This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
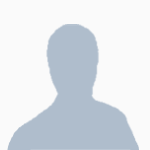
change computer ID?
Started by mikesaa309, 15 June 2013 - 07:05 AMPosted 15 June 2013 - 09:05 AM
if you have a computer set up to send something over rednet to say a computer with the ID 13, how would you write a code to be able to change the ID it sends to so you could change the specified computer to send it to? I ask this because i'm making a computercraft controlled train station and may need to change the id to which a computer sends to and also i'm going to place the finished program in the main lua programs folder inside the mod zip file so i can place any computer down and launch the program but i'd need to be able to change the id and you can't edit the code if its in the main program bit in the zip folder.
Posted 15 June 2013 - 09:44 AM
You can't change the ID of a computer. You can, however, use the Modem API and use different channels. http://computercraft.info/wiki/Modem_(API)
Posted 15 June 2013 - 10:13 AM
sorry to sound like a noob (which i am) but how would you implement it in to this code:
–Variables
local stationName = ""
local modemSide = someSide
local rsSide = someSide
local frequence = someFrequence
–Initialisation
rednet.open("left")
–Functions
print("press enter to enter/change station name")
local function waitForKeypress()
while true do
local sEvent, sKey = os.pullEvent()
if sEvent == "key" and sKey == keys.enter then
term.write("Station Name: ")
stationName = read()
end
end
end
local function waitForRs()
while true do
os.pullEvent("redstone")
if rs.getInput("top") then
rednet.send(0, "A train is at "..stationName)
else rednet.send(0, "train has left "..stationName)
end
sleep(0)
end
end
–Main function
local function main()
parallel.waitForAny(waitForKeypress, waitForRs)
end
–BSoD
local _,err = pcall(main)
if err then
term.clear()
term.setCursorPos(1,3)
print("exit")
print("\n\npress any key to exit…")
while true do
local sEvent = os.pullEvent()
if sEvent == "key" then
term.clear()
term.setCursorPos(1, 1)
error()
end
end
end
–Variables
local stationName = ""
local modemSide = someSide
local rsSide = someSide
local frequence = someFrequence
–Initialisation
rednet.open("left")
–Functions
print("press enter to enter/change station name")
local function waitForKeypress()
while true do
local sEvent, sKey = os.pullEvent()
if sEvent == "key" and sKey == keys.enter then
term.write("Station Name: ")
stationName = read()
end
end
end
local function waitForRs()
while true do
os.pullEvent("redstone")
if rs.getInput("top") then
rednet.send(0, "A train is at "..stationName)
else rednet.send(0, "train has left "..stationName)
end
sleep(0)
end
end
–Main function
local function main()
parallel.waitForAny(waitForKeypress, waitForRs)
end
–BSoD
local _,err = pcall(main)
if err then
term.clear()
term.setCursorPos(1,3)
print("exit")
print("\n\npress any key to exit…")
while true do
local sEvent = os.pullEvent()
if sEvent == "key" then
term.clear()
term.setCursorPos(1, 1)
error()
end
end
end
Posted 15 June 2013 - 10:30 AM
A method which doesn't involve learning more about events (something you should do anyway, but whatever…):
When your program starts, have your remote terminals use rednet.broadcast() to send a message, and then immediately have them go into receive mode. Have the server machine rigged to recognise that message (use a certain passphrase, eg "I want the train server's ID!") and, in response, send a message back.
Once it receives that response your client/terminal machine then has the server's ID stored in a variable and can simply use that from then on (taking care not to overwrite it with something else later). In this way, you (the programmer) never need to know the IDs of your machines, or care whether they change - they'll work it all out at run-time.
In the long run, learning how to get the modem API to work will see you better off - it can do everything the rednet API can do, and more.
When your program starts, have your remote terminals use rednet.broadcast() to send a message, and then immediately have them go into receive mode. Have the server machine rigged to recognise that message (use a certain passphrase, eg "I want the train server's ID!") and, in response, send a message back.
Once it receives that response your client/terminal machine then has the server's ID stored in a variable and can simply use that from then on (taking care not to overwrite it with something else later). In this way, you (the programmer) never need to know the IDs of your machines, or care whether they change - they'll work it all out at run-time.
In the long run, learning how to get the modem API to work will see you better off - it can do everything the rednet API can do, and more.
