any help would be much apreiciated
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
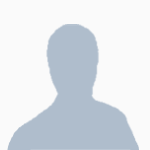
assigning words in an input to arrays
Started by thebadgerherder1, 03 July 2013 - 10:42 AMPosted 03 July 2013 - 12:42 PM
i was trying to create a somewhat intelligent way of controlling my base using a chatbox peripheral. However i wanted to make it so that it would only acknolege the command if the first word of my message was one perticular word like computer, so that my other messages would suddenly start messing with my base. I would like to assign each word of the message from the "chat" event to an array such that if the message was "computer turn the lights on" array[1] would be computer, array[2] would be turn, ect.
any help would be much apreiciated
any help would be much apreiciated
Posted 03 July 2013 - 01:15 PM
Split into new topic.
The usual way of doing this is:
The usual way of doing this is:
local inputString
local commandArray = {}
for match in string.gmatch(inputString, "([^ \t]+)") do
table.insert(commandArray, match)
end
Posted 03 July 2013 - 03:26 PM
Another ways you could do it is:
local inputstring
local array = {}
for word in string.gmatch(inputstring,"[^%s]+") do
table.insert(array,word)
end
Posted 03 July 2013 - 06:59 PM
%S would work in place of ^%s. I used the pattern I did because that's what gets used in ComputerCraft's shell.run (or perhaps os.run, I can't recall) to split arguments.
Posted 04 July 2013 - 10:09 AM
Thanks, your code is much simpler than the method i was trying to use. I was also wondering (however this isnt strictly related to the topic) how i would detect if one specific word was used anywhere in the input. my current code is pastebin.com/uzi34GWh, but it replies to the chat message regardless of whether its name is used in the message preceeding it.
Posted 04 July 2013 - 10:35 AM
To search a string for a specific word or phrase use string.find
make sure that you do terminate any special characters with % because string.find does use patterns.
str = "hello world, Lua can be good from time-to-time!"
if str:find("good") then
print("Good was found")
else
print("Good was not found")
end
make sure that you do terminate any special characters with % because string.find does use patterns.
Posted 04 July 2013 - 10:40 AM
Thanks for the help
Posted 04 July 2013 - 01:06 PM
I wasn't sure about this, but I looked it up, and you can safely pass any string to string.find and it won't be interpreted as a pattern string. (Hope you don't mind me jacking your example, BIT :P/> )
Where the 1 is where the search starts, and "true" is to tell the function to use a plain string. http://www.lua.org/m...pdf-string.find
In this specific example, it probably would be easier to just escape the dashes, but it's helpful in the event that you don't actually know what word you're trying to find, e.g. in some sort of a searching algorithm where the user inputs keywords.
str = "hello world, Lua can be good from time-to-time!"
if str:find("time-to-time", 1, true) then
print("'time-to-time' was found")
else
print("'time-to-time' was not found")
end
Where the 1 is where the search starts, and "true" is to tell the function to use a plain string. http://www.lua.org/m...pdf-string.find
In this specific example, it probably would be easier to just escape the dashes, but it's helpful in the event that you don't actually know what word you're trying to find, e.g. in some sort of a searching algorithm where the user inputs keywords.
Posted 04 July 2013 - 08:09 PM
Really? I tried it before I posted it, and I swear that it didn't like things like `.` and `+`, maybe I made a mistake in my code…. And I definitely know you can use patterns in it, for example with my code doing "[good]" would actually trigger if any of those letters appeared in the string.I wasn't sure about this, but I looked it up, and you can safely pass any string to string.find and it won't be interpreted as a pattern string. (Hope you don't mind me jacking your example, TOB :P/>/> )
Side note: How many times have I told you Kingdaro, its either TOBIT or BIT (latter preferred)
Posted 05 July 2013 - 02:25 AM
That's because of the "true" parameter you can pass to the function. Under normal circumstances, yes, it would use a pattern string.