task main()
{
motor[left] = 127;
motor[right] = 127;
wait1Msec(5000);
motor[left] = 0;
motor[right] = 0;
}
You can probably tell it makes the robot go forwards for 5 seconds (127 is full power to motors). The problem is that in ComputerCraft, you can make a turtle go forwards one block with a simple line of code. Is there any way to change the turtle API so that you can have something similar to the c code above? For example, if the left and right motors are both on 127, the turtle will go forward indefinitely; If one is reversed, it will spin indefinitely. Can I accomplish this by adding a peripheral? Decompiling (not sure if possible/allowed) and editing the mod? Re-writing bios.lua? Any help would be appreciated. I'm not too worried about lack of things like task main(), curly braces, semicolons, and parentheses in lua as these are easy to teach later. I will add that I can understand Java and Lua, but I am a bit finicky when it comes to programming it (I have only made a bukkit plugin and basic turtle scripts for my FTB world).
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
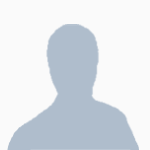
Changing turtle movement system
Started by tunasushi, 29 June 2013 - 11:07 PMPosted 30 June 2013 - 01:07 AM
I want to teach kids in my robotics class about how to code robots using ComputerCraft turtles. The real code is written in RobotC (basically c) and the most basic code is the following:
Posted 01 July 2013 - 12:46 PM
Split into new topic.
This is essentially impossibleto modify ComputerCraft to do. ComputerCraft's turtles exist as blocks, which means that they are locked into positions on the coordinate grid. The implications of the changes you're talking about would allow for non-right-angle movements and rotations, which would mean you'd need an entity. You would be better off creating your own mod to gain that sort of functionality, I think. A peripheral could be written to create and control those entities, but adapting ComputerCraft itself is likely to not be a viable path.
You might also try contacting dan200 to present your question/idea directly. He has worked on some educational-related projects.
This is essentially impossibleto modify ComputerCraft to do. ComputerCraft's turtles exist as blocks, which means that they are locked into positions on the coordinate grid. The implications of the changes you're talking about would allow for non-right-angle movements and rotations, which would mean you'd need an entity. You would be better off creating your own mod to gain that sort of functionality, I think. A peripheral could be written to create and control those entities, but adapting ComputerCraft itself is likely to not be a viable path.
You might also try contacting dan200 to present your question/idea directly. He has worked on some educational-related projects.
Posted 01 July 2013 - 05:47 PM
I know that turtles are blocks and they can't move to inbetween spaces, but what I was asking was for the turtle to do something like move forward infinitely if it's motors are on. From what it sounds like, my easiest option would be writing an OS on top of the default one that interprets the code and feeds normal move commands to lua. The current problem is that I have no idea how. Would anyone be willing to help me, or should I just spend the next few days learning more lua? Thanks for the help.
Posted 01 July 2013 - 10:42 PM
[indent=1]In order to help you I need a little more information. All you want to do is make the turtle go forwards/ backwards/ spin based upon the input of the motors? And am I correct in assuming that 0 is the motors not running, and that if positive numbers are forward then negative numbers would be reverse?[/indent]
Posted 02 July 2013 - 01:12 AM
Ok so it is possible by making some modifications to `bios.lua` and to the turtle API file in `rom`. Feeling a bit generous today, so I've provided an example…
Replace contents of `<cc zip file>/rom/apis/turtle/turtle` with this: http://pastebin.com/zjNLW0f4
NOTE: I've checked code for syntax, but since I'm using CCDesk I could not run on a turtle, logic is sound though, just may require a few tweaks.
At the bottom of the `bios.lua` file replace the following code
Now all your students need to do is modify the motor values to be either 0 or 127, to be able to move forward or rotate left and right (I've assumed that there is no backwards, not hard to add though if there is).
NOTE: For your students to modify they must use motor.left, motor.right, motor["left"], or motor["right"], and are not able to use any of the turtle API as the code is removed by the above program WHEN the turtle is first turned on (this is why I got you to put it in `<cc zip file>/rom/apis/turtle/turtle`)
NOTE 2: There could potentially be a way to be able to only have the code run when the values are changed (maybe, I'm having a bit of a brain derp today, but I think there should be a way to detect the changing of values in a table). If possible it would remove the need to have a loop running (the bit added to `bios.lua`) and should be made to call the moveTurtle function when those values have been modified.
Replace contents of `<cc zip file>/rom/apis/turtle/turtle` with this: http://pastebin.com/zjNLW0f4
NOTE: I've checked code for syntax, but since I'm using CCDesk I could not run on a turtle, logic is sound though, just may require a few tweaks.