Posted 15 June 2013 - 10:54 AM
is there any tutorials on the modem api? i tried using it in this code:
rednet.open("left")
mod.open(1)
term.clear()
term.setCursorPos(1, 1)
while true do
local id, message, distance = rednet.receive()
print(message)
sleep(1)
end
but didn't work it said "attempt to index ? a nil value". I've obviously entered it in wrong but from the wiki this is how i gathered you receive something using the modem api. this is the code on a receiving computer btw.
rednet.open("left")
mod.open(1)
term.clear()
term.setCursorPos(1, 1)
while true do
local id, message, distance = rednet.receive()
print(message)
sleep(1)
end
but didn't work it said "attempt to index ? a nil value". I've obviously entered it in wrong but from the wiki this is how i gathered you receive something using the modem api. this is the code on a receiving computer btw.
Posted 15 June 2013 - 11:27 AM
ok so i looked at the tutorial you sent me an did this to the code but it still gives an error but because its a BSoD it doesn't say what error:
–Variables
local stationName = ""
local modemSide = someSide
local rsSide = someSide
local frequence = someFrequence
–Initialisation
rednet.open("left")
–Functions
print("press enter to enter/change station name")
local function waitForKeypress()
while true do
local sEvent, sKey = os.pullEvent()
if sEvent == "key" and sKey == keys.enter then
term.write("Station Name: ")
stationName = read()
end
end
end
local function waitForRs()
while true do
os.pullEvent("redstone")
if rs.getInput("back") then
rednet.send(18, "A train is at "..stationName)
else rednet.send(18, "train has left "..stationName)
end
sleep(0)
end
end
–Main function
local function main()
parallel.waitForAny(waitForKeypress, waitForRs)
end
–BSoD
local _,err = pcall(main)
if err then
term.clear()
term.setCursorPos(1,3)
print("Some bad error has occured D:\n\n")
print(" " .. tostring(err) .. " ")
print("\n\npress any key to exit…")
while true do
local sEvent = os.pullEvent()
if sEvent == "key" then
term.clear()
term.setCursorPos(1,1)
print(os.version() .. "\n\n")
end
end
–Variables
local stationName = ""
local modemSide = someSide
local rsSide = someSide
local frequence = someFrequence
–Initialisation
rednet.open("left")
–Functions
print("press enter to enter/change station name")
local function waitForKeypress()
while true do
local sEvent, sKey = os.pullEvent()
if sEvent == "key" and sKey == keys.enter then
term.write("Station Name: ")
stationName = read()
end
end
end
local function waitForRs()
while true do
os.pullEvent("redstone")
if rs.getInput("back") then
rednet.send(18, "A train is at "..stationName)
else rednet.send(18, "train has left "..stationName)
end
sleep(0)
end
end
–Main function
local function main()
parallel.waitForAny(waitForKeypress, waitForRs)
end
–BSoD
local _,err = pcall(main)
if err then
term.clear()
term.setCursorPos(1,3)
print("Some bad error has occured D:\n\n")
print(" " .. tostring(err) .. " ")
print("\n\npress any key to exit…")
while true do
local sEvent = os.pullEvent()
if sEvent == "key" then
term.clear()
term.setCursorPos(1,1)
print(os.version() .. "\n\n")
end
end
Posted 15 June 2013 - 11:32 AM
I put comments on the code that was missing
EDIT: btw to do that ^^^ with the code use [code][/code] tags
local stationName = ""
local modemSide = someSide
local rsSide = someSide
local frequence = someFrequence
rednet.open("left")
print("press enter to enter/change station name")
local function waitForKeypress()
while true do
local sEvent, sKey = os.pullEvent()
if sEvent == "key" and sKey == keys.enter then
term.write("Station Name: ")
stationName = read()
end
end
end
local function waitForRs()
while true do
os.pullEvent("redstone")
if rs.getInput("back") then
rednet.send(18, "A train is at "..stationName)
else
rednet.send(18, "train has left "..stationName)
end
sleep(0)
end
end
local function main()
parallel.waitForAny(waitForKeypress, waitForRs)
return true --# you missed this
end
local ok, err = pcall(main)
if not ok and err ~= "Terminated" then
term.clear()
term.setCursorPos(1,3)
print("Some bad error has occured D:\n\n")
print(" " .. tostring(err) .. " ")
print("\n\npress any key to exit...")
while true do
local sEvent = os.pullEvent()
if sEvent == "key" then
term.clear()
term.setCursorPos(1,1)
print(os.version() .. "\n\n")
end
end
end --# you missed this end
EDIT: btw to do that ^^^ with the code use [code][/code] tags
Edited on 15 June 2013 - 09:33 AM
Posted 15 June 2013 - 02:52 PM
how do I code it so that i can change it to send to a different computer other than id 18 without editing the code?
Posted 15 June 2013 - 03:18 PM
Just use read() to get an answer before you send the message.