Another valid (and less redundant) way to go about it would be to simply not bother checking if "x isn't a value".
if x == 1 then
-- Some code
elseif x != 1 then
-- Some other code
end
Is exactly the same as:
if x == 1 then
-- Some code
else
-- Some other code
end
Because the "elseif" statement in the first example will only be processed if x is not 1, there's no point in specifically telling it to make that check again.
Posted 28 May 2013 - 10:30 PM
Changed it, still not working. It's hard to describe what it does, it clears the screen of all writeable characters, but they're "ghost characters" like I can write over them and such. I changed the term.write's to prints, still not doing anything.
(http://pastebin.com/REpPpqRu)
Oh, and a change that's not in the code is that I made the values blstat, zpstat, and wsstat true when the program is run.
(In the pastebin code, that is.)
(http://pastebin.com/REpPpqRu)
Oh, and a change that's not in the code is that I made the values blstat, zpstat, and wsstat true when the program is run.
(In the pastebin code, that is.)
Posted 29 May 2013 - 12:08 PM
Add term.clear() at the top of your while loop- after line 10 http://pastebin.com/REpPpqRu
http://computercraft.info/wiki/Term.clear
Since you print the whole screen every time, that should take care of your 'ghost characters'. =)
http://computercraft.info/wiki/Term.clear
Since you print the whole screen every time, that should take care of your 'ghost characters'. =)
Posted 29 May 2013 - 05:31 PM
Thanks, but doesn't fix the whole problem :/Add term.clear() at the top of your while loop- after line 10 http://pastebin.com/REpPpqRu
http://computercraft...wiki/Term.clear
Since you print the whole screen every time, that should take care of your 'ghost characters'. =)
Posted 29 May 2013 - 10:49 PM
Still not printing anything as stated it should… still need help :c
Posted 29 May 2013 - 11:02 PM
Ok, so I built my first "advanced" computer and gave it a shot.
"term.setCursorPos(0,0)" is invalid; the top left of the screen is actually at "term.setCursorPos(1,1)". You'd expect it to give you an error, but instead it causes the program to break without error, which is worse… :/
Another problem you'll run in to is that you can't add strings and booleans together in your print statements. Instead use something like:
This allows you to evaluate the value of "wstat" and return a value (a string, in this case) based on whether it's true or not.
While I was at it, I condensed your program to make troubleshooting easier. This is what I ended up with, if you're interested.
It could be condensed further with some numerically indexed tables and some "for" loops. I don't want to make things too confusing by demonstrating that at this point, but let me know if you're interested.
"term.setCursorPos(0,0)" is invalid; the top left of the screen is actually at "term.setCursorPos(1,1)". You'd expect it to give you an error, but instead it causes the program to break without error, which is worse… :/
Another problem you'll run in to is that you can't add strings and booleans together in your print statements. Instead use something like:
print("Wither Skeletons: "..(wsstat and "On" or "Off"))
This allows you to evaluate the value of "wstat" and return a value (a string, in this case) based on whether it's true or not.
While I was at it, I condensed your program to make troubleshooting easier. This is what I ended up with, if you're interested.
local x = 1
local wsstat,zpstat,blstat = false
term.clear()
while true do
term.setCursorPos(1,1)
term.setBackgroundColor((x==1) and colors.blue or colors.black)
print("Wither Skeletons: "..(wsstat and "On " or "Off"))
term.setBackgroundColor((x==2) and colors.blue or colors.black)
print("Zombie Pigmen: "..(zpstat and "On " or "Off"))
term.setBackgroundColor((x==3) and colors.blue or colors.black)
print("Blazes: "..(blstat and "On " or "Off"))
event, key = os.pullEvent("key")
if key == 208 then -- Down key pressed
x = x + 1
if x > 3 then x = 1 end
end
if key == 200 then -- Up key pressed
x = x - 1
if x < 1 then x = 3 end
end
if key == 28 or key == 57 then -- Enter or space keys pressed
if x == 1 then
wsstat = not wsstat
rs.setOutput("back", wsstat)
elseif x == 2 then
zpstat = not zpstat
rs.setOutput("top", zpstat)
elseif x == 3 then
blstat = not blstat
rs.setOutput("left", blstat)
end
end
end
It could be condensed further with some numerically indexed tables and some "for" loops. I don't want to make things too confusing by demonstrating that at this point, but let me know if you're interested.
Posted 29 May 2013 - 11:22 PM
An alternative to using Lua's ternary, you could also do thisAnother problem you'll run in to is that you can't add strings and booleans together in your print statements. Instead use something like:print("Wither Skeletons: "..(wstat and "On" or "Off"))
print("Wither Skeletons: "..tostring(wstat))
which would print out as:`Wither Skeletons: true`
or
`Wither Skeletons: false`
either way really. just illuminating the fact that there is a tostring function.
Posted 29 May 2013 - 11:24 PM
Good to know it works on bools, thanks. :)/>
Though with that you'd want to add an extra .." " to avoid stray characters hanging around the display.
Though with that you'd want to add an extra .." " to avoid stray characters hanging around the display.
Posted 30 May 2013 - 08:13 PM
Why thank you! I appreciate all the help I've received. c: