992 posts
Posted 20 April 2012 - 11:28 AM
I looking for advice on how to best implement word wrap around.
so this is the program so far It works ok but all the words are being cut off on the ends.
also any improvements i could make would be greatly appreciated.
http://pastebin.com/raw.php?i=YjXzaq35
378 posts
Location
In the TARDIS
Posted 20 April 2012 - 11:50 AM
Just add n between. That creates a new line
992 posts
Posted 20 April 2012 - 11:56 AM
the point is it will scale with the screens size while allowing it to be scrolled with up/down keys or else I would have just used print()
new line will not help.
Pictures showing scroll
Spoiler
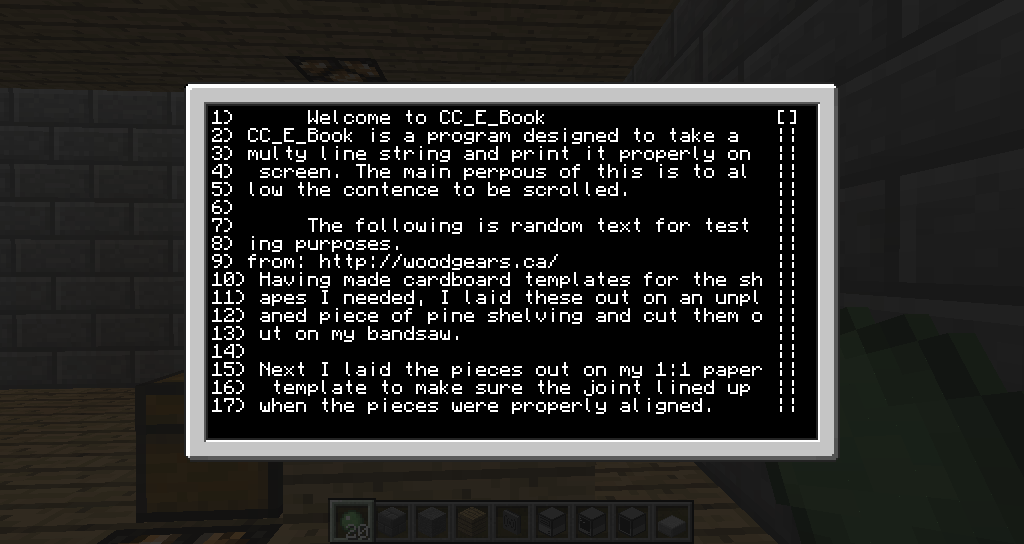
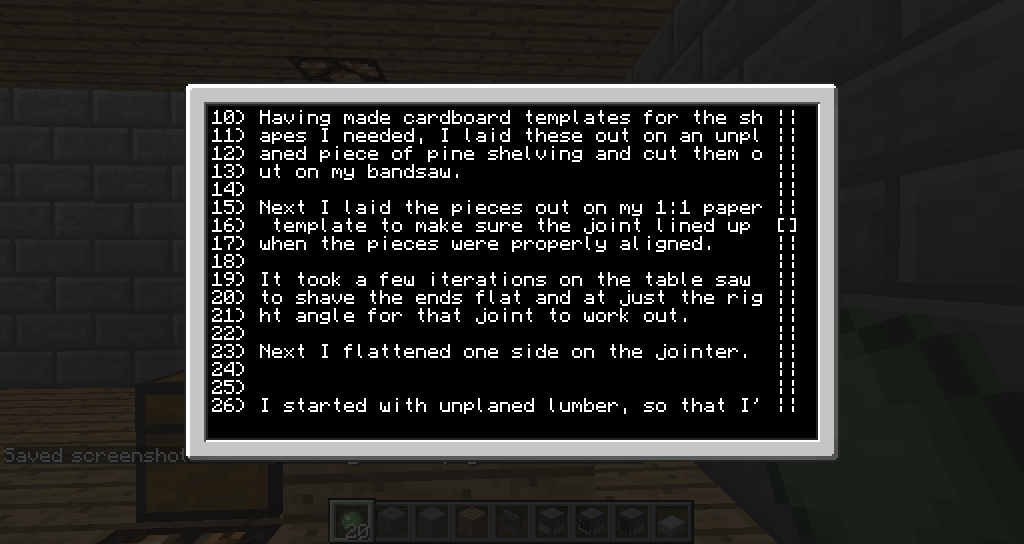
193 posts
Posted 20 April 2012 - 12:33 PM
Spoiler
function write( sText )
local w,h = term.getSize()
local x,y = term.getCursorPos()
local nLinesPrinted = 0
local function newLine()
if y + 1 <= h then
term.setCursorPos(1, y + 1)
else
term.scroll(1)
term.setCursorPos(1, h)
end
x, y = term.getCursorPos()
nLinesPrinted = nLinesPrinted + 1
end
-- Print the line with proper word wrapping
while string.len(sText) > 0 do
local whitespace = string.match( sText, "^[ t]+" )
if whitespace then
-- Print whitespace
term.write( whitespace )
x,y = term.getCursorPos()
sText = string.sub( sText, string.len(whitespace) + 1 )
end
local newline = string.match( sText, "^n" )
if newline then
-- Print newlines
newLine()
sText = string.sub( sText, 2 )
end
local text = string.match( sText, "^[^ tn]+" )
if text then
sText = string.sub( sText, string.len(text) + 1 )
if string.len(text) > w then
-- Print a multiline word
while string.len( text ) > 0 do
if x > w then
newLine()
end
term.write( text )
text = string.sub( text, (w-x) + 2 )
x,y = term.getCursorPos()
end
else
-- Print a word normally
if x + string.len(text) > w then
newLine()
end
term.write( text )
x,y = term.getCursorPos()
end
end
end
return nLinesPrinted
end
Thats write function with proper word wrapping.
Its found in bios.lua.
You can look at how its does it then modify your own code with code from that :)/>/>
992 posts
Posted 20 April 2012 - 12:39 PM
Spoiler
function write( sText )
local w,h = term.getSize()
local x,y = term.getCursorPos()
local nLinesPrinted = 0
local function newLine()
if y + 1 <= h then
term.setCursorPos(1, y + 1)
else
term.scroll(1)
term.setCursorPos(1, h)
end
x, y = term.getCursorPos()
nLinesPrinted = nLinesPrinted + 1
end
-- Print the line with proper word wrapping
while string.len(sText) > 0 do
local whitespace = string.match( sText, "^[ t]+" )
if whitespace then
-- Print whitespace
term.write( whitespace )
x,y = term.getCursorPos()
sText = string.sub( sText, string.len(whitespace) + 1 )
end
local newline = string.match( sText, "^n" )
if newline then
-- Print newlines
newLine()
sText = string.sub( sText, 2 )
end
local text = string.match( sText, "^[^ tn]+" )
if text then
sText = string.sub( sText, string.len(text) + 1 )
if string.len(text) > w then
-- Print a multiline word
while string.len( text ) > 0 do
if x > w then
newLine()
end
term.write( text )
text = string.sub( text, (w-x) + 2 )
x,y = term.getCursorPos()
end
else
-- Print a word normally
if x + string.len(text) > w then
newLine()
end
term.write( text )
x,y = term.getCursorPos()
end
end
end
return nLinesPrinted
end
Thats write function with proper word wrapping.
Its found in bios.lua.
You can look at how its does it then modify your own code with code from that :)/>/>
i will look into that
Thanks it looks like it will be able to do what i want it to. after a few modifications
378 posts
Location
In the TARDIS
Posted 20 April 2012 - 03:33 PM
OK Good to hear that you managed it. And you can place functions IN functions?!?
161 posts
Posted 21 April 2012 - 04:39 AM
Wolvan: Lua functions are first-class. A function actually doesn't have a name inherently; it's just a value which can be assigned to a name. This:
function f()
…
end
is actually equivalent to this:
f = function()
…
end
and you can also (as you discovered) create functions inside other functions, assign them into variables, pass them as parameters, return them from function calls, use them as values in tables, and so on. Basically everything from "function()" to "end" is a single giant expression.