At the bottom of the `bios.lua` file replace the following code
-- Run the shell
local ok, err = pcall( function()
parallel.waitForAny(
function()
os.run( {}, "rom/programs/shell" )
end,
function()
rednet.run()
end )
end )
With this
-- Run the shell
local ok, err = pcall( function()
parallel.waitForAny(
function()
os.run( {}, "rom/programs/shell" )
end,
function()
rednet.run()
end,
function()
turtle.run() --# this line will run the turtle movement loop
end )
end )
Now all your students need to do is modify the motor values to be either 0 or 127, to be able to move forward or rotate left and right (I've assumed that there is no backwards, not hard to add though if there is).
NOTE: For your students to modify they must use motor.left, motor.right, motor["left"], or motor["right"], and are not able to use any of the turtle API as the code is removed by the above program WHEN the turtle is first turned on (this is why I got you to put it in `<cc zip file>/rom/apis/turtle/turtle`)
NOTE 2: There could potentially be a way to be able to only have the code run when the values are changed (maybe, I'm having a bit of a brain derp today, but I think there should be a way to detect the changing of values in a table). If possible it would remove the need to have a loop running (the bit added to `bios.lua`) and should be made to call the moveTurtle function when those values have been modified.
Posted 02 July 2013 - 01:48 AM
Wow. Thanks! Won't get to try it till tomorrow, but my hopes have already skyrocketed! I will post again once I have tested it.
Posted 02 July 2013 - 02:12 AM
Ok so after a chat with KaoS ( lately known to me as BITs–derp–fixer :P/> ) the auto movement when they change the motor value is definitely possible, and quite easy to do. However I'm not currently at home, so I'll post the new solution when I get home (unless Pastebin for iPhone app decides to be nice to me, in that event I'll have the better solution for your use case posted soon :)/> )
Posted 02 July 2013 - 11:41 AM
Ok so, according to the info I got from KaoS this should be fine, it does not require the `bios.lua` to be modified, only the turtle API… When the motor value is changed it will present itself on the turtle… much better suited to your scope… Again this is untested for the turtles, but is tested for syntax errors and there may be a few logic errors… http://pastebin.com/bGYrZgvc
Posted 02 July 2013 - 04:48 PM
I've spent the last hour trying to get the above solution to work. After installing it, I tried a simple program called "go":
Lastly, just to make sure I installed correctly, do I replace /lua/rom/apis/turtle/turtle with the latest paste bin code you gave me, and just recompile it? I didn't change anything else. As always, thanks for the help!
motor.left = 127
motor.right = 127
I refueled, but I got:
refuel:15: attempt to call nil
I then tried to refuel through the lua shell and got this:
lua:52: attempt to call nil
Then, I turned off the need for fuel in the config and still nothing happened when I tried to use the go program. The odd thing is that no error messages were thrown with the program, it just did nothing. I tried running run("forward") in the lua shell and got this again:
lua:52: attempt to call nil
Lastly, just to make sure I installed correctly, do I replace /lua/rom/apis/turtle/turtle with the latest paste bin code you gave me, and just recompile it? I didn't change anything else. As always, thanks for the help!
Posted 02 July 2013 - 08:34 PM
Oh yeh I should have told you that you do need to turn off fuel… the refuelling is done through turtle.refuel and turtle.native.refuel, which in the new API I removed, meaning you would never be able to do it…I refueled, but I got:I then tried to refuel through the lua shell and got this:refuel:15: attempt to call nil
lua:52: attempt to call nil
Another option is after the line turtle = nil add in something like turtle.refuel = native.refuel then you will be able to refuel again.
That is because the run function I localised to the API, so nothing else would be able to access it.Then, I turned off the need for fuel in the config and still nothing happened when I tried to use the go program. The odd thing is that no error messages were thrown with the program, it just did nothing. I tried running run("forward") in the lua shell and got this again:lua:52: attempt to call nil
Yes that is the correct file. Unzip, replace, rezip, that should be all that is needed to be done.Lastly, just to make sure I installed correctly, do I replace /lua/rom/apis/turtle/turtle with the latest paste bin code you gave me, and just recompile it? I didn't change anything else.
I'll test it now in Minecraft for you…
Posted 03 July 2013 - 01:11 AM
Thanks for clearing that up. But I still don't get why the turtle wasn't moving… I'll look into it again tomorrow when I'm back at my computer. Sorry about my lack of lua knowledge!
Posted 03 July 2013 - 02:27 AM
No problems, I wouldn't be a very good help if I didn't :P/>Thanks for clearing that up.
Ugh, I do……………. I'll try and figure out what's going wrong now and post it up when I have a solution.But I still don't get why the turtle wasn't moving…
That's fine… We all have to start somewhere…Sorry about my lack of lua knowledge